こんにちは streampack チームのメディです。
https://cloudpack.jp/service/option/streampack.html
In my opinion ReactPlayer is a really interesting player and I would like to show you common use cases.
ReactPlayer は興味深いプレイヤーなので、一般的な使用法を説明します。
##Copyrights of videos
Big Buck Bunny
© copyright 2008, Blender Foundation | www.bigbuckbunny.org
Sintel
© copyright Blender Foundation | www.sintel.org
#What is ReactPlayer ・ ReactPlayerとは
ReactPlayer is a React component that supports HLS , MPEG-DASH, YouTube, Facebook, Twitch, SoundCloud, Streamable, Vimeo, Wistia, Mixcloud, and DailyMotion.
ReactPlayerは、HLS、MPEG-DASH、YouTube、Facebook、Twitch、SoundCloud、Streamable、Vimeo、Wistia、Mixcloud、DailyMotionをサポートするReactコンポーネントです。
#Create the React App ・ React Appを作成してください
yarn create react-app my-player
cd my-player
yarn add react-player
yarn start
Please go to :
次のURLにアクセスしてください:
http://localhost:3000/
You should see something similar to this:
これに似たものが表示されます。
To try the following examples, simply replace the code defined in
my-player/src/App.js
次の例を試すには、my-myplayer/src/App.js
CSS
###Responsive web design ・レスポンシブウェブデザイン
.player-wrapper {
position: relative;
padding-top: 56.25% /* Player ratio: 100 / (1280 / 720) */
}
.react-player {
position: absolute;
top: 0;
left: 0;
}
NB: I will use the same CSS code for all examples.
注意: すべての例で同じCSSコードを使用します。
##PIP: Picture In Picture !・ PIP: ピクチャインピクチャ
Chrome only !
Chromeのみ!
import React, { Component } from 'react';
import ReactPlayer from 'react-player';
class ResponsivePlayer extends Component {
state = {
url: '//download.blender.org/durian/trailer/sintel_trailer-480p.mp4',
pip: false,
playing: true,
muted: true
}
load = url => {
this.setState({
url,
pip: false
})
}
componentDidMount(){
console.log("component did mount")
}
pip = () => {
this.setState({ pip: !this.state.pip })
}
onEnablePIP = () => {
console.log('onEnablePIP')
