概要
この記事では、S3上に保存されたCSVファイルをLambdaで取り出し、jsonで返す方法を紹介します。
HTTP通信の窓口にはAPIGatewayを使用しています。
APIGatewayのイベントをトリガーにLambdaからS3へアクセスします。
この記事では、S3のポリシー設定、Lambdaのロール設定、LambdaとAPIGatewayの設定は割愛し、Lambdaの実装にフォーカスします。
前提条件
CSVファイルは以下のものを使用しました。
全体のソースコードは下に載せます。
またAWSサービスの認証はそれぞれの環境でお願いします。
"name","age"
"fuga","22"
"hoge","20"
Lambda
の実装
実装にはGo
を用います。
大まかに以下の流れです。
- s3にアクセスするためのクライアントを作成
- s3からオブジェクトを取得する
- オブジェクトを構造体にマーシャルする
- 全ての処理が成功したらレスポンスを返す
以下で詳しく説明していきます。
Lambda
からS3
にアクセスするための設定
S3にアクセスするためのクライアントを作成し、バケット名とファイル名で検索しています。
func hander(request events.APIGatewayProxyRequest) (events.APIGatewayProxyResponse, error) {
session := session.New()
s3Client := s3.New(session)
bucketName := "qiitahoge"
objectKey := "hoge.csv"
object, err := s3Client.GetObject(&s3.GetObjectInput{
Bucket: aws.String(bucketName),
Key: aws.String(objectKey),
})
if err != nil {
return events.APIGatewayProxyResponse{
Body: err.Error(),
StatusCode: 500,
}, nil
}
...
}
S3オブジェクトを読み込む
標準パッケージであるencoding/csv
を使ってCSVファイルを読み込みます。
func hander(request events.APIGatewayProxyRequest) (events.APIGatewayProxyResponse, error) {
...
defer object.Body.Close()
reader := csv.NewReader(object.Body)
rows, err := reader.ReadAll()
if err != nil {
return events.APIGatewayProxyResponse{
Body: err.Error(),
StatusCode: 500,
}, nil
}
...
}
読み込んだ結果を構造体に割り当てる
Hoge
という構造体を定義し、CSVの読み込み結果を割り当てます。
type Hoge struct {
Name string `json: "name"`
Age string `json: "age"`
}
func hander(request events.APIGatewayProxyRequest) (events.APIGatewayProxyResponse, error) {
...
var response []Hoge
for i, v := range rows {
if i == 0 {
continue
}
name := v[0]
age := v[1]
hoge := Hoge{
Name: name,
Age: age,
}
response = append(response, hoge)
}
...
}
レスポンス実装
今までの処理で問題がなければ、ステータスコード200と共にjsonオブジェクトを返してあげます。
func hander(request events.APIGatewayProxyRequest) (events.APIGatewayProxyResponse, error) {
...
bytes, err := json.Marshal(response)
if err != nil {
return events.APIGatewayProxyResponse{
Body: err.Error(),
StatusCode: 500,
}, nil
}
return events.APIGatewayProxyResponse{
Body: string(bytes),
StatusCode: 200,
}, nil
}
func main() {
lambda.Start(hander)
}
最後に
上の手順でlambdaの実装は完了です。
この後にgoファイルを圧縮して、lambdaにアップロードします。
最後に、Lambda
とAPIGateway
を繋いでAPIの完成です。
Postmanからリクエストを送ったところ、CSVの内容をjsonで確認することができました。
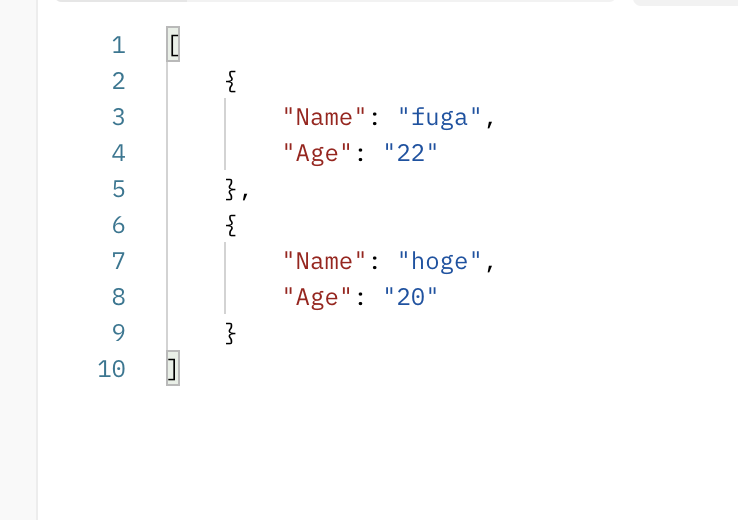
全ソースコードはこちらです。
参考