Visual Studio Code での Extension開発03(拡張機能)
概要
ここでは拡張機能について理解を深めたいと思います
公式ドキュメントと前回分は以下の通りです
-
公式ドキュメント あなたの最初の拡張 Visual Studioコード拡張API
前回までに作成したファイル
extension.ts
import * as vscode from 'vscode';
export function activate(context: vscode.ExtensionContext) {
console.log('Congratulations, your extension "helloworld" is now active!');
let disposable = vscode.commands.registerCommand('extension.helloWorld', () => {
vscode.window.showWarningMessage("WarningMessag");
});
context.subscriptions.push(disposable);
}
export function deactivate() {}
package.json
{
"name": "helloworld",
"displayName": "HelloWorld",
"description": "LEAVE BLANK",
"version": "0.0.1",
"engines": {
"vscode": "^1.33.0"
},
"categories": [
"Other"
],
"activationEvents": [
"onCommand:extension.helloWorld"
],
"main": "./out/extension.js",
"contributes": {
"commands": [
{
"command": "extension.helloWorld",
"title": "myApp"
}
]
},
"scripts": {
"vscode:prepublish": "npm run compile",
"compile": "tsc -p ./",
"watch": "tsc -watch -p ./",
"postinstall": "node ./node_modules/vscode/bin/install",
"test": "npm run compile && node ./node_modules/vscode/bin/test"
},
"devDependencies": {
"typescript": "^3.3.1",
"vscode": "^1.1.28",
"tslint": "^5.12.1",
"@types/node": "^10.12.21",
"@types/mocha": "^2.2.42"
}
}
共通機能
Common Capabilities are important building blocks for your extensions. Almost all extensions use some of these functionalities.
日本語google翻訳
共通機能はあなたの拡張のための重要な構成要素です
ほとんどすべての拡張機能はこれらの機能のいくつかを使用します
共通機能の概説
no | 機能 | 概要 |
---|---|---|
1 | Command | コマンドメニューを開いてコマンドを実行する カスタムキーバインディングをコマンドにバインドする 右クリックしてコンテキストメニューのコマンドを呼び出す |
2 | Configuration | Contribution Pointを使用した拡張機能固有の設定 |
3 | Keybinding | 拡張機能のカスタムキーバインドの設定 |
4 | Context Menu | 右クリック時のコンテキストメニューの挙動を制御 |
5 | Data Storage | データを保存 |
6 | Display Notifications | 通知の表示 |
7 | Quick Pick | ユーザの入力補助 |
8 | File Picker | ユーザのファイル選択補助 |
9 | Output Channel | 出力パネル |
10 | Progress API | 進行状況の更新 |
共通機能を詳しく
1 Command
コマンドは一意の識別子を持つ関数(コマンドハンドラとも呼ばれる)
コマンドの登録方法については以下の通りです
-
registerCommand
またはregisterTextEditorCommand
関数を使用して、コマンドハンドラをエディタに登録
(※メソッドについて➡ vscode-api) -
コマンド識別子を package.json のcontributes.commands.title に結びつける
2 Configuration
設定項目 Contribution Points を package.json のcontributes
以下に設定します
An extension can contribute extension-specific settings with the contributes.configuration
日本語google翻訳
エクステンションはcontrib.configurationでエクステンション特有の設定を提供することができます
Contribution Pointsについて
設定項目がたくさんあります
いまは以下の3つにとどめます
- commands
- menus
- keybindings
1 commands | コマンドの登録とカテゴライズ
設定箇所:contributes.commands.category
起動するタイトルとコマンドからなるエントリをコマンドパレットに設定できます
オプションで、コマンドタイトルの前に付けるカテゴリ文字列を定義し、グループ化もできます
項目名 | 概要 | |
---|---|---|
contributes.commands. | command | コマンドの名前 |
^ | title | コマンドパレットに表示させる名前 |
^ | category | カテゴリ名 |