this.setState({ pip: true })
}
onDisablePIP = () => {
console.log('onDisablePIP')
this.setState({ pip: false })
}
render () {
const { url, playing, muted, pip } = this.state
return (
<div className='player-wrapper'>
<ReactPlayer
className='react-player'
controls
width='640px'
height='360px'
url={url}
pip={pip}
muted={muted}
playing={playing}
onEnablePIP={this.onEnablePIP}
onDisablePIP={this.onDisablePIP}
/>
<button onClick={this.pip}>PIP</button>
</div>
)
}
}
export default ResponsivePlayer;
Events & logging ・ イベントとロギング
import React, { Component } from 'react';
import ReactPlayer from 'react-player';
import './App.css';
class ResponsivePlayer extends Component {
state = {
url: '//download.blender.org/durian/trailer/sintel_trailer-480p.mp4',
playing: true,
muted: true,
playbackRate: 1.0,
loop: false
}
load = url => {
this.setState({
url
})
}
componentDidMount(){
console.log("component did mount")
}
onPlay = () => {
console.log('onPlay')
this.setState({ playing: true })
}
onPause = () => {
console.log('onPause')
this.setState({ playing: false })
}
onProgress = state => {
console.log('onProgress', state)
// We only want to update time slider if we are not currently seeking
if (!this.state.seeking) {
this.setState(state)
}
}
onEnded = () => {
console.log('onEnded')
this.setState({ playing: this.state.loop })
}
onDuration = (duration) => {
console.log('onDuration', duration)
this.setState({ duration })
}
render () {
const { url, playing, muted, loop, playbackRate} = this.state
return (
<div className='player-wrapper'>
<ReactPlayer
className='react-player'
controls
width='100%'
height='100%'
url={url}
playing={playing}
loop={loop}
playbackRate={playbackRate}
muted={muted}
onReady={() => console.log('onReady')}
onStart={() => console.log('onStart')}
onPlay={this.onPlay}
onPause={this.onPause}
onBuffer={() => console.log('onBuffer')}
