予備知識
xdel(winsid());
clear;
clearglobal;
※行末の..
は継続行です
いきなりExample
SciLab ヘルプのGUIの uicontorol のExampleを試してみる
f=figure(0); // (window ID 0 を指定し)図を作成
// 位置を指定して、GUIオブジェクト(リストボックス)を作成
// figure fのstyleをlistboxに、positionをx:10,y:10,w:150,h:160
h=uicontrol(f, 'style','listbox', 'position',[10 10 150 160]);
// リストの値を設定する
set(h, 'string', "item 1|item 2|item3");
ここまで実行するとグラフィックウィンドウ0の左下にitem 1からitem 3までのリストボックスが表示される。
例ではこのあと要素の1と3を選択状態にするのですが、エラーになります。
set(h, 'value', [1 3]);// リストで要素1と3を選択
item 1とitem 3を選択済みにするためのコードだが、先にValueプロパティで設定できる最大値をMaxプロパティにセットしておかないといけない。
set(h, 'Max',3);
set(h, 'value', [1 3]);// リストで要素1と3を選択
//close(f);
使ってみた
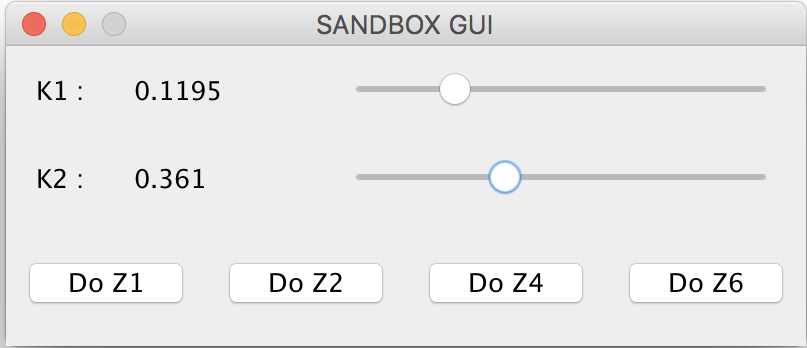
//********************************************************************
// CLEAN
//********************************************************************
xdel(winsid());
clear;
clearglobal;
GUI部品をクリックしたときに呼び出すコールバック関数を定義する。cb_slide_K1()は、スライダーK1をスライドしたときの処理。"SL_K1"のタグがついたGUI部品の"value"の値を読み込み、文字列に変換したものを"K1"タグのついたGUI部品(テキスト)の"string"にセットする。
cb_doit(dim)は、K1,K2の"string"をよみとり、引数dimに指定した処理を行う。
********************************************************************
// GUI FUNCTIONS
//********************************************************************
function cb_slide_K1()
k1 = get("SL_K1", "value");
set("K1", "string", string(k1));
endfunction
function cb_slide_K2()
k2 = get("SL_K2", "value");
set("K2", "string", string(k2));
endfunction
function cb_doit(dim)
k1 = evstr(get("K1", "string"));
k2 = evstr(get("K2", "string"));
select dim
case 1 then
//...
case 2 then
//...
else
end
//...
endfunction
ウインドウにフレームを2つセットする。
********************************************************************
// GUI
//********************************************************************
// TOP WINDOW
sb_top_fig=figure(...
"dockable", "off", "infobar_visible", "off", ...
"toolbar_visible", "off", "toolbar", "none", ...
"menubar_visible", "off", "menubar", "none", ...
"layout", "none", "resize", "off", ...
"visible", "off", ...
"figure_position", [0 0], ...
"axes_size", [400, 150], ...
"figure_name", "SANDBOX GUI", ...
"layout", "gridbag", ...
"tag", "SANDBOX_top_figure");
// FRAME
// MEMO
// GRIDBAG では createConstraints("gridbag", grid, weight, fill, anchor, padding)
// grid [pos_x, pos_y, width, height]
// weight [weight_x, weight_y]
// fill {"none"} | "horizontal" | "vertical" | "both"
// anchor {"center"} | "upper" | "upper_right" | "right" | "lower_right" | "lower" | "lower_left" | "left" | "upper_left"
sb_vars_frame = uicontrol(sb_top_fig, ...
"layout", "gridbag", ...
"style", "frame", ...
"constraints", createConstraints("gridbag", [1, 1, 1, 1], [1, 0.5], "both"));
1つ目には、文字"K1"と値を表示するテキストと、スライダーで構成させるGUI部品を2セットセット.
