Gtk.Imageに表示する
EmbededResourceから画像を取得し表示する
画像をアプリケーションの中でバンドルした状態で取得し表示できます
画像のプロパティからBuildActionをEmbededResourceに変更する必要があります
画像をEmbededResourceから取得し、Stream形式にしてからPixbufに代入すると表示できます
System.Reflection.Assembly myAssembly = System.Reflection.Assembly.GetExecutingAssembly();
Stream myStream = myAssembly.GetManifestResourceStream( myAssembly.GetName().Name + ".photo2.jpg");
Gdk.Pixbuf pix = new Gdk.Pixbuf(myStream);
image1.Pixbuf = pix;
パスから表示する
Fileに絶対パス、相対パスから表示ができる
image1.File = "./photo1.jpg";
Gdk.Pixbuf bmp = new Gdk.Pixbuf("./photo3.jpg",50,50);
image1.Pixbuf = bmp;
Byte形式から表示する
ダウンロードデータがByteの時などに利用
string fileName = "./photo1.jpg";
byte[] buff = null;
FileStream fs = new FileStream(fileName,
FileMode.Open,
FileAccess.Read);
BinaryReader br = new BinaryReader(fs);
long numBytes = new FileInfo(fileName).Length;
buff = br.ReadBytes((int)numBytes);
Gdk.Pixbuf pix = new Gdk.Pixbuf(buff);
image1.Pixbuf = pix;
Webから画像をダウンロードし表示する
string url = "https://cdn.pixabay.com/photo/2022/01/16/15/03/finch-6942278_1280.jpg";
System.Net.WebClient wc = new System.Net.WebClient();
wc.Encoding = System.Text.Encoding.UTF8;
byte[] byteData = wc.DownloadData(url);
wc.Dispose();
Gdk.Pixbuf WindowIcon = new Gdk.Pixbuf(byteData,100,100);
image1.Pixbuf = WindowIcon;
Gdk.Pixbufで画像のサイズを設定する
Gdk.Pixbufは画像読み込み時にサイズを指定できます
Gdk.Pixbuf pix = new Gdk.Pixbuf("./photo3.jpg",100,100);
image1.Pixbuf = pix;
Gtk.Buttonに画像を配置する
EmbededResourceから画像を設定する例
System.Reflection.Assembly myAssembly = System.Reflection.Assembly.GetExecutingAssembly();
btn1.Image = Gtk.Image.LoadFromResource(myAssembly.GetName().Name + ".photo2.jpg");
ボタンに画像を表示する場合
画像がついたラベルを空にする必要があります。
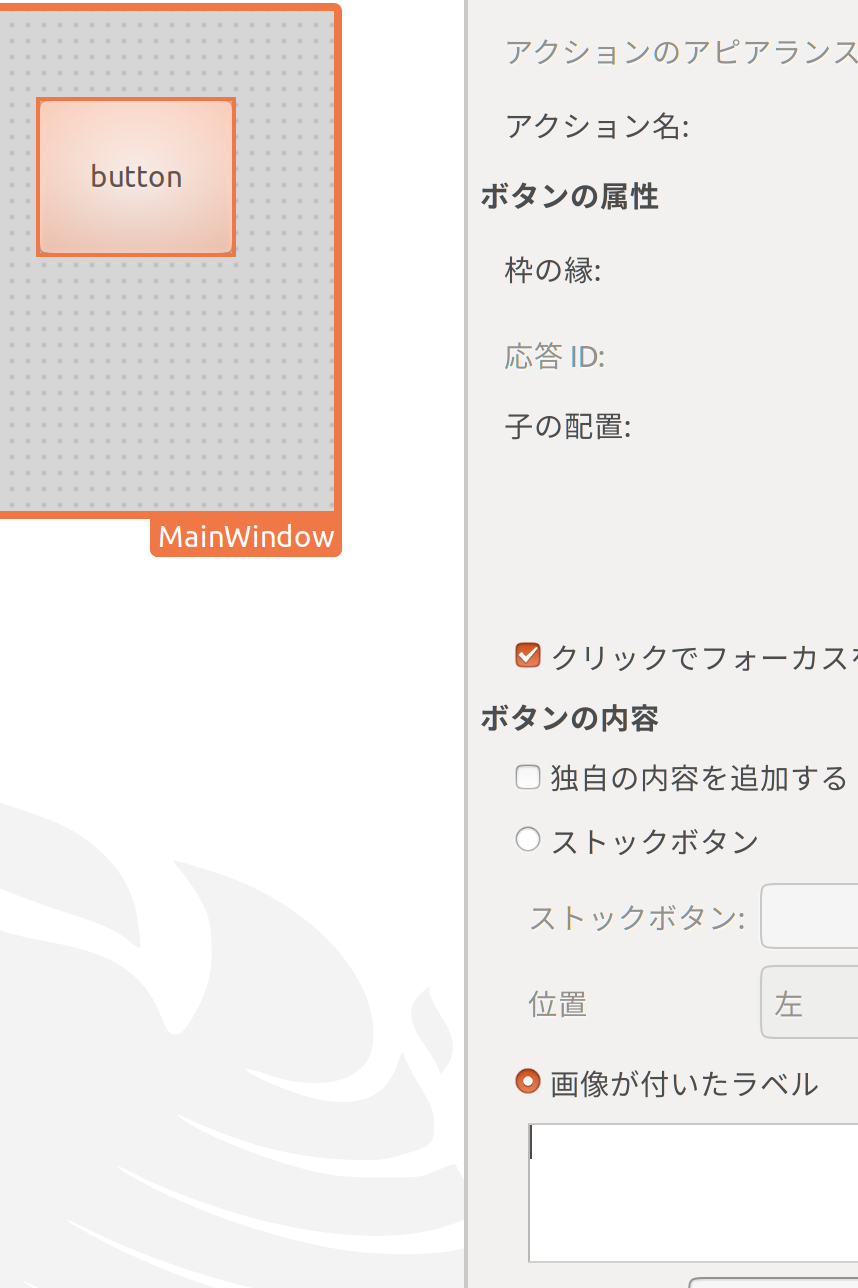
TreeViewのCellに画像を表示する
System.Reflection.Assembly myAssembly = System.Reflection.Assembly.GetExecutingAssembly();