✓helloworld\ package.json のコマンドにカテゴリを追加してみます
contributes.commands.category
に "group1" を追加
⇩
実行結果
コマンドパレットに group1:myApp と表示されるようになりました
(さらにwhen節
によってコマンドパレットに表示されるタイミングも設定できるようです)
2 menus | メニューの設定
設定箇所: contributes.menus
コマンドのメニュー項目をエディタまたはエクスプローラに設定できます
✓とりあえずサンプルをhelloworld\ package.json 反映させてみます
"menus": {
"editor/title": [{
"when": "resourceLangId == markdown",
"command": "markdown.showPreview",
"alt": "markdown.showPreviewToSide",
"group": "navigation"
}]
}
⇩
実行結果
メニューバーにマークダウンのプレビューを開くアイコンが設定されました
✓ editor/ title を editor/ context に変更してみます
⇩
実行結果
右クリック時のコンテキストメニューの中に項目が追加されました
✓extension.tsで作成した「helloWorld」をコンテキストメニューに加えてみます
commandをextension.helloWorld
にします
"menus": {
"editor/context": [{
"command": "extension.helloWorld",
"group": "navigation"
}]
}
⇩
実行結果
右クリック時のコンテキストメニューの中に myApp が追加されました
✓ editor/context を editor/title に変更してみます
⇩
実行結果
メニューバーに myApp が追加されました
項目名 | 概要 | |
---|---|---|
contributes.menus. | commandPalette | グローバルなコマンドパレットを設定対象にする |
^ | editor/context | エディタのコンテキストメニューを設定対象にする |
^ | editor/title | エディタのタイトルメニューバーを設定対象にする |
contributes.menus.[設定対象]. | when | 条件節を設定する |
^ | command | コマンドを割り当てる 必須項目 |
^ | group | メニュー項目のソートとグループ化を定義 navigationグループは常にメニューの先頭に表示される |
※when についてはまた別の機会で詳細の理解を深める必要がありそうです |
3 keybindings | キーボードショートカットの設定
設定箇所: contributes.keybindings
✓helloworld\ package.json に以下を追記します
"keybindings": [{
"command": "extension.helloWorld",
"key": "ctrl+f1",
"mac": "cmd+f1"
}]
⇩
実行結果
ctrl+f1
でextension.helloWorld
が呼び出されました
3 Keybinding
キーボードショートカットの競合に注意が必要
結構キーボードショートカットの割り当ては大変かもしれないです
さらに詳しく Visual Studioコードの主なバインディング
4 Context Menu
上述しました
5 Data Storage
割愛します
6 Display Notifications
以下の通知表示があります
- window.showInformationMessage
- window.showWarningMessage
- window.showErrorMessage
7 Quick Pick
ユーザの入力補助を実現します
上記を参考にしながらサンプルを動かしたいと思います
githubからファイル群をダウンロード
ローカルでquickinput-sample
フォルダを開きF5
を押下したところ
以下のエラーが発生しました
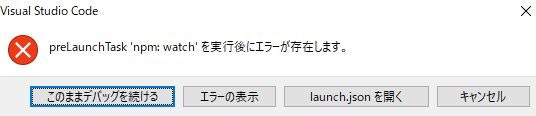
npm install
をコマンドラインで実行し動作するようになりました
コード内に記載されているキーワードと参考サイトを列挙します
※いったん保留
-
Promise
-
async
- Qiita async await の使い方
-
ExtensionContext
-
subscriptions.push
-
window.createQuickPick
-
items
-
onDidChangeSelection
-
catch
-
onDidHide
-
dispose
-
show
8 File Picker
showOpenDialog(options: OpenDialogOptions): Thenable<Uri[] | undefined>
上記のメソッドでファイルオープンダイアログをユーザに表示することができます
9 Output Channel
割愛します
10 Progress API
vscode.Progress
で進捗バーを表示できます
まとめ
package.json
は設定
extension.ts
はソース
ということが理解できました
そしてextension.ts
はtypeScript
,javaScript
が土台にあって
vscode-api
を絡めていくイメージが持てました
今更ですが、typeScript
,javaScript
の基礎がないとextension.ts
が読めません
なので、少し迂回します
typeScript
,javaScript
の基礎学習に
参考
-
公式ドキュメント 共通の機能| Visual Studioコード拡張API
-
quickinput githubに用意されているサンプル
-
Qiita Promiseについて0から勉強してみた
-
Qiita async await の使い方
-
progress githubに用意されているサンプル