はじめに
下記の記事で UICollectionView
でドット絵を表示する方法を紹介したのですがやっぱりドット絵配列を作るのがめんどくさすぎるので今回は画像から読み込む方法をご紹介します。
UICollectionViewでドット絵表示
こんな感じで表示します。
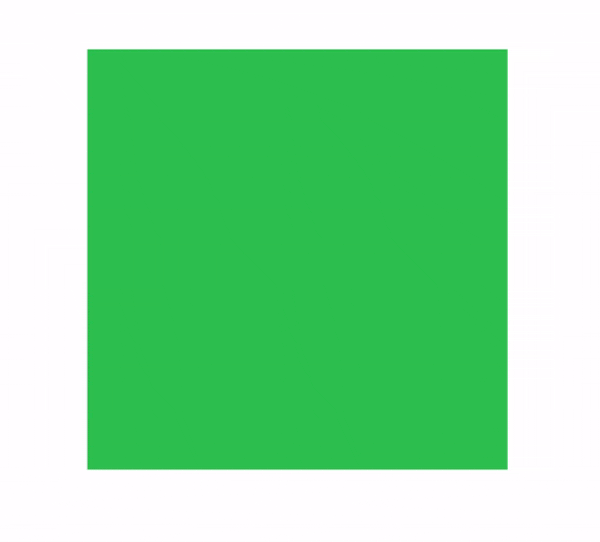
画像読み込み
画像の読み込み方法はこんな感じです(たまにうまく読み込めない画像もあります)。
extension UIImage {
func colorsData() -> [[UInt8]] {
let pixelDataByteSize = 4
let pixelData = self.cgImage!.dataProvider!.data
let length = CFDataGetLength(pixelData)
var rawData = [UInt8](repeating: 0, count: length)
CFDataGetBytes(pixelData, CFRange(location: 0, length: length), &rawData)
return rawData.chunked(by: pixelDataByteSize)
}
}
extension Array {
func chunked(by chunkSize: Int) -> [[Element]] {
return stride(from: 0, to: self.count, by: chunkSize).map {
Array(self[$0..<Swift.min($0 + chunkSize, self.count)])
}
}
}
画像の読み込み方法は下記の記事を参考にさせていただきました。
配列の分割は下記を参考にしました。
ドット絵表示
今回使った画像はこちら。
たけのこ | きのこ |
---|---|
![]() |
![]() |
表示処理以外はほぼ前回と同じです。
// 型を[[UInt8]]に変更
private var dataList: [[UInt8]] = []
private var mushroom: [[UInt8]] = []
private var bambooshoot: [[UInt8]] = []
// データを画像から読み込み
mushroom = UIImage(named: "mushroom")!.colorsData()
bambooshoot = UIImage(named: "bambooshoot")!.colorsData()
// 表示処理修正
dataSource = UICollectionViewDiffableDataSource<Section, Int>(collectionView: collectionView) {
(collectionView: UICollectionView, indexPath: IndexPath, item: Int) -> UICollectionViewCell? in
let cell = collectionView.dequeueReusableCell(withReuseIdentifier: "Cell", for: indexPath)
let data = self.dataList[item]
if data.isEmpty {
cell.backgroundColor = .systemGreen
} else {
let r = CGFloat(data[0]) / 255
let g = CGFloat(data[1]) / 255
let b = CGFloat(data[2]) / 255
let a = CGFloat(data[3]) / 255
cell.backgroundColor = UIColor(red: r, green: g, blue: b, alpha: a)
}
return cell
}
おわりに
これでより手軽に UICollectionView
を使ってドット絵をシュバババと表示できるようになりました
64×64 の画像でもやってみましたがリロード処理が重すぎてうまくアニメーションしなかったので 32×32 の画像がちょうどいいかもしれません。