CollectionViewControllerのCell表示
CollectionViewやTableViewのcellの表示にいつも悩むので、色々試してまとめました。
試したパターン
- StoryboardのCollectionViewControllerのなかにcell作る
- xib作ってCollectionViewControllerで読み込み
- xib作ってCustomCellで読み込み
大前提
以下三つの関数の設定
// MARK: UICollectionViewDataSource
override func numberOfSections(in collectionView: UICollectionView) -> Int {
return 1
}
override func collectionView(_ collectionView: UICollectionView, numberOfItemsInSection section: Int) -> Int {
return 3
}
override func collectionView(_ collectionView: UICollectionView, cellForItemAt indexPath: IndexPath) -> UICollectionViewCell {
let cell = collectionView.dequeueReusableCell(withReuseIdentifier: reuseIdentifier, for: indexPath)
return cell
}
それとCollectionViewControllerのクラスがきちんとStoryboardに紐づいていることなど
方法その1: CollectionViewControllerのCollectionView内にCell配置
CollectionViewControllerのCollectionView内にCell配置 + CellのreuseIdentifier設定
一番簡単です
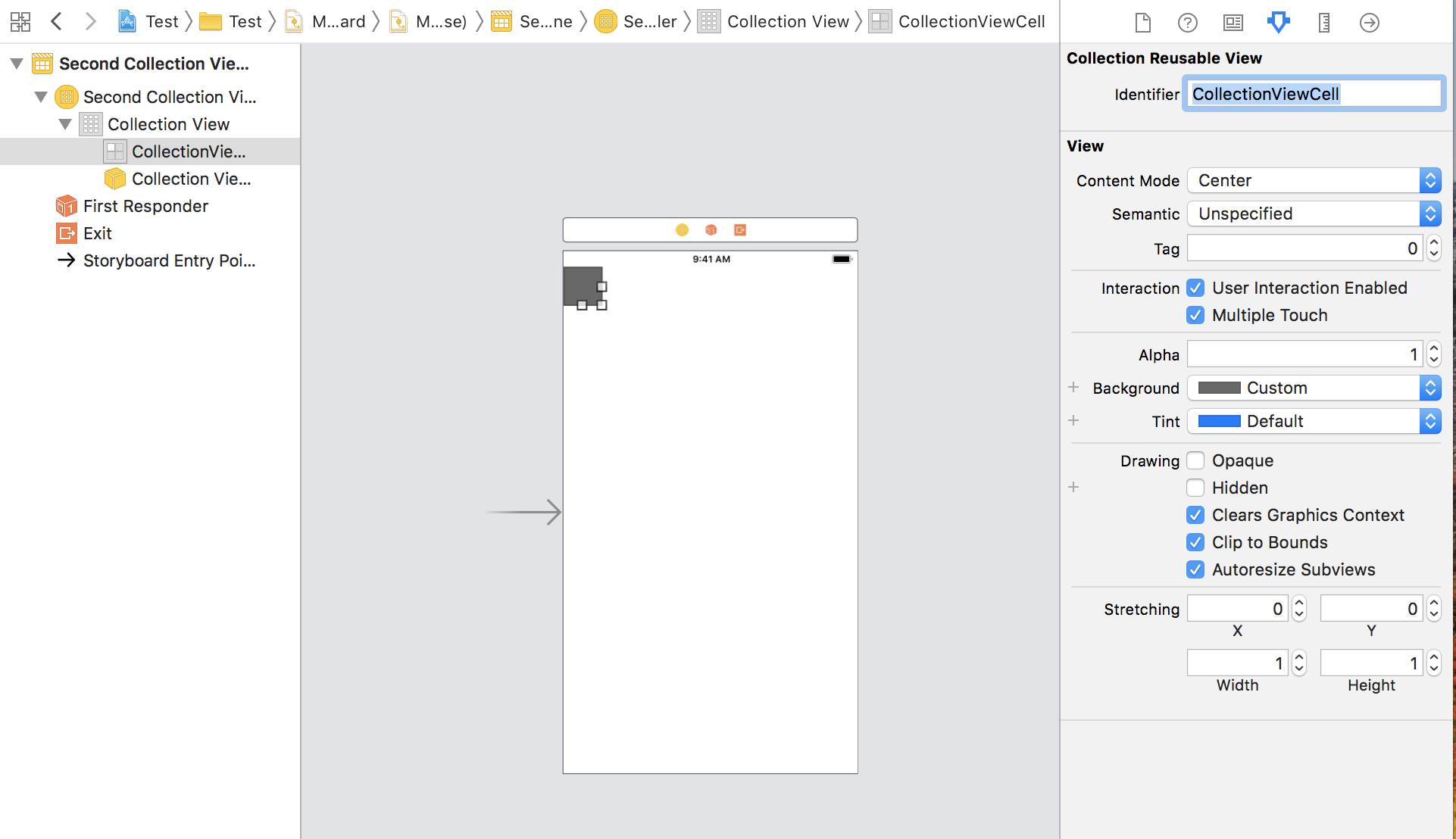
上のやり方のcellをカスタムクラスに紐付けるには
- CustomCellクラスを作成して、CellのCustomClassに設定
CustomClassの名前はreuseIdentifierと異なっていても良い
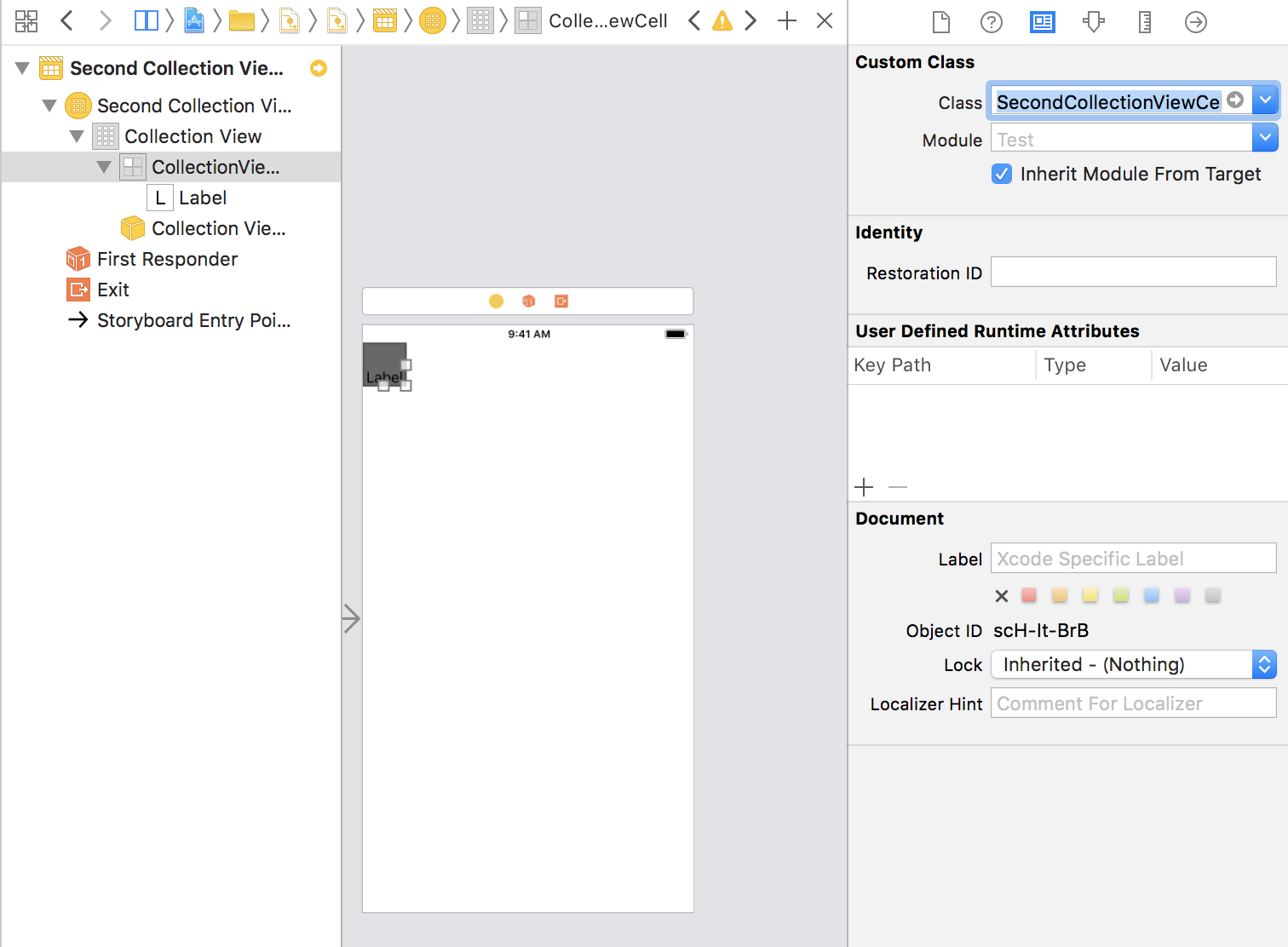
方法その2: xib + CollectionViewControllerでnib登録
UINib(nibName:)でxib読み込み(xibにreuseIdentifierの設定不要) + viewDidLoadでregister
補足: ファイル名をreuseIdentifierと違うものにして、xibないのcellのreuseIdentifierを設定してもエラー
private let reuseIdentifier = "CollectionViewCell"
override func viewDidLoad() {
super.viewDidLoad()
let nib = UINib(nibName: "xibのファイル名(.xibいらない)", bundle: nil)
collectionView!.register(nib, forCellWithReuseIdentifier: reuseIdentifier)
}
これでとりあえずxib通り表示される
上のやり方のcellをカスタムクラスに紐付けるには
UICollectionViewCellを継承したCustomクラスを作成 + xibのcellのCustom Classに作成したCustomクラスを設定
あとは普通にlabelなどxibからコードにつなぎこんで利用
方法その3: customのCollectionViewCellでxib読み込み
UICollectionViewCellのxib作成 + UICollectionViewCellのカスタムクラスを作成してxib読み込み + CollectionViewController側でcellの登録
