はじめに
業務でOpenAPIを用いたAPI設計を行っているため、備忘録も兼ねてまとめる。
入門用なので、基本的な部分のみ解説。
概要
OpenAPI
What Is OpenAPI?
OpenAPIとは、RESTful APIを記述するためのフォーマットのこと。
Swagger 2.0を拡張して実装されている。
Swagger
Swaggerとは、OpenAPIを用いてREST APIを設計する際に使用するツールセットのこと。
メジャーなものとしては以下。
ツール名 | 概要 |
---|---|
Swagger Editor | ブラウザベースでOpenAPIを記述できるエディター。Dockerイメージも配布されており、ローカルでの実行も可能。 |
Swagger UI | OpenAPIの記述を、動的にAPIドキュメントとしてレンダリングするツール。上記のSwagger Editorの右半分に出ているのはコレ。 |
Swagger Codegen | OpenAPIの記述から、サーバー/クライアントのコードを生成するツール。多くの言語に対応。 |
記述形式
OpenAPIの記述は以下の2種類から選択可能。
どちらの形式で記述しても、アウトプットは同じ。
またYAML
→JSON
、JSON
→YAML
の相互変換が可能。(Swagger Editorの機能)
- YAML形式
- JSON形式
基本構造
本記事では、YAML
形式で記述していく。
openapi: 3.0.0
info:
...
servers:
...
paths:
...
components:
...
security:
...
tags:
...
externalDocs:
...
フィールド名 | 必須 | 概要 |
---|---|---|
openapi | YES | セマンティックなバージョニングを記述する。今回は3.0.0 を用いる。詳しくはドキュメントを参照。 |
info | YES | APIのメタデータを記述する。 |
servers | APIを提供するサーバーを記述する。配列で複数記述可能(STG, PROD等)。 | |
paths | YES | APIで利用可能なエンドポイントやメソッドを記述する。 |
components | YES | APIで使用するオブジェクトスキーマを記述する。 |
security | API全体を通して使用可能なセキュリティ仕様を記述する。(OAuth等) | |
tags | APIで使用されるタグのリスト。各種ツールによってパースされる際は、記述された順序で出力される。タグ名はユニークで無ければならない。 | |
externalDocs | 外部ドキュメントを記述する(API仕様書等)。 |
各フィールドの詳細
info
ドキュメント: Info Object
info:
title: Sample API
description: A short description of API.
termsOfService: http://example.com/terms/
contact:
name: API support
url: http://www.example.com/support
email: support@example.com
license:
name: Apache 2.0
url: http://www.apache.org/licenses/LICENSE-2.0.html
version: 1.0.0
フィールド名 | 必須 | 概要 |
---|---|---|
title | YES | APIの名称。 |
description | APIの簡潔な説明。CommonMarkシンタックスが使える。 | |
termsOfService | APIの利用規約。URL形式でなければならない。 | |
contact | コンタクト情報。(サポートページのURLやメールアドレス等) | |
license | ライセンス情報。ライセンスページのURLも記述可能。 | |
version | YES | APIドキュメントのバージョン。 |
servers
ドキュメント: Server Object
servers:
- url: https://dev.sample-server.com/v1
description: Development server
- url: https://stg.sample-server.com/v1
description: Staging server
- url: https://api.sample-server.com/v1
description: Production server
フィールド名 | 必須 | 概要 |
---|---|---|
url | YES | APIサーバーのURL。 |
description | APIサーバーの説明。 |
Swagger UIでの表示
複数の場合、ドロップダウンで表示される。

paths
ドキュメント: Paths Object
paths:
# paths オブジェクト
/users:
# path item オブジェクト
get: # GET
# Operationオブジェクト
tags:
- users
summary: Get all users.
description: Returns an array of User model
parameters: []
responses: # レスポンス定義
'200': # HTTP status
description: A JSON array of User model
content:
application/json: # レスポンスの形式指定
schema:
type: array
items:
$ref: '#/components/schemas/User' # 参照するモデル
example: # サンプルデータ
- id: 1
name: John Doe
- id: 2
name: Jane Doe
post: # POST
tags:
- users
summary: Create a new User
description: Create a new User
parameters: []
requestBody: # リクエストボディ
description: user to create
content:
application/json:
schema: # POSTするオブジェクト
$ref: '#/components/schemas/User'
example:
id: 3
name: Richard Roe
responses:
'201':
description: CREATED
/users/{userId}:
get:
tags:
- users
summary: Get user by ID.
