はじめに
自分の学習の記録、忘備録としての内容になります。Qiita初投稿のため、見にくい箇所、不備等があると思います。
内容: AzureBotServiceを使い、LINE 翻訳BOTを作る。
言語によって日本各地の方言を使って返答する。
完成動画
やったこと
1.node.jsでのLINE Botの作成
まず、この内容を一通り試し、基本的なLINE Botの作り方の学習をしました。 非常に丁寧に作られていて、分かり易かったです。1時間でLINE BOTを作るハンズオン (資料+レポート) in Node学園祭2017 #nodefest
- LINE Botの基礎知識学習
- LINE Messaging APIの基礎知識学習
- Node.jsでBot開発
- ngrokでトンネリング
- nowでデプロイ
2.AzureBotServiceを利用した、LINE翻訳Botの作成
今後、Azureでの開発も進めて行きたいこともあり、上記 node.js、nowを使った開発部分をAzureBotServiceを利用して行ってみることにしました。 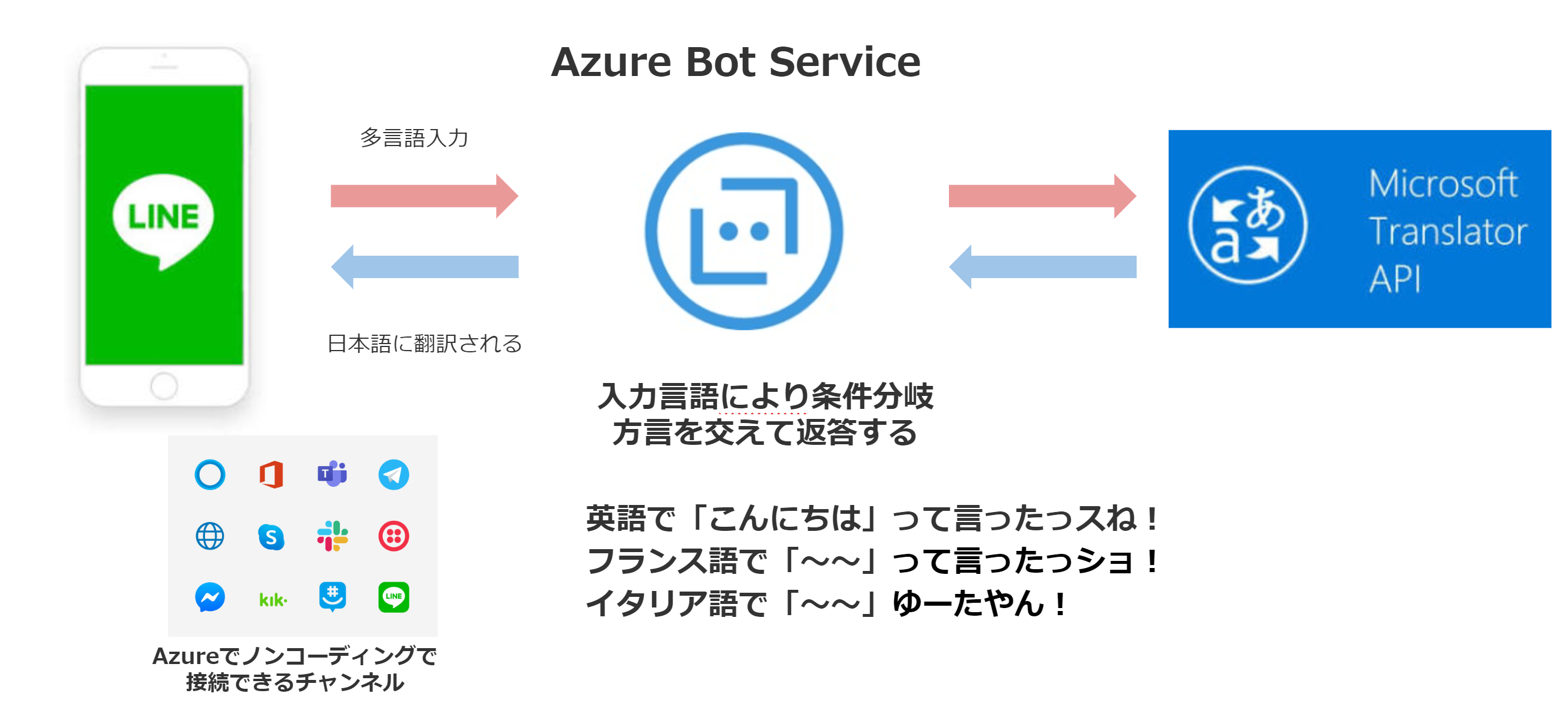下記サイトで、AzureBotServiceとLINEを簡単に連携できるチャンネルができたことを知り、この内容に沿って作成してみました。
祝 LINE チャンネル追加!Azure Bot Service で翻訳チャットボット作成
(1)Azure Bot Service から LINE 接続 編
祝 LINE チャンネル追加!Azure Bot Service で翻訳チャットボット作成
(2)Cognitive Services Translator Text API 編
非常にわかりやすくかかれており、これに沿って進めさせていただきました。
LINEのレスポンス部分の編集はApp Service Editor画面の bot.jsから行えます。
bot.js
// Copyright (c) Microsoft Corporation. All rights reserved.
