ライブラリをインポートする
import pandas as pd # データ分析に用いるライブラリ
import matplotlib.pyplot as plt # グラフ表示に用いるライブラリ
pd.set_option('display.unicode.east_asian_width', True) # 表示のずれを少し緩和
plt.rcParams['font.family'] = 'IPAexGothic' # グラフ表示におけるフォントの指定
この4つを毎回、冒頭に記入すること。
データを読み込む
data_path = "./titanic.csv"
df_data = pd.read_csv(data_path, encoding="utf-8-sig")
data_path
にファイルのパスを指定して、df_data
に読み込んだデータを格納する。
データを確認する
ランダムにいくつか表示させてみる
print(df_data.sample(10))

データ数を表示してみる
print(df_data.shape)

データの項目(横方向・列方向)を表示してみる
print(df_data.columns)
ある部分だけ表示してみる
print(df_data.loc[0:10, "年齢"])
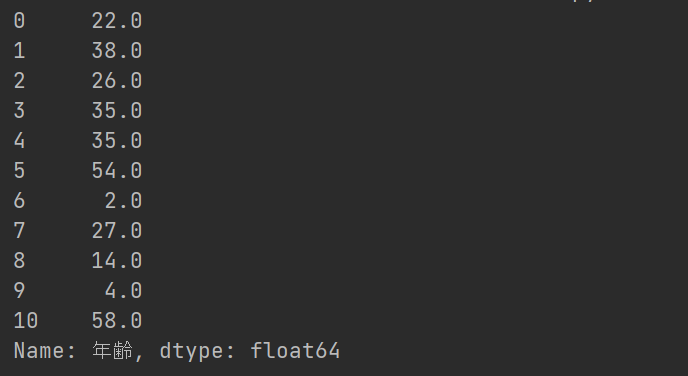
最初の10行の年齢
が表示される。
print(df_data.loc[:, "年齢"])

print(df_data.loc[:, ["性別", "年齢"]])

項目の値を全て抽出する
print(df_data.loc[:, "出港地"].unique())


条件に合ったデータを表示する場合
mask = df_data["生存状況"] == 1
print(df_data[mask])

指定する列の値で並べ替える場合
print(df_data.sort_values(by="旅客クラス", ascending=True))

特定の列のある値でまとめた平均値等を表示する場合
print(df_data.groupby("旅客クラス").mean())

特定の列の値の頻度を求める場合
print(df_data["旅客クラス"].value_counts(sort=False))

データの分析(組合せ編)
特定の列&値の頻度
print(df_data.loc[:, ["旅客クラス", "生存状況"]].value_counts(sort=False))

条件&並べ替え&特定の列
mask = df_data["生存状況"] == 1
print(df_data[mask].sort_values(by="旅客クラス", ascending=True).loc[:, ["旅客クラス", "運賃"]])
