Androidアプリで色んなグラフ類を描画したいことがあると思います。
折れ線グラフとか棒グラフとかパイチャートとか。
Kotlinの練習用に大変便利な
MPAndroidChartを使って
PieChartを描画してみました。練習中なので変なところあるかも。
参考にさせて頂きました。
導入
gradle
allprojects {
repositories {
maven { url "https://jitpack.io" }
}
}
dependencies {
〜中略〜
compile 'com.github.PhilJay:MPAndroidChart:v3.0.3'
}
画面
layout
<?xml version="1.0" encoding="utf-8"?>
<android.support.constraint.ConstraintLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:app="http://schemas.android.com/apk/res-auto"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
tools:context="com.example.ssuzaki.piesample.MainActivity">
<com.github.mikephil.charting.charts.PieChart
android:id="@+id/pie"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:layout_margin="20dp"
/>
</android.support.constraint.ConstraintLayout>
コード
kotlin
package com.example.ssuzaki.piesample
import android.graphics.Color
import android.support.v7.app.AppCompatActivity
import android.os.Bundle
import com.github.mikephil.charting.charts.PieChart
import com.github.mikephil.charting.components.Description
import com.github.mikephil.charting.components.Legend
import com.github.mikephil.charting.data.PieData
import com.github.mikephil.charting.data.PieDataSet
import com.github.mikephil.charting.data.PieEntry
import com.github.mikephil.charting.formatter.PercentFormatter
import com.github.mikephil.charting.utils.ColorTemplate
import java.util.*
class MainActivity : AppCompatActivity() {
var mPie: PieChart? = null
override fun onCreate(savedInstanceState: Bundle?) {
super.onCreate(savedInstanceState)
setContentView(R.layout.activity_main)
setupPieChartView()
}
fun setupPieChartView() {
mPie = findViewById(R.id.pie)
mPie?.setUsePercentValues(true)
val desc: Description = Description()
desc.text = "PieChartのサンプルだよ"
mPie?.description = desc
val legend: Legend? = mPie?.legend
legend?.horizontalAlignment = Legend.LegendHorizontalAlignment.LEFT
/**
* サンプルデータ
*/
val value = Arrays.asList(10f, 20f, 30f, 40f)
val label = Arrays.asList("A", "B", "C", "D")
val entry = ArrayList<PieEntry>()
for(i in value.indices) {
entry.add( PieEntry(value.get(i), label.get(i)) )
}
/**
* ラベル
*/
val dataSet = PieDataSet(entry, "チャートのラベル")
dataSet.colors = ColorTemplate.COLORFUL_COLORS.toList()
dataSet.setDrawValues(true)
val pieData = PieData(dataSet)
pieData.setValueFormatter(PercentFormatter())
pieData.setValueTextSize(20f)
pieData.setValueTextColor(Color.WHITE)
mPie?.data = pieData
}
}
結果
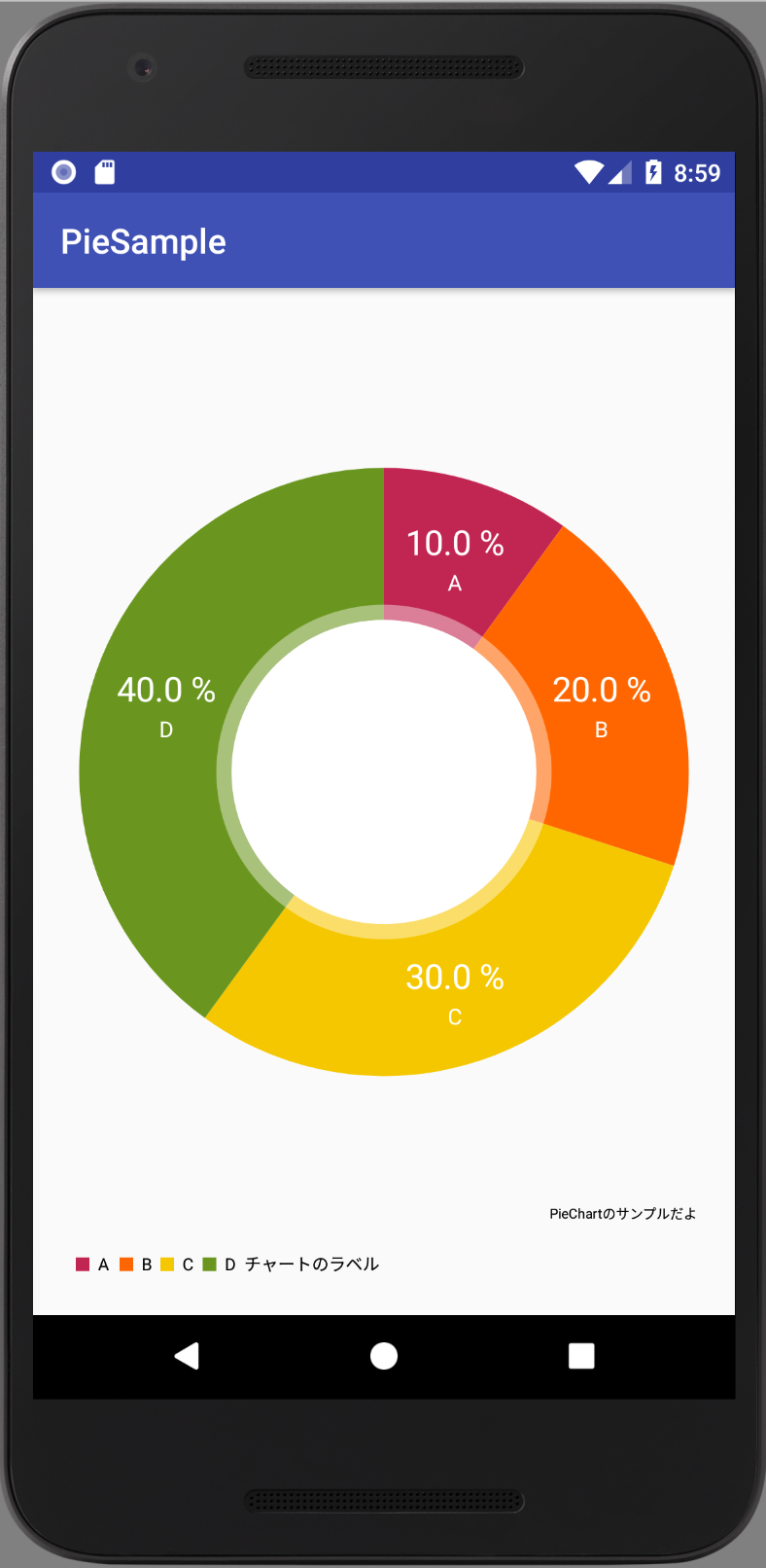