description: Returns a single User model
parameters: # リクエストパラメータ
- name: userId
in: path # パラメータをパス内に含める
description: user id
required: true
schema:
type: integer
responses:
'200':
description: A single User model
content:
application/json:
schema:
type: object
items:
$ref: '#/components/schemas/User'
example:
id: 1
name: John Doe
paths
オブジェクト
|フィールド名|概要|
|:--|:--|:--|
|/{path}|各エンドポイントのパスを記述する。servers
で定義したURLにこのパスを結合したものが最終的なエンドポイントとなる。|
path item
オブジェクト
|フィールド名|概要|
|:--|:--|:--|
|summary|エンドポイントのサマリ。|
|descriptions|エンドポイントの簡潔な説明。CommonMarkシンタックスを使用可能。|
|get, post, delete...|HTTPメソッドを定義。GET, PUT, POST, DELETE, OPTIONS, DELETE, PATCH, TRACEが使用可能。|
Swagger UIでの表示

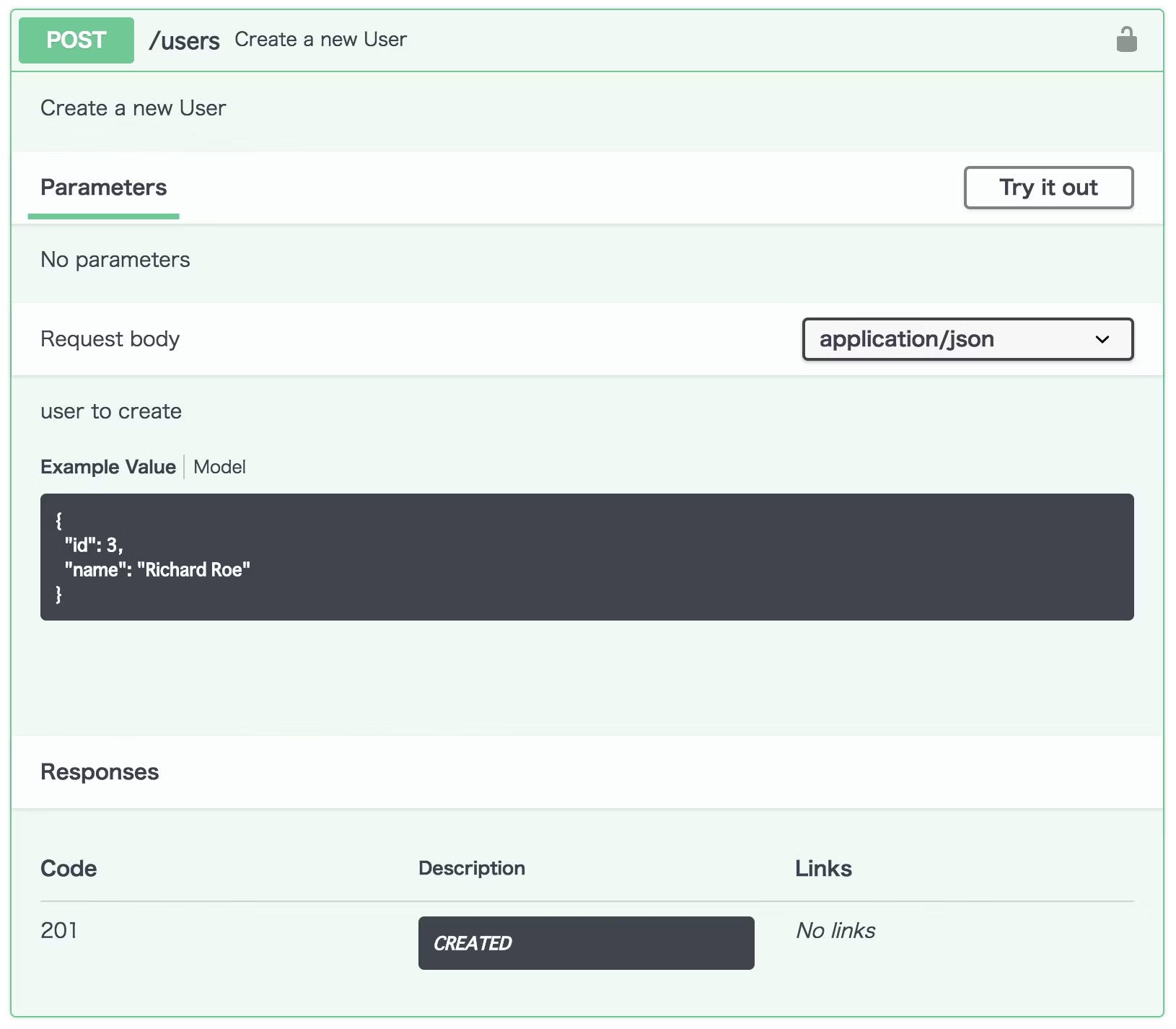
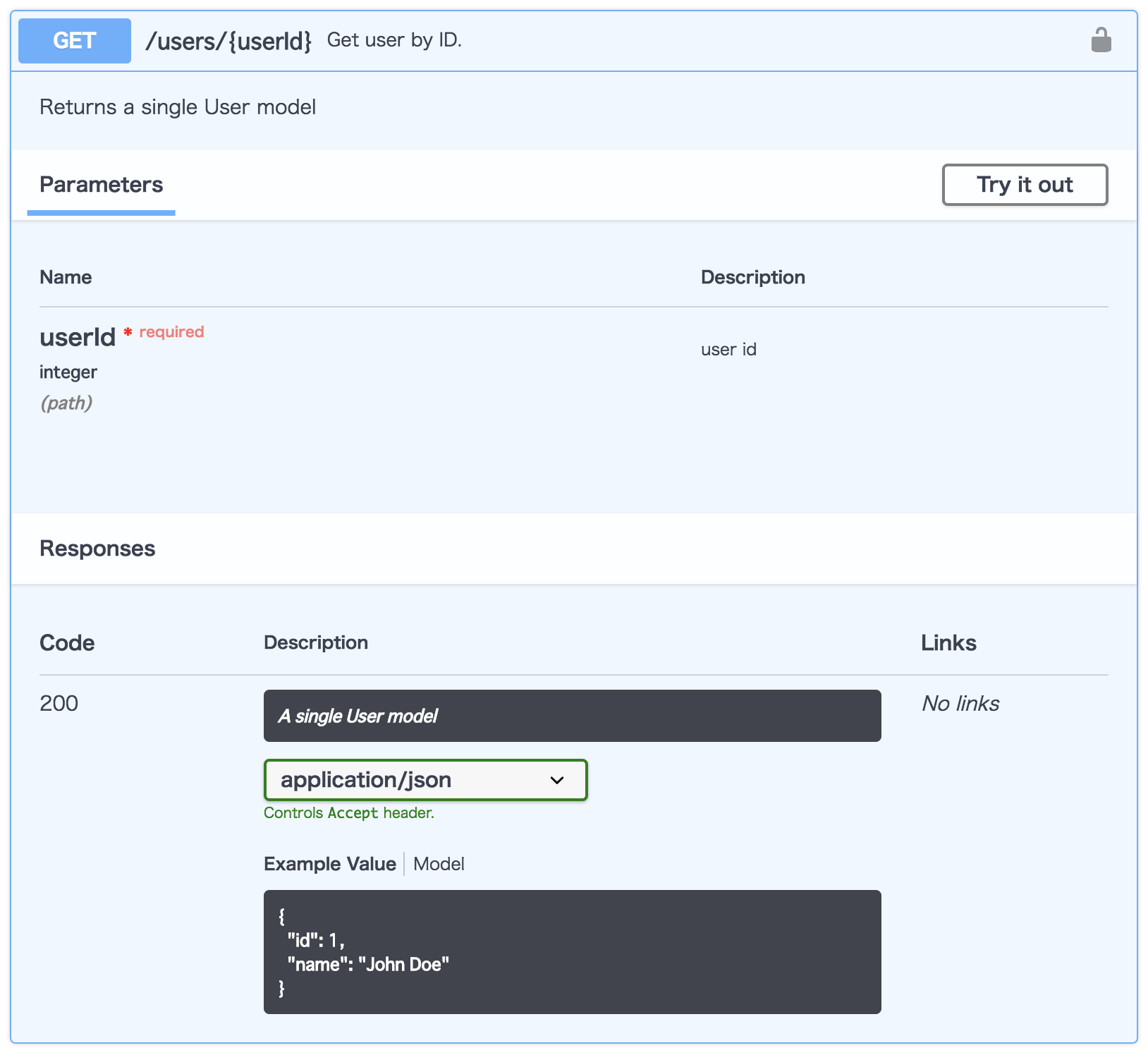
components
ドキュメント: Components Object
-
schemas
:User
やProduct
等のモデル -
requestBodies
: リクエストボディ -
responses
: APIレスポンス -
headers
: リクエストヘッダ -
parameters
: リクエストパラメータ
等、API定義で再利用可能なオブジェクトを定義できる。
components
フィールドに定義したモデルは、paths
で記述したように
$ref: '#/components/schemas/User'
のように参照する。
components:
schemas: # スキーマオブジェクトの定義
User: # モデル名
type: object # 型
required: # 必須フィールド
- id
properties:
id: # プロパティ名
type: integer # 型
format: int64 # フォーマット(int32, int64等)
name:
type: string
Product:
type: object
