#Select 要素を操作
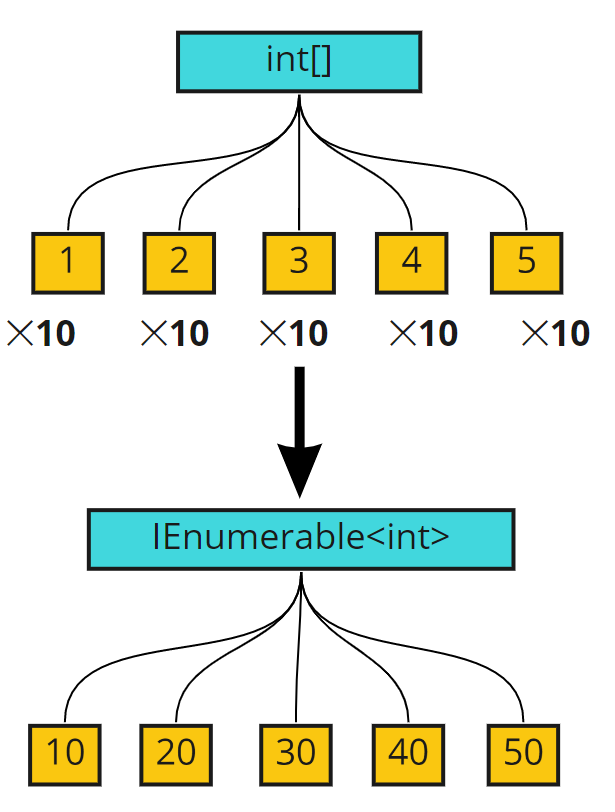
using System;
class Prog{
static void Main() {
int[] list ={1, 2, 3, 4, 5};
int[] list2 = new int[list.Length];
for(int i=0; i<list.Length; i++) {
list2[i] = list[i] * 10;
}
foreach(int i in list2){
Console.WriteLine( i);
}
}
}
LINQにすると
using System;
using System.Linq;
using System.Collections.Generic;
class Prog{
static void Main() {
int[] list ={1, 2, 3, 4, 5};
IEnumerable<int> list2 = list.Select( i => i*10);
foreach(int i in list2){
Console.WriteLine( i);
}
}
}
Selectは、各要素を操作してIEnumerable<TResult>で返す
#SelectMany 平坦化

using System;
using System.Collections.Generic;
class Prog{
static void Main() {
List<int[]> ls = new List<int[]>(); //配列のリスト
for(int i=0; i<3; i++){ //{0,0} {0,0} {0,0}の3つの配列が入ったリスト
ls.Add( new int[2]);
}
List<int> ls2 = new List<int>(); //これに全ての要素を入れ直す
foreach(int[] array in ls){
foreach(int i in array){
ls2.Add(i);
}
}
foreach(var val in ls2) {
Console.WriteLine(val);
}
}
}
LINQにすると
using System;
using System.Linq;
using System.Collections.Generic;
class Prog{
static void Main() {
int[] ls = { 2,2,2};
IEnumerable<int> ls2 = ls.SelectMany( x => new int[x] );
//IEnumerable<int[]> ls3 = ls.Select(x => new int[x]); //戻り値は配列のコレクション
foreach(int i in ls2) {
Console.WriteLine(i);
}
}
}
SelectManyは、コレクションの要素を操作してバラバラにして返します。
ラムダ式で作られる配列を1つずつintにして、intのコレクション
にして返します。
一方Selectは、要素の操作までは同じですがバラバラにしないで、int[]のコレクション
で返します。
#Where 条件で抽出

using System;
using System.Collections.Generic;
class Prog{
static void Main() {
int[] ls = {1,2,3,4,5};
List<int> ls2 = new List<int>();
foreach(int i in ls) {
if( i> 3) ls2.Add(i);
}
foreach(var val in ls2) {
Console.WriteLine(val);
}
}
}
LINQにすると
using System;
using System.Linq;
using System.Collections.Generic;
class Prog{
static void Main() {
int[] ls = {1,2,3,4,5};
IEnumerable<int> ls2 = ls.Where( x => x > 3);
foreach(int i in ls2) {
Console.WriteLine(i);
}
}
}
Whereは、条件を満たす要素だけ抽出します。
#Whereと、Selectの組み合わせ
int[] ls = {1,2,3,4,5};
//出力
40
50
4以上の数値だけに10をかけて出力
using System;
using System.Linq;
using System.Collections.Generic;
class Prog{
static void Main() {
int[] ls = {1,2,3,4,5};
IEnumerable<int> ls2 = ls.Where(x => x > 3).Select(x => x *10);
foreach(int i in ls2) {
Console.WriteLine(i);
}
}
}
#OrderBy 並べ替え

using System;
using System.Linq;
class Prog {
public static void Main() {
int[] ary = { 6, 12, 7, 1, 4 };
IOrderedEnumerable<int> order = ary.OrderBy(x => x);
foreach(int i in order) {
Console.WriteLine(i);
}
}
}
#匿名クラス
using System;
using System.Linq;
class Prog{
public static void Main(string[] args) {
string[] names = {
"ナポレオン",
"ピカソ",
"エジソン",
};
}
}
LINQを使って文字数を出力
ナポレオンは、5文字
ピカソは、3文字
エジソンは、4文字
using System;
using System.Linq;
class Prog{
public static void Main(string[] args) {
string[] names = {
"ナポレオン",
"ピカソ",
"エジソン",
};
foreach(var ins in names.Select((name) => new { Name = name, Count = name.Length })) {
string output = string.Format("{0}は、{1}文字", ins.Name, ins.Count);
Console.WriteLine(output);
}
}
}
Selectは、匿名クラスのインスタンスを返しています。