2024年8月追記
本記事のパワーアップバージョンを電子書籍としてzennにて公開しました。
あわせてご確認いただけますと幸いです。
前回の記事が思いのほか好評だったので、今回はPythonの基礎を図解にまとめてみました。
これからPythonに入門する方、初学者の方への参考になれれば幸いです。
各分野で関連記事のリンクが貼ってある場合があります。詳しく知りたい方はそちらをご活用ください。
前回の記事↓
押さえたい基礎
押さえたい基礎の分野は9つになります。
以下で詳しく見ていきます。
数値計算
数値の型(int・float)
# 整数
print(type(1))
# 小数
print(type(1.0))
<class 'int'>
<class 'float'>
演算子一覧
# x + y
print(1 + 2)
# x - y
print(2 - 1)
# x * y
print(2 * 3)
# x / y
print(10 / 5)
# x // y
print(7 // 3)
# x % y
print(7 % 3)
# x ** y
print(3 ** 2)
3
1
6
2.0
2
1
9
文字列
文字列の型(str)
# str("文字列")
print(type("Python"))
<class 'str'>
インデックスとスライス
word = "Python"
# インデックス
print(word[0])
# スライス
print(word[1:5])
P
ytho
エスケープシーケンス
print("こんにちは\n世界")
print("こんにちは\t世界")
print('doesn\'t')
こんにちは
世界
こんにちは 世界
doesn't
ちなみにエスケープシーケンスを認識して欲しくないときは、raw文字列を使います。
文字列の先頭にrかRを付けます。
# raw文字列
print(r"こんにちは\n世界")
こんにちは\n世界
関連記事
f-string
year = 2023
place = 'Tokyo'
print(f'I was born in {place} in {year}')
I was born in Tokyo in 2023
関連記事
str.format
year = 2023
place = 'Tokyo'
print('I was born in {} in {}'.format(place, year))
I was born in Tokyo in 2023
f-stringはPython3.6以降に追加された機能になります。
str.formatよりスッキリ書けるというメリットがあります。
条件分岐
Pythonにおける条件分岐は、if、elif、elseになります。
if・elif・else
num = -1
if num < 0:
print("負の数です。")
elif num == 0:
print("0です。")
else:
print("0より大きいです。")
負の数です。
関数
関数は必要な時に何度でも呼び出すことができるので、同じコードを何回も書かなくて済みます。
仮引数と実引数
# 仮引数:length
# 実引数:5
def test_func(length):
square = length**2
print(square)
test_func(5)
25
キーワード引数と位置引数
# キーワード引数
def test_func(name, old, address):
print("名前は" + name + "です。")
print("年齢は" + str(old) + "です。")
print("住所は" + address + "です。")
test_func(address="大阪", name="山田", old=30)
名前は山田です。
年齢は30です。
住所は大阪です。
# 位置引数
def test_func(name, old, address):
print("名前は" + name + "です。")
print("年齢は" + str(old) + "です。")
print("住所は" + address + "です。")
test_func("山田", 30, "大阪")
名前は山田です。
年齢は30です。
住所は大阪です。
関連記事
return
def test_func(length):
square = length**2
return square
result = test_func(8)
print(result)
64
lambda式
add_lambda = lambda a, b: a + b
add_lambda(5,4)
# 使わない場合
# def add_def(a, b):
# return a + b
# add_def(5,4)
9
ビルトイン関数(一部を紹介)
len関数
# len関数(オブジェクトの長さを返す)
len("python")
6
関連記事
type関数
# type関数(オブジェクトの型を返す)
type("python")
str
関連記事
dir関数
# dir関数(引数の属性をリストで返す)
dir(list)
['__add__',
'__class__',
'__class_getitem__',
'__contains__',
'__delattr__',
'__delitem__',
'__dir__',
'__doc__',
'__eq__',
'__format__',
'__ge__',
