ジョイスティックとは?
レバーによって方向入力が行える装置のことですが、
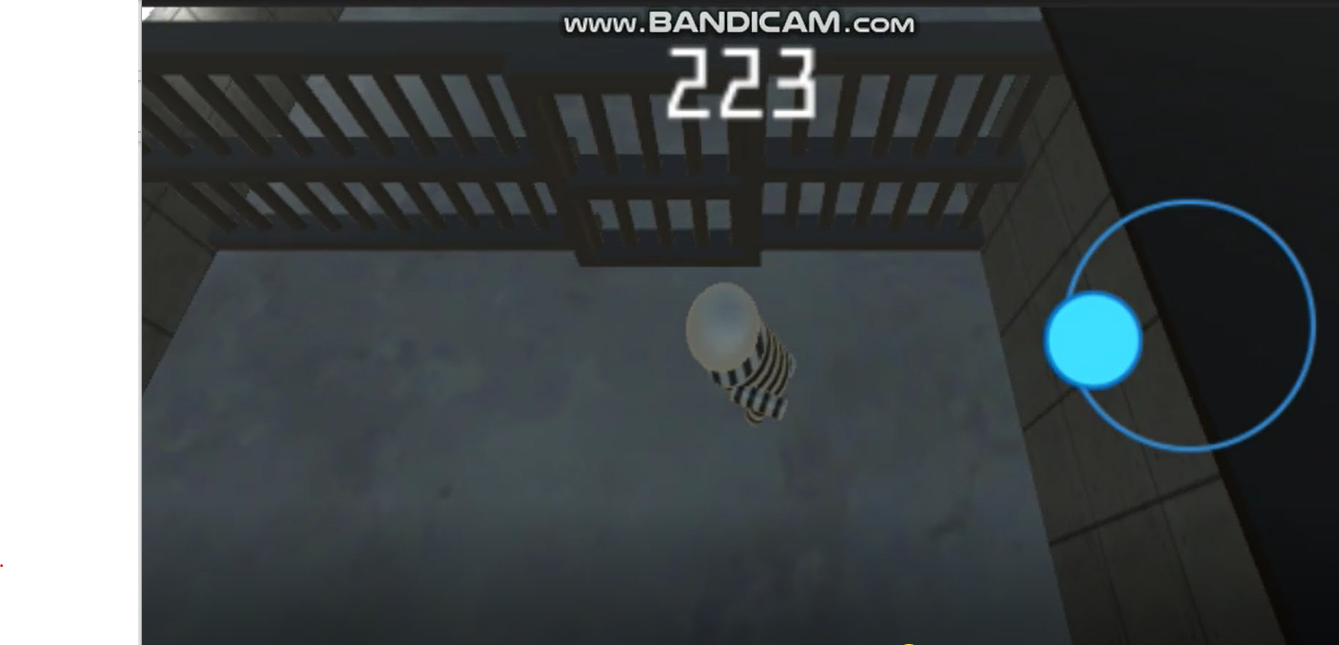
ここでは上の画像のように、丸を動かしてキャラクターを操作できるやつのことを指します。ジョイスティックの導入と、キャラクターがジョイスティックを動かした向きを向くようにする方法を初心者向けに解説していきます。
ジョイスティックの導入方法
なんだかとっても大変そうですが、
Asset Storeに無料で提供されているSimple Touch Controllerを使えば簡単! まずはこれをImportしましょう。
SimpleTouchControllerに同封されているSceneのExample1~3を参考にしましょう。Exampleでは球を動かすLeftControllerとカメラを動かすRightControllerが搭載されていますが、今回はプレイヤーをバーチャルパッドで動かせるだけなので、LeftControllerのみ使えるようにすれば良いです。
では、どんな操作が必要だったのか説明していきます。
SimpleTouchController>Scenes>Example2を開き、Left SimpleTouch Joystickをコピーして、使いたいシーンのCanvasにペーストします。
次にプレイヤーにPlayerMoveControllerをつけます。
このまま実行するとRight Controllerがないためにエラーになったり、
プレイヤーが正面を向いたまま移動したりしてしまうので、コードを少しいじります。(記事の一番下にPlayerMoveControllerをいじったコードが置いてあります。)
今回使うのはLeft Controllerなので、Right Controllerに関係する式は取っ払ってしまいましょう。
プレイヤーの向きをジョイスティックにと連動させる命令は、
//スティックの倒れた向きを向く の下に書かれているコードで制御しています。
float h2 = leftController.GetTouchPosition.x; ```
v2とh2にそれぞれ、Left Controllerのy軸の値とx軸の値をとり、
```if (v2 == 0 && h2 == 0) {
speed = 0f;
} else {
speed = 10f;
}```
あとLeft Controllerを動かしていないときはspeedが0になるようにしましょう。
勝手に動いてしまいます。
```Vector3 direction = new Vector3(h2,0,v2);
transform.localRotation = Quaternion.LookRotation (direction);```
向きを代入してあげています。
なお、以下のコードには取っ払い損ねているものもありますが、動きには影響ないです。
```C#:PlayerMoveController
using UnityEngine;
using System.Collections;
[RequireComponent(typeof(Rigidbody))]
public class PlayerMoveController : MonoBehaviour {
// PUBLIC
public SimpleTouchController leftController;
public SimpleTouchController rightController;
public Transform headTrans;
public float speed;
public float speedContinuousLook = 100f;
public float speedProgressiveLook = 3000f;
public GameObject Message;
// PRIVATE
private Rigidbody _rigidbody;
[SerializeField] bool continuousRightController = true;
Animator animator;
void Awake()
{
animator = this.gameObject.GetComponent <Animator> ();
_rigidbody = GetComponent<Rigidbody>();
}
public bool ContinuousRightController
{
set{continuousRightController = value;}
}
void Update()
{
// move
if (Message.activeSelf) {
speed = 0;
}else{
speed = 10f;
}
_rigidbody.velocity = new Vector3 (leftController.GetTouchPosition.x, 0, leftController.GetTouchPosition.y) * speed;
// スティックの倒れた向きを向く---------------------------------
float v2 = leftController.GetTouchPosition.y; //y軸方向の値をとる
float h2 = leftController.GetTouchPosition.x; //x軸方向の値をとる
Debug.Log (v2 + ", "+h2);
if (v2 == 0 && h2 == 0) {
speed = 0f;
} else {
speed = 10f;
}
//ジョイスティックが傾いている方向を向く
Vector3 direction = new Vector3(h2,0,v2);
transform.localRotation = Quaternion.LookRotation (direction);
// WalkingFlag
if (speed == 0f) {
Debug.Log (speed);
animator.SetBool ("WalkingFlag", false);
}else{
animator.SetBool ("WalkingFlag", true);
}
}
void UpdateAim(Vector2 value)
{
if(headTrans != null)
{
Quaternion rot = Quaternion.Euler(0f,
transform.localEulerAngles.y - value.x * Time.deltaTime * -speedProgressiveLook,
0f);
_rigidbody.MoveRotation(rot);
rot = Quaternion.Euler(headTrans.localEulerAngles.x - value.y * Time.deltaTime * speedProgressiveLook,
0f,
0f);
headTrans.localRotation = rot;
}
else
{
Quaternion rot = Quaternion.Euler(transform.localEulerAngles.x - value.y * Time.deltaTime * speedProgressiveLook,
transform.localEulerAngles.y + value.x * Time.deltaTime * speedProgressiveLook,
0f);
_rigidbody.MoveRotation(rot);
}
}
}
これで動くはずです。
お疲れさまでした!