TL;DR
- providerを使ってlistviewの構成および、そのlistviewへの動的な追加を実装する際にベースとなる実装を書きました
- なるべくいろんな呼び出し方を使っています
- 静的解析はしていないので適宜const等追加してください
- 非同期な処理の場合は実際は
async/await
を挟むだとか、addした後にテキストをクリアしたい場合はTextEditingController
を追加することになります - providerは便利
準備
pubspec.yaml
dependencies:
provider: any
ChangeNotifierを実装したクラス
chats.dart
import 'package:flutter/material.dart';
class ChatData with ChangeNotifier {
final _chats = <String>[];
List<String> get chats => _chats;
int get chatCount => _chats.length;
String getChat(int index) {
return _chats[index];
}
void addChat(String chat) {
_chats.add(chat);
notifyListeners();
}
}
main.dart
main.dart
void main() => runApp(ChangeNotifierProvider<ChatData>(
create: (context) => ChatData(),
child: App(),
));
class App extends StatelessWidget {
@override
Widget build(BuildContext context) {
return MaterialApp(
title: 'provider sample',
home: ChatScreen(),
);
}
}
チャット画面
screen.dart
class ChatScreen extends StatelessWidget {
const ChatScreen({Key key}) : super(key: key);
@override
Widget build(BuildContext context) {
return Scaffold(
body: SafeArea(
child: Column(
children: <Widget>[
Expanded(
child: ChatList(),
),
Divider(height: 2, color: Colors.black),
ChatInput(),
],
),
));
}
}
class ChatList extends StatelessWidget {
const ChatList({Key key}) : super(key: key);
@override
Widget build(BuildContext context) {
return ListView.separated(
shrinkWrap: true,
separatorBuilder: (context, index) => Divider(color: Colors.black),
itemCount: Provider.of<ChatData>(context).chatCount,
itemBuilder: (BuildContext context, int index) {
return ChatListTile(index: index);
},
);
}
}
class ChatListTile extends StatelessWidget {
const ChatListTile({Key key, @required this.index}) : super(key: key);
final int index;
@override
Widget build(BuildContext context) {
return Consumer<ChatData>(
builder: (context, chatData, child) {
final currentChat = chatData.getChat(index);
return ListTile(
title: Text(currentChat),
);
},
);
}
}
class ChatInput extends StatelessWidget {
const ChatInput({Key key}) : super(key: key);
@override
Widget build(BuildContext context) {
return SizedBox(
height: 40,
child: TextField(
onSubmitted: Provider.of<ChatData>(context, listen: false).addChat,
),
);
}
}
完成
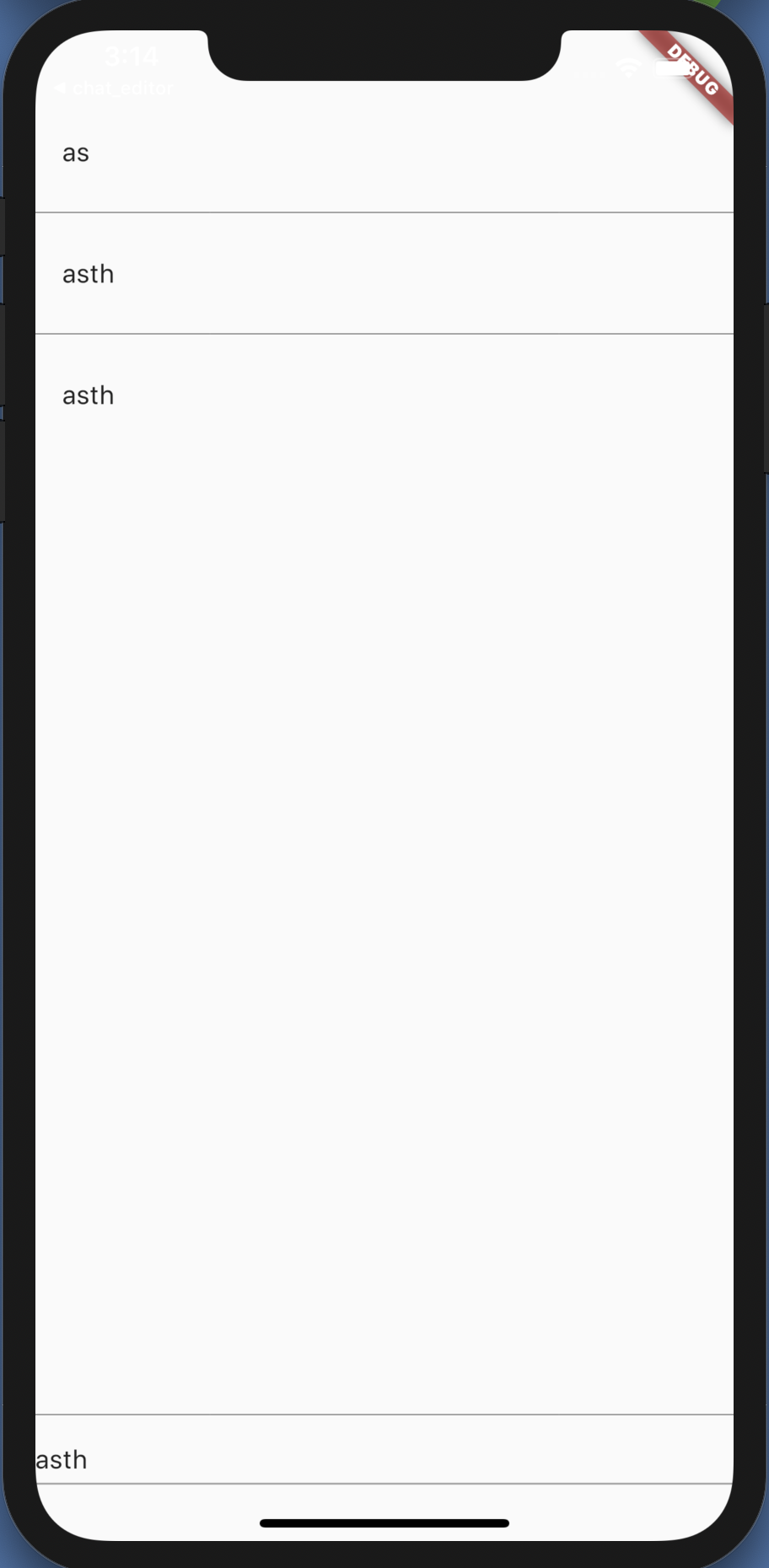