概要
ツイートに付加されている位置情報を Ruby + Twitter API で取得し、どのようなレスポンスが返ってきているのかを調べる。
位置情報ツイートの種類
今回、位置情報を取得するツイートのタイプは以下の3種類。
- 場所を指定したツイート
- 場所と正確な位置情報を指定したツイート
- Swarm (by Foursquare) アプリ https://ja.swarmapp.com/ からのツイート
『正確な位置情報』は、公式 Twitter アプリからツイートする際に付加することができる。
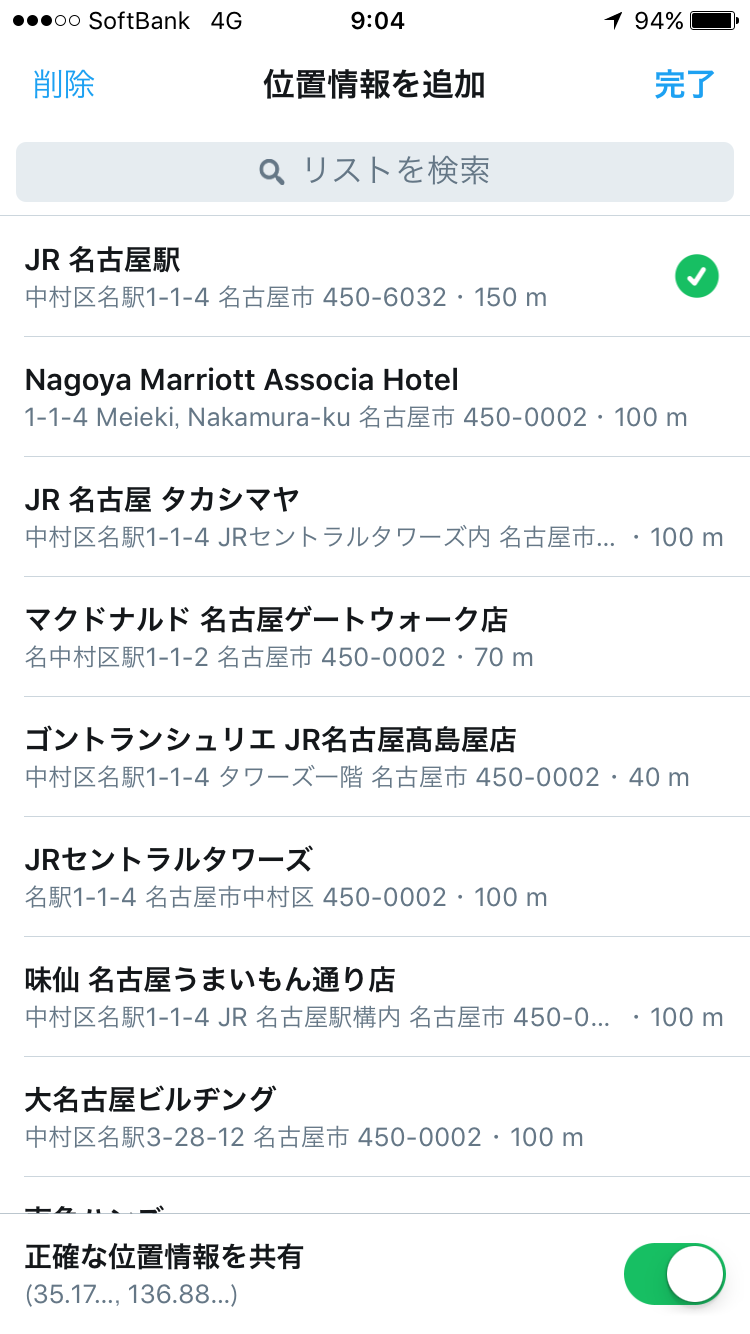
コールする Twitter API
今回コールする Twitter の API は以下の2種類。
- GET statuses/show/:id — Twitter Developers https://dev.twitter.com/rest/reference/get/statuses/show/id
- GET geo/id/:place_id — Twitter Developers https://dev.twitter.com/rest/reference/get/geo/id/place_id
ツイートの位置情報を取得するサンプルコード
twitter_api ライブラリのインストール
Twitter API のラッパー・ライブラリ https://rubygems.org/gems/twitter_api をインストール。
$ gem install twitter_api
サンプルコード
require 'twitter_api'
require 'json'
# Twitter API の認証情報をセット
t = TwitterAPI::Client.new({
:consumer_key => 'YOUR_CONSUMER_KEY',
:consumer_secret => 'YOUR_CONSUMER_SECRET',
:token => 'YOUR_ACCESS_TOKEN',
:token_secret => 'YOUR_ACCESS_SECRET'
})
def show_tweet(t, status_id)
# GET statuses/show/:id
s_res = t.statuses_show_id({'id' => status_id})
status = JSON.parse(s_res.body)
place_id = status['place']['id'] if status['place'] && status['place']['id']
# GET geo/id/:place_id
if place_id
p_res = t.geo_id_place_id({'place_id' => status['place']['id']})
place = JSON.parse(p_res.body)
end
puts "========== Status ID: #{status_id} =========="
puts JSON.pretty_generate({'text' => status['text']})
puts JSON.pretty_generate({'geo' => status['geo']})
puts JSON.pretty_generate({'coordinates' => status['coordinates']})
if place
puts "========== Place ID: #{place_id} =========="
puts JSON.pretty_generate({'name' => place['name']})
puts JSON.pretty_generate({'geometry' => place['geometry']})
puts JSON.pretty_generate({'centroid' => place['centroid']})
end
puts '========== GET statuses/show/:id =========='
puts JSON.pretty_generate(status)
if place
puts '========== GET geo/id/:place_id =========='
puts JSON.pretty_generate(place)
end
end
# JR名古屋駅 – 場所: JR 名古屋駅
show_tweet(t, '844701177438781441')
# JR名古屋駅 + 正確な位置情報 – 場所: JR 名古屋駅
show_tweet(t, '844701423103365124')
# Swarm (@ JR 名古屋駅 in Nagoya-shi, Aichi-ken)
show_tweet(t, '844701716528447492')
ツイートから取得した位置情報
場所を指定したツイート
場所を指定したツイートでは、ツイートの情報に緯度・経度は含まれない。
場所 (Place) の情報に緯度経度が含まれている。こちらは、場所が持つエリア (ポリゴン) の中心点 (重心 centroid) だと思われる。
========== Status ID: 844701177438781441 ==========
{
"text": "JR名古屋駅"
}
{
"geo": null
}
{
"coordinates": null
}
========== Place ID: 09efc38f35d6c000 ==========
{
"name": "JR 名古屋駅"
}
{
"geometry": {
"type": "Point",
"coordinates": [
136.881856394562,
35.1707973153594
]
}
}
{
"centroid": [
136.881856394562,
35.1707973153594
]
}
場所と正確な位置情報を指定したツイート
場所と正確な位置情報を指定したツイートでは、ツイートの情報に緯度・経度が含まれる。
場所 (Place) の情報にも緯度経度が含まれている。こちらも、場所が持つエリア (ポリゴン) の中心点 (重心 centroid) だと思われる。
========== Status ID: 844701423103365124 ==========
{
"text": "JR名古屋駅 + 正確な位置情報"
}
{
"geo": {
"type": "Point",
"coordinates": [
