こんばんは、SNS mixiのAndroidアプリを書いているAKKUMAです。
今年はAndroid 7.1で追加された、ショートカットメニューを表示するAPIであるApp Shortcutsを使ってみた話について書きます。
App Shortcuts
Android 7.1(SDK 25)で追加された、ランチャー上のアプリアイコンを長押しすると出るショートカットメニューの機能です。
タップすることで特定のIntentを起動できるようです。メニュー右端の=アイコンをドラッグすることでショートカットをアイコン化をすることもできみたいです。
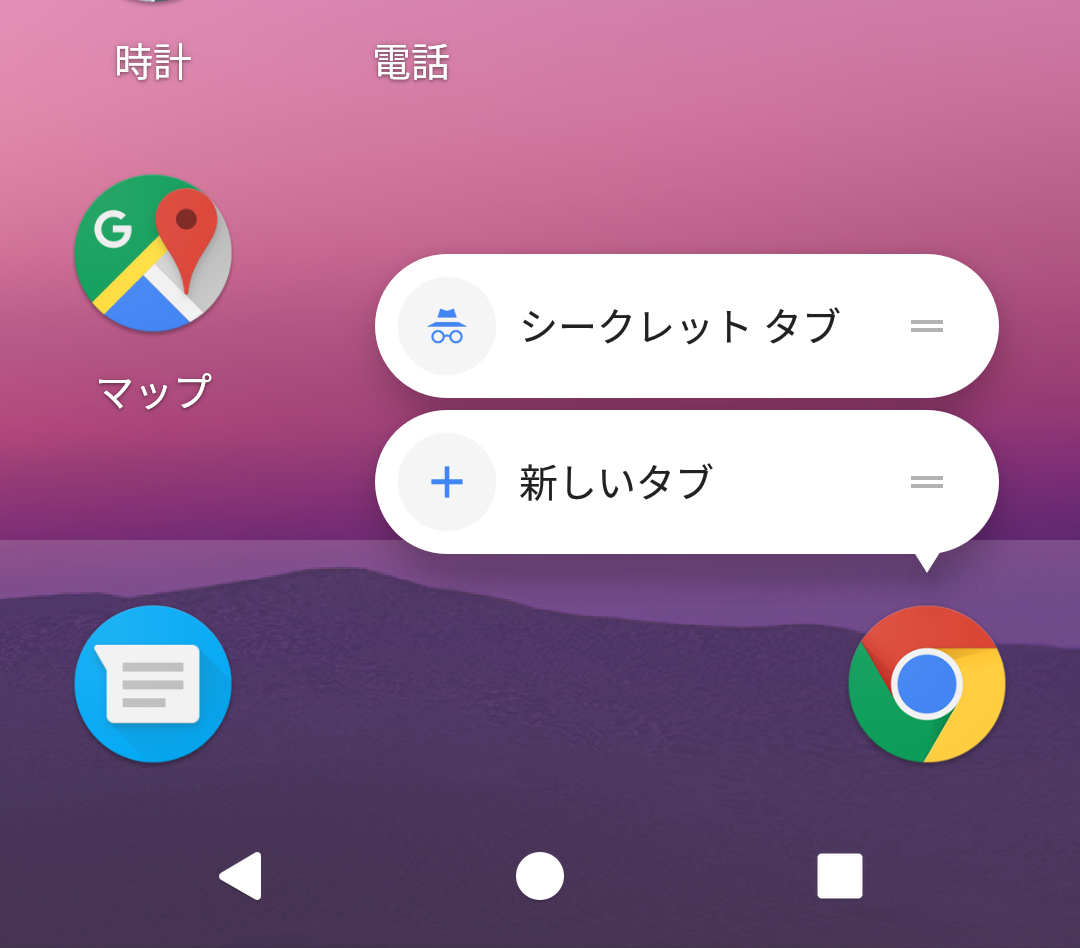
使ってみる
ショートカットの種類が2つあり、Static Shortcuts と Dynamic Shortcuts に分かれているようです。
Static Short は xml 上で定義する静的なショートカットメニューを定義します。
Dynamic Shortcuts は ShortcutManager を利用し、動的にショートカットメニューを設定します。
SDK Level
まずは compileSdkVersion
を 25 に設定する必要があります。
android {
compileSdkVersion 25
}
Static Shortcuts
xmlを利用し静的なショートカットメニューを定義してみます。
res/xml/shortcuts.xml
に配置します。valuesではないので注意。
android:icon
にアイコンを指定、android:shortcutShortLabel
等に文字列を指定できます。
文字列はこのxml内には直接書けないのでres/values/strings.xml
に定義しましょう。
intent
タグ内でタップ時に発動するIntentを定義できます。
<?xml version="1.0" encoding="utf-8"?>
<shortcuts xmlns:android="http://schemas.android.com/apk/res/android">
<shortcut
android:shortcutId="hoge"
android:enabled="true"
android:icon="@drawable/ic_motorcycle_black_24dp"
android:shortcutShortLabel="@string/shortcut_short_label"
android:shortcutLongLabel="@string/shortcut_long_label"
android:shortcutDisabledMessage="@string/shortcut_disabled_message">
<intent
android:action="info.akkuma.appshortcutssample.action.HOGE"
android:targetPackage="info.akkuma.appshortcutssample"
