SwiftUIの3つのView Layout
SwiftUIには3つのレイアウトを調整するStackView的なLayoutが存在する
- HStack (X軸を基準として横並びにViewを並べるレイアウト)
- VStack (Y軸を基準として縦並びにViewを並べるレイアウト)
- ZStack (Z軸を基準として重ねてViewを載せるレイアウト)
発想がAndroidのUIに似ています。
HStackはLinearLayout(Horizontal)のそれに似ていますし、
VStackはLinearLayout(Vertical)に似ています。
ということはSwiftUIはコンポーネントの配置の方法はpaddingやmarginを設定して調整するものだろうと推測できます。
HStack (X軸を基準として横並びにViewを並べるレイアウト)
ContentView.swift
struct ContentView : View {
var body: some View {
HStack {
ParentView()
SubView()
}
.frame(width: 400, height: 200, alignment: .center)
.background(Color.yellow)
}
}
struct ParentView: View {
var body: some View {
Text("ParentView")
.color(Color.white)
.frame(width: 200, height: 200, alignment: .center)
.background(Color.red)
}
}
struct SubView: View {
var body: some View {
Text("SubView")
.color(Color.orange)
.frame(width: 200, height: 200, alignment: .center)
.background(Color.blue)
}
}
Viewの見え方

VStack (Y軸を基準として縦並びにViewを並べるレイアウト)
ContentView.swift
struct ContentView : View {
var body: some View {
VStack {
ParentView()
SubView()
}
.frame(width: 400, height: 200, alignment: .center)
.background(Color.yellow)
}
}
struct ParentView: View {
var body: some View {
Text("ParentView")
.color(Color.white)
.frame(width: 200, height: 200, alignment: .center)
.background(Color.red)
}
}
struct SubView: View {
var body: some View {
Text("SubView")
.color(Color.orange)
.frame(width: 200, height: 200, alignment: .center)
.background(Color.blue)
}
}
Viewの見え方

ZStack (Z軸を基準として重ねてViewを載せるレイアウト)
ContentView.swift
struct ContentView : View {
var body: some View {
ZStack {
ParentView()
SubView()
}
.frame(width: 300, height: 300, alignment: .center)
.background(Color.yellow)
}
}
struct ParentView: View {
var body: some View {
Text("ParentView")
.color(Color.white)
.frame(width: 200, height: 200, alignment: .center)
.background(Color.red)
}
}
struct SubView: View {
var body: some View {
Text("SubView")
.color(Color.orange)
.frame(width: 100, height: 100, alignment: .center)
.background(Color.blue)
}
}
Viewの見え方
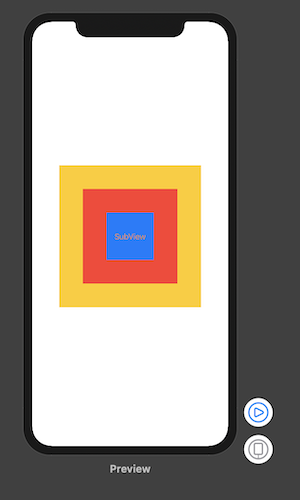
参考ドキュメント