a-1-1-3.ActivityとFragment間のデータ受け渡し
目標設定
課題
- ActivityにFragmentで定義したXMLを組み込めるか。
- Activityに配置したFragmentのイベントをActivityで受け取れるか。
- ActivityからのイベントをFragmentで受け取れるか。
- ActivityからFragmentの呼び出しにパラメーターを追加できるか。
Github
テスト実装
FragmentTestActivity.kt
class FragmentTestActivity : AppCompatActivity(), TestFragment.OnButtonClickListener {
lateinit var fragmentContainerView: FragmentContainerView
lateinit var text: TextView
@SuppressLint("SetTextI18n")
override fun onCreate(savedInstanceState: Bundle?) {
super.onCreate(savedInstanceState)
setContentView(R.layout.activity_fragment_test)
/*
4. ActivityからFragmentの呼び出しにパラメーターを追加できるか。
・可能でした。
*/
val fragment = TestFragment()
val args = Bundle()
args.putString("string_arg", "Initial text.")
//args.putInt("int_arg", 33)
fragment.setArguments(args)
val transaction = supportFragmentManager.beginTransaction()
transaction.add(R.id.fragmentContainerView, fragment)
transaction.commit()
fragmentContainerView = findViewById(R.id.fragmentContainerView)
findViewById<Button>(R.id.button1).setOnClickListener {
text.setText("Activity event.")
}
}
/*
3. ActivityからのイベントをFragmentで受け取れるか。
・可能でした。
*/
override fun onTestFragmentViewCreated() {
text = fragmentContainerView.findViewById(R.id.textView1)
}
/*
2. Activityに配置したFragmentのイベントをActivityで受け取れるか。
・可能でした。
*/
@SuppressLint("SetTextI18n")
override fun onTestFragmentButtonClicked() {
text.setText("Fragment event.")
}
}
activity_fragment_test.xml
<?xml version="1.0" encoding="utf-8"?>
<androidx.constraintlayout.widget.ConstraintLayout
xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:app="http://schemas.android.com/apk/res-auto"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
tools:context=".FragmentTestActivity">
<!--
1. ActivityにFragmentで定義したXMLを組み込めるか。
・可能でした。
-->
<!--
android:name="com.example.androidtest.TestFragment"
でデフォルトのフラグメントを設定することができる。
-->
<androidx.fragment.app.FragmentContainerView
android:id="@+id/fragmentContainerView"
android:layout_width="match_parent"
android:layout_height="match_parent"
app:layout_constraintLeft_toLeftOf="parent"
app:layout_constraintRight_toRightOf="parent"
app:layout_constraintTop_toTopOf="parent"
app:layout_constraintBottom_toBottomOf="parent"
android:layout_marginStart="23dp"
android:layout_marginEnd="23dp"
android:layout_marginTop="23dp"
android:layout_marginBottom="23dp">
