今回は例題として、月ごとでシートを用意して、その中に月ごとの日付を記述しようと思います。
前回記事を参考にlaravelでExcelを扱えるようにしておきます。
laravel5.8でExcelお手軽ダウンロード
複数シートは公式サイトにありましたので、それを参考にさせていただきます。
構成としては、
・月ごとの日付を出すシート用クラス(DayPerMonthSheet.php)
・複数シートを管理するクラス(DateExport.php)
・ルートからダウンロードにつなぐコントローラー(SampleController.php)
月ごとの日付を出すシート用クラス(DayPerMonthSheet.php)
DayPerMonthSheet.php
<?php
namespace App\Exports;
use Carbon\Carbon;
use Maatwebsite\Excel\Concerns\FromCollection;
use Maatwebsite\Excel\Concerns\WithTitle;
class DayPerMonthSheet implements FromCollection , WithTitle
{
private $year;
private $month;
public function __construct($year, $month)
{
$this->year = $year;
$this->month = $month;
}
public function collection()
{
$daysInMonth = Carbon::parse($this->year. '-'. $this->month)->daysInMonth;
return collect([
0 => [range(1, $daysInMonth)]
]);
}
public function title(): string
{
return "{$this->month}月";
}
}
constructで年月を受け取り、collectionメソッドでシートに記述する内容(月ごとの日付)を返しています。
titleメソッドで、シートのタイトルを指定できます。
複数シートを管理するクラス(DateExport.php)
DateExport.php
<?php
namespace App\Exports;
use Maatwebsite\Excel\Concerns\Exportable;
use Maatwebsite\Excel\Concerns\WithMultipleSheets;
class DateExport implements WithMultipleSheets
{
use Exportable;
private $year;
public function __construct($year)
{
$this->year = $year;
}
public function sheets(): array
{
$sheets = [];
for($month = 1; $month <= 12; $month++){
$sheets[] = new DayPerMonthSheet($this->year, $month);
}
return $sheets;
}
}
複数シートを実現するために、WithMultipleSheetsを実装します。
sheetメソッドにシート用に作ったクラスを配列に詰めて、returnさせます。
ルートからダウンロードにつなぐコントローラー(SampleController.php)
SampleController.php
<?php
namespace App\Http\Controllers;
use App\Exports\DateExport;
class SampleController extends Controller
{
public function export()
{
return (new DateExport('2019'))->download('date.xlsx');
}
}
これでアクセスしたら、ダウンロードできます。(Excelファサードを使っても可)

Fontなんかを変えてみる
<?php
namespace App\Exports;
use Carbon\Carbon;
use Maatwebsite\Excel\Concerns\FromCollection;
use Maatwebsite\Excel\Concerns\ShouldAutoSize;
use Maatwebsite\Excel\Concerns\WithEvents;
use Maatwebsite\Excel\Concerns\WithMappedCells;
use Maatwebsite\Excel\Concerns\WithTitle;
use Maatwebsite\Excel\Events\AfterSheet;
class DayPerMonthSheet implements FromCollection, WithTitle, ShouldAutoSize, WithEvents
{
private $year;
private $month;
public function __construct($year, $month)
{
$this->year = $year;
$this->month = $month;
}
public function collection()
{
$daysInMonth = Carbon::parse($this->year. '-'. $this->month)->daysInMonth;
return collect([
0 => [range(1, $daysInMonth)]
]);
}
public function title(): string
{
return "{$this->month}月";
}
public function registerEvents(): array
{
return [
AfterSheet::class => function(AfterSheet $event) {
$cellRange = 'A1:W1'; // All headers
$event->sheet->getDelegate()->getStyle($cellRange)->getFont()->setSize(20);
},
];
}
}
これでフォントサイズを変更できました。
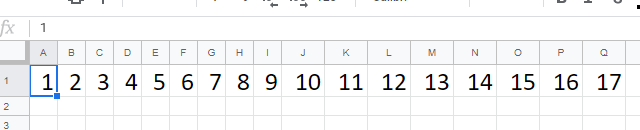