required:
- id
- price
properties:
id:
type: integer
format: int64
example: 1
name:
type: string
example: Laptop
price:
type: integer
example: 1200
Swagger UIでの表示
Model
エリアに表示され、構造やサンプル値が参照できる。
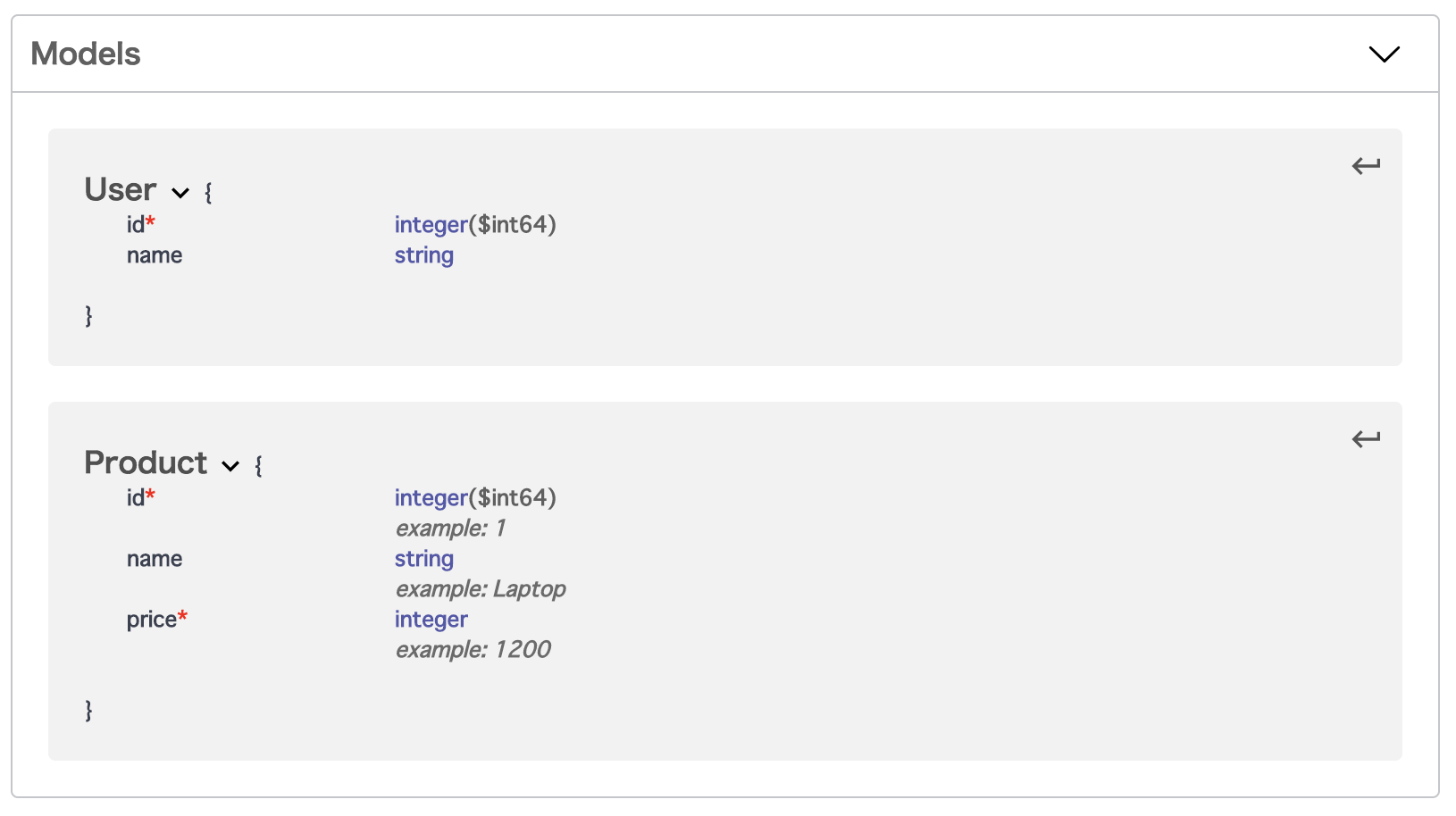
security
ドキュメント: Security Requirement Object
security:
# Non-OAuth setting
- api_key: []
# OAuth setting
- users_auth:
- write:users
- read:users
Swagger UIでの表示
メソッド定義部分に鍵マークが表示される。

tags
ドキュメント: Tag Object
tags:
- name: users
description: Access to Users
- name: products
description: Access to Products
フィールド名 | 必須 | 概要 |
---|---|---|
name | YES | タグ名称。 |
description | タグの説明。 | |