</androidx.fragment.app.FragmentContainerView>
<Button
android:id="@+id/button1"
android:layout_width="100dp"
android:layout_height="wrap_content"
android:text="Activity Button"
android:textAllCaps="false"
app:layout_constraintRight_toRightOf="parent"
app:layout_constraintBottom_toBottomOf="parent"
android:layout_marginEnd="23dp"
android:layout_marginBottom="23dp"
tools:ignore="HardcodedText"
/>
</androidx.constraintlayout.widget.ConstraintLayout>
TestFragment.kt
class TestFragment : Fragment() {
interface OnButtonClickListener {
fun onTestFragmentViewCreated()
fun onTestFragmentButtonClicked()
}
override fun onCreateView(inflater: LayoutInflater,
container: ViewGroup?,
savedInstanceState: Bundle?): View {
super.onCreateView(inflater, container, savedInstanceState)
return inflater.inflate(R.layout.fragment_test, container,
false)
}
@SuppressLint("SetTextI18n")
override fun onViewCreated(view: View, savedInstanceState: Bundle?) {
super.onViewCreated(view, savedInstanceState)
val args = arguments
args ?: return
val stringArg = args.getString("string_arg")
//val intArg = args.getInt("int_arg")
val listener = context as? OnButtonClickListener
listener?.onTestFragmentViewCreated()
val textView = view.findViewById<TextView>(R.id.textView1)
textView.setText(stringArg)
view.findViewById<Button>(R.id.button1).setOnClickListener {
listener?.onTestFragmentButtonClicked()
}
}
}
fragment_test.xml
<?xml version="1.0" encoding="utf-8"?>
<androidx.constraintlayout.widget.ConstraintLayout
xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:app="http://schemas.android.com/apk/res-auto"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent">
<TextView
android:id="@+id/textView1"
android:layout_width="100dp"
android:layout_height="wrap_content"
android:text="TextView"
app:layout_constraintLeft_toLeftOf="parent"
app:layout_constraintTop_toTopOf="parent"
tools:ignore="HardcodedText" />
<Button
android:id="@+id/button1"
android:layout_width="100dp"
android:layout_height="wrap_content"
android:text="Fragment Button"
android:textAllCaps="false"
app:layout_constraintLeft_toLeftOf="parent"
app:layout_constraintTop_toBottomOf="@id/textView1"
android:layout_marginTop="11dp"
tools:ignore="HardcodedText" />
</androidx.constraintlayout.widget.ConstraintLayout>
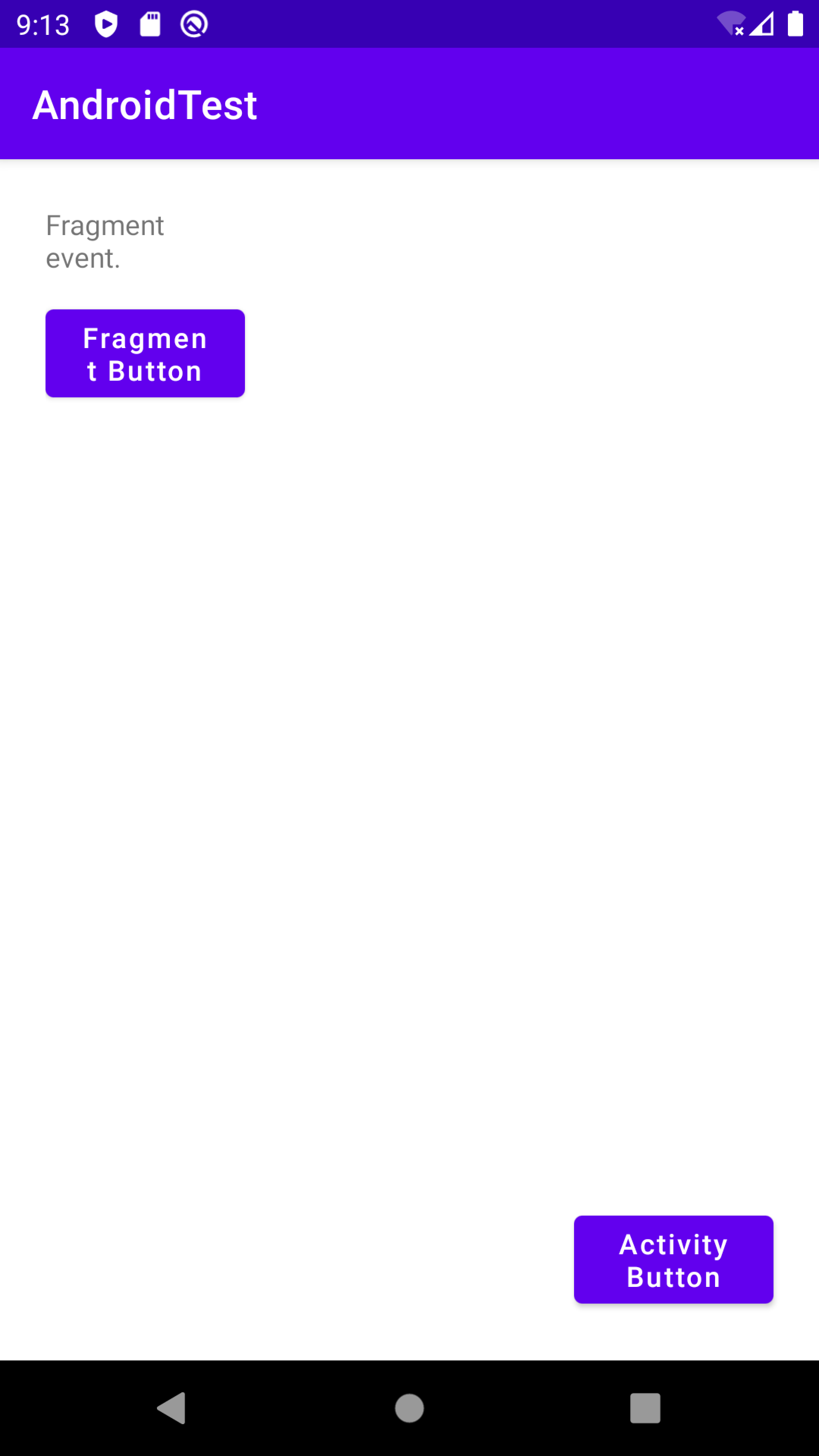