35.1714313,
136.88348942
]
}
}
{
"coordinates": {
"type": "Point",
"coordinates": [
136.88348942,
35.1714313
]
}
}
========== Place ID: 09efc38f35d6c000 ==========
{
"name": "JR 名古屋駅"
}
{
"geometry": {
"type": "Point",
"coordinates": [
136.881856394562,
35.1707973153594
]
}
}
{
"centroid": [
136.881856394562,
35.1707973153594
]
}
Swarm (by Foursquare) からのツイート
Swarm (by Foursquare) からのツイートでは、ツイートの情報に緯度・経度が含まれる。
場所 (Place) の情報にも緯度経度が含まれているが、指定した「JR 名古屋駅」ではなく、なぜか「名古屋市 中村区」に紐付いている。
========== Status ID: 844701716528447492 ==========
{
"text": "Swarm (@ JR 名古屋駅 in Nagoya-shi, Aichi-ken) https://t.co/IcfhIqAehH"
}
{
"geo": {
"type": "Point",
"coordinates": [
35.1706326,
136.88226594
]
}
}
{
"coordinates": {
"type": "Point",
"coordinates": [
136.88226594,
35.1706326
]
}
}
========== Place ID: d11899c51981283a ==========
{
"name": "名古屋市 中村区"
}
{
"geometry": null
}
{
"centroid": [
136.870387809842,
35.16556250243865
]
}
レスポンスJSONすべてを含む出力結果
場所を指定したツイート
========== Status ID: 844701177438781441 ==========
{
"text": "JR名古屋駅"
}
{
"geo": null
}
{
"coordinates": null
}
========== Place ID: 09efc38f35d6c000 ==========
{
"name": "JR 名古屋駅"
}
{
"geometry": {
"type": "Point",
"coordinates": [
136.881856394562,
35.1707973153594
]
}
}
{
"centroid": [
136.881856394562,
35.1707973153594
]
}
========== GET statuses/show/:id ==========
{
"created_at": "Thu Mar 23 00:03:49 +0000 2017",
"id": 844701177438781441,
"id_str": "844701177438781441",
"text": "JR名古屋駅",
"truncated": false,
"entities": {
"hashtags": [
],
"symbols": [
],
"user_mentions": [
],
"urls": [
]
},
"source": "<a href=\"http://twitter.com/download/iphone\" rel=\"nofollow\">Twitter for iPhone</a>",
"in_reply_to_status_id": null,
"in_reply_to_status_id_str": null,
"in_reply_to_user_id": null,
"in_reply_to_user_id_str": null,
"in_reply_to_screen_name": null,
"user": {
"id": 14165670,
"id_str": "14165670",
"name": "niwasawa",
"screen_name": "niwasawa",
"location": "名古屋",
"description": "迷子になりがちな地図・位置情報系プログラマ。迷子ラボ https://t.co/5bFcUNmHtY はじめました。",
"url": "http://t.co/FlopUlzGhS",
"entities": {
"url": {
"urls": [
{
"url": "http://t.co/FlopUlzGhS",
"expanded_url": "http://d.hatena.ne.jp/niwasawa/",
"display_url": "d.hatena.ne.jp/niwasawa/",
"indices": [
0,
22
]
}
]
},
"description": {
"urls": [
{
"url": "https://t.co/5bFcUNmHtY",
"expanded_url": "http://maigolab.hatenablog.com/",
"display_url": "maigolab.hatenablog.com",
"indices": [
27,
50
]
}
]
}
},
"protected": false,
"followers_count": 1083,
"friends_count": 812,
"listed_count": 104,
"created_at": "Mon Mar 17 20:35:09 +0000 2008",
"favourites_count": 3333,
"utc_offset": 32400,
"time_zone": "Tokyo",
"geo_enabled": true,
"verified": false,
"statuses_count": 6441,
"lang": "ja",
"contributors_enabled": false,
"is_translator": false,
"is_translation_enabled": false,
"profile_background_color": "C0DEED",
"profile_background_image_url": "http://abs.twimg.com/images/themes/theme1/bg.png",
