TextBlock
<Window x:Class="WpfApp9.MainWindow"
xmlns="http://schemas.microsoft.com/winfx/2006/xaml/presentation"
xmlns:x="http://schemas.microsoft.com/winfx/2006/xaml"
xmlns:d="http://schemas.microsoft.com/expression/blend/2008"
xmlns:mc="http://schemas.openxmlformats.org/markup-compatibility/2006"
mc:Ignorable="d"
Title="MainWindow" Height="450" Width="800">
<Grid>
<TextBlock Text="HelloWorld"></TextBlock>
</Grid>
</Window>
上記の.xamlがWPFのView部分を表す。で囲われた部分がコンテンツの内容となる。
<TextBlock></TextBlock>
はテキストを表示する。
<TextBlock></TextBlock>
に値をいくつか設定することによって表示のさせかたを変えることができる。
<Window x:Class="WpfApp9.MainWindow"
xmlns="http://schemas.microsoft.com/winfx/2006/xaml/presentation"
xmlns:x="http://schemas.microsoft.com/winfx/2006/xaml"
xmlns:d="http://schemas.microsoft.com/expression/blend/2008"
xmlns:mc="http://schemas.openxmlformats.org/markup-compatibility/2006"
mc:Ignorable="d"
Title="MainWindow" Height="450" Width="800">
<Grid>
<TextBlock Text="HelloWorld" Width="100" Height="50" FontSize="20"></TextBlock>
</Grid>
</Window>
表示結果
Button
<Window x:Class="WpfApp9.MainWindow"
xmlns="http://schemas.microsoft.com/winfx/2006/xaml/presentation"
xmlns:x="http://schemas.microsoft.com/winfx/2006/xaml"
xmlns:d="http://schemas.microsoft.com/expression/blend/2008"
xmlns:mc="http://schemas.openxmlformats.org/markup-compatibility/2006"
mc:Ignorable="d"
Title="MainWindow" Height="450" Width="800">
<Grid>
<Button Width="50" Height="50" Content="Button"></Button>
</Grid>
</Window>
ボタンを表示させるには<Button></Button>
タグによって表示させることができる。
ComboBox
<Window x:Class="WpfApp9.MainWindow"
xmlns="http://schemas.microsoft.com/winfx/2006/xaml/presentation"
xmlns:x="http://schemas.microsoft.com/winfx/2006/xaml"
xmlns:d="http://schemas.microsoft.com/expression/blend/2008"
xmlns:mc="http://schemas.openxmlformats.org/markup-compatibility/2006"
mc:Ignorable="d"
Title="MainWindow" Height="450" Width="800">
<Grid>
<ComboBox Width="200" Height="30">
<ComboBoxItem Content="アメリカ"></ComboBoxItem>
<ComboBoxItem Content="日本"></ComboBoxItem>
<ComboBoxItem Content="中国"></ComboBoxItem>
</ComboBox>
</Grid>
</Window>
ComboBoxはHTMLでいうところの、Selectにあたる。
ただ、選択した時の文字がずれるのでどうしているのか...
StackPanel
<Window x:Class="WpfApp9.MainWindow"
xmlns="http://schemas.microsoft.com/winfx/2006/xaml/presentation"
xmlns:x="http://schemas.microsoft.com/winfx/2006/xaml"
xmlns:d="http://schemas.microsoft.com/expression/blend/2008"
xmlns:mc="http://schemas.openxmlformats.org/markup-compatibility/2006"
mc:Ignorable="d"
Title="MainWindow" Height="450" Width="800">
<Grid>
<StackPanel Orientation="Horizontal" Height="50" VerticalAlignment="Center" HorizontalAlignment="Center">
<Button Content="Button1"></Button>
<Button Content="Button2"></Button>
<Button Content="Button3"></Button>