'__getattribute__',
'__getitem__',
'__gt__',
'__hash__',
'__iadd__',
'__imul__',
'__init__',
'__init_subclass__',
'__iter__',
'__le__',
'__len__',
'__lt__',
'__mul__',
'__ne__',
'__new__',
'__reduce__',
'__reduce_ex__',
'__repr__',
'__reversed__',
'__rmul__',
'__setattr__',
'__setitem__',
'__sizeof__',
'__str__',
'__subclasshook__',
'append',
'clear',
'copy',
'count',
'extend',
'index',
'insert',
'pop',
'remove',
'reverse',
'sort']
関連記事
その他の組み込み関数はこちらをご確認ください。
ループ処理
ループ処理の基本と便利な機能を押さえます。
for
word_lists = ["Python","Java","C++"]
for word in word_lists:
print(word)
Python
Java
C++
while
num = 1
while num < 5:
print(num)
num += 1
1
2
3
4
range
for i in range(5):
print(i)
0
1
2
3
4
関連記事
break
for i in range(1, 6):
if i == 3:
break
print(i)
1
2
continue
for num in range(2, 6):
if num % 2 == 0:
print(str(num)+"は偶数です")
continue
print(str(num)+"は奇数です")
2は偶数です
3は奇数です
4は偶数です
5は奇数です
enumerate
word_lists = ["Python","Java","C++"]
for i, word in enumerate(word_lists):
print(i, word)
0 Python
1 Java
2 C++
関連記事
else
for i in range(5):
print(i)
else:
print("終わり")
0
1
2
3
4
終わり
zip
num_lists = [1, 2, 3]
word_lists = ["Python","Java","C++"]
for num, word in zip(num_lists, word_lists):
print(num, word)
1 Python
2 Java
3 C++
要素の数が一致しない場合、多い分は無視されます。
num_lists = [1, 2]
word_lists = ["Python","Java","C++"]
for num, word in zip(num_lists, word_lists):
print(num, word)
1 Python
2 Java
関連記事
データ格納オブジェクト
リスト
# 角カッコの中にカンマ区切りの値を入れていく
sample_list=[1,2,3,4,5]
# インデックス操作・スライス操作が使える
print(sample_list[0])
print(sample_list[2:4])
# 連結ができる
print(sample_list + [6,7,8,9,10])
# 様々なメソッドがある
# 末尾にアイテムを1つ追加する
sample_list.append(98)
print(sample_list)
# 末尾にイテラブルを追加する
sample_list.extend([99, 100])
print(sample_list)
# 指定された位置にアイテムを挿入する
sample_list.insert(3, 10)
print(sample_list)
# リストから全てのアイテムを削除する
sample_list.clear()
print(sample_list)
1
[3, 4]
[1, 2, 3, 4, 5, 6, 7, 8, 9, 10]
[1, 2, 3, 4, 5, 98]
[1, 2, 3, 4, 5, 98, 99, 100]
[1, 2, 3, 10, 4, 5, 98, 99, 100]
[]
タプル
# カンマで区切られた値からなる
t1 = 10, "a", [1,2]
print(t1)
# 入れ子構造ができる
t2 = t1, (5, "b", 7)
print(t2)
# アイテムが0のタプル
empty_tuple = ()
print(empty_tuple)
# アイテムが1のタプル
single_tuple = "hello",
print(single_tuple)
# タプルはイミュータブルである(値を改変できない)
t1[0] = 5
(10, 'a', [1, 2])
((10, 'a', [1, 2]), (5, 'b', 7))
()
('hello',)
TypeError: 'tuple' object does not support item assignment
入力の際は囲みの丸カッコはなくても可
(式の一部として使っている場合は必要)
辞書