// Licensed under the MIT License.
const { ActivityHandler } = require('botbuilder');
const rp = require('request-promise');
class EchoBot extends ActivityHandler {
constructor() {
super();
// See https://aka.ms/about-bot-activity-message to learn more about the message and other activity types.
/*
this.onMessage(async (context, next) => {
await context.sendActivity(`You said '${ context.activity.text }'`);
// By calling next() you ensure that the next BotHandler is run.
await next();
});
*/
this.onMessage(async (context, next) => {
console.log('got a message.');
// Translate
const subscriptionKey = '<SUBSCRIPTION_KEY>';
const options = {
method: 'POST',
baseUrl: 'https://api.cognitive.microsofttranslator.com/',
url: 'translate',
qs: {
'api-version': '3.0',
'to': 'ja'
},
headers: {
'Ocp-Apim-Subscription-Key': subscriptionKey,
'Content-type': 'application/json'
},
body: [{
'text': context.activity.text
}],
json: true,
};
const responseBody = await rp(options);
const translated = responseBody[0].translations[0].text;
const responseMessage = `英語で「${translated}」って言ったっスね!`
+ `(検出言語: ${responseBody[0].detectedLanguage.language})`;
await context.sendActivity(responseMessage);
await next();
});
this.onMembersAdded(async (context, next) => {
const membersAdded = context.activity.membersAdded;
for (let cnt = 0; cnt < membersAdded.length; ++cnt) {
if (membersAdded[cnt].id !== context.activity.recipient.id) {
await context.sendActivity('Hello and welcome!');
}
}
// By calling next() you ensure that the next BotHandler is run.
await next();
});
}
}
module.exports.EchoBot = EchoBot;
3.入力した言語により、翻訳に方言を交えて返答するBotの作成
入力した言語により、Switch文で条件分岐し イタリア後で「こんにちは」ゆーたやん!という風に文末を日本各地の方言を交えて返答する。bot.js
// Copyright (c) Microsoft Corporation. All rights reserved.
// Licensed under the MIT License.
const { ActivityHandler } = require('botbuilder');
const rp = require('request-promise');
class EchoBot extends ActivityHandler {
constructor() {
super();
// See https://aka.ms/about-bot-activity-message to learn more about the message and other activity types.
this.onMessage(async (context, next) => {
console.log('got a message.');
// Translate
const subscriptionKey = 'e2d153f610de4a8086ba83b7360c4c5b';
const options = {
method: 'POST',
baseUrl: 'https://api.cognitive.microsofttranslator.com/',
url: 'translate',
qs: {
'api-version': '3.0',
'to': 'ja'
},
headers: {
'Ocp-Apim-Subscription-Key': subscriptionKey,
'Content-type': 'application/json'
},
body: [{
'text': context.activity.text
}],
json: true,
};
const responseBody = await rp(options);
const translated = responseBody[0].translations[0].text;
/////////////////////////////////ここから変更//////////////////////////////////////////
const la = responseBody[0].detectedLanguage.language;
let lang = null;
let end_sent = 'って言ったっスね!';
switch(la) {
case 'en':
lang = '英語';
break
case 'fr':
lang = 'フランス語';
end_sent = 'って言ったっショ!';
break
case 'it':
lang = 'イタリア語';
end_sent = 'ゆーたやん!';
break
case 'es':
lang = 'スペイン語';
end_sent = 'っつったべ!';
break
case 'ja':
lang = '日本語';
end_sent = 'ちゆうちょっと!';
break
case 'ko':
lang = '韓国語';
end_sent = 'っゆったぺよ!';
break
case 'zh-Hans':
lang = '中国語';
end_sent = 'って言ったアルネ!';
break
case 'de':
lang = 'ドイツ語';
end_sent = 'っちゅうたじゃろ!';
break
case 'ru':
lang = 'ロシア語';
end_sent = 'って言うたがいね!';
break
default:
lang = 'ホゲホゲ語';
end_sent = 'っていったべさ!';
break
}
const responseMessage =lang+`で「${translated}」`+ end_sent
+ `(検出言語: ${responseBody[0].detectedLanguage.language})`;
/////////////////////////////////ここまで変更//////////////////////////////////////////
await context.sendActivity(responseMessage);
await next();
});
this.onMembersAdded(async (context, next) => {
const membersAdded = context.activity.membersAdded;
for (let cnt = 0; cnt < membersAdded.length; ++cnt) {
if (membersAdded[cnt].id !== context.activity.recipient.id) {
await context.sendActivity('Hello and welcome!');
}
}
// By calling next() you ensure that the next BotHandler is run.
await next();
});
}
}
module.exports.EchoBot = EchoBot;
最後に
LINEのチャンネルができたことで非常に簡単にLINE翻訳Botを作ることができました。 response部分の編集により色々バリエーションが作れます。今後、LUISを絡めた開発にもチャレンジしていきたいと思います。