はじめに
- pymatgenでcifデータを取得する方法
- 取得したcifデータの詳細と扱い方
について解説していきます。
※マテリアルズインフォマティクス関係の内容を他にも投稿していますので、よろしければこちらの一覧から他の投稿も見て頂けますと幸いです。
CIFとは?
- Crystallographic Information Fileの略
- 結晶構造が収められたデータフォーマット
- 結晶構造データを得るために行われた実験や計算条件などもデータとして含まれる
pymatgenでcifデータを取得する
環境
- windows10
- conda 4.9.2
- python 3.7.1
- pymatgen 2020.4.29
データの取得
NaClのデータを取得していきます。こちらのページから引用しています。
# ライブラリのインポート
import pandas as pd
from pymatgen.ext.matproj import MPRester
# Materials Project の API キーの入力(自分のAPI keyを入力)
API_KEY = 'Your API'
# m.get_data(chemsys_formula_id)は Material Projectから指定化合物のデータを取得
# chemsys_formula_id は、元素の組み合わせ(e.g.,Li-Fe-O)、化合物名(e.g.,Fe2O3)、
# Material Projectの材料id (e.g., mp-1234)のいずれかを入力
with MPRester(API_KEY) as m:
NaCl_df = pd.DataFrame(m.get_data('NaCl',data_type='vasp'))
# 結果を保存
NaCl_df.to_csv('NaCl_df.csv')
# 結果を出力
NaCl_df
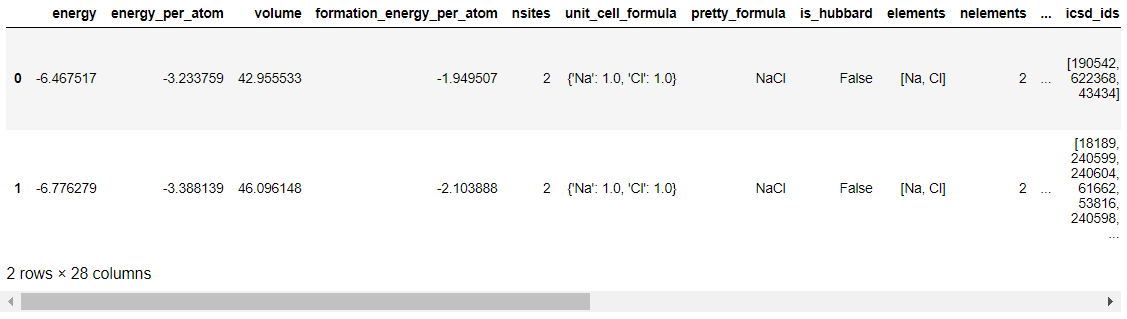
cifデータの取得
2行目の1のデータのcifファイルを取得します。こちらを参考にさせて頂きました。
# pymatgen.io.cifモジュールは構造からcifを入出力するためのラッパークラス(基本型の値をラップ)
import pymatgen.io.cif as pycif
# CIFファイルを解析(Parse)する
from pymatgen.io.cif import CifParser
#cifを取り出す
NaCl_cif_1 = CifParser.from_string(NaCl_df['cif'][1])
# CifParser.get_structureメソッドはcifのデータを入力するとStructureの部分をリスト型に整形して返す
NaCl_1 =CifParser.get_structures(NaCl_cif_1)
# NaCl_1は要素が1つのリスト
NaCl_1
[Structure Summary
Lattice
abc : 4.02463542 4.02463542 4.02463542
angles : 60.00000000000001 60.00000000000001 60.00000000000001
volume : 46.09614820053437
A : 1.1618121715635514 3.2861010599106226 2.0123177100000005
B : 1.1618121715635514 3.2861010599106226 -2.0123177099999996
C : -2.3236243431271024 3.2861010599106226 -0.0
PeriodicSite: Na (0.0000, 0.0000, 0.0000) [0.0000, 0.0000, 0.0000]
PeriodicSite: Cl (0.0000, 4.9292, 0.0000) [0.5000, 0.5000, 0.5000]]
.latticeで格子に関するデータを取得します。
NaCl_1[0].lattice
Lattice
abc : 4.02463542 4.02463542 4.02463542
angles : 60.00000000000001 60.00000000000001 60.00000000000001
volume : 46.09614820053437
A : 1.1618121715635514 3.2861010599106226 2.0123177100000005
B : 1.1618121715635514 3.2861010599106226 -2.0123177099999996
C : -2.3236243431271024 3.2861010599106226 -0.0
以下のように個別のデータを取り出すことも可能です。
NaCl_1[0].lattice.volume
46.09614820053437
このようにcifデータから構造に関する数値データを取得することができました。取得した数値は機械学習モデルへのインプットとして使えそうですね。
cifデータの解説
上記ではcifファイルから数値データを取得する方法を説明しましたが、実際のcifファイルにはどのような情報が含まれているのでしょうか。以下のコードで出力したcsvファイルのcifデータに関する部分を見ていきましょう。
# 結果を保存
NaCl_df.to_csv('NaCl_df.csv')
# generated using pymatgen
data_NaCl
_symmetry_space_group_name_H-M 'P 1'
_cell_length_a 4.02463542
_cell_length_b 4.02463542
_cell_length_c 4.02463542
_cell_angle_alpha 60.00000000
_cell_angle_beta 60.00000000
_cell_angle_gamma 60.00000000
_symmetry_Int_Tables_number 1
_chemical_formula_structural NaCl
_chemical_formula_sum 'Na1 Cl1'
_cell_volume 46.09614833
_cell_formula_units_Z 1
loop_
_symmetry_equiv_pos_site_id
_symmetry_equiv_pos_as_xyz
1 'x, y, z'
loop_
_atom_site_type_symbol
_atom_site_label
_atom_site_symmetry_multiplicity
_atom_site_fract_x
_atom_site_fract_y
_atom_site_fract_z
_atom_site_occupancy
Na Na0 1 0.00000000 0.00000000 0.00000000 1
Cl Cl1 1 0.50000000 0.50000000 0.50000000 1
こちらを参考にcifデータを解説していきます。
CIF文法上のルール
- 各々の化合物データは“data_”から始まる
- 各々の CIF 構文の記述は常に“_”から始まる
- 格子定数などの単位として“Å”を使用
“loop_”
対称操作、原子座標、温度因子など繰り返し同じ構文データ(パラメーター)が続く場合に用いられる
“chemical_”
IUPACが規定した命名法による化合物名、物質の融点、分子量などを記述する際に用いられる
“space_group_”
空間群・対称操作情報が含まれる
“cell_”
格子定数などの基礎結晶学的データが含まれる
最後に
- pymatgenでcifデータを取得する方法
- 取得したcifデータの詳細と扱い方
について解説致しました。こちらや公式ドキュメントを参考にするとcifデータについて様々な扱い方があることが分かります。また勉強していこうと思います。