<Button Content="Button4"></Button>
</StackPanel>
</Grid>
</Window>
StackPanelは複数のものを並べて配置することが多い。
Orientationで縦に並べるか、横に並べるかを決める。
Verticalが縦。Horizontalが横。
VerticalAlignment,HorizontalAlignmentの二つは横の位置、縦の位置を定める。
RadioButton
<Window x:Class="WpfApp9.MainWindow"
xmlns="http://schemas.microsoft.com/winfx/2006/xaml/presentation"
xmlns:x="http://schemas.microsoft.com/winfx/2006/xaml"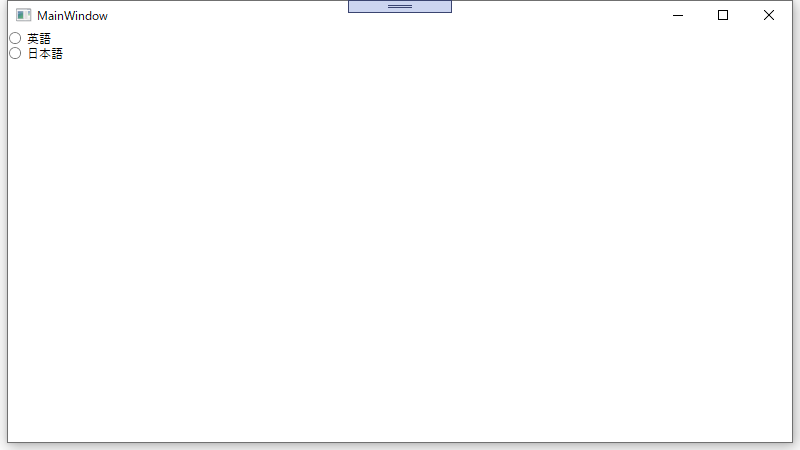
xmlns:d="http://schemas.microsoft.com/expression/blend/2008"
xmlns:mc="http://schemas.openxmlformats.org/markup-compatibility/2006"
mc:Ignorable="d"
Title="MainWindow" Height="450" Width="800">
<Grid>
<StackPanel>
<RadioButton Content="英語"></RadioButton>
<RadioButton Content="日本語"></RadioButton>
</StackPanel>
</Grid>
</Window>
RadioButtonはその名の通りラジオボタン。英語をチェックすれば日本語のチェックが外れるし、
日本語をチェックすれば英語のチェックが外れる。
<StackPanel></StackPanel>
がないと表示がだぶってしまい上手く表示されない。
TextBox
<Window x:Class="WpfApp9.MainWindow"
xmlns="http://schemas.microsoft.com/winfx/2006/xaml/presentation"
xmlns:x="http://schemas.microsoft.com/winfx/2006/xaml"
xmlns:d="http://schemas.microsoft.com/expression/blend/2008"
xmlns:mc="http://schemas.openxmlformats.org/markup-compatibility/2006"
mc:Ignorable="d"
Title="MainWindow" Height="450" Width="800">
<Grid>
<TextBox Height="20" Width="100"></TextBox>
</Grid>
</Window>
TextBoxはHTMLでいうところのinput type="text"にあたる。
DataGrid
画面側
<Window x:Class="WpfApp9.MainWindow"
xmlns="http://schemas.microsoft.com/winfx/2006/xaml/presentation"
xmlns:x="http://schemas.microsoft.com/winfx/2006/xaml"
xmlns:d="http://schemas.microsoft.com/expression/blend/2008"
xmlns:mc="http://schemas.openxmlformats.org/markup-compatibility/2006"
mc:Ignorable="d"
Title="MainWindow" Height="450" Width="800">
<Grid>
<DataGrid Width="200" HorizontalAlignment="Left" Name="Items"></DataGrid>
</Grid>
</Window>
コード側
using System.Collections.Generic;
using System.Windows;
using System.Windows.Controls;
namespace WpfApp9
{
/// <summary>
/// MainWindow.xaml の相互作用ロジック
/// </summary>
public partial class MainWindow : Window
{
public MainWindow()
{
InitializeComponent();
var list = new List<Fruit>()
{
new Fruit(){Name="apple", Number=3, Price=150},
new Fruit(){Name="banana", Number=5, Price=200},
new Fruit(){Name="cherry", Number=4, Price=180}
};
Items.ItemsSource = list;
}
}
public class Fruit
{
public string Name { get; set; }
public int Number { get; set; }
public int Price { get; set; }
}
}
実行結果
DataGridはいろいろと機能がある分単純な表組として使いたい場合はその使える機能をOFFにしていかないといけない。
コード側ではリストを用意してあげて、Xaml側でDataGridに名前をつけてコード側から要素を入れてあげている。
機能の数が色々とあるので、またいつか。
まとめ
Xaml | HTML |
---|---|
TextBlock | ただの文字列?? |
TextBox | <input type="text"/> |
Button | button |
ComboBox | select |
RadioButton | <input type="radio"/> |
CheckBox | checkbox |
DataGrid | table?? |