実装イメージ
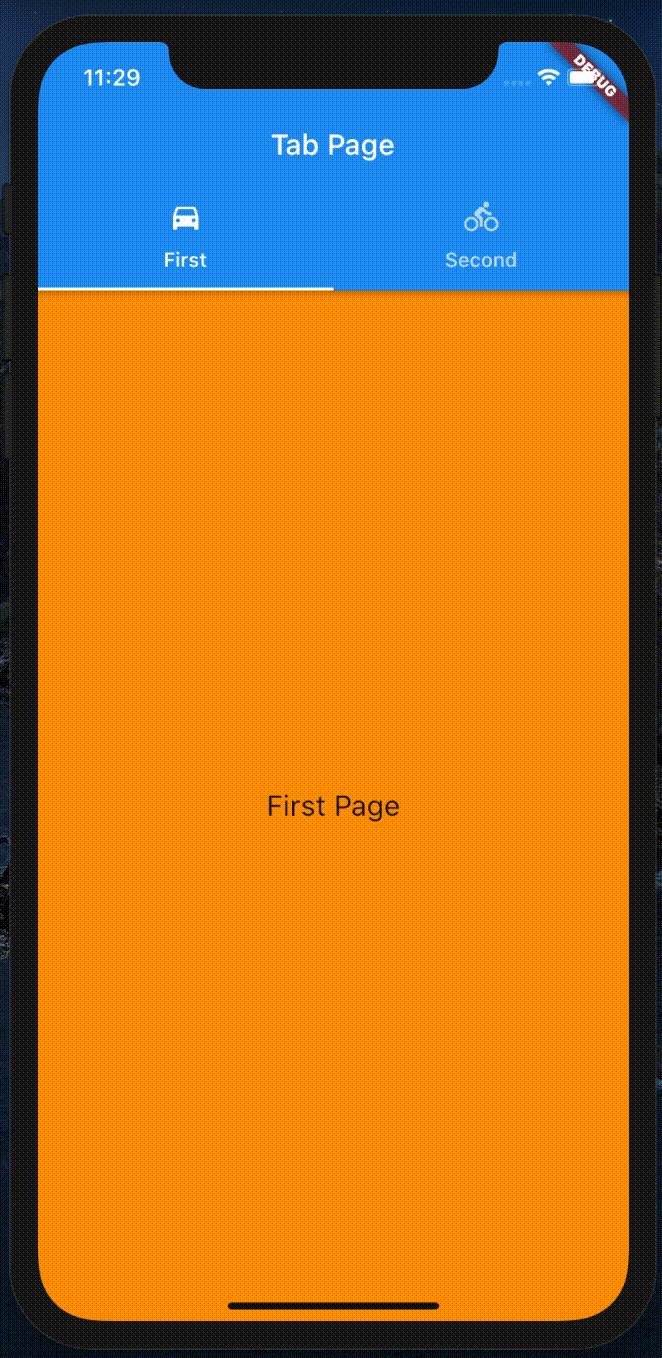
実装方法
タブの小画面を準備する
真ん中に文字があるだけの画面です。
これを2つ(FirstPage、SecondPage)作成します。
FirstPage.dart
import 'package:flutter/material.dart';
class FirstPage extends StatelessWidget {
@override
Widget build(BuildContext context) {
return Scaffold(
backgroundColor: Colors.orange,
body: Center(
child: Text(
"First Page",
style: TextStyle(
fontSize: 20
),
),
),
);
}
}
タブ画面を作成します。
TabPage.dart
import 'package:flutter/material.dart';
import 'package:flutter_app/FirstPage.dart';
import 'package:flutter_app/SecondPage.dart';
class TabPage extends StatelessWidget {
final _tab = <Tab> [
Tab( text:'First', icon: Icon(Icons.directions_car)),
Tab( text:'Second', icon: Icon(Icons.directions_bike)),
];
@override
Widget build(BuildContext context) {
return DefaultTabController(
length: _tab.length,
child: Scaffold(
appBar: AppBar(
title: Text("Tab Page"),
bottom: TabBar(
tabs: _tab,
),
),
body: TabBarView(
children: <Widget>[
FirstPage(),
SecondPage(),
],
),
),
);
}
}
ポイント
final _tab = <Tab> [
Tab( text:'First', icon: Icon(Icons.directions_car)),
Tab( text:'Second', icon: Icon(Icons.directions_bike)),
];
ここでタブの要素を定義しています。
@override
Widget build(BuildContext context) {
return DefaultTabController(
length: _tab.length,
child: Scaffold(
appBar: AppBar(
title: Text("Tab Page"),
bottom: TabBar(
tabs: _tab,
),
),
body: TabBarView(
children: <Widget>[
FirstPage(),
SecondPage(),
],
),
),
);
}
DefaultTabController
を初期化し、length
にタブ数を、child
にタブバーの要素と画面を設定しています。
Main.dartに設定
最後にMain.dart
に設定して完了です。
Main.dart
import 'package:flutter/material.dart';
import 'package:flutter_app/TabPage.dart';
void main() {
runApp(MyApp());
}
class MyApp extends StatelessWidget {
@override
Widget build(BuildContext context) {
return MaterialApp(
theme: ThemeData(
primarySwatch: Colors.blue,
visualDensity: VisualDensity.adaptivePlatformDensity,
),
home: TabPage(),
);
}
}
参考