Stream myStream = myAssembly.GetManifestResourceStream( myAssembly.GetName().Name + ".photo2.jpg");
Gdk.Pixbuf pix = new Gdk.Pixbuf(myStream,50,50);
Gtk.ListStore musicListStore = new Gtk.ListStore (typeof (Gdk.Pixbuf));
treeView1.AppendColumn ("Image", new Gtk.CellRendererPixbuf (), "pixbuf", 0);
musicListStore.AppendValues (pix);
treeView1.Model = musicListStore;
TreeViewのCellに画像を表示する その2
public partial class testModel
{
public string? imagePath { get; set; } = null;
}
Gtk.ListStore testModelListStore = new Gtk.ListStore (typeof (testModel));
private void _mkTreeView()
{
Gtk.TreeViewColumn imageColumn = new Gtk.TreeViewColumn ();
imageColumn.Title = "Image";
Gtk.CellRendererPixbuf imageCell = new Gtk.CellRendererPixbuf();
imageColumn.PackStart(imageCell, true);
imageColumn.SetCellDataFunc (imageCell, new Gtk.TreeCellDataFunc (RenderMethod));
treeView1.AppendColumn (imageColumn);
}
private void _mkTreeViewBinding()
{
testModelListStore = new Gtk.ListStore (typeof (testModel));
testModel testModel1 = new testModel();
testModel1.imagePath = "./photo3.jpg";
testModelListStore.AppendValues (testModel1);
treeView1.Model = testModelListStore;
}
private void RenderMethod(Gtk.TreeViewColumn column, Gtk.CellRenderer cell, Gtk.ITreeModel model, Gtk.TreeIter iter)
{
testModel testModel1 = (testModel) model.GetValue (iter, 0);
Gdk.Pixbuf pix = new Gdk.Pixbuf(testModel1.imagePath,50,50);
(cell as Gtk.CellRendererPixbuf).Pixbuf = pix;
}
その他
ファイル名からEmbedResourceの一覧の中からEmbedResourc名を取得する
static public string _getEmbedResourceName(string fileName)
{
Assembly asm = Assembly.GetExecutingAssembly();
string[] resources = asm.GetManifestResourceNames();
foreach (string resource in resources)
{
if (resource.IndexOf("." + fileName) != -1)
{
return resource;
}
}
return "";
}