externalDocs | 外部ドキュメント |
Swagger UIでの表示
各エンドポイントでtags
に指定することで、定義をタグで分類できる。
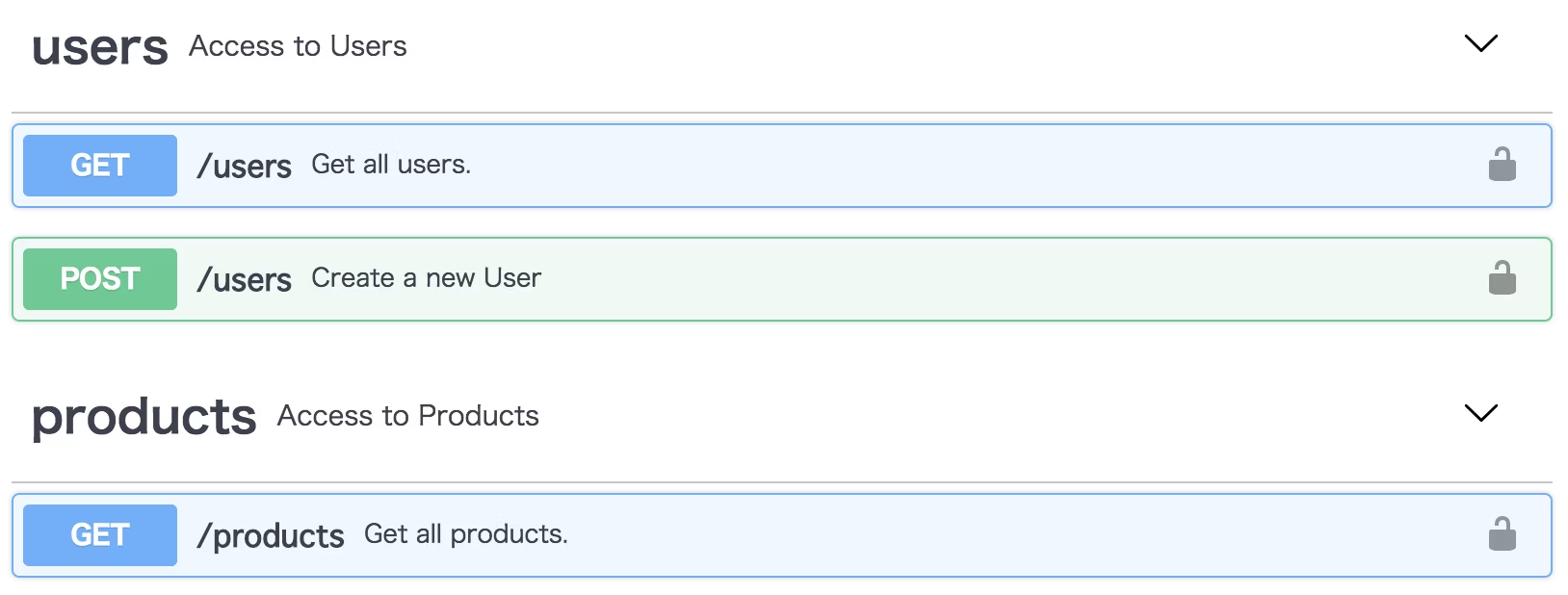
externalDocs
ドキュメント: External Documentation Object
description: Find more info here
url: https://example.com
フィールド名 | 必須 | 概要 |
---|---|---|
description | 外部ドキュメントの説明。 | |
url | YES | 外部ドキュメントのURL。 |
Swagger UIでの表示
ドキュメント上部にリンクが表示される。
まとめ
以上、OpenAPIの基本的な部分を紹介した。
OpenAPIはSwagger 2.0をベースにしているが、記法が異なる部分が多いため、公式ドキュメントをしっかり読み込んで行く必要がある。
手軽にRESTful APIの定義ができるうえ、Amazon API Gatewayと連携し定義のインポート/エクスポートが出来たりと非常に便利なため、ぜひ使ってみてほしい。
Swagger 定義をインポートしてエッジ最適化 API をセットアップする
API Gateway から API をエクスポートする
今回作成したサンプル