// VAR1 K1
uicontrol(sb_vars_frame, ...
"style", "text", ...
"string", "K1 : ", ...
"constraints", createConstraints("gridbag", [1, 1, 1, 1], [0.5, 1], "horizontal", "center"), ...
"margins", [5 5 5 5], ...
"horizontalAlignment", "center");
uicontrol(sb_vars_frame, ...
"style", "text", ...
"string", "0.1", ...
"constraints", createConstraints("gridbag", [2, 1, 1, 1], [1, 1], "horizontal", "center"), ...
"tag", "K1", ...
"margins", [5 5 5 5]);
uicontrol(sb_vars_frame, ...
"Style" , "slider", ...
"String" , "Start", ...
"margins", [5 5 5 5], ...
"Max",0.5, ...
"tag", "SL_K1", ...
"callback" , "cb_slide_K1()", ...
"constraints", createConstraints("gridbag", [3, 1, 1, 1], [1, 1], "horizontal", "center"));
// VAR2 K2
uicontrol(sb_vars_frame, ...
"style", "text", ...
"string", "K2 : ", ...
"constraints", createConstraints("gridbag", [1, 2, 1, 1], [0.5, 1], "horizontal", "center"), ...
"margins", [5 5 5 5], ...
"Max",0.2, ...
"horizontalAlignment", "center");
uicontrol(sb_vars_frame, ...
"style", "text", ...
"string", "0.05", ...
"constraints", createConstraints("gridbag", [2, 2, 1, 1], [1, 1], "horizontal", "center"), ...
"tag", "K2", ...
"margins", [5 5 5 5]);
uicontrol(sb_vars_frame, ...
"Style" , "slider", ...
"String" , "Start", ...
"margins", [5 5 5 5], ...
"tag", "SL_K2", ...
"callback" , "cb_slide_K2()", ...
"constraints", createConstraints("gridbag", [3, 2, 1, 1], [1, 1], "horizontal", "center"));
2つめのフレームには、ボタンを4つわりあてる。
//slider frame
action_frame = uicontrol(sb_top_fig, ...
"layout", "gridbag", ...
"style", "frame", ...
"constraints", createConstraints("gridbag", [1, 2, 1, 1], [1, 1], "both"));
//"border", createBorder("line","navy blue"), ...
// The associated callback needs to be interruptible (when clicking on stop or reset for example)
uicontrol("parent", action_frame, ...
"Style" , "pushbutton", ...
"String" , "Do Z1", ...
"margins", [5 5 5 5], ...
"callback" , "cb_doit(1)", ...
"constraints", createConstraints("gridbag", [1, 1, 1, 1], [1, 1], "horizontal", "center"));
uicontrol("parent", action_frame, ...
"Style" , "pushbutton", ...
"String" , "Do Z2", ...
"margins", [5 5 5 5], ...
"callback" , "cb_doit(2)", ...
"constraints", createConstraints("gridbag", [2, 1, 1, 1], [1, 1], "horizontal", "center"));
uicontrol("parent", action_frame, ...
"Style" , "pushbutton", ...
"String" , "Do Z4", ...
"margins", [5 5 5 5], ...
"callback" , "cb_doit(4)", ...
"constraints", createConstraints("gridbag", [3, 1, 1, 1], [1, 1], "horizontal", "center"));
uicontrol("parent", action_frame, ...
"Style" , "pushbutton", ...
"String" , "Do Z6", ...
"margins", [5 5 5 5], ...
"callback" , "cb_doit(6)", ...
"constraints", createConstraints("gridbag", [4, 1, 1, 1], [1, 1], "horizontal", "center"));
完成したので表示する
sb_top_fig.visible = "on";
##SciLab関連ドキュメントリンク
install
macOSでSciLabを動かすまで
SciNoteのオートコンプリートをとめたい
実践
SciLabでCSV読み込み
SciLabでGUIを使ってみる
Mac/Windows共用scilabスクリプト未公開
SciLabでフィルタを適用する未公開
プロットメモ未公開
プロットあれこれ未公開
他の言語と比較・書き換え
Scilab (NumPy) - plotの書き方
[配列と文字列処理の比較](https://qiita.com/0x20FE/items/ b6ea768692297b721951)
関数とオブジェクトの書き方メモ
Scilab,NumPy,R行列処理の比較