Gtk3のTreeViewの表示
gtk-sharpチュートリアルに書かれているサンプルをコピペするだけでTreeViewを表示できます
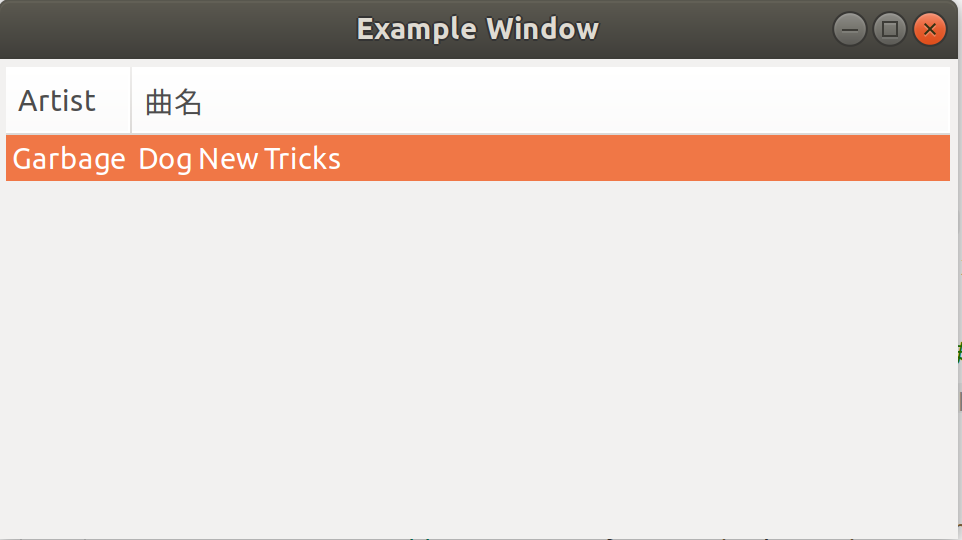
Gladeの編集
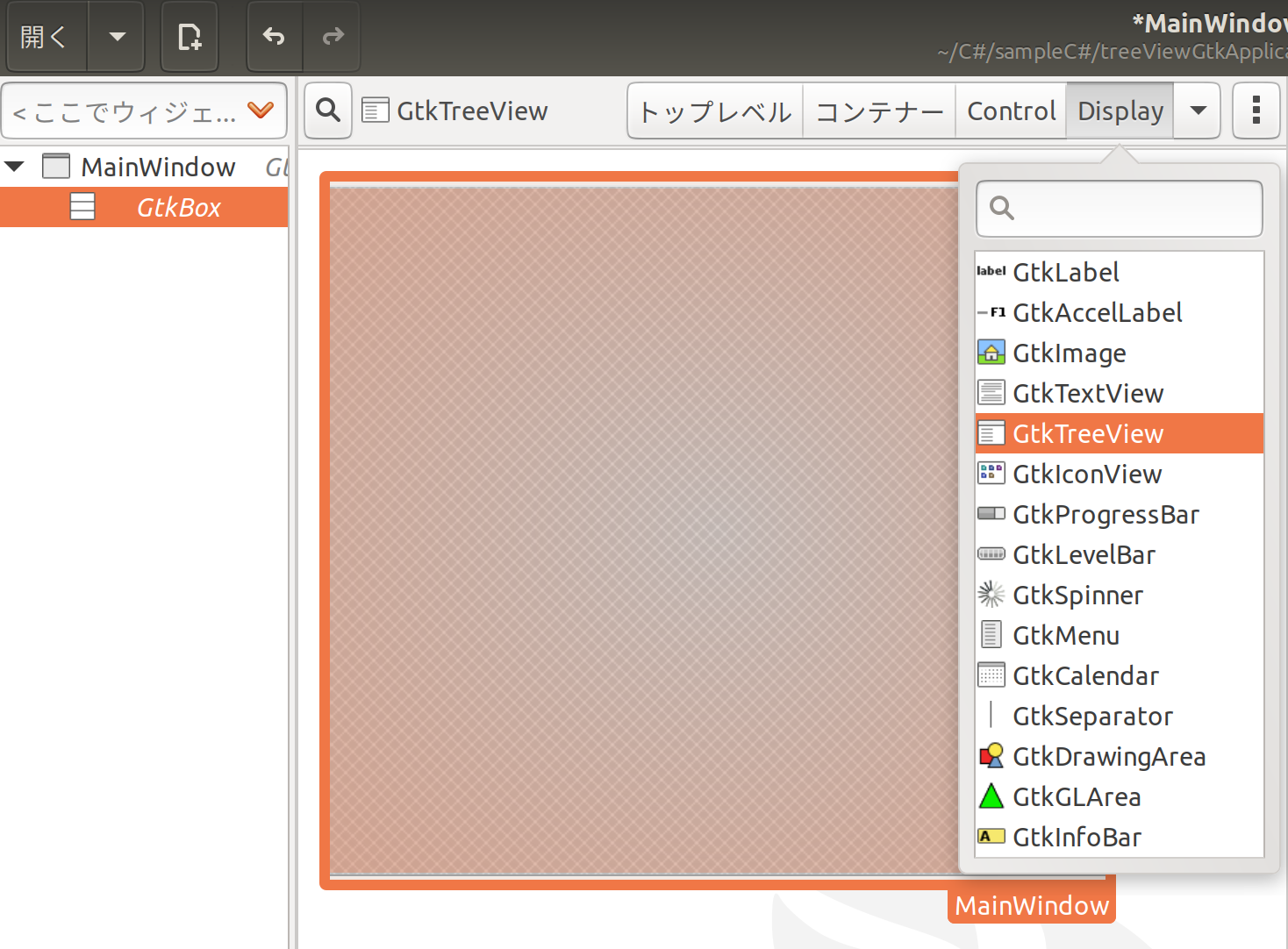
TreeViewにIdのところに名前をつけます
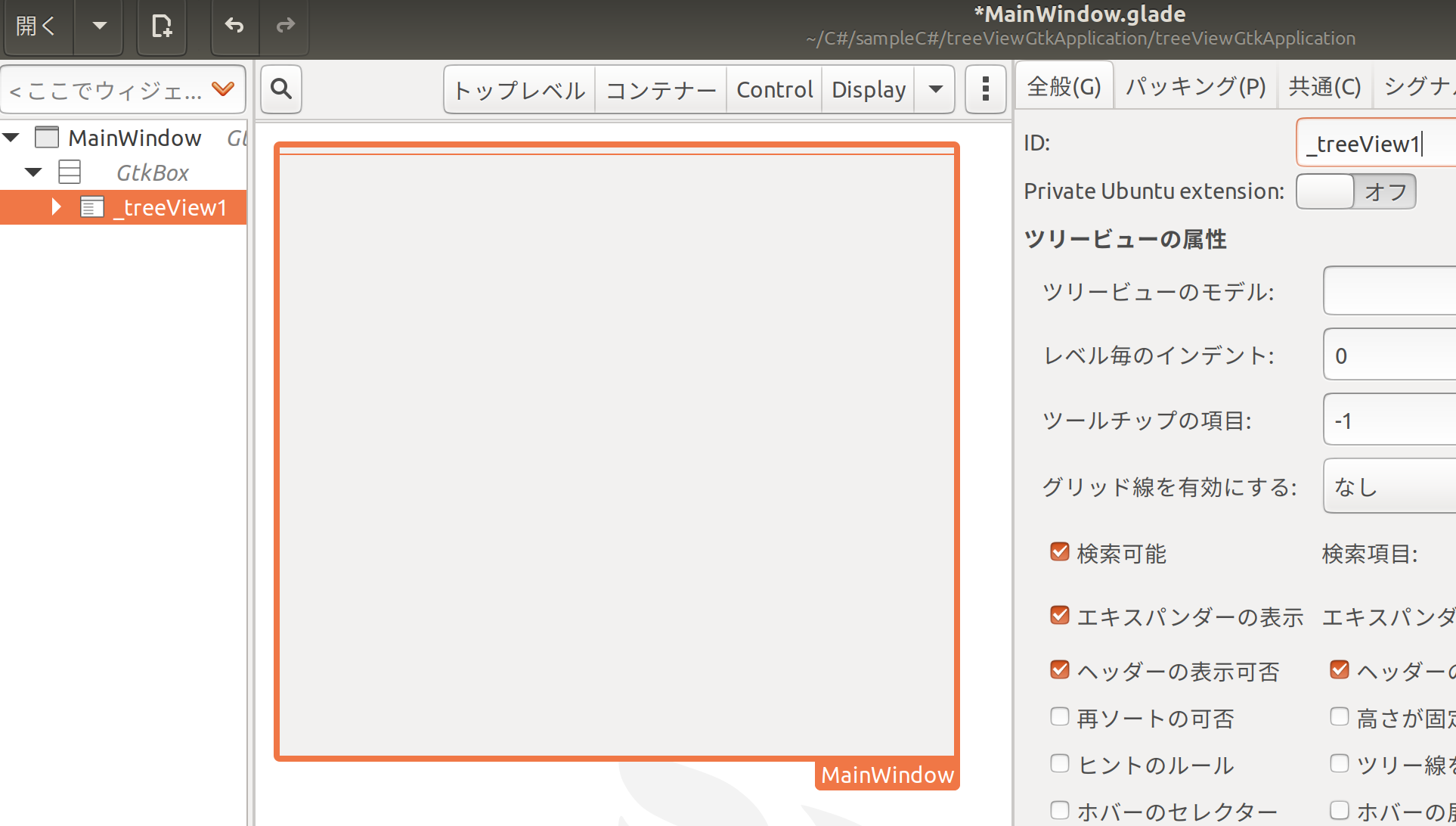

using System;
using Gtk;
using UI = Gtk.Builder.ObjectAttribute;
namespace treeViewGtkApplication
{
class MainWindow : Window
{
[UI] private TreeView _treeView1 = null;
public MainWindow() : this(new Builder("MainWindow.glade"))
{
}
private MainWindow(Builder builder) : base(builder.GetRawOwnedObject("MainWindow"))
{
builder.Autoconnect(this);
_mkTreeView();
}
void _mkTreeView()
{
Gtk.TreeViewColumn artistColumn = new Gtk.TreeViewColumn ();
artistColumn.Title = "Artist";
// アーティスト名を表示するテキストセルを作成する
Gtk.CellRendererText artistNameCell = new Gtk.CellRendererText ();
// セルを列に追加する
artistColumn.PackStart (artistNameCell, true);
// 曲名の列を作る
Gtk.TreeViewColumn songColumn = new Gtk.TreeViewColumn ();
songColumn.Title = "曲名";
// 曲名の列にも同じことをする
Gtk.CellRendererText songTitleCell = new Gtk.CellRendererText ();
songColumn.PackStart (songTitleCell, true);
// TreeViewにカラムを追加する
_treeView1.AppendColumn (artistColumn);
_treeView1.AppendColumn (songColumn);
// モデル内のどのアイテムを表示するかをセルレンダラーに伝える
artistColumn.AddAttribute (artistNameCell, "text", 0);
songColumn.AddAttribute (songTitleCell, "text", 1);
