はじめに
Androidのアプリ開発でAPIとスタブを通信するのに、今まではリクエストURLなどを切り替えて対応しておりました。
今回、OkHttpのInterceptor機能を使うと簡単に向き先を切り替えることが出来る事を知ったので記事にまとめました。
Interceptorを使うと、アプリケーションとOkHttpの間に、任意の処理を割り込むことが出来る機能です。
https://github.com/square/okhttp/wiki/Interceptors
スタブ仕様
今回はAssetsからレスポンスを取得したいので、
APIと同じレスポンスフォーマットのJSONファイルを用意する。
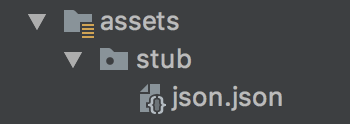
実装
1. スタブ用のInterceptorを実装する
class StubInterceptor(private val context: Context) : Interceptor {
companion object {
private val RESPONSE_TYPE = MediaType.parse("application/json; charset=utf-8")
}
override fun intercept(chain: Interceptor.Chain): Response {
val request = chain.request()
val response = chain.proceed(request)
var code = 200
val body = try {
loadFromAssets(request)
} catch (e: FileNotFoundException) {
// File not found
code = 404
e.toString()
}
val responseBody = ResponseBody.create(RESPONSE_TYPE, body)
return response.newBuilder()
.code(code)
.body(responseBody)
.build()
}
private fun loadFromAssets(request: Request): String {
val url = request.url().url().path.substring(1)
return context.assets.open("mock/$url.json").use {
it.reader().readText()
}
}
}
2.クライアントにStubInterceptorを追加する
val client = OkHttpClient().newBuilder().apply {
addInterceptor(StubInterceptor(context))
}
3.呼び出し元
val requestUrl = "https://xxxxxxxx/api/token" // <- "token.json" をAssetsに配置する
val request = Request.Builder()
.url(requestUrl)
.get()
.build()
val response = client.newCall(request).execute()
Retrofitと連携する場合
private val retrofit = Retrofit.Builder()
.baseUrl("https://xxxxxxxx/")
.client(client) // OkHttpクライアントを設定する
.addConverterFactory(GsonConverterFactory.create())
.addCallAdapterFactory(RxJava2CallAdapterFactory.create())
.build()
以上、意外と簡単に実装できる。