はじめに
今回は、アロー関数について書きます。
シリーズ
本記事はReact基礎講座のための連載になっています。気になる方のために、前の章は以下です。
Reactを使ってJSXの内容をレンダリングする(create-react-app)(React基礎講座1) - Qiita
JSXの中で変数を使いたい場合
変数の中身を展開させたい場合、なみ括弧(ブレース){ }
で囲うと展開されます。
const greeting = "Hello, React"; // 変数代入
const jsx = <h1>{greeting}</h1>; // 変数の中身を展開
JSXの中は1つのタグで囲う
なので、以下だとエラーになります。
const greeting = "Hello, React";
const introduction = "My Name is JS";
const jsx = <h1>{greeting}</h1><p>{introduction}</p>;
エラーメッセージは
Adjacent JSX elements must be wrapped in an enclosing tag. Did you want a JSX fragment <>...</>?
タグは複数ある場合は、それを囲むタグを用意せよと。では、div
タグで囲って見ましょう。
import React from "react";
import { render } from "react-dom";
const greeting = "Hello, React";
const introduction = "My Name is JS";
const jsx = (
<div>
<h1>{greeting}</h1>
<p>{introduction}</p>
</div>
);
render(jsx, document.getElementById("root"));
これでエラーは出なくなりました。
JSXで関数を使いたい場合
展開の仕方は変数と同じです。今回は、JSに最初から定義されているDateクラスの関数を使ってみます。
import React from "react";
import { render } from "react-dom";
// 今日の日付で Date オブジェクトを作成
let now = new Date();
const greeting = "Hello, React";
const introduction = "My Name is JS";
const jsx = (
<div>
<h1>{greeting}</h1>
<p>{introduction}</p>
<p>This Year is {now.getFullYear()}</p>
</div>
);
render(jsx, document.getElementById("root"));
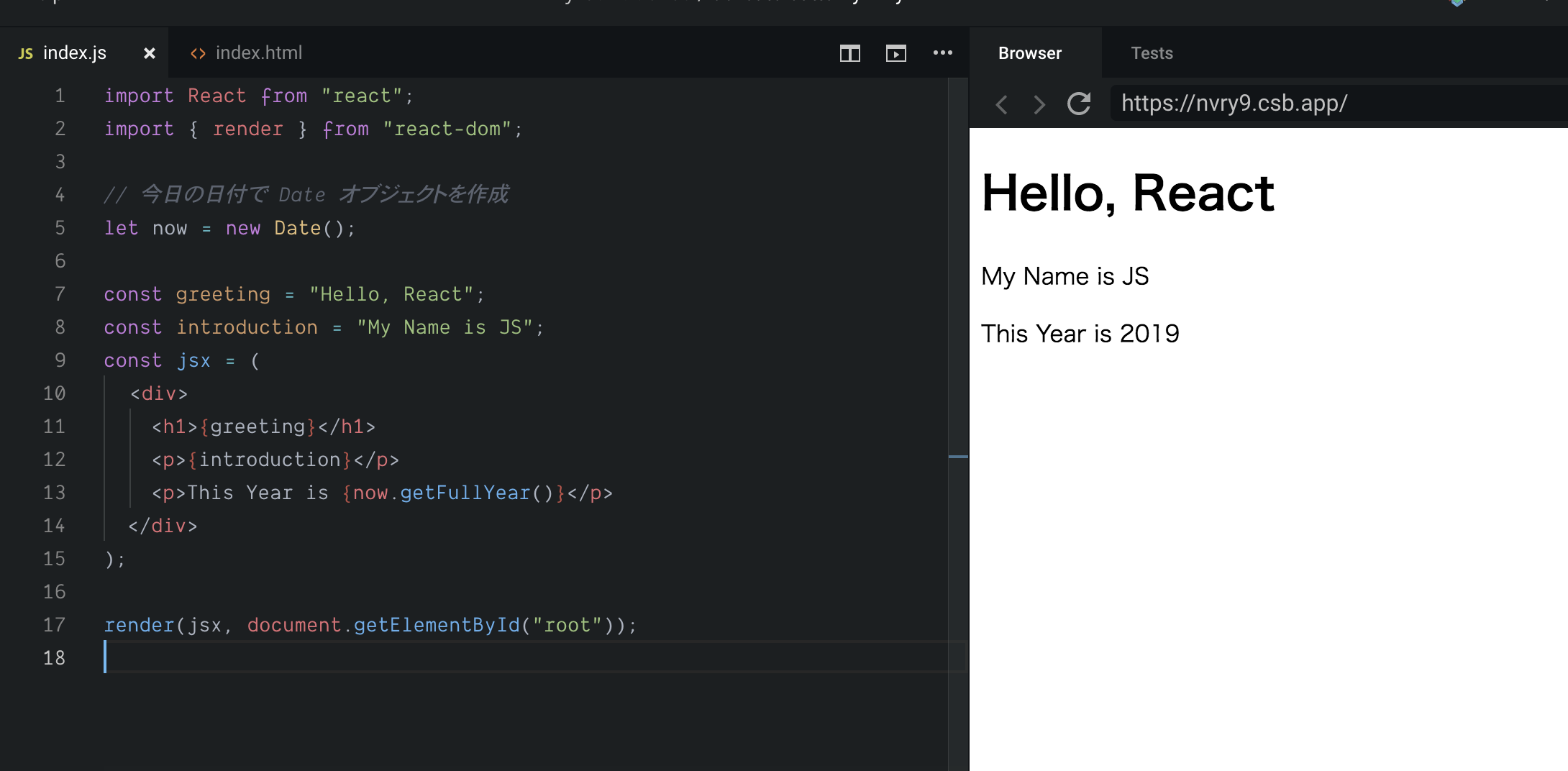
今年の年号が展開されましたね。なみ括弧(ブレース){ }
で囲うと、変数だけでなく関数やオブジェクトも使えると言うことがわかりました。
アロー関数で定義した中身を表示させたい場合
文字列とセットで今日の年号を返すアロー関数を定義して見ました。
import React from "react";
import { render } from "react-dom";
// 文字列とセットで今日の年号を返すアロー関数
const returnThisYear = () => {
// 今日の日付で Date オブジェクトを作成
let now = new Date();
return `This Year is ${now.getFullYear()}`
}
