今回の記事ではクエリ言語のGraphQLでDynamoDBに保存されたデータを実際に画面上に出力してみたいと思います.
データのインサートに関しては以前の記事で投稿しているので
GraphQL、AppSyncを使ってDynamoDBにデータを挿入してみたを参照してみてください。
環境
- Pug を使用
- VuetifyのコンポーネントをUIで使用
- コーディング規約は Nuxt.js に則って StandardJS
- Visual Studio Code
Vuetifyの導入
Vue CLI の場合
Vue CLI を使っている場合は、
vue add vuetify
これでVuetifyのUIコンポーネントが使用できます
Nuxt.js の場合
Nuxt.js の場合は、Vuetify の Quick start のドキュメントにあるように、
$ yarn add @nuxtjs/vuetify -D
# OR
$ npm install @nuxtjs/vuetify -D
してインストールした後、 nuxt.config.js
の buildModules
に
{
buildModules: [
'@nuxtjs/vuetify'
]
}
を追加してあげたらOKです。
GraphQLを使えるようにする
AmplifyのライブラリからGraphQLのクエリをインポートし、API(GraphQL)を呼び出します。
plugins
フォルダに、 amplify.js
を作って、内容を以下のようにします。
import Amplify, { graphqlOperation } from 'aws-amplify'
import awsConfig from '@/src/aws-exports'
Amplify.configure(awsConfig)
export default ({ app }, inject) => {
inject('graphql', (operation, vars = {}) => Amplify.API.graphql(graphqlOperation(operation, vars)))
}
こうすることで、Vueコンポーネントの中から、
this.$graphql(...)
で呼び出してあげることができるようになります。
データの出力
DynamoDBに保存されているデータを出力するためのシンプルなコードを書きました。
-
default.vue
→簡易的なメインレイアウト -
index.vue
→Home.vue
コンポーネントを表示させる見出し -
Home.vue
→コンポーネント。DynamoDBのデータを出力させるコードを切り出している
<template lang="pug">
v-layout(
wrap
)
v-flex
home