// アーティスト名と曲名の2つの文字列を保持するモデルを作成します。
Gtk.ListStore musicListStore = new Gtk.ListStore (typeof (string), typeof (string));
// ストアにデータを追加します。
musicListStore.AppendValues ("Garbage", "Dog New Tricks");
// TreeViewにモデルを割り当てる
_treeView1.Model = musicListStore;
}
}
}
チュートリアルのサンプルの書き換える必要があります。
Mono
Gtk.TreeModel
↓
GTK3
Gtk.ITreeModel
Modelを使った表示の仕方

チュートリアルに書かれている内容をコピペで表示できました。
using System;
using System.Collections.Generic;
using Gtk;
using UI = Gtk.Builder.ObjectAttribute;
namespace treeView2Application
{
class MainWindow : Window
{
[UI] private TreeView _treeView1 = null;
List<Song> songs;
public MainWindow() : this(new Builder("MainWindow.glade"))
{
}
private MainWindow(Builder builder) : base(builder.GetRawOwnedObject("MainWindow"))
{
builder.Autoconnect(this);
_mkTreeView();
}
void _mkTreeView()
{
songs = new List<Song>();
songs.Add (new Song ("Dancing DJs vs. Roxette", "Fading Like a Flower"));
songs.Add (new Song ("Xaiver", "Give me the night"));
songs.Add (new Song ("Daft Punk", "Technologic"));
Gtk.TreeViewColumn artistColumn = new Gtk.TreeViewColumn ();
artistColumn.Title = "Artist";
Gtk.CellRendererText artistNameCell = new Gtk.CellRendererText ();
artistColumn.PackStart (artistNameCell, true);
Gtk.TreeViewColumn songColumn = new Gtk.TreeViewColumn ();
songColumn.Title = "Song Title";