"profile_background_image_url_https": "https://abs.twimg.com/images/themes/theme1/bg.png",
"profile_background_tile": false,
"profile_image_url": "http://pbs.twimg.com/profile_images/253822088/maigo_twitter_normal.png",
"profile_image_url_https": "https://pbs.twimg.com/profile_images/253822088/maigo_twitter_normal.png",
"profile_link_color": "1DA1F2",
"profile_sidebar_border_color": "C0DEED",
"profile_sidebar_fill_color": "DDEEF6",
"profile_text_color": "333333",
"profile_use_background_image": true,
"has_extended_profile": false,
"default_profile": true,
"default_profile_image": false,
"following": true,
"follow_request_sent": false,
"notifications": false,
"translator_type": "none"
},
"geo": null,
"coordinates": null,
"place": {
"id": "09efc38f35d6c000",
"url": "https://api.twitter.com/1.1/geo/id/09efc38f35d6c000.json",
"place_type": "poi",
"name": "JR 名古屋駅",
"full_name": "JR 名古屋駅",
"country_code": "JP",
"country": "日本",
"contained_within": [
],
"bounding_box": {
"type": "Polygon",
"coordinates": [
[
[
136.881856394562,
35.1707973153594
],
[
136.881856394562,
35.1707973153594
],
[
136.881856394562,
35.1707973153594
],
[
136.881856394562,
35.1707973153594
]
]
]
},
"attributes": {
}
},
"contributors": null,
"is_quote_status": false,
"retweet_count": 0,
"favorite_count": 0,
"favorited": false,
"retweeted": false,
"lang": "ja"
}
========== GET geo/id/:place_id ==========
{
"id": "09efc38f35d6c000",
"url": "https://api.twitter.com/1.1/geo/id/09efc38f35d6c000.json",
"place_type": "poi",
"name": "JR 名古屋駅",
"full_name": "JR 名古屋駅",
"country_code": "JP",
"country": "日本",
"contained_within": [
{
"id": "d11899c51981283a",
"url": "https://api.twitter.com/1.1/geo/id/d11899c51981283a.json",
"place_type": "city",
"name": "名古屋市 中村区",
"full_name": "愛知 名古屋市 中村区",
"country_code": "JP",
"country": "日本",
"centroid": [
136.870387809842,
35.16556250243865
],
"bounding_box": {
"type": "Polygon",
"coordinates": [
[
[
136.834336005073,
35.1427100006125
],
[
136.834336005073,
35.1884150042648
],
[
136.900606995939,
35.1884150042648
],
[
136.900606995939,
35.1427100006125
],
[
136.834336005073,
35.1427100006125
]
]
]
},
"attributes": {
}
}
],
"geometry": {
"type": "Point",
"coordinates": [
136.881856394562,
35.1707973153594
]
},
"polylines": [
],
"centroid": [
136.881856394562,
35.1707973153594
],
"bounding_box": {
"type": "Polygon",
"coordinates": [
[
[
136.881856394562,
35.1707973153594
],
[
136.881856394562,
35.1707973153594
],
[
136.881856394562,
35.1707973153594
],
[
136.881856394562,
35.1707973153594
],
[
136.881856394562,
35.1707973153594
]
]
]
},
"attributes": {
"street_address": "中村区名駅1-1-4 名古屋市 450-6032"
},
"vendor_info": {
"yelp": {
"url": "https://www.yelp.co.jp/biz/jr-%E5%90%8D%E5%8F%A4%E5%B1%8B%E9%A7%85-%E5%90%8D%E5%8F%A4%E5%B1%8B%E5%B8%82-3?adjust_creative=twitter&utm_campaign=yelp_feed&utm_medium=feed_v2&utm_source=twitter",