</template>
<script>
import Home from '~/components/Home'
export default {
components: {
Home
},
middleware: 'auth',
data () {
}
}
</script>
<template lang="pug">
v-app
v-toolbar(color="indigo" dark fixed app)
v-toolbar-side-icon(@click.stop="drawer = !drawer")
v-tabs(color="indigo")
v-tab(nuxt to="/") OVERVIEW
v-tab(nuxt to="/signup") Customer
v-tab(nuxt to="/signin") SIGNIN
v-tab(nuxt to="/housekeeping") HOUSE KEEPING
v-tab(nuxt to="/reports") REPORTS
v-content
nuxt
v-footer(color="indigo" app)
span.white--text © 2017
</template>
<script>
export default {
data: () => ({
drawer: false
})
}
</script>
<template lang="pug">
v-container
v-layout(
row
wrap
)
v-flex(
xs12
sm6
md4
lg4
v-for="room in rooms"
:key="room.name"
)
v-card.ma-3(
flat
v-show="checkRoom(room)"
)
v-card-title
| {{ room.number }}
v-spacer
span(
v-if="room.guestInfo"
) {{ room.guestInfo.name }}
</template>
<script>
import { listUserTypes } from '@/src/graphql/queries'
export default {
data () {
return {
rooms: []
}
},
created () {
this.loadUserTypes()
},
methods: {
async loadUserTypes () {
const data = await this.$graphql(listUserTypes, {
limit: 100
})
}
}
}
</script>
※UserType
はテーブルの変数名です。
今回の記事の目的はDynamoDBのデータを出力させることなので、Home.vue