# (キー(key): 値(value) のペア)
d = {'key1': 1, 'key2': 2, 'key3': 3}
# delで削除が可能
del d["key1"]
print(d)
#「キー = 新しい値」で辞書内容を変更できる
d["key2"] = 20
print(d)
# 辞書にないキーを指定した場合は追加される
d["key4"] = 4
print(d)
# キーを指定すると値を取り出すことができる
print(d["key3"])
# 空の辞書を生成
empty_dict = {}
print(empty_dict)
{'key2': 2, 'key3': 3}
{'key2': 20, 'key3': 3}
{'key2': 20, 'key3': 3, 'key4': 4}
3
{}
集合
# {}またはset()関数を使用する
sample_set1 = {"s", "w", "r", "t", "o", "a", "w","f","o"}
print(sample_set1)
sample_set2 = set("absbdfsabaa")
print(sample_set2)
{'r', 'a', 't', 's', 'o', 'w', 'f'}
{'a', 'b', 's', 'd', 'f'}
集合の演算
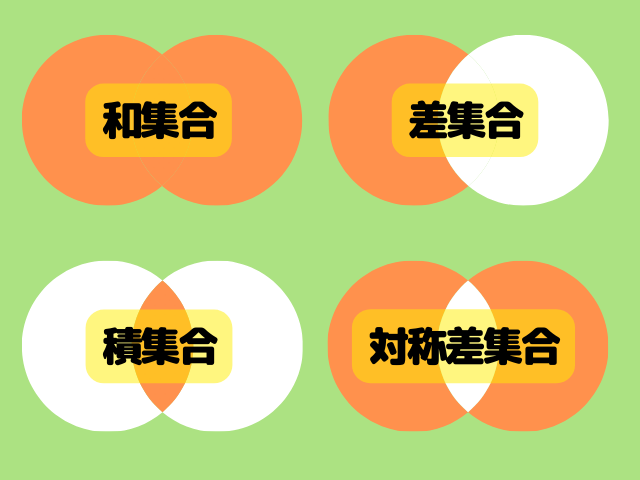
# 和集合
print(sample_set1 | sample_set2)
# 差集合
print(sample_set1 - sample_set2)
# 積集合
print(sample_set1 & sample_set2)
# 対称差集合
print(sample_set1 ^ sample_set2)
{'a', 't', 's', 'o', 'w', 'b', 'r', 'd', 'f'}
{'r', 't', 'w', 'o'}
{'a', 's', 'f'}
{'r', 'd', 't', 'b', 'w', 'o'}
関連記事
例外処理
例外
1/0
ZeroDivisionError: division by zero
構文エラー
# 構文エラー(閉じかっこがない)
print("Hello World!"
SyntaxError: incomplete input
その他の組み込み例外はこちらをご確認ください。
try・except
try:
1 / 0
except ZeroDivisionError:
print("ゼロで割り算できません!")
ゼロで割り算できません!
finally
try:
1 / 0
except ZeroDivisionError:
print("0で割り算はできません")
finally:
print("プログラムを終了します")
0で割り算はできません
プログラムを終了します
else
try:
1 / 0
except ZeroDivisionError:
print("0で割り算はできません")
else:
print("プログラムを終了します")
0で割り算はできません
try:
1 / 1
except ZeroDivisionError:
print("0で割り算はできません")
else:
print("プログラムを終了します")
プログラムを終了します
raise
>>> raise SyntaxError
Traceback (most recent call last):
File "<stdin>", line 1, in <module>
SyntaxError: None
from
>>> raise OSError from ZeroDivisionError
ZeroDivisionError
The above exception was the direct cause of the following exception:
Traceback (most recent call last):
File "<stdin>", line 1, in <module>
OSError
with文
ファイルオブジェクトが即座に正しくクリーンアップされることを保証します。
➝たとえ処理中に問題があったとしても、常にcloseされます。
>>> with open("sample.txt") as f:
... for line in f:
... print(line, end="")
...
あいうえお
かきくけこ
さしすせそ>>>
>>> f.closed
True
モジュール
モジュール・パッケージ・ライブラリの関係性
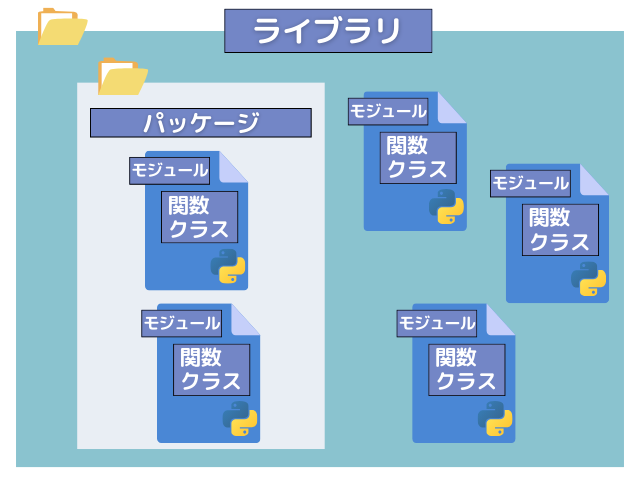
import・from・as
def square(num):
return num**2
def cube(num):
return num**3
# import
import calc
print(calc.square(2))
# from
from calc import cube
print(cube(3))
# as
import calc as cl
print(cl.cube(4))
4
27
64
ちなみにモジュールは以下の優先順位でインポートされます。
- 組み込みモジュール
- カレントディレクトリ
- PYTHONPATH
- インストールごとのデフォルト
1はsys.builtin_module_names
、2~4はsys.path
で確認することができます。
>>> import sys
