はじめに
web3.jsを使ってEthereumの送金処理を作成してみました。
フレームワークには、Nuxt.jsを使いました。
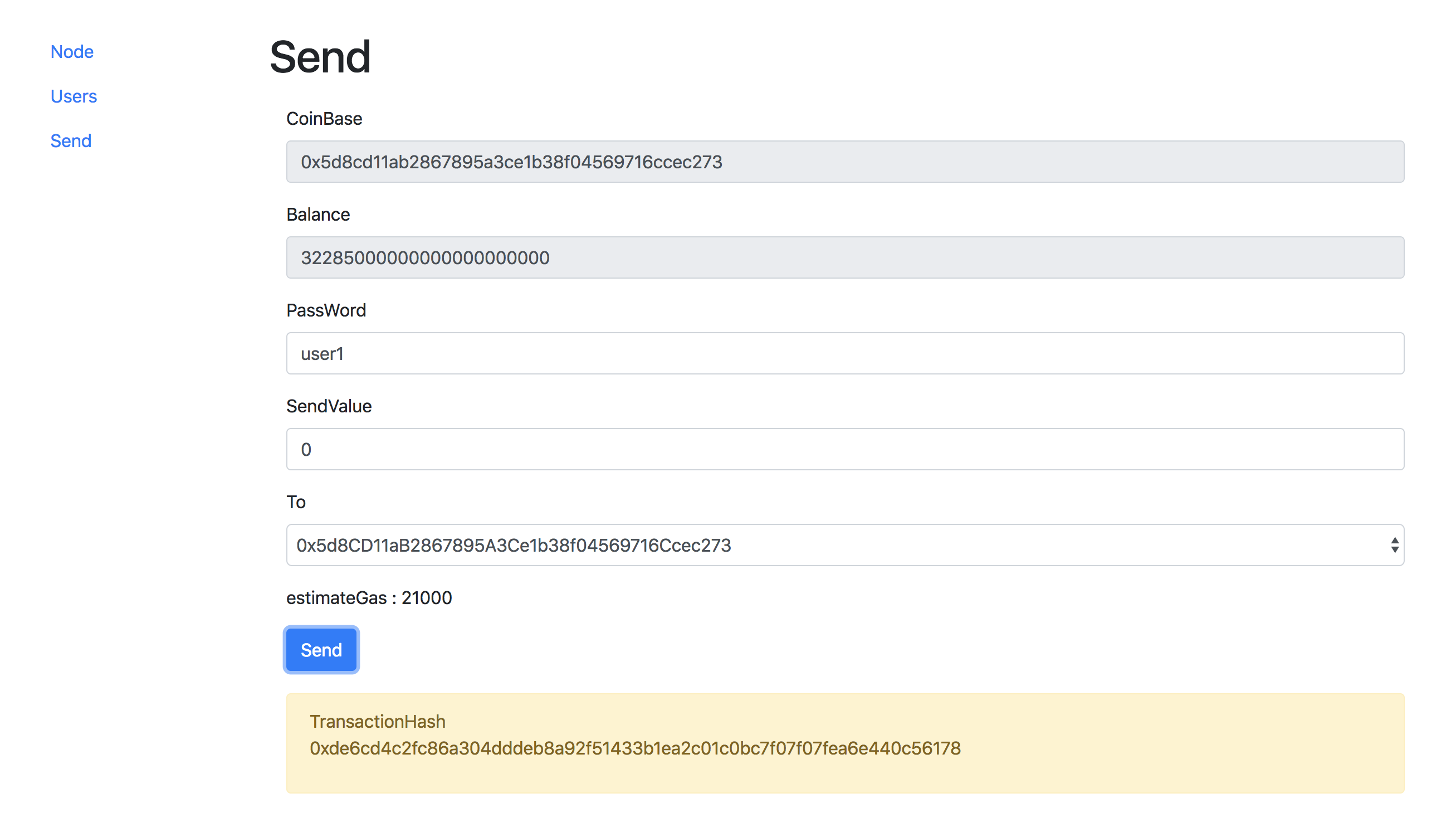
ひとまず、実装したのは以下の基本的な機能のみ。
web3.jsからプライベートネットのAPIを叩いていきます。
- nodeの状態確認
- addressの新規作成
- addressの一覧(及びbalance)
- 送金機能
事前準備 PrivateNet
前提として、EthereumのPrivateNetを起動する必要があります。
以下のようなコマンドになりますが、この部分の詳しい説明はここでは省きます。
PrivateNetを起動する事によりweb3.js
から利用できるAPIが用意されます。
geth --networkid "10" \
--nodiscover \
--datadir `pwd` \
--rpc \
--rpcaddr "localhost" \
--rpcport "8545" \
--rpccorsdomain "*" \
--rpcapi "eth,net,web3,personal,accounts" \
console 2>> `pwd`/geth_err.log
--rpcapi "eth,net,web3,personal,accounts"
rpcapi
のオプションで、web3
を許可しておく必要があります。
rpcapi
はAPIで受け付けるHTTP-RPCインターフェースの種類の指定になります。
アカウント新規作成
> personal.newAccount("password")
coinbaseの設定
> miner.setEtherbase(eth.accounts[0])
マイニングを開始
> miner.start()
マイニングを開始する事で、送金時のトランザクションが処理されます。
web3.js (Ethereum JavaScript API)
Generic JSON RPC仕様を実装したEthereum互換のJavaScript APIとの事。
https://github.com/ethereum/web3.js
npm install web3
web3.jsを経由してNuxt内部でEthereumのAPIを利用していきます。
CoinBase
balanceを取得する簡単な例。
import Web3 from 'web3';
const web3 = new Web3(new Web3.providers.HttpProvider("http://localhost:8545"));
const address = "0x5d8cd11ab2867895a3ce1b38f04569716ccec273"
web3.eth.getBalance(address).then( balance => {
console.log(balance)
});
=> 27638999999998999999700
JSON-RPC
web3.jsのAPIは、JSON-RPCから取得する方法もあります。
以下、初歩的な呼び出し方法。
curl -H "Content-type: application/json" -H "Accept: application/json" -X POST http://localhost:8545 --data '{"jsonrpc":"2.0","method":"web3_clientVersion","params":[],"id":1}'
