敵オブジェクトと衝突の際にプレイヤーを爆発四散させる
敵とぶつかった際にプレイヤーをDestroyする処理は簡単ですが、それプラス爆発のエフェクトを出すようにしました。仕組みとしてはEnemyタグのあるオブジェクトに接触したら、プレイヤーをDestroyして、なおかつ直前の座標に爆発アニメーションを入れたPrefabを生成する形です。
衝突判定があればプレイヤーにアタッチしているAnimationを爆発エフェクトにしても良かったのですが、それだと何故か上手く行かなかったので、上画像のような力技です。
プレイヤー側の設定
以下がプレイヤーにアタッチしているScriptです。
汚いところも多いけどスルーしておいてください。下の方に今回の記事に関係のある部分だけを抜き出しておきます。
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
using UnityEngine.SceneManagement;
public class Player : MonoBehaviour
{
//変数定義
float Slide = 30f; //横移動の力の強さ
float MaxSpeed = 5f; //最大の移動スピード
float flap = 5000f; //ジャンプ時の力の大きさ
Rigidbody2D rb2d;
bool jump = false; //ジャンプが可能かの判定
SpriteRenderer Spr; // 表示スプライト
Animator anm; // アニメーションコンポーネント
GameObject obj;
void Start()
{
//コンポーネント読み込み
rb2d = GetComponent<Rigidbody2D>();
anm = GetComponent<Animator>();
Spr = GetComponent<SpriteRenderer>();
obj = (GameObject)Resources.Load("Prefabs/Explode"); //死亡時のアニメーションプレハブを読み込み
}
void Update()
{
//キーボード操作
if (Input.GetKey(KeyCode.RightArrow))//右入力時
{
rb2d.AddForce(Vector2.right * Slide);
anm.Play("PlayerRun"); // 右向きアニメーション
Spr.flipX = false; // 左右反転オフ
}
else if (Input.GetKey(KeyCode.LeftArrow)) //左入力時
{
//左入力時
rb2d.AddForce(Vector2.left * Slide);
anm.Play("PlayerRun"); // 左向きアニメーション
Spr.flipX = true; // 左右反転オン
}
if (Input.GetKeyDown("space") && jump == false)
{
//ジャンプ時 ※(未実装)ジャンプかつ横移動時はPlayerJumpアニメーションを適用したい
rb2d.AddForce(Vector2.up * flap);
jump = true;
anm.Play("PlayerJump"); // 右向きアニメーション
}
//静止状態のときの処理
if (rb2d.velocity.magnitude == 0f)
{
anm.Play("PlayerIdle"); // アイドルアニメーション
}
// 速度制限 MaxSpeedを超えたら処理
if (rb2d.velocity.magnitude > MaxSpeed)
{
//MaxSpeedを掛けて最大速度までしか出さないようにする
rb2d.velocity = rb2d.velocity.normalized * MaxSpeed;
}
}
//設置判定があればジャンプ判定をfalseへ変更
void OnCollisionEnter2D(Collision2D col)
{
jump = false; //接地中はジャンプのフラグをNGにする
if (col.gameObject.tag == "Enemy")
{
Destroy(this.gameObject); //playerを破壊する
//explodeプレハブを生成する。
Instantiate(obj, new Vector3(rb2d.transform.position.x, rb2d.transform.position.y), Quaternion.identity);
}
}
}
下のScriptが上のもののうち、本記事に関係のあるところの抜粋です。
public class Player : MonoBehaviour
{
//変数定義
GameObject obj;
void Start()
{
//コンポーネント読み込み
obj = (GameObject)Resources.Load("Prefabs/Explode"); //死亡時のアニメーションプレハブを読み込み
}
void OnCollisionEnter2D(Collision2D col)
{
if (col.gameObject.tag == "Enemy")
{
Destroy(this.gameObject); //playerを破壊する
//explodeプレハブを生成する。
Instantiate(obj, new Vector3(rb2d.transform.position.x, rb2d.transform.position.y), Quaternion.identity);
}
}
}
爆発Animationの用意と保存場所
AnimationのアセットはSunny Landを利用しています。
Animationに関しては「Unity でスプライトアニメーションを作る」が参考になります。
まずはAnimationを作ってプロジェクトウィンドウにドラッグしてプレハブ化します。このプレハブは呼び出し可能にするため、下記画像のようにResourcesフォルダを作って、直下に置くか、適当にフォルダを作って置きます。(Resources内に無いと呼び出しが出来ないため)
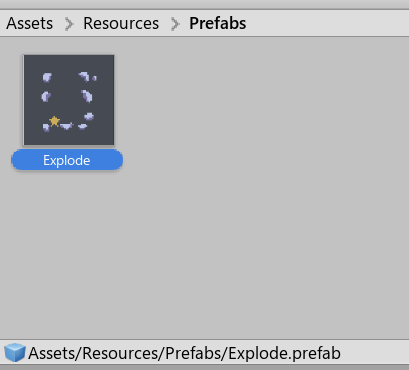
爆発の処理内容
まずは「GameObject obj;」で入れ物を作ってあげて
「obj = (GameObject)Resources.Load("Prefabs/Explode");」で入れ物に爆発のプレハブを入れる
最後にEnemyタグが付いているオブジェクト衝突した際に「Instantiate(obj, new Vector3(rb2d.transform.position.x, rb2d.transform.position.y), Quaternion.identity);」でプレイヤーがいた場所にプレハブを呼び出します。