概要
Flutterでウェブサイトを再現してみます。
Dartというプログラミング言語を使って記述します。
完成イメージ

プロジェクト作成
Flutterのインストール
Flutter download
適当なところでファイルをunzipし、PATHに追加します。.zshrcまたは、.bash_profile
export PATH="$PATH:`pwd`/flutter/bin"
完成したら、flutter doctor
を実行して、問題なければインストール完成です。
Apple Silicon Macの場合は事前に下記をインストールします。
sudo softwareupdate --install-rosetta --agree-to-license
VisualStudioCodeでFlutterPluginをインストール
プロジェクトをスムーズに作成したりコマンドを利用するために、VisualStudioCodeにPluginをインストールします。
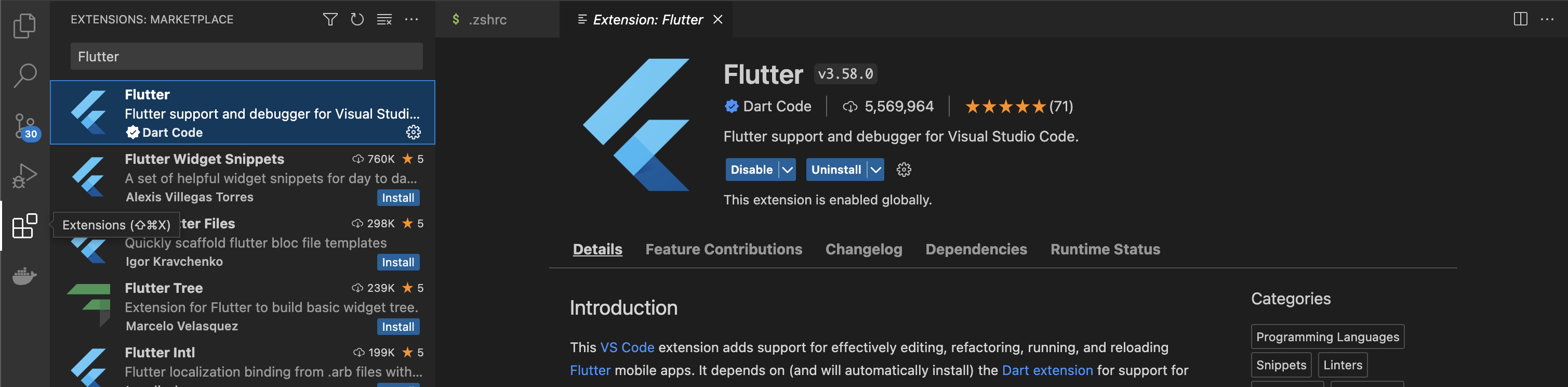
プロジェクトの作成
Cmd + Shift + Pを使って、flutterを入力すると、Flutter: New Project オプションを使います。
保存場所とアプリケーションの名前を設定して作成を進めます。
今回は「flutter_website」という名前にしました。
できたら、エミュレータで確認してみます。
確認したい端末を起動させた状態でメニューから「実行」>「デバックの開始」をクリックします。
AndroidのNexus 6で確認した場合このようになります。
TOPページ作成
ページごとにファイルを作成します。
libフォルダの中にtop.dartを作成します。
material.dartをインポートして、FlutterのMaterial Design GUIライブラリを利用できるようにします。
まず、FlutterではWidgetを組み合わせて画面に表示する要素を構成していきます。
TOPページは動的に変更が加わらないページなので、StatelessWidgetで記述していきます。
Buildメソッドを使ってフレームワークが画面上にレンダリングするWidgetツリーを返します。
Scaffoldは画面構成を実装する基礎となる部分です。Scafflodにの上に構成を載せていきます。
Scaffoldの中にメインとなる画面のbodyを記述していきます。
画像表示
picsumから画像を取得して表示します。
import 'package:flutter/material.dart';
class TopPage extends StatelessWidget {
const TopPage({super.key});
@override
Widget build(BuildContext context) {
return Scaffold(
body: Center(
child: Column(
children: <Widget>[
Container(
width: MediaQuery.of(context).size.width,
height: MediaQuery.of(context).size.height / 4,
decoration: const BoxDecoration(
image: DecorationImage(
image: NetworkImage("https://picsum.photos/1000/400"),
fit: BoxFit.cover),
),
),
],
),
),
);
}
}
確認
確認のため、main.dartでTopページを表示できるようにします。
import 'package:flutter/material.dart';
import 'top.dart';
void main() {
runApp(const MyApp());
}
class MyApp extends StatelessWidget {
const MyApp({super.key});
// This widget is the root of your application.
