シンプルなTODOアプリにバリデーション(入力制限)を追加していきます。
バリデーションはcreate
、save
、update
などのメソッドが呼び出されたタイミングで実行されます。
##バリデーションを追加する
タスクの最大文字数を20文字に設定するバリデーションをmodelに記述します。
文法はvalidates :{カラム名}, {ルール名}: {ルールの内容}
です。
class Task < ApplicationRecord
validates :title, length: {maximum: 20}
end
##エラーメッセージを表示する
バリデーションに失敗するとfalseが返され、@{オブジェクト名}.errors.full_messages
でエラーメッセージの配列を取得することができます。
まずはControllerを修正し、エラーが発生した場合時にトップページに移動しないようにします。
class TasksController < ApplicationController
.
.
def create
@task = Task.new(task_params)
if @task.save
redirect_to tasks_url
else
render 'tasks/new'
end
end
.
.
def update
@task = Task.find(params[:id])
if @task.update(task_params)
redirect_to tasks_url
else
render 'tasks/edit'
end
end
.
.
end
次に、Viewファイルにエラーメッセージを表示する記述を追加します。
<h1>新規タスク</h1>
<% if @task.errors.any? %>
<div>
<ul style="color: red">
<% @task.errors.full_messages.each do |message| %>
<li><%= message %></li>
<% end %>
</ul>
</div>
<% end %>
<%= form_for(@task) do |f| %>
・
・
<h1>タスク編集</h1>
<% if @task.errors.any? %>
<div>
<ul style="color: red">
<% @task.errors.full_messages.each do |message| %>
<li><%= message %></li>
<% end %>
</ul>
</div>
<% end %>
<%= form_for(@task) do |f| %>
・
・
・
##エラーメッセージを日本語化する
デフォルトのエラーメッセージは英語です。rails-i18n
というgemを使って日本語化します。まずはgemを追加しましょう。
gem 'rails-i18n'
$ bundle install
次に/config/application.rb
に以下の記述を追加します。
.
.
module BoardApp
class Application < Rails::Application
.
.
config.i18n.default_locale = :ja
end
end
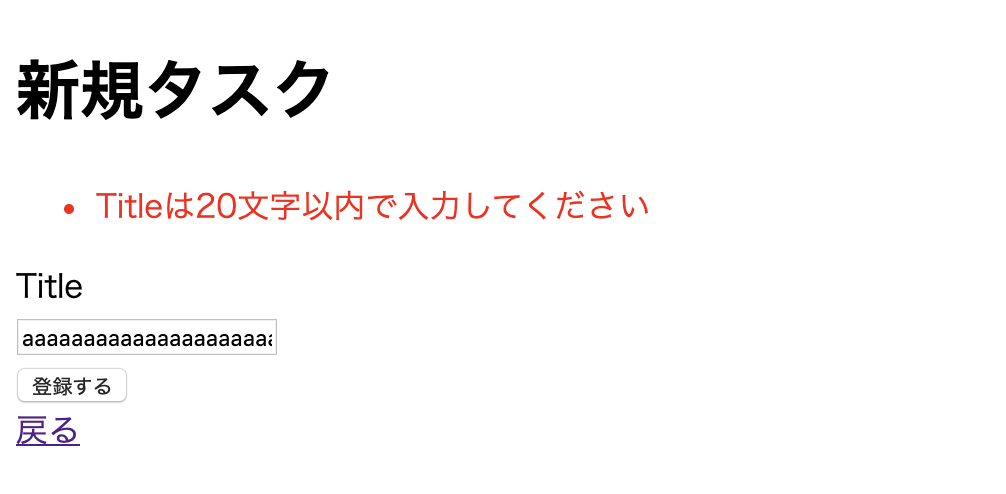
ja:
activerecord:
models:
task: #model名
attributes:
task: #model名
title: タスク名 #カラム名
作成したファイルへのパスを通します。
.
.
config.i18n.default_locale = :ja
config.i18n.load_path += Dir[Rails.root.join('config', 'locales', '**', '*.yml').to_s]
.
.

##よく使うバリデーション一覧
・最大文字数:length: { maximum: 20 }
・最小文字数:length: { minimum: 20 }
・文字数の範囲:length: { in: 1..30 }
・空でない:presence: true
・他と被っていない:uniqueness: true
・正規表現:format: { with: /<正規表現>/}
・「<属性名>_confirmation」と一致:confirmation: true
##複数指定する場合
複数指定する場合、ルールをカンマで区切ります。
class Task < ApplicationRecord
validates :title, length: {maximum: 20}, presence: true, uniqueness: true
end
メールアドレスの入力制限
VALID_EMAIL_REGEX = /\A[\w+\-.]+@[a-z\d\-.]+\.[a-z]+\z/i
validates :email, presence: true, uniqueness: true, format: { with: VALID_EMAIL_REGEX }