コンポーネントを中心に解説していきます。取得したいデータのテーブルは以下のようになっています

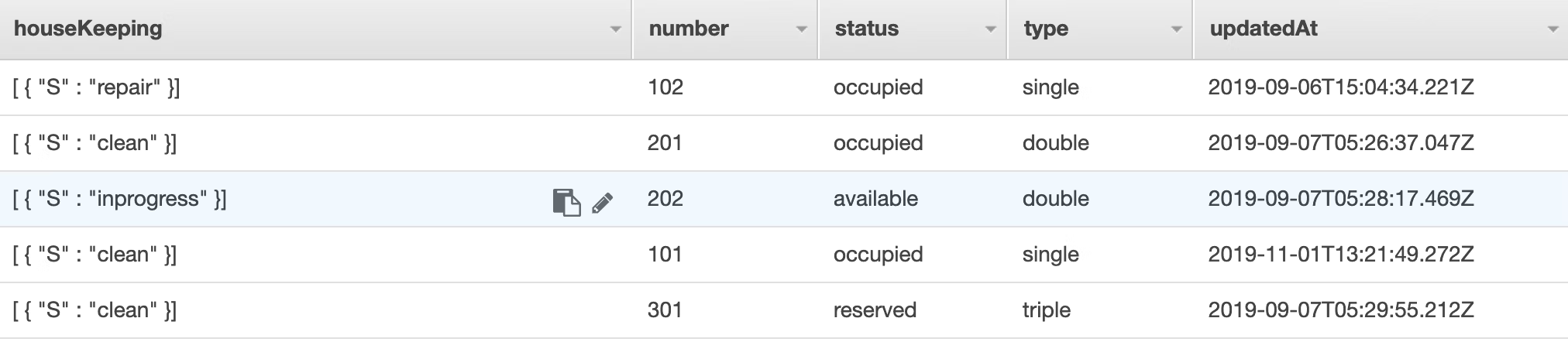
##出力までの手順
###1. listクエリをインポート
listクエリでデータの全てを取得したいので、query.js
の中にあるlistUserTypes
をインポートします
import { listUserTypes } from '@/src/graphql/queries'
###2. GraphQLクエリが取得したデータを変数に代入
listクエリで取得したUserType
テーブルのデータをrooms
に代入します
data () {
return {
rooms: []
}
},
created () {
this.loadUserTypes()
},
methods: {
async loadUserTypes () {
const user = await this.$graphql(listUserTypes, {
limit: 100
})
this.rooms = user.data.listUserTypes.items
}
}
-
created
フックにより、インスタンスが生成された直後loadUserTypes
メソッドを呼び出します。 -
loadUserTypes
メソッドはdata
の中に格納されているrooms
の空配列に対して、クエリで取得したデータを代入します
定数user
からdata.listUserTypes.items
と書いてあげることで、12個のデータをrooms
に代入することができます
3.出力
UIは以下のようになります。
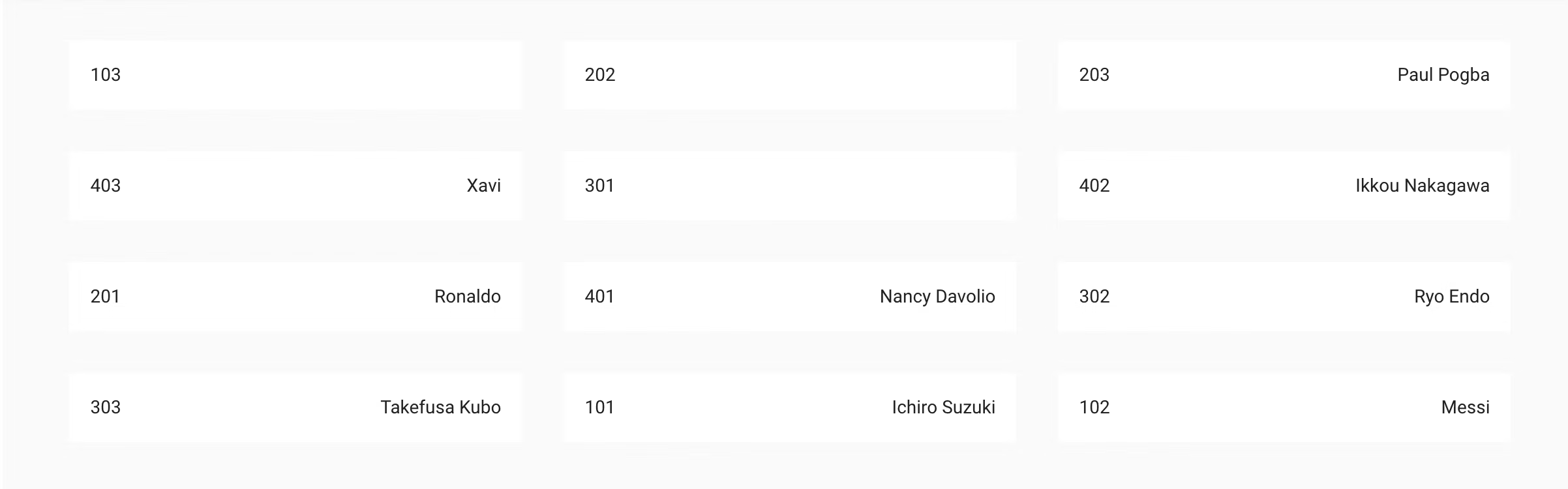
これだと見るに耐えないので少し修正を加えたいと思います。
部屋番号がランダムに並べてあるので、これを101から順に配置したいと思います。
そのために、sortメソッドを使います
loadUserTypes
メソッドにsort
メソッドを追加します
async loadUserTypes () {
const user = await this.$graphql(listUserTypes, {
limit: 12
})
const result = user.data.listUserTypes.items
result.sort(function (a, b) {
if (a.number > b.number) {
return 1
} else {
return -1
}
})
this.rooms = result
}
部屋番号順に並び替えることができました!
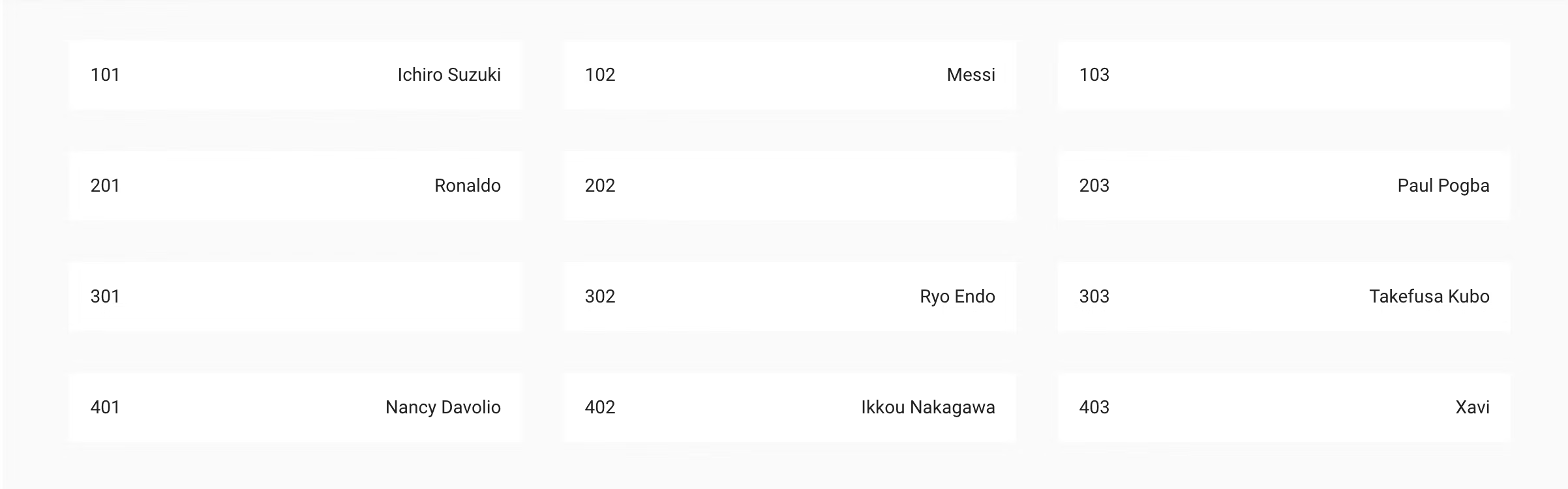