この記事では、ETHの基本的な送金をするためのコードを書きます。それらがどう言った意味を持つのかを最も基礎的な部分として作成します。
前提
- テストネットのアカウントを2つ持っていること(この部分についても簡単に書きます。)
- 必ず1つのウォレットには、すでに残高があること
- とりあえず送金してみたいという興味
今回の記事では以下のようになっています。
- ウォレットA:
- アドレス: 0x47FD9A30F48a656D9062aDE956c65f0ec8e2186c
- 残高 3.3455 ETH (Sepolia)
- ウォレットB:
- アドレス: 0xc20e03E025C3F40772f9bDc0F9BeD896fB5E3dCA
- 残高 0.27525 ETH (Sepolia)
アドレスの生成
イーサリアムのアドレス(公開鍵)とプライベートキーを作成します。
必要なパッケージ
$ npm i ethers typescript ts-node
生成するコード
generateAddress.ts
import { ethers } from 'ethers';
function generateEthereumAddress() {
const wallet = ethers.Wallet.createRandom();
return {
address: wallet.address,
privateKey: wallet.privateKey
};
}
const newAddress = generateEthereumAddress();
console.log(`アドレス(公開鍵): ${newAddress.address}`);
console.log(`秘密鍵: ${newAddress.privateKey}`);
出力結果↓
// 実行コマンド
$ npx ts-node generateAddress.ts
// 出力
アドレス(公開鍵): 0x0~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~
秘密鍵: 0x~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~
送金
transfer.ts
import { ethers } from "ethers";
// 送信者の秘密鍵と受信者のアドレスを設定
const senderPrivateKey = '<ここに送金元のアドレスの秘密鍵を入れる: ウォレットAの秘密鍵>';
const recipientAddress = '<ここに送金先のアドレスを入れる: ウォレット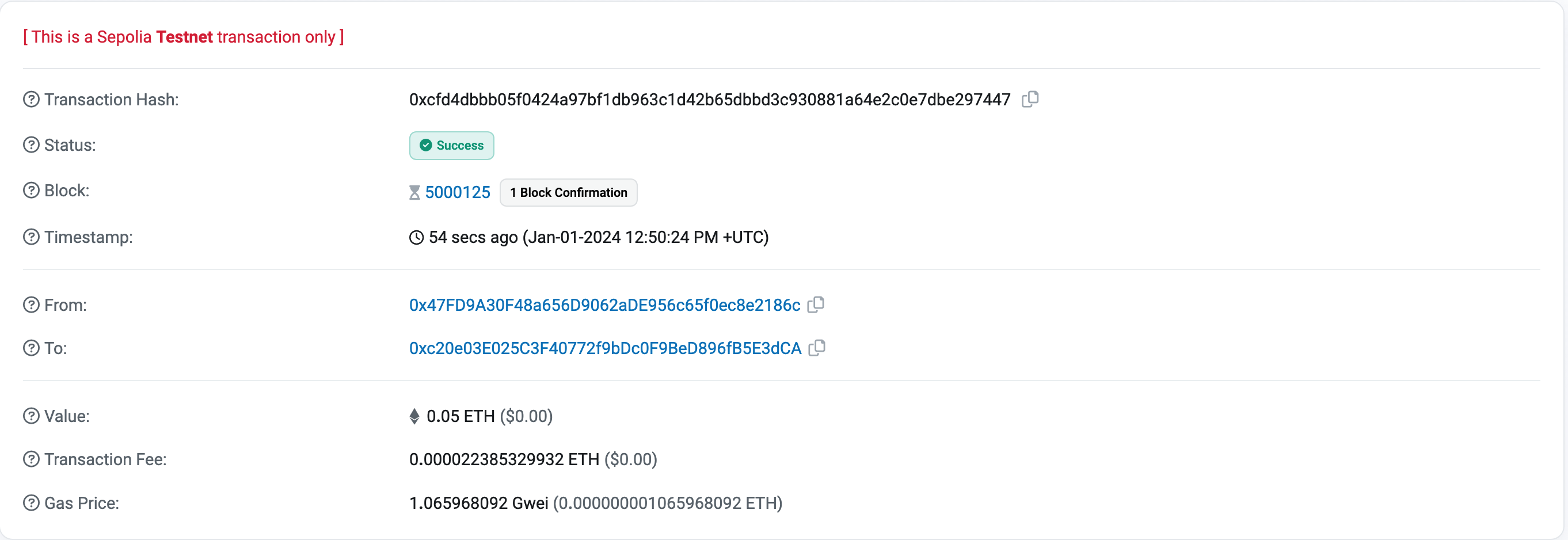
Bのアドレス>';
// SepoliaテストネットのRPCプロバイダー
const sepoliaProvider = new ethers.JsonRpcProvider('https://rpc.sepolia.org');
async function sendEth() {
// プロバイダーとウォレットを初期化
const wallet = new ethers.Wallet(senderPrivateKey, sepoliaProvider);
// トランザクションの作成(送金先と送金額)
const tx = {
to: recipientAddress,
value: ethers.parseEther("0.05")
};
// トランザクションを送信
const txResponse = await wallet.sendTransaction(tx);
console.log('トランザクションハッシュ:', txResponse.hash);
// トランザクションの確認が生成できたことを確認
const receipt = await txResponse.wait();
console.log('成功したトランザクションの詳細:', receipt);
}
sendEth();
出力結果↓
トランザクションハッシュ: 0xcfd4dbbb05f0424a97bf1db963c1d42b65dbbd3c930881a64e2c0e7dbe297447
成功したトランザクションの詳細: TransactionReceipt {
provider: JsonRpcProvider {},
to: '0xc20e03E025C3F40772f9bDc0F9BeD896fB5E3dCA',
from: '0x47FD9A30F48a656D9062aDE956c65f0ec8e2186c',
contractAddress: null,
hash: '0xcfd4dbbb05f0424a97bf1db963c1d42b65dbbd3c930881a64e2c0e7dbe297447',
index: 71,
blockHash: '0x4962365fc9a4f9211e8bfa0ad13a8b6139de3b589ff4fbe53e77b5b09428970b',
blockNumber: 5000125,
logsBloom: '0x00000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000',
gasUsed: 21000n,
cumulativeGasUsed: 9754898n,
gasPrice: 1065968092n,
type: 2,
status: 1,
root: undefined
}
エクスプローラーで確認
トランザクションの確認
Success! になっていますね!
From: 0x47FD9A30F48a656D9062aDE956c65f0ec8e2186c (ウォレットA)
To: 0xc20e03E025C3F40772f9bDc0F9BeD896fB5E3dCA (ウォレットB)
以上のようになっていて、送金ができていることがわかりますね!
ウォレットAの残高が減っているのか
元の残高: 3.3455 ETH
↓
送金後の残高: 3.295477614670068 ETH
ウォレットBの残高が増えているのか
元の残高: 0.27525 ETH
↓
送金後の残高: 0.32525 ETH
結果を見てみると、0.5がウォレットBには入っていますが、ウォレットAからは0.5より多く取られていますね。これは、トランザクションの詳細を見てみると
gasPrice: 1065968092n,
というところから手数料分がかかっていることがわかりますね。そのため、0.5の送金料と別に手数料が存在します。この手数料については、こちらのチャートが参考になります。(詳細は別途)