xibはCustomClassやFilesOwner等設定しなくてもいいです。
/// カスタムcell
class CollectionViewCell: UICollectionViewCell {
override init(frame: CGRect) {
super.init(frame: frame)
// ここでxib読み込み xibの名前はCustomCellの名前と同じじゃなくても良い
Bundle.main.loadNibNamed("TestCollectionViewCell", owner: self, options: nil)
}
// これがないとエラー
required init?(coder aDecoder: NSCoder) {
super.init(coder: aDecoder)
// fatalError("init(coder:) has not been implemented") // これでもよい
}
}
private let reuseIdentifier = "CollectionViewCell"
// CollectionViewController側
override func viewDidLoad() {
super.viewDidLoad()
collectionView!.register(CollectionViewCell.self, forCellWithReuseIdentifier: reuseIdentifier)
}
上のやり方のcellをカスタムクラスに紐付けるには
- xib内のContentViewのCustom classを設定
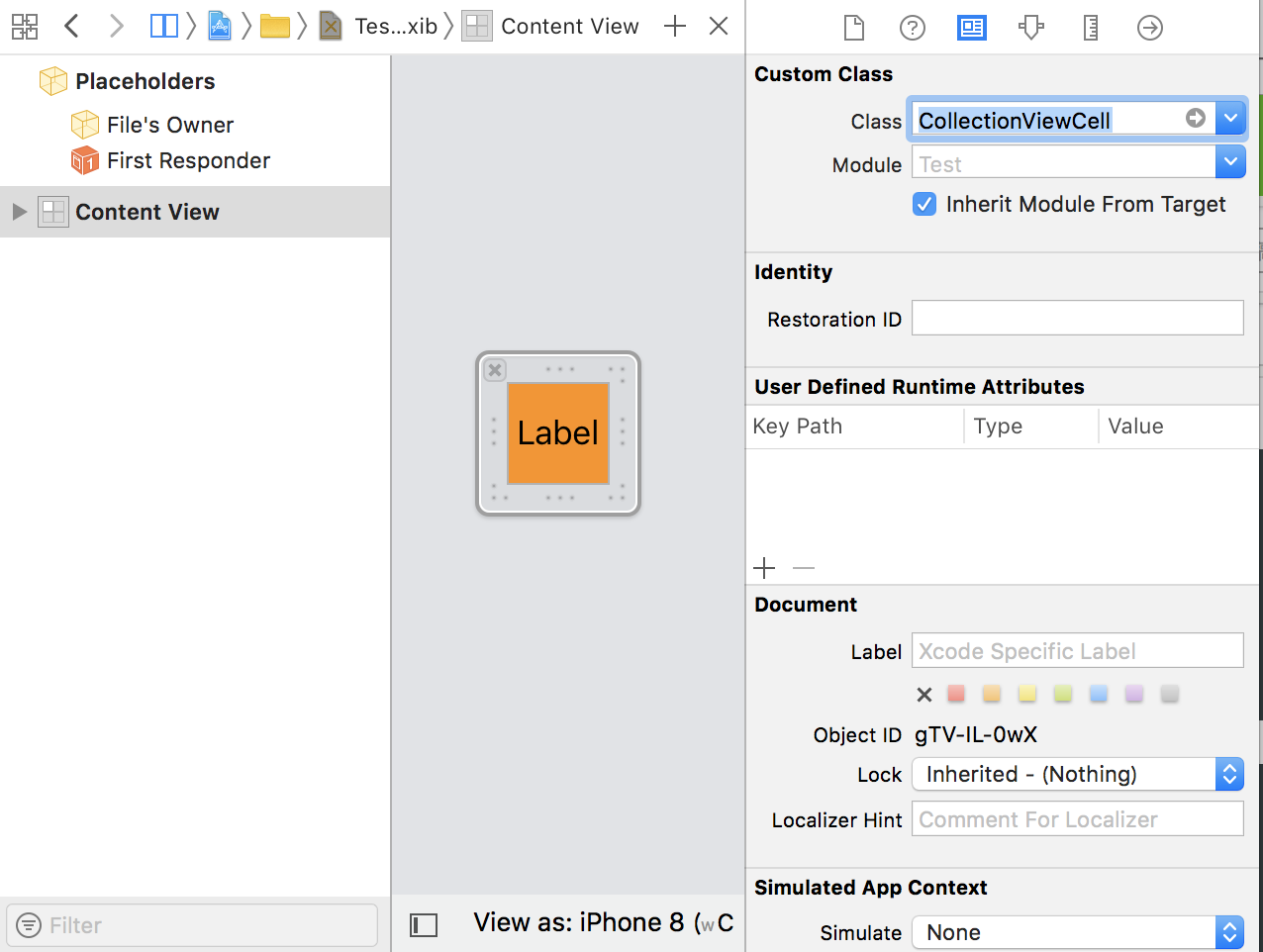
- File's Ownerだけを設定しても、CustomCellクラスにlabelを紐付けできたのですが、なぜかawakeFromNibなどで変更できません。collection viewなどからの変更は可能でした。
終わりに
他に違ったやり方あれば教えてください
xibはジブと読むらしい