本記事の内容
TypeScriptやGo言語をサポートしているPrismaの説明です。MySQLのCRUD処理に関した記事となります。
※TBLの作成方法はマイグレーション編を参考にしてください。
基本情報
更新対象TBL(マイグレーション編で作成済)
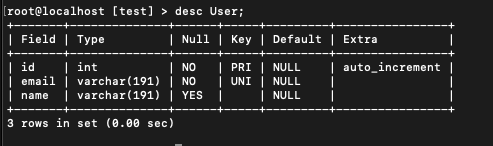ディレクトリ構成
~/develop/study/starter $ tree -I node_modules
.
├── package-lock.json
├── package.json
├── prisma
│ ├── dev.db
│ └── schema.prisma
├── script.ts
└── tsconfig.json
1 directory, 6 files
CREATE(INSERT文)
1レコード追加
【script.ts】
import { PrismaClient } from '@prisma/client'
const prisma = new PrismaClient()
/** Userテーブルに1レコード追加 */
async function main() {
/** テーブル名はprisma.TBL名の箇所で指定。 */
const allUsers = await prisma.user.create({
data:{
email:'user@mail.com',
name:'user1'
}
})
console.dir(allUsers, { depth: null })
}
main()
.catch(e => {
throw e
})
.finally(async () => {
await prisma.$disconnect()
})
複数レコード追加
【script.ts】
import { PrismaClient } from '@prisma/client'
const prisma = new PrismaClient()
/** Userテーブルに複数レコード追加 */
async function main() {
/** テーブル名はprisma.TBL名の箇所で指定。 */
const allUsers = await prisma.user.createMany({
data:[
{ email:'user1@mail.com',name:'user1'},
{ email:'user2@mail.com',name:'user2'},
{ email:'user3@mail.com',name:'user3'},
]
})
console.dir(allUsers, { depth: null })
}
main()
.catch(e => {
throw e
})
.finally(async () => {
await prisma.$disconnect()
})
Read(SELECT文)
1レコードのみ取得
【script.ts】
import { PrismaClient } from '@prisma/client'
const prisma = new PrismaClient()
/** Userテーブルに1レコードのみ取得 */
async function main() {
/** テーブル名はprisma.TBL名の箇所で指定。 */
const allUsers = await prisma.user.findUnique({
where: {
email: 'user1@mail.com',
},
})
console.dir(allUsers, { depth: null })
}
main()
.catch(e => {
throw e
})
.finally(async () => {
await prisma.$disconnect()
})
複数レコード取得
【script.ts】
import { PrismaClient } from '@prisma/client'
const prisma = new PrismaClient()
/** Userテーブルに複数レコード取得 */
async function main() {
/** テーブル名はprisma.TBL名の箇所で指定。 */
const allUsers = await prisma.user.findMany({
})
console.dir(allUsers, { depth: null })
}
main()
.catch(e => {
throw e
})
.finally(async () => {
await prisma.$disconnect()
})
複数条件でのレコード取得
【script.ts】
import { PrismaClient } from '@prisma/client'
const prisma = new PrismaClient()
/** Userテーブルに複数レコード取得 */
async function main() {
/** テーブル名はprisma.TBL名の箇所で指定。 */
const allUsers = await prisma.user.findMany({
where: {
AND: {
email:'user1@mail.com',
name:'user1'
}
}
})
console.dir(allUsers, { depth: null })
}
main()
.catch(e => {
throw e
})
.finally(async () => {
await prisma.$disconnect()
})
UPDATE(UPDATE文)
1レコードのみ更新
import { PrismaClient } from '@prisma/client'
const prisma = new PrismaClient()
/** Userテーブルに1レコードのみ更新 */
async function main() {
/** テーブル名はprisma.TBL名の箇所で指定。 */
const allUsers = await prisma.user.update({
/**更新レコードを指定 */
where: {
email:'user1@mail.com',
},
/**更新内容 */
data:{
name:'kouji',
},
})
console.dir(allUsers, { depth: null })
}
main()
.catch(e => {
throw e
})
.finally(async () => {
await prisma.$disconnect()
})
複数レコード更新
import { PrismaClient } from '@prisma/client'
const prisma = new PrismaClient()
/** Userテーブルに複数レコード更新 */
async function main() {
/** テーブル名はprisma.TBL名の箇所で指定。 */
const allUsers = await prisma.user.updateMany({
/**更新内容 */
data:{
name:'kkfactory',
},
})
console.dir(allUsers, { depth: null })
}
main()
.catch(e => {
throw e
})
.finally(async () => {
await prisma.$disconnect()
})
DELTE(DELTE文)
1レコードのみ削除
import { PrismaClient } from '@prisma/client'
const prisma = new PrismaClient()
/** Userテーブルに複数レコード取得 */
async function main() {
/** テーブル名はprisma.TBL名の箇所で指定。 */
const allUsers = await prisma.user.delete({
where:{
email:'user1@mail.com'
}
})
console.dir(allUsers, { depth: null })
}
main()
.catch(e => {
throw e
})
.finally(async () => {
await prisma.$disconnect()
})
複数レコード削除
import { PrismaClient } from '@prisma/client'
const prisma = new PrismaClient()
/** Userテーブルに複数レコード取得 */
async function main() {
/** テーブル名はprisma.TBL名の箇所で指定。 */
const allUsers = await prisma.user.deleteMany({
})
console.dir(allUsers, { depth: null })
}
main()
.catch(e => {
throw e
})
.finally(async () => {
await prisma.$disconnect()
})