Introduction
React.js is a javascript frontend library developed by facebook. It is used for building reusable UI component according to React official documentation.
React Features
JSX : React developers are recommended to use Javascript Syntax Extension.
Components : React application consist of components. We have to think of everything as components. It will help us to maintain the code easier when working with a large scale project.
Unidirectional data flow and flux : React implements one-way data flow which makes it easy to reason about your app. Flux is a pattern that helps keeping your data unidirectional.
Virtual DOM : React use virtual DOM instead of regular DOM to import app performance.
React.js Environment setup
To install React, we need to install Node.js first. In case you don't have NodeJS installed please go to the NodeJS official website to download and install. Once the NodeJS is installed, open the terminal and type:
node --version
npm --version
It will show up the NodeJS and NPM version that you are using. After we successfully install NodeJS, we can go and install ReactJS. There are two way we can install ReactJS:
- By using webpack and babel
- By using create-react-app command
Install using webpack and babel
- Create root folder for the application
mkdir react-app && cd react-app
After that run npm init
to generate package.json file.
- Install React and react-dom
npm install react react-dom --save
we use --save
to add it to package.json
- Install webpack
npm install webpack webpack-dev-server webpack-cli --save
- Install bebel
npm install babel-core babel-loader babel-preset-env babel-preset-react html-webpack-plugin --save-dev
-
Create all required file
-
index.html
-
App.js
-
main.js
-
webpack.config.js
-
.babelrc
-
Setup webpack (Compiler, Loaders and Server)
Now openwebpack.config.js
We are setting webpack entry point to be main.js. Output path is the place where bundled app will be served. We are also setting the development server to 8001 port. You can choose any port you want.
This is webpack.config.js
const path = require('path');
const HtmlWebpackPlugin = require('html-webpack-plugin');
module.exports = {
entry: './main.js',
output: {
path: path.join(__dirname, '/bundle'),
filename: 'index_bundle.js'
},
devServer: {
inline: true,
port: 8080
},
module: {
rules: [
{
test: /\.jsx?$/,
exclude: /node_modules/,
loader: 'babel-loader',
query: {
presets: ['es2015', 'react']
}
}
]
},
plugins:[
new HtmlWebpackPlugin({
template: './index.html'
})
]
}
Now open package.json
file and delete "test" "echo "Error: no test specified" && exit 1" inside the scripts object and add start and build command instead
"start": "webpack-dev-server --mode development --open --hot",
"build": "webpack --mode production"
- Add content to
index.html
<!DOCTYPE html>
<html lang = "en">
<head>
<meta charset = "UTF-8">
<title>React App</title>
</head>
<body>
<div id = "app"></div>
<script src = 'index_bundle.js'></script>
</body>
</html>
div id = "app" is the root element of the application
- Add content to
main.js
andApp.js
App.js
import React, { Component } from 'react';
class App extends Component{
render(){
return(
<div>
<h1>Hello World</h1>
</div>
);
}
}
export default App;
This is going to be the root component of the application (App component)
main.js
import React from 'react';
import ReactDOM from 'react-dom';
import App from './App.js';
ReactDOM.render(<App />, document.getElementById('app'));
Next, add this following content into .babelrc
{
"presets":["env", "react"]
}
-
Run the server
In terminal window type this command to start running the development server
npm start
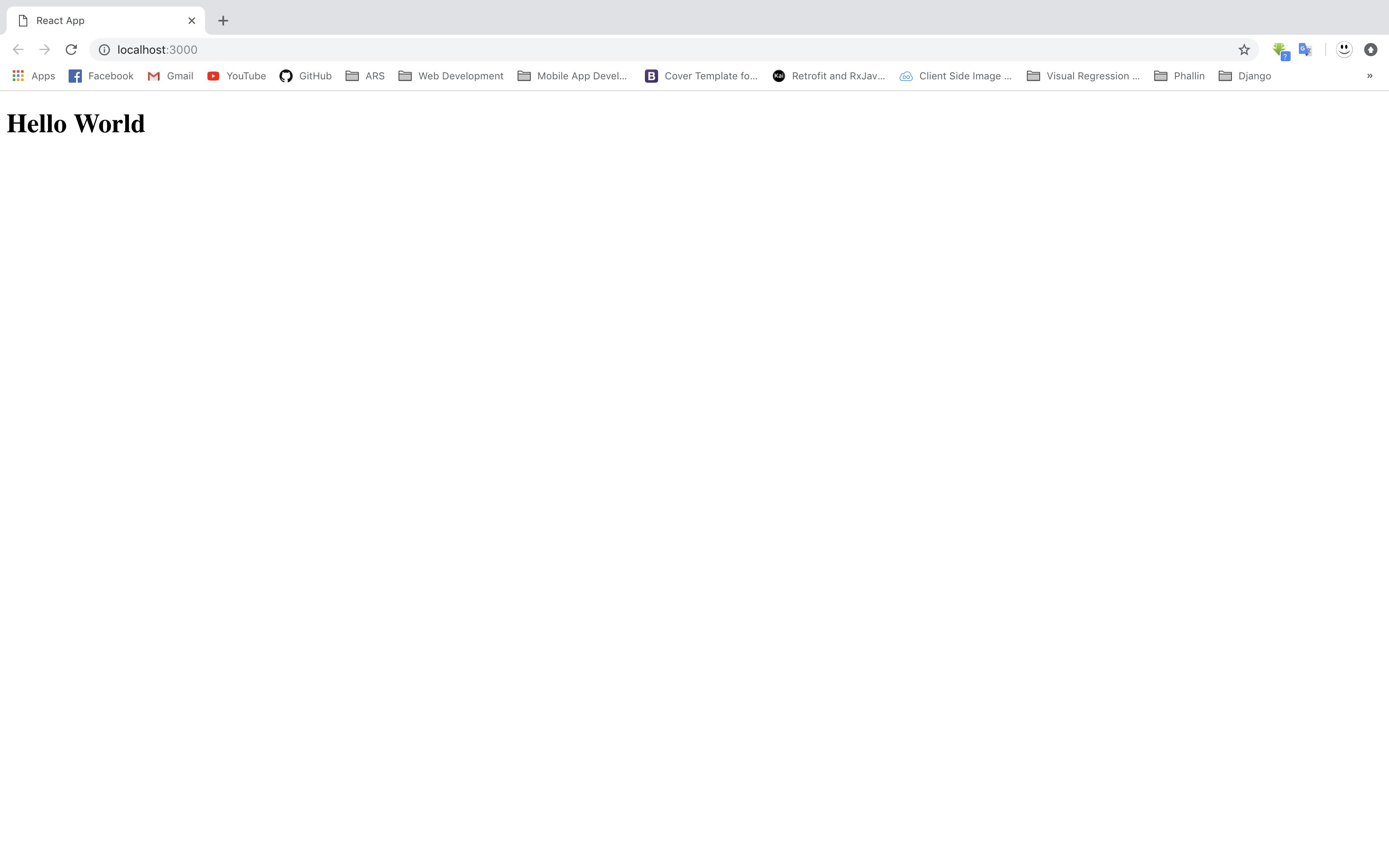
-
Generate the bundle
We can generate the bundle using build command
npm run build
Install using create-react-app
command
npx create-react-app react-sample && cd react-sample
This command will do everything for you. After this command complete we can install all the dependency and everything is good to go.
npm install
After that we can run the server using start command
npm start
Then the browser window is show up and we can proceed with the development part. Using this method is more easier than using webpack and babel which we have to do everything.