>>> sys.builtin_module_names
('_abc', '_ast', '_bisect', '_blake2', '_codecs', '_codecs_cn', '_codecs_hk', '_codecs_iso2022', '_codecs_jp', '_codecs_kr', '_codecs_tw', '_collections', '_contextvars', '_csv', '_datetime', '_functools', '_heapq', '_imp', '_io', '_json', '_locale', '_lsprof', '_md5', '_multibytecodec', '_opcode', '_operator', '_pickle', '_random', '_sha1', '_sha256', '_sha3', '_sha512', '_signal', '_sre', '_stat', '_statistics', '_string', '_struct', '_symtable', '_thread', '_tracemalloc', '_warnings', '_weakref', '_winapi', '_xxsubinterpreters', 'array', 'atexit', 'audioop', 'binascii', 'builtins', 'cmath', 'errno', 'faulthandler', 'gc', 'itertools', 'marshal', 'math', 'mmap', 'msvcrt', 'nt', 'parser', 'sys', 'time', 'winreg', 'xxsubtype', 'zlib')
>>> import sys
>>> sys.path
['',
'C:\\Users\\User\\Desktop',
'C:\\Users\\User\\anaconda3\\python38.zip',
'C:\\Users\\User\\anaconda3\\DLLs',
'C:\\Users\\User\\anaconda3\\lib',
'C:\\Users\\User\\anaconda3',
'C:\\Users\\User\\AppData\\Roaming\\Python\\Python38\\site-packages',
'C:\\Users\\User\\anaconda3\\lib\\site-packages',
'C:\\Users\\User\\anaconda3\\lib\\site-packages\\locket-0.2.1-py3.8.egg',
'C:\\Users\\User\\anaconda3\\lib\\site-packages\\win32',
'C:\\Users\\User\\anaconda3\\lib\\site-packages\\win32\\lib',
'C:\\Users\\User\\anaconda3\\lib\\site-packages\\Pythonwin']
PYTHONPATHは環境変数から設定することができます。
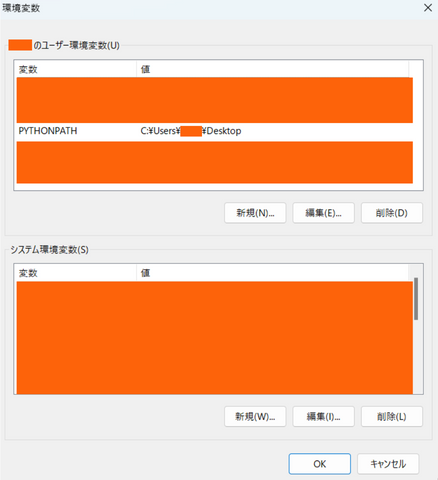
クラス
クラス・インスタンスのイメージ
クラスの基本構造
class Person:
# メソッド
# __init__に初期化処理を書く。第一引数はself
def __init__(self, name):
self.name = name
def get_name(self):
return self.name
# インスタンス生成
n = Person('tanaka')
# 属性(get_name())
n.get_name()
tanaka
オーバーライド
class Person:
def __init__(self, name):
self.name = name
def hello(self):
print('Hello,', str(self.name))
p = Person("tanaka")
p.hello()
Hello, tanaka
class Student(Person):
def hello(self):
print('Hello!!!', str(self.name))
s = Student("suzuki")
s.hello()
Hello!!! suzuki
プライベート変数
class Person:
def __init__(self, name):
self.__name = name
p = Person("sato")
p.__name
AttributeError: 'Person' object has no attribute '__name'
class Person:
def __init__(self, name):
self.__name = name
p = Person("sato")
p._Person__name
sato
おわりに
最後までお読みいただきありがとうございました。
25年1月追記
本記事の理解度をチェックできる「Pyhton基礎 100本ノック」を執筆しました。
挑戦お待ちしています!
勉強方法にお困りの方はこちらの記事もご参考にしてください。
YouTubeにて、Pythonチュートリアル(公式ドキュメント)を使ってPythonの基礎文法を解説しています。
今回の内容をより詳しく知りたい方はこちらもご活用ください。