const greeting = "Hello, React";
const introduction = "My Name is JS";
const jsx = (
<div>
<h1>{greeting}</h1>
<p>{introduction}</p>
<p>{returnThisYear()}</p>
</div>
);
render(jsx, document.getElementById("root"));
呼び出すときは、今回もブレースで囲みます。{returnThisYear()}
正しく呼び出されたと思います。
アロー関数とは
アロー関数は ES2015(ES6) 以降生まれた 関数を定義するためのシンタックスです。this
の挙動をコントロールしやすくなるなどの利点があり利用されています。
シンタックス
昔からある関数の定義と比較して見ましょう。両方とも、文字列を返すだけの単純な関数です。
// 昔の関数
const mukashi_func = function() {
return "昔の関数"
}
console.log(mukashi_func);
// => function mukashi_func() {}
// => <constructor>: "Function"
// => name: "Function"
// アロー関数
const arrow_func = () => {
return "アロー関数"
}
console.log(arrow_func);
// => function arrow_func() {}
// => <constructor>: "Function"
// => name: "Function"
アロー関数で引数を渡す場合
const arrow_func = (param) => {
console.log(param)
}
arrow_func('test');
// => test
引数が1つなら、かっこを省略できます。
const arrow_func = param => {
console.log(param)
}
arrow_func('test');
// => test
引数が2つの場合は ()
を省略できません。
const arrow_func = (param1, param2) => {
console.log(param1, param2);
};
arrow_func('first', 'second' )
// => first second
1ライナーでの書き方
const arrow_func = () => {
console.log("one line");
}
arrow_func();
// => one line
上記は、return
するだけなので、以下のように1行で書くことができます。
const arrow_func = () => console.log("one line");
arrow_func();
// => one line
アロー関数の色々な書き方(省略形)を紹介しました。
React でアロー関数を使用する
引数を2つ渡して、アロー関数でもらった引数を整形して、render()
中のJSXに返す処理を書きます。
import React from "react";
import { render } from "react-dom";
const arrow_func = (no, name) => {
return `no is ${no}, name is ${name}`
}
render(arrow_func(1,'taro'), document.getElementById("root"));

参考
- 改訂新版JavaScript本格入門 ~モダンスタイルによる基礎から現場での応用まで | 山田 祥寛