Vue.jsのフレームワークの中でd3.jsを使う場合どうすればいいのか困ったので、メモとして投稿します。
Vue.jsを触ったことのある人、かつd3.jsを触ったことある人を対象にしています。
※ Vue.js v2.5.2 / d3.js Version 4.11.0にて確認
コード全文
<head>
<script src="https://unpkg.com/vue"></script>
<script src="https://d3js.org/d3.v4.min.js"></script>
</head>
<body>
<div id="vue">
<svg id="circle-001" :width="width" :height="height"></svg>
<div>r: {{ r }}</div>
</div>
<script>
new Vue({
el: '#vue',
data: {
width: 500,
height: 500,
r: 75
},
mounted: function(){
var svg = d3.select('#circle-001');
this.circle = svg.append('circle')
.attr('cx', this.width/2)
.attr('cy', this.height/2)
.attr('r', this.r)
.style('fill','rgba(0, 0, 0, 0.8)')
}
})
</script>
</body>
結果
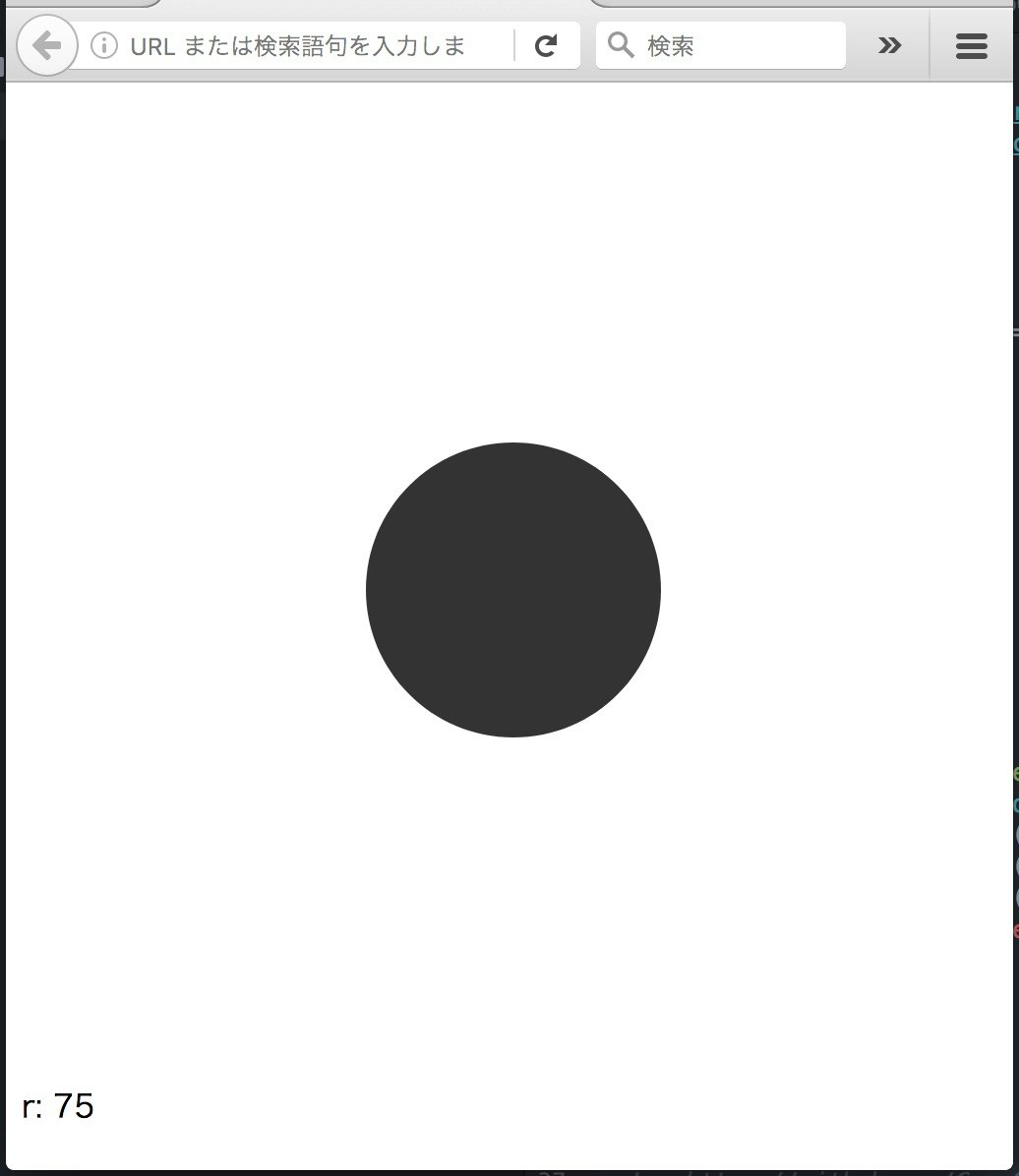
コードの解説
①まずはライブラリを読み込みます
<head>
<script src="https://unpkg.com/vue"></script>
<script src="https://d3js.org/d3.v4.min.js"></script>
</head>
ここは説明不要ですね。
②svgの表示エリアを作成します
<div id="vue">
<svg id="circle-001" :width="width" :height="height"></svg>
<div>r: {{ r }}</div>
</div>
Vueの支配下に置きたいdiv(#vue)を用意し、その下に円を表示するためのsvgエリアを記述しておきます。
ついでに円の半径px(r)を表示するエリアを作っています。
また、svgエリアはあとでd3からselectできるように「#circle-001」というidを振っています。
svgのwidthとheightは、この後出てくるVueインスタンスのdataである「width」および「height」とバインドします。
③Vueインスタンスを作成します
<script>
new Vue({
el: '#vue',
data: {
width: 500,
height: 500,
r: 75
},
mounted: function(){
var svg = d3.select('#circle-001');
this.circle = svg.append('circle')
.attr('cx', this.width/2)
.attr('cy', this.height/2)
.attr('r', this.r)
.style('fill','rgba(0, 0, 0, 0.8)')
}
})
</script>
まずは「el: '#vue'」で「#vue」をVueの支配下に置くことを宣言します。
dataとしてはsvgの横幅、高さ、およびcircleの半径を持つこととします。
さて、「#vue」を完全にVueの支配下に置いたところからd3.jsの出番となります。
「mounted:」で指定した関数は、Vueがelを完全に支配下に置いたタイミングで実行されます。
(参考→ https://jp.vuejs.org/v2/guide/instance.html )
「mounted」の中に書き慣れたd3.jsのコードを書きましょう。
「mounted」が実行されるタイミングではすでに(「#vue」の子供の)「svg#circle-001」がVueの支配下に置かれていますので、mountedの中の関数から「#circle-001」を呼び出すことができます。
d3.selectで「#circle-001」を選択し、そこにcircleをappendすれば、ブラウザに円が表示されます。