ちょこっと業務を簡略化したい
この記事では、ちょこっと効率化させたいことをプログラミングやツールをつかって解決していきます
ちょこっと削減した時間を、もっと人生楽しくなることに使いましょう!
はじめてPygameつかってみた
今まで、作業効率のためにコードを書いていたので、GUIは気にせず作成してきました。今回はちょっと遊び心入れてみました
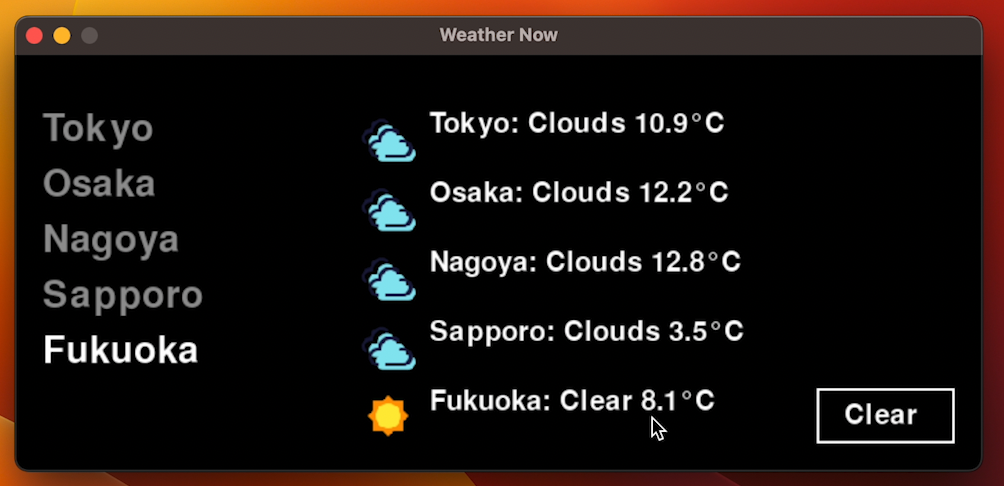
コード解説
① openweathermapのAPIを作る
② 天気アイコンはPngにしてフォルダーに保存しておく
③ Pathの位置を変更する
import pygame
import requests
import os
# OpenWeatherMap API設定
# ここ変更する
API_KEY = "Your_api_key"
BASE_URL="http://api.openweathermap.org/data/2.5/weather"
# 天気アイコン
# ここ変更する(画像用意しておく)
WEATHER_ICONS = {
"Clear": "sun.png",
"Clouds": "cloud.png",
"Rain": "rain.png",
"Snow": "snow.png",
"Thunderstorm": "storm.png"
}
# ここ変更する
CITIES = ["Tokyo", "Osaka", "Nagoya", "Sapporo", "Fukuoka"]
def get_weather(city):
params = {"q": city, "appid": API_KEY, "units": "metric"}
response = requests.get(BASE_URL, params=params)
if response.status_code == 200:
data = response.json()
weather = data["weather"][0]["main"]
temp = data["main"]["temp"]
return weather, temp
else:
return None, None
def get_icon_path(weather):
icon_file = WEATHER_ICONS.get(weather)
# ここ変更する(PATHの位置)
if icon_file:
return os.path.join(os.path.expanduser("~"), "Desktop/icons", icon_file)
return None
def main():
pygame.init()
# 画面設定
screen = pygame.display.set_mode((700, 300))
pygame.display.set_caption("シンプル天気アプリ")
font = pygame.font.SysFont("Meiryo", 40)
small_font = pygame.font.SysFont("Meiryo", 30)
clock = pygame.time.Clock()
selected_city_index = 0
weather_data = []
# クリアボタンの位置とサイズ
clear_button_rect = pygame.Rect(580, 240, 100, 40)
running = True
while running:
mouse_pos = pygame.mouse.get_pos()
for event in pygame.event.get():
if event.type == pygame.QUIT:
running = False
if event.type == pygame.KEYDOWN:
if event.key == pygame.K_DOWN:
selected_city_index = (selected_city_index + 1) % len(CITIES)
elif event.key == pygame.K_UP:
selected_city_index = (selected_city_index - 1) % len(CITIES)
elif event.key == pygame.K_RETURN:
city = CITIES[selected_city_index]
weather, temp = get_weather(city)
print(weather)
icon_path = get_icon_path(weather)
if icon_path and os.path.exists(icon_path):
icon = pygame.image.load(icon_path)
icon = pygame.transform.scale(icon, (40, 40))
# 温度の数字に℃を追加
temp_with_unit = f"{temp:.1f}"
weather_data.append((city, weather, temp_with_unit, icon))
if event.type == pygame.MOUSEBUTTONDOWN:
if clear_button_rect.collidepoint(mouse_pos):
weather_data.clear()
screen.fill((0, 0, 0))
# 都市リストの表示
for i, city in enumerate(CITIES):
color = (255, 255, 255) if i == selected_city_index else (150, 150, 150)
city_surface = font.render(city, True, color)
screen.blit(city_surface, (20, 40 + i * 40))
# 選択された都市の天気とアイコンを横に並べて表示
for i, (city, weather, temp_with_unit, icon) in enumerate(weather_data):
screen.blit(icon, (250, 40 + i * 50))
info_surface = small_font.render(f"{city}: {weather} {temp_with_unit}\u00b0C", True, (255, 255, 255))
screen.blit(info_surface, (300, 40 + i * 50))
# クリアボタンの描画(白枠で囲む)
pygame.draw.rect(screen, (255, 255, 255), clear_button_rect, 2)
clear_text = small_font.render("Clear", True, (255, 255, 255))
screen.blit(clear_text, (600, 250))
pygame.display.flip()
clock.tick(30)
pygame.quit()
if __name__ == "__main__":
main()
ーーChatGPTよりーー
pygameとは
Pygameは、Pythonでゲームやインタラクティブなアプリケーションを作成するためのオープンソースのライブラリです。2000年に初めて公開され、以来、多くの開発者に利用されています。PygameはSDL(Simple DirectMedia Layer)を基盤としており、マルチプラットフォーム対応で、Windows、macOS、Linuxといった主要なOSで動作します。
Pygameの特徴として、2Dグラフィックスの描画が簡単に行える点が挙げられます。例えば、画像の表示や図形の描画、アニメーションの実装を短いコードで実現できます。また、イベント駆動型の設計になっており、ユーザーの入力(キーボード、マウス)を簡単に処理できるため、ゲームだけでなく、インタラクティブなツールやアプリケーションの開発にも適しています。さらに、Pygameは軽量で依存関係が少ないため、シンプルな環境でもスムーズに動作します。
最後に
まだまだpythonの知らないライブラリについても挑戦していきます!