=> {"jsonrpc":"2.0","id":1,"result":"Geth/v1.8.3-stable/darwin-amd64/go1.10.1"}
Node情報の取得
web3.jsから情報を取得して、Vue.jsのdataに格納しています。
this.Host = web3.currentProvider.host;
web3.eth.isMining().then(val=>this.IsMining=val);
web3.eth.getHashrate().then(val=>this.HashRate=val);
web3.eth.getGasPrice().then(val=>this.GasPrice=val);
web3.eth.getBlockNumber().then(val=>this.BlockNumber=val);
web3.eth.net.getId().then(val=>this.NetId=val);
web3.eth.net.getPeerCount().then(val=>this.PeerCount=val);
Addressの新規作成
web3.eth.personal.newAccount(this.Password)
Addressの一覧の取得
web3.eth.getAccounts().then(val=>this.Accounts=val);
送金処理
web3.eth.personal.unlockAccount(this.CoinBase, this.PassWord).then(()=> {
web3.eth.sendTransaction({
from: this.CoinBase,
to: this.To,
value: this.SendValue
}, (error, txHash) => {
console.log("Transaction Hash:", txHash, error);
this.TransactionHash = txHash;
this.SendValue = 0;
this.To = this.Accounts[0];
if (error) {
alert("Ether Transfer Failed");
}
});
Command
web3.jsを利用せずに直接ethを送金するコマンド
> eth.sendTransaction({from: eth.accounts[1], to: eth.accounts[0], value: web3.toWei(3, "ether")})
バランスの確認
> eth.getBalance(eth.accounts[0])
ブロックナンバーの確認
> eth.blockNumber
ハッシュレートの確認
> eth.hashrate
coinBase指定
> miner.setEtherbase(eth.accounts[1])
現在のcoinBase確認
eth.coinbase
=>"0x4338f1861bca03160caa6986fe6b816411c74807"
GitHub
今回作成したサンプルはこちらから
https://github.com/tkc/nuxt-ethereum-wallet