android:targetClass="info.akkuma.appshortcutssample.MainActivity" />
</shortcut>
</shortcuts>
次に AndroidManifest.xml
上に先程のshortcuts.xml
を登録します。
ランチャーに表示できないActivityに入れてもしょうがないので
<category android:name="android.intent.category.LAUNCHER" />
が付いているActivityに入れましょう。
<activity android:name=".MainActivity">
<intent-filter>
<action android:name="android.intent.action.MAIN" />
<category android:name="android.intent.category.LAUNCHER" />
</intent-filter>
<meta-data android:name="android.app.shortcuts"
android:resource="@xml/shortcuts" />
</activity>
これだけでショートカットメニューができます。
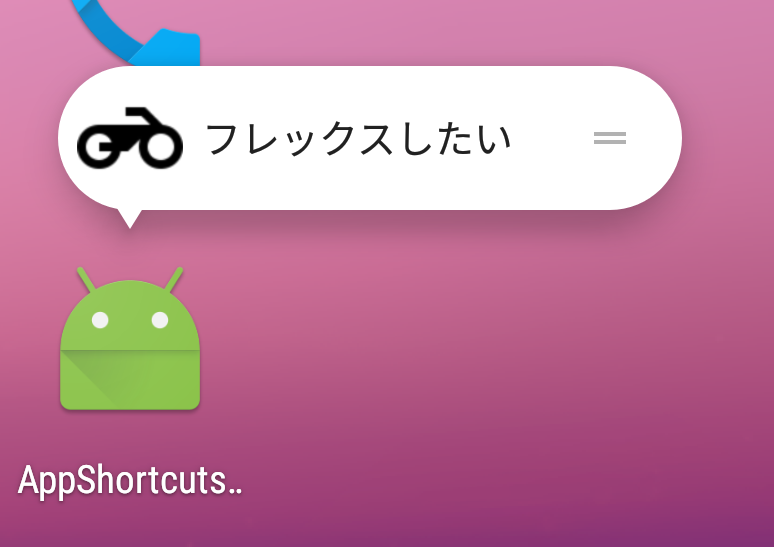
Dynamic Shortcuts
次はShortcutManagerを利用し動的にメニューを追加してみます。
ShortcutManager
を利用してショートカットメニューを設定したり追加したりします。
ショートカット内容はShortcutInfo
を使い作成しますが、IntentにはActionを指定しないと例外がthrow
されるので要注意です。
また、最大数を超えるショートカットメニューを追加しようとした時もthrow
されます。ショートカットは合計4つ以下でなければならないようです。
既にある同一IDのショートカットメニューを追加した場合は、無視されるようです。
ShortcutManager manager = (ShortcutManager) context.getSystemService(SHORTCUT_SERVICE);
int shortcutCount = manager.getDynamicShortcuts().size();
Intent intent = new Intent(Intent.ACTION_VIEW, null, this, MainActivity.class);
ShortcutInfo info = new ShortcutInfo.Builder(context, "fuga" + shortcutCount)
.setShortLabel("LGTM " + shortcutCount)
.setIcon(Icon.createWithResource(context, R.drawable.ic_thumb_up_black_24dp))
.setIntent(intent)
.build();
manager.addDynamicShortcuts(Collections.singletonList(info));