onSeek={e => console.log('onSeek', e)}
onEnded={this.onEnded}
onError={e => console.log('onError', e)}
onProgress={this.onProgress}
onDuration={this.onDuration}
/>
</div>
)
}
}
export default ResponsivePlayer;
####Full list of callback props ・コールバックの完全なリスト
Subtitles ・ 字幕

import React, { Component } from 'react';
import ReactPlayer from 'react-player';
import './App.css';
class ResponsivePlayer extends Component {
render () {
return (
<div className='player-wrapper'>
<ReactPlayer
url='//iandevlin.github.io/mdn/video-player-with-captions/video/sintel-short.mp4'
className='react-player'
controls
width='100%'
height='100%'
config={{ file: {
attributes: {
crossOrigin: 'true'
},
tracks: [
{kind: 'subtitles', src: '//iandevlin.github.io/mdn/video-player-with-captions/subtitles/vtt/sintel-en.vtt', srcLang: 'en', default: true},
{kind: 'subtitles', src: '//iandevlin.github.io/mdn/video-player-with-captions/subtitles/vtt/sintel-de.vtt', srcLang: 'de'},
{kind: 'subtitles', src: '//iandevlin.github.io/mdn/video-player-with-captions/subtitles/vtt/sintel-es.vtt', srcLang: 'es'}
]
}}}
/>
</div>
)
}
}
export default ResponsivePlayer;
HLS
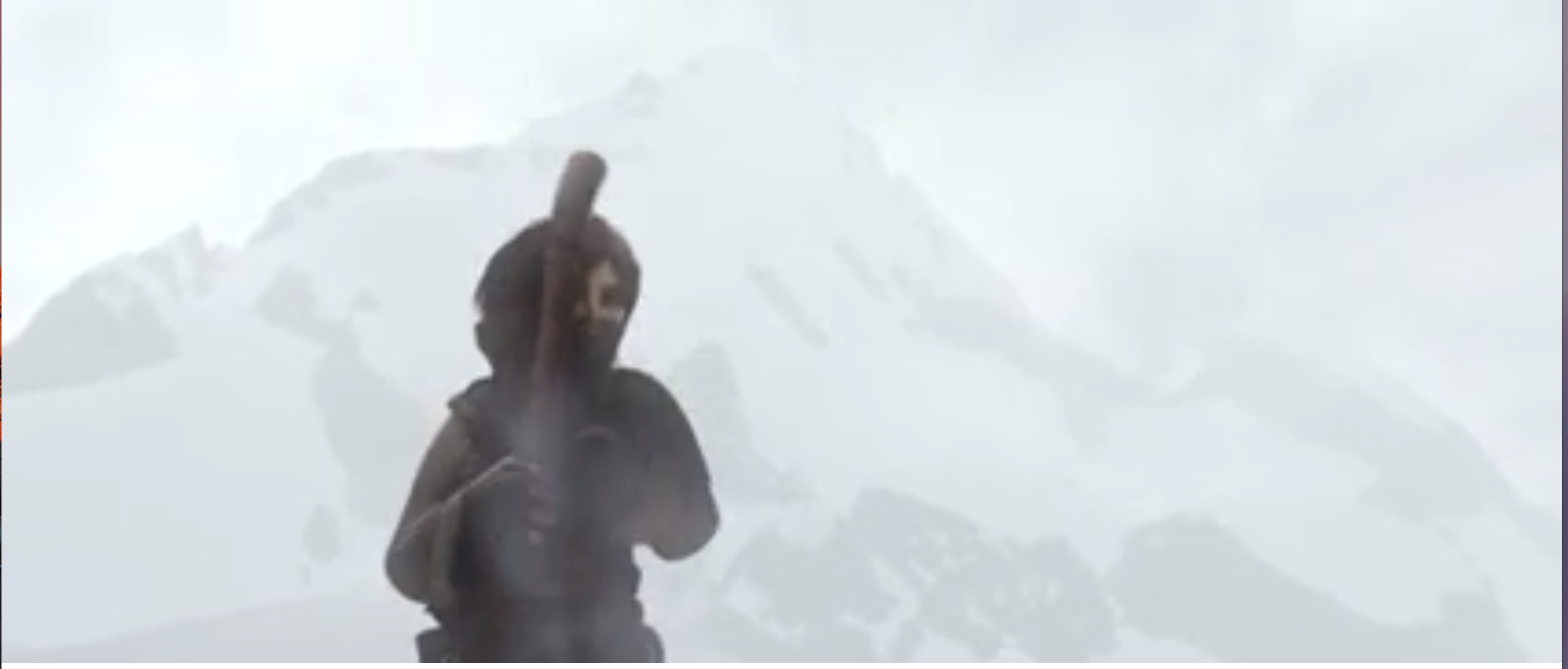
import React, { Component } from 'react';
import ReactPlayer from 'react-player';
import './App.css';
class ResponsivePlayer extends Component {
render () {
return (
<div className='player-wrapper'>
<ReactPlayer
url='//bitdash-a.akamaihd.net/content/sintel/hls/playlist.m3u8'
className='react-player'
controls
width='100%'
height='100%'
/>
</div>
)
}
}
export default ResponsivePlayer;
MPEG-DASH
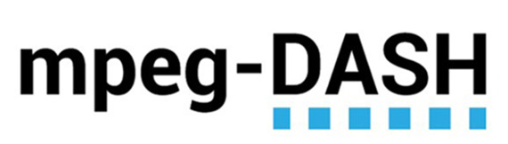
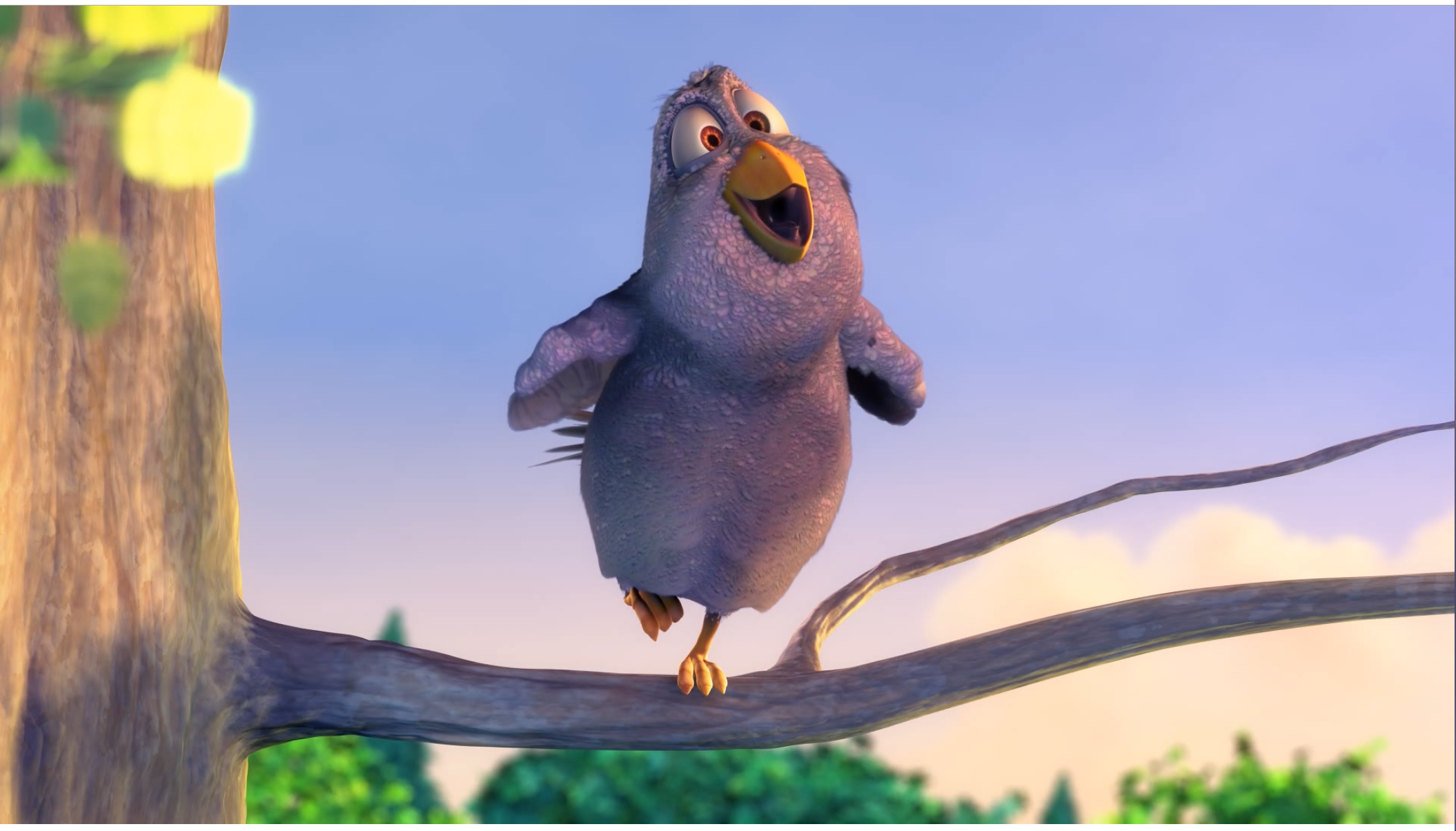
import React, { Component } from 'react';
import ReactPlayer from 'react-player';
import './App.css';
class ResponsivePlayer extends Component {
render () {
return (
<div className='player-wrapper'>
<ReactPlayer