Gtk.CellRendererText songTitleCell = new Gtk.CellRendererText ();
songColumn.PackStart (songTitleCell, true);
_treeView1.AppendColumn (artistColumn);
_treeView1.AppendColumn (songColumn);
Gtk.ListStore musicListStore = new Gtk.ListStore (typeof (Song));
foreach (Song song in songs) {
musicListStore.AppendValues (song);
}
artistColumn.SetCellDataFunc (artistNameCell, new Gtk.TreeCellDataFunc (RenderArtistName));
songColumn.SetCellDataFunc (songTitleCell, new Gtk.TreeCellDataFunc (RenderSongTitle));
_treeView1.Model = musicListStore;
}
private void RenderArtistName (Gtk.TreeViewColumn column, Gtk.CellRenderer cell, Gtk.ITreeModel model, Gtk.TreeIter iter)
{
Song song = (Song) model.GetValue (iter, 0);
if (song.Artist.StartsWith ("X") == true) {
(cell as Gtk.CellRendererText).Foreground = "red";
} else {
(cell as Gtk.CellRendererText).Foreground = "darkgreen";
}
(cell as Gtk.CellRendererText).Text = song.Artist;
}
private void RenderSongTitle (Gtk.TreeViewColumn column, Gtk.CellRenderer cell, Gtk.ITreeModel model, Gtk.TreeIter iter)
{
Song song = (Song) model.GetValue (iter, 0);
(cell as Gtk.CellRendererText).Text = song.Title;
}
}
public class Song
{
public Song (string artist, string title)
{
this.Artist = artist;
this.Title = title;
}
public string Artist;
public string Title;
}
}
課題
レンダー用の関数を毎回書かないといけなく面倒
SetCellDataFunc関数を毎回書かないようにしたい。
追記