"mobile_url": "https://m.yelp.com/biz/jr-%E5%90%8D%E5%8F%A4%E5%B1%8B%E9%A7%85-%E5%90%8D%E5%8F%A4%E5%B1%8B%E5%B8%82-3?country=JP&adjust_creative=twitter&utm_campaign=yelp_feed&utm_medium=feed_v2&utm_source=twitter",
"business_id": "pqt6QfWKsc0CICrL5oroMg"
}
}
}
場所と正確な位置情報を指定したツイート
========== Status ID: 844701423103365124 ==========
{
"text": "JR名古屋駅 + 正確な位置情報"
}
{
"geo": {
"type": "Point",
"coordinates": [
35.1714313,
136.88348942
]
}
}
{
"coordinates": {
"type": "Point",
"coordinates": [
136.88348942,
35.1714313
]
}
}
========== Place ID: 09efc38f35d6c000 ==========
{
"name": "JR 名古屋駅"
}
{
"geometry": {
"type": "Point",
"coordinates": [
136.881856394562,
35.1707973153594
]
}
}
{
"centroid": [
136.881856394562,
35.1707973153594
]
}
========== GET statuses/show/:id ==========
{
"created_at": "Thu Mar 23 00:04:47 +0000 2017",
"id": 844701423103365124,
"id_str": "844701423103365124",
"text": "JR名古屋駅 + 正確な位置情報",
"truncated": false,
"entities": {
"hashtags": [
],
"symbols": [
],
"user_mentions": [
],
"urls": [
]
},
"source": "<a href=\"http://twitter.com/download/iphone\" rel=\"nofollow\">Twitter for iPhone</a>",
"in_reply_to_status_id": null,
"in_reply_to_status_id_str": null,
"in_reply_to_user_id": null,
"in_reply_to_user_id_str": null,
"in_reply_to_screen_name": null,
"user": {
"id": 14165670,
"id_str": "14165670",
"name": "niwasawa",
"screen_name": "niwasawa",
"location": "名古屋",
"description": "迷子になりがちな地図・位置情報系プログラマ。迷子ラボ https://t.co/5bFcUNmHtY はじめました。",
"url": "http://t.co/FlopUlzGhS",
"entities": {
"url": {
"urls": [
{
"url": "http://t.co/FlopUlzGhS",
"expanded_url": "http://d.hatena.ne.jp/niwasawa/",
"display_url": "d.hatena.ne.jp/niwasawa/",
"indices": [
0,
22
]
}
]
},
"description": {
"urls": [
{
"url": "https://t.co/5bFcUNmHtY",
"expanded_url": "http://maigolab.hatenablog.com/",
"display_url": "maigolab.hatenablog.com",
"indices": [
27,
50
]
}
]
}
},
"protected": false,
"followers_count": 1083,
"friends_count": 812,
"listed_count": 104,
"created_at": "Mon Mar 17 20:35:09 +0000 2008",
"favourites_count": 3333,
"utc_offset": 32400,
"time_zone": "Tokyo",
"geo_enabled": true,
"verified": false,
"statuses_count": 6441,
"lang": "ja",
"contributors_enabled": false,
"is_translator": false,
"is_translation_enabled": false,
"profile_background_color": "C0DEED",
"profile_background_image_url": "http://abs.twimg.com/images/themes/theme1/bg.png",
"profile_background_image_url_https": "https://abs.twimg.com/images/themes/theme1/bg.png",
"profile_background_tile": false,
"profile_image_url": "http://pbs.twimg.com/profile_images/253822088/maigo_twitter_normal.png",
"profile_image_url_https": "https://pbs.twimg.com/profile_images/253822088/maigo_twitter_normal.png",
"profile_link_color": "1DA1F2",
"profile_sidebar_border_color": "C0DEED",
"profile_sidebar_fill_color": "DDEEF6",
"profile_text_color": "333333",
"profile_use_background_image": true,