url='//rdmedia.bbc.co.uk/dash/ondemand/bbb/2/client_manifest-common_init.mpd'
className='react-player'
controls
width='100%'
height='100%'
/>
</div>
)
}
}
export default ResponsivePlayer;
Youtube, Vimeo, Dailymotion, Facebook, Twitch... !
It's very easy to play contents from famous video sharing platforms in ReactPlayer.
ReactPlayerで有名なビデオ共有プラットフォームのコンテンツを再生するのはとても簡単です。
####Supported services・サポートされるサービス
- YouTube
- Twitch
- SoundCloud
- Streamable
- Vimeo
- Wistia
- Mixcloud
- DailyMotion
YouTube ・ YouTubeの例

import React, { Component } from 'react';
import ReactPlayer from 'react-player';
import './App.css';
class ResponsivePlayer extends Component {
render () {
return (
<div className='player-wrapper'>
<ReactPlayer
className='react-player'
url='https://www.youtube.com/watch?v=YE7VzlLtp-4'
width='100%'
height='100%'
/>
</div>
)
}
}
export default ResponsivePlayer;
Vimeo ・ Vimeoの例

import React, { Component } from 'react';
import ReactPlayer from 'react-player';
import './App.css';
class ResponsivePlayer extends Component {
render () {
return (
<div className='player-wrapper'>
<ReactPlayer
url='//player.vimeo.com/video/277572065'
className='react-player'
controls
width='100%'
height='100%'
/>
</div>
)
}
}
export default ResponsivePlayer;
#Information sources ・ 情報源
Player homepage
https://github.com/CookPete/react-player
Player demo
https://github.com/CookPete/react-player/blob/master/src/demo/App.js
https://cookpete.com/react-player/
Media files
http://www.bbc.co.uk/rd/blog/2013-09-mpeg-dash-test-streams
http://docs.evostream.com/sample_content/table_of_contents
https://bitmovin.com/mpeg-dash-hls-examples-sample-streams/
http://iandevlin.github.io/mdn/video-player-with-captions/
https://www.youtube.com/watch?v=YE7VzlLtp-4
https://player.vimeo.com/video/277572065
https://www.blender.org/about/projects/
https://download.blender.org/durian/trailer/