こんにちは。僕は未来電子でインターンさせてもらっているものです。
今回は前回の続きなので、登録完了したことを表示させる段階ですね。
#!/usr/bin/env python3
# -*- coding: utf-8 -*-
import sqlite3
import csv
import sys
import io
import cgi
まずこれらをインポートします。
# windowsにおける文字化け回避
sys.stdout = io.TextIOWrapper(sys.stdout.buffer, encoding="utf-8")
# 使用するデータベースを指定
db_path = "sample.db"
# sample.dbを使ってsqlite3を起動
con = sqlite3.connect("sample.db")
# 属性名で値を取り出すように設定
con.row_factory = sqlite3.Row
# カーソル(sqlでデータベースを操作するやつ)を作成
cur = con.cursor()
次にこのような流れでデータベース部分を扱います。
htmlText = ""
そして続けてこのように書きます。これによってhtmlTextをHTML部分に挿入することができます。
以下sqlの実行
try:
cur.execute(
"create table sampleTable(id integer primary key autoincrement, name text);"
)
except sqlite3.Error as e:
print("ERROR:", e.args[0])
try:
cur.execute("insert into sampleTable(name)values('test');")
except sqlite3.Error as e:
print("ERROR:", e.args[0])
try:
cur.execute("select * from sampleTable;")
except sqlite3.Error as e:
print("ERROR:", e.args[0])
rows = cur.fetchall()
新しいテーブルであるsampleTableのカラムをidとnameにしています。
insert文でnameに'test'を入れます。
エラーがあった時はERRORと表示できるようにしています。
# データベースから値を取り出す
if not rows:
htmlText = "No Data"
else:
for row in rows:
if str(row["id"]):
htmlText += str(row[0]) + "|" + str(row[1]) + "</br>"
次にデータベースから値を取り出すのですが、リストにあるidとtestを取り出すためにこのようにインデックスを使って取り出します。また、htmlTextにこのリストを入れて、実際にHTML部分に表示できるようにします。
# 以下のコードを書かないと、htmlとして読み込んでもらえない。
print("Content-type: text/html; charset=utf-8")
# htmlの部分。printでHTMLコードを表示させることで、ブラウザがHTMLコードとして認識してくれる。
print(
(
"""
<html>
<head>
<meta http-equiv=\"Content-Type\" content=\ "text/html
charset=utf-8\" / >
<meta charset="utf-8">
<title>入力完了</title>
</head>
<body>
<h1>入力完了</h1>
<h5>登録が完了しました。</h5>
<br/>
<p>ありがとうございました!</p>
<a href="index.py">学生一覧へ</a>
%s
</body>
</html>
"""
) % htmlText )
%sの部分にhtmlTextを入れます。最後に% htmlTextを入れます。
すると画面ではどうなるかというと、
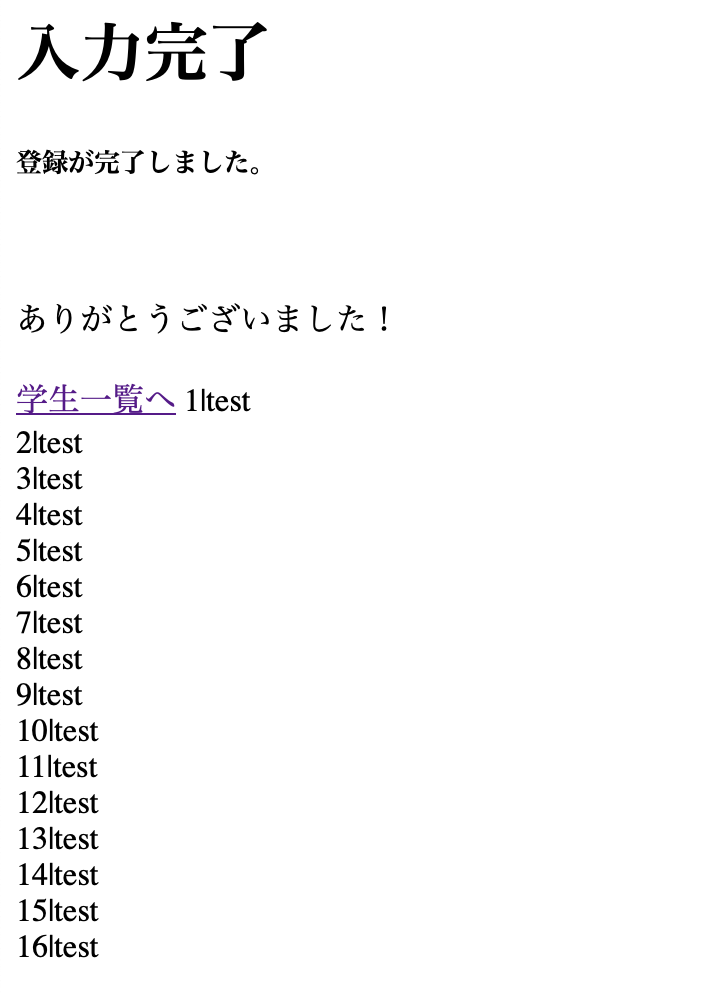
このように試行回数と試行内容であるテストがしっかり表示されました。
今回は登録完了したことを表示させて、テストの回数と内容も表示させました。
全体のコードはこんな感じです。
#!/usr/bin/env python3
# -*- coding: utf-8 -*-
import sqlite3
import csv
import sys
import io
import cgi
# windowsにおける文字化け回避
sys.stdout = io.TextIOWrapper(sys.stdout.buffer, encoding="utf-8")
# 使用するデータベースを指定
db_path = "sample.db"
# sample.dbを使ってsqlite3を起動
con = sqlite3.connect("sample.db")
# 属性名で値を取り出すように設定
con.row_factory = sqlite3.Row
# カーソル(sqlでデータベースを操作するやつ)を作成
cur = con.cursor()
htmlText = ""
# 以下sqlの実行
try:
cur.execute(
"create table sampleTable(id integer primary key autoincrement, name text);"
)
except sqlite3.Error as e:
print("ERROR:", e.args[0])
try:
cur.execute("insert into sampleTable(name)values('test');")
except sqlite3.Error as e:
print("ERROR:", e.args[0])
try:
cur.execute("select * from sampleTable;")
except sqlite3.Error as e:
print("ERROR:", e.args[0])
rows = cur.fetchall()
# データベースから値を取り出す
if not rows:
htmlText = "No Data"
else:
for row in rows:
if str(row["id"]):
htmlText += str(row[0]) + "|" + str(row[1]) + "</br>"
# 以下のコードを書かないと、htmlとして読み込んでもらえない。
print("Content-type: text/html; charset=utf-8")
# htmlの部分。printでHTMLコードを表示させることで、ブラウザがHTMLコードとして認識してくれる。
print(
(
"""
<html>
<head>
<meta http-equiv=\"Content-Type\" content=\ "text/html
charset=utf-8\" / >
<meta charset="utf-8">
<title>入力完了</title>
</head>
<body>
<h1>入力完了</h1>
<h5>登録が完了しました。</h5>
<br/>
<p>ありがとうございました!</p>
<a href="index.py">学生一覧へ</a>
%s
</body>
</html>
"""
) % htmlText )
次回は、index.pyで今までのデータをすべて一覧で表示させる方法についてみていきます。
ありがとうございました。