"has_extended_profile": false,
"default_profile": true,
"default_profile_image": false,
"following": true,
"follow_request_sent": false,
"notifications": false,
"translator_type": "none"
},
"geo": {
"type": "Point",
"coordinates": [
35.1714313,
136.88348942
]
},
"coordinates": {
"type": "Point",
"coordinates": [
136.88348942,
35.1714313
]
},
"place": {
"id": "09efc38f35d6c000",
"url": "https://api.twitter.com/1.1/geo/id/09efc38f35d6c000.json",
"place_type": "poi",
"name": "JR 名古屋駅",
"full_name": "JR 名古屋駅",
"country_code": "JP",
"country": "日本",
"contained_within": [
],
"bounding_box": {
"type": "Polygon",
"coordinates": [
[
[
136.881856394562,
35.1707973153594
],
[
136.881856394562,
35.1707973153594
],
[
136.881856394562,
35.1707973153594
],
[
136.881856394562,
35.1707973153594
]
]
]
},
"attributes": {
}
},
"contributors": null,
"is_quote_status": false,
"retweet_count": 0,
"favorite_count": 0,
"favorited": false,
"retweeted": false,
"lang": "ja"
}
========== GET geo/id/:place_id ==========
{
"id": "09efc38f35d6c000",
"url": "https://api.twitter.com/1.1/geo/id/09efc38f35d6c000.json",
"place_type": "poi",
"name": "JR 名古屋駅",
"full_name": "JR 名古屋駅",
"country_code": "JP",
"country": "日本",
"contained_within": [
{
"id": "d11899c51981283a",
"url": "https://api.twitter.com/1.1/geo/id/d11899c51981283a.json",
"place_type": "city",
"name": "名古屋市 中村区",
"full_name": "愛知 名古屋市 中村区",
"country_code": "JP",
"country": "日本",
"centroid": [
136.870387809842,
35.16556250243865
],
"bounding_box": {
"type": "Polygon",
"coordinates": [
[
[
136.834336005073,
35.1427100006125
],
[
136.834336005073,
35.1884150042648
],
[
136.900606995939,
35.1884150042648
],
[
136.900606995939,
35.1427100006125
],
[
136.834336005073,
35.1427100006125
]
]
]
},
"attributes": {
}
}
],
"geometry": {
"type": "Point",
"coordinates": [
136.881856394562,
35.1707973153594
]
},
"polylines": [
],
"centroid": [
136.881856394562,
35.1707973153594
],
"bounding_box": {
"type": "Polygon",
"coordinates": [
[
[
136.881856394562,
35.1707973153594
],
[
136.881856394562,
35.1707973153594
],
[
136.881856394562,
35.1707973153594
],
[
136.881856394562,
35.1707973153594
],
[
136.881856394562,
35.1707973153594
]
]
]
},
"attributes": {
"street_address": "中村区名駅1-1-4 名古屋市 450-6032"
},
"vendor_info": {
"yelp": {
"url": "https://www.yelp.co.jp/biz/jr-%E5%90%8D%E5%8F%A4%E5%B1%8B%E9%A7%85-%E5%90%8D%E5%8F%A4%E5%B1%8B%E5%B8%82-3?adjust_creative=twitter&utm_campaign=yelp_feed&utm_medium=feed_v2&utm_source=twitter",
"mobile_url": "https://m.yelp.com/biz/jr-%E5%90%8D%E5%8F%A4%E5%B1%8B%E9%A7%85-%E5%90%8D%E5%8F%A4%E5%B1%8B%E5%B8%82-3?country=JP&adjust_creative=twitter&utm_campaign=yelp_feed&utm_medium=feed_v2&utm_source=twitter",
"business_id": "pqt6QfWKsc0CICrL5oroMg"
}
}
}
Swarm (by Foursquare) からのツイート
========== Status ID: 844701716528447492 ==========
{
"text": "Swarm (@ JR 名古屋駅 in Nagoya-shi, Aichi-ken) https://t.co/IcfhIqAehH"
