Swift で円弧を描きたい時、UIBezierPath を使います。
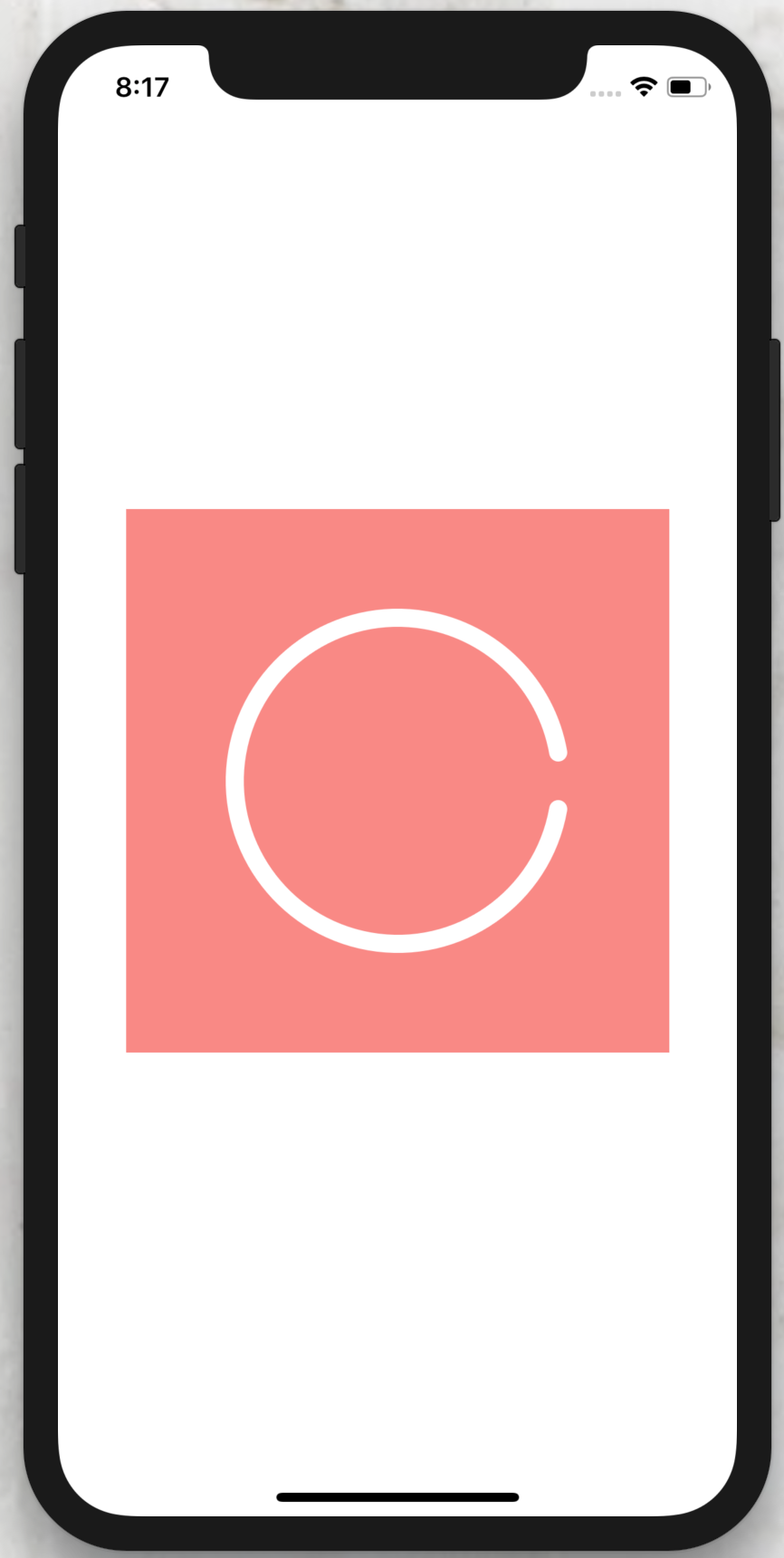
1. UIViewクラスを作る
PathDraw.swift
import UIKit
class PathDraw: UIView {
override func draw(_ rect: CGRect) {
// 円弧
let arc = UIBezierPath(arcCenter: CGPoint(x: 150, y: 150), // 円弧の中心
radius: 90, // 半径
startAngle: CGFloat(Double.pi) * 2 * 10.0 / 360.0, // 開始角度
endAngle: CGFloat(Double.pi) * 2 * 350.0 / 360.0, // 終了角度
clockwise: true) // trueだと時計回り, falseだと反時計回り
// 色の指定
let color = UIColor.white
color.setStroke()
// 線幅の指定
arc.lineWidth = 10
// パスの角を丸くする
arc.lineCapStyle = .round
// 描画する
arc.stroke()
}
}
startAngle と endAngle の求め方
3時の方向が0度になる。
直径×円周率×描画したい角度/360度で求めることができる。
サンプルコードでは、10°のからスタートして、350°まで描画している。
2. ViewController から、1.で作成したPathDrawを呼び出す
ViewController.swift
import UIKit
class ViewController: UIViewController {
// 背景のviewをstoryBoardで生成。
@IBOutlet weak var arcBackgroundView: UIView!
// PathDraw のインスタンスを生成する。
var pathDrawView = PathDraw()
override func viewDidLoad() {
super.viewDidLoad()
// 描画したいサイズを指定します。
pathDrawView = PathDraw(frame: CGRect(x: 0, y: 0, width: 300, height: 300))
// 背景を透明にする。デフォルトは黒色。
pathDrawView.isOpaque = false
// arcBackgroundView に追加
arcBackgroundView.addSubview(pathDrawView)
}
}