@override
Widget build(BuildContext context) {
return MaterialApp(
title: 'Flutter Website',
theme: ThemeData(
primarySwatch: Colors.blue,
),
home: const TopPage(),
);
}
}
シミュレータで下記の感じです。
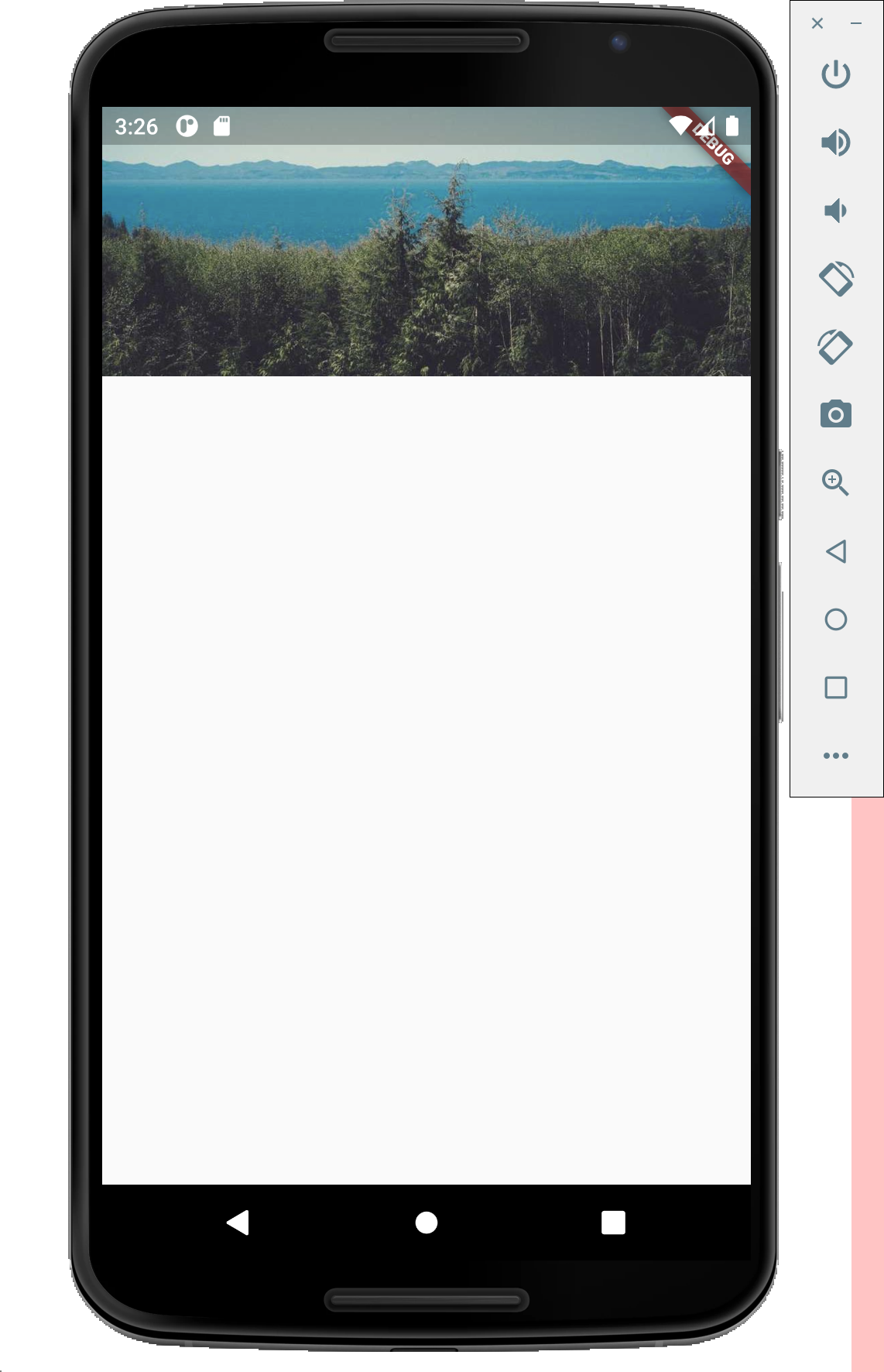
メインタイトル追加
Bluecode, Lifestyle developer.を追加します。
import 'package:flutter/material.dart';
class TopPage extends StatelessWidget {
const TopPage({super.key});
@override
Widget build(BuildContext context) {
return Scaffold(
body: Center(
child: Column(
children: <Widget>[
Container(
width: MediaQuery.of(context).size.width,
height: MediaQuery.of(context).size.height / 4,
decoration: const BoxDecoration(
image: DecorationImage(
image: NetworkImage("https://picsum.photos/1000/400"),
fit: BoxFit.cover),
),
+ child: const Center(
+ child: Text(
+ "bluecode, Lifestyle developer.",
+ textAlign: TextAlign.center,
+ style: TextStyle(color: Colors.white),
+ ),
+ ),
),
],
),
),
);
}
}
child: const Center(
child: Text(
"bluecode, Lifestyle developer.",
textAlign: TextAlign.center,
style: TextStyle(color: Colors.white),
),
),
同様の手順でその下も追加します。
import 'package:flutter/material.dart';
class TopPage extends StatelessWidget {
const TopPage({super.key});
@override
Widget build(BuildContext context) {
return Scaffold(
body: Center(
child: Column(
children: <Widget>[
Container(
width: MediaQuery.of(context).size.width,
height: MediaQuery.of(context).size.height / 4,
decoration: const BoxDecoration(
image: DecorationImage(
image: NetworkImage("https://picsum.photos/1000/400"),
fit: BoxFit.cover),
),
child: const Center(
child: Text(
"bluecode, Lifestyle developer.",
textAlign: TextAlign.center,
style: TextStyle(color: Colors.white),
),
),
),
+ Container(
+ margin: const EdgeInsets.only(top: 20),
+ child: Column(
+ children: const [
+ Text('websiteの最新情報'),
+ Text('最新情報をご案内します。'),
+ ],
+ ),
+ ),
],
),
),
);
}
}
Container(
margin: const EdgeInsets.only(top: 20),
child: Column(
children: const [
Text('websiteの最新情報'),
Text('最新情報をご案内します。'),
],
),
),
エミュレータを起動中の場合は自動で更新が走りこのようになっているはずです。