はじめに
MTCNNを使って顔検出するときにどんな情報が得られるのか知りたくて、ラズパイでサンプルコードを実行してみました。
全ての情報は以下にあります。
実行環境
ライブラリ | バージョン |
---|---|
python | 3.7.3 |
mtcnn | 0.1.1 |
opencv-python | 4.5.3.56 |
tensorflow | 1.14.0 |
やったこと
example.pyの内容を少しいじって、以下のコードを実行しました。
import cv2
from mtcnn import MTCNN
filename = "ivan.jpg"
output = "drow_" + filename
detector = MTCNN()
image = cv2.cvtColor(cv2.imread(filename), cv2.COLOR_BGR2RGB)
result = detector.detect_faces(image)
# Result is an array with all the bounding boxes detected. We know that for 'ivan.jpg' there is only one.
bounding_box = result[0]['box']
keypoints = result[0]['keypoints']
cv2.rectangle(image,
(bounding_box[0], bounding_box[1]),
(bounding_box[0]+bounding_box[2], bounding_box[1] + bounding_box[3]),
(0,155,255),
2)
cv2.circle(image,(keypoints['left_eye']), 2, (0,155,255), 2)
cv2.circle(image,(keypoints['right_eye']), 2, (0,155,255), 2)
cv2.circle(image,(keypoints['nose']), 2, (0,155,255), 2)
cv2.circle(image,(keypoints['mouth_left']), 2, (0,155,255), 2)
cv2.circle(image,(keypoints['mouth_right']), 2, (0,155,255), 2)
cv2.imwrite(output, cv2.cvtColor(image, cv2.COLOR_RGB2BGR))
print(result)
実行結果
顔を検出した箇所にマーキングされました。画像のサイズは561×561のファイルです。
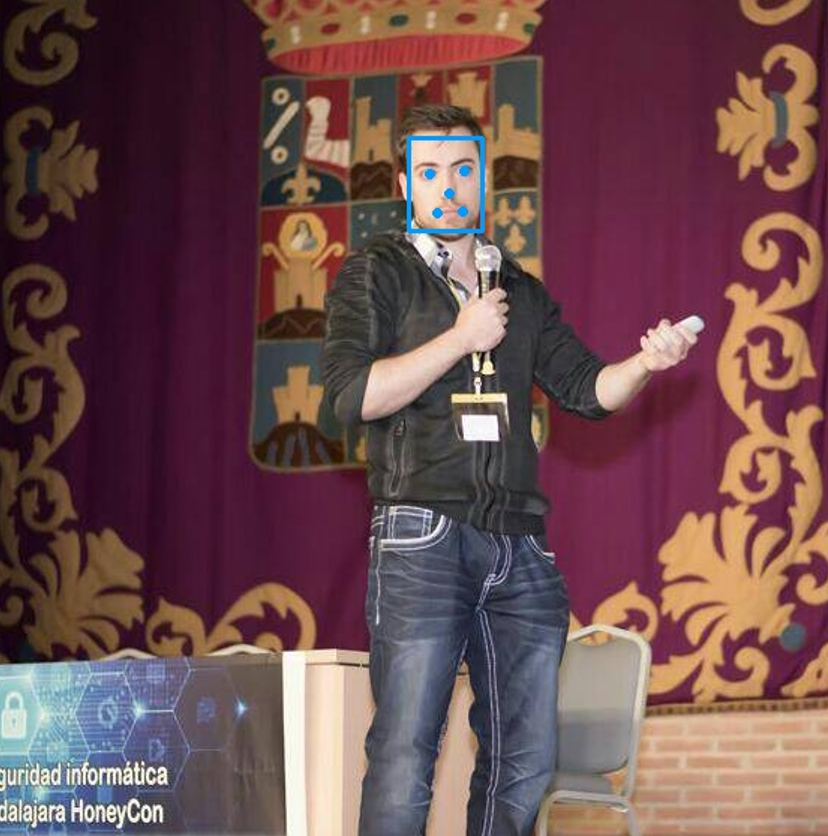
resultは以下のように出力されました。※見やすいように改行入れてます。
[{'box': [277, 93, 49, 62], 'confidence': 0.9999709725379944,
'keypoints': {'left_eye': (291, 117), 'right_eye': (314, 115),
'nose': (304, 130), 'mouth_left': (296, 143), 'mouth_right': (313, 142)}},
{'box': [307, 173, 37, 55], 'confidence': 0.8657229542732239,
'keypoints': {'left_eye': (327, 194), 'right_eye': (339, 191),
'nose': (341, 199), 'mouth_left': (334, 215), 'mouth_right': (342, 213)}}]
なんだか2か所の顔を検出しているように見えるので、result[1]の方を書き込むようにして実行してみました。
どうやらマイクを持った右手で顔検出しているようです。
あんまり精度が良くないのかもしれません。サンプルの画像なのできちんと検出するものを置いておけばいいのに。
気になった点
コード実行時、以下のエラーとワーニングが出てました。このままでも動いたのでスルーしましたが、いつか気が向いたら調べようと思います。
E tensorflow/core/platform/hadoop/hadoop_file_system.cc:132]
HadoopFileSystem load error: libhdfs.so: cannot open shared object file: No such file or directory
WARNING:tensorflow:From /home/pi/.local/lib/python3.7/site-packages/tensorflow_core/python/ops/resource_variable_ops.py:1630:
calling BaseResourceVariable.__init__ (from tensorflow.python.ops.resource_variable_ops) with constraint is deprecated and will be removed in a future version.
Instructions for updating:
If using Keras pass *_constraint arguments to layers.