}
{
"geo": {
"type": "Point",
"coordinates": [
35.1706326,
136.88226594
]
}
}
{
"coordinates": {
"type": "Point",
"coordinates": [
136.88226594,
35.1706326
]
}
}
========== Place ID: d11899c51981283a ==========
{
"name": "名古屋市 中村区"
}
{
"geometry": null
}
{
"centroid": [
136.870387809842,
35.16556250243865
]
}
========== GET statuses/show/:id ==========
{
"created_at": "Thu Mar 23 00:05:57 +0000 2017",
"id": 844701716528447492,
"id_str": "844701716528447492",
"text": "Swarm (@ JR 名古屋駅 in Nagoya-shi, Aichi-ken) https://t.co/IcfhIqAehH",
"truncated": false,
"entities": {
"hashtags": [
],
"symbols": [
],
"user_mentions": [
],
"urls": [
{
"url": "https://t.co/IcfhIqAehH",
"expanded_url": "https://www.swarmapp.com/c/hhdjWl1k5vG",
"display_url": "swarmapp.com/c/hhdjWl1k5vG",
"indices": [
43,
66
]
}
]
},
"source": "<a href=\"http://foursquare.com\" rel=\"nofollow\">Foursquare</a>",
"in_reply_to_status_id": null,
"in_reply_to_status_id_str": null,
"in_reply_to_user_id": null,
"in_reply_to_user_id_str": null,
"in_reply_to_screen_name": null,
"user": {
"id": 14165670,
"id_str": "14165670",
"name": "niwasawa",
"screen_name": "niwasawa",
"location": "名古屋",
"description": "迷子になりがちな地図・位置情報系プログラマ。迷子ラボ https://t.co/5bFcUNmHtY はじめました。",
"url": "http://t.co/FlopUlzGhS",
"entities": {
"url": {
"urls": [
{
"url": "http://t.co/FlopUlzGhS",
"expanded_url": "http://d.hatena.ne.jp/niwasawa/",
"display_url": "d.hatena.ne.jp/niwasawa/",
"indices": [
0,
22
]
}
]
},
"description": {
"urls": [
{
"url": "https://t.co/5bFcUNmHtY",
"expanded_url": "http://maigolab.hatenablog.com/",
"display_url": "maigolab.hatenablog.com",
"indices": [
27,
50
]
}
]
}
},
"protected": false,
"followers_count": 1083,
"friends_count": 812,
"listed_count": 104,
"created_at": "Mon Mar 17 20:35:09 +0000 2008",
"favourites_count": 3333,
"utc_offset": 32400,
"time_zone": "Tokyo",
"geo_enabled": true,
"verified": false,
"statuses_count": 6441,
"lang": "ja",
"contributors_enabled": false,
"is_translator": false,
"is_translation_enabled": false,
"profile_background_color": "C0DEED",
"profile_background_image_url": "http://abs.twimg.com/images/themes/theme1/bg.png",
"profile_background_image_url_https": "https://abs.twimg.com/images/themes/theme1/bg.png",
"profile_background_tile": false,
"profile_image_url": "http://pbs.twimg.com/profile_images/253822088/maigo_twitter_normal.png",
"profile_image_url_https": "https://pbs.twimg.com/profile_images/253822088/maigo_twitter_normal.png",
"profile_link_color": "1DA1F2",
"profile_sidebar_border_color": "C0DEED",
"profile_sidebar_fill_color": "DDEEF6",
"profile_text_color": "333333",
"profile_use_background_image": true,
"has_extended_profile": false,
"default_profile": true,
"default_profile_image": false,
"following": true,
"follow_request_sent": false,
"notifications": false,
"translator_type": "none"
},
"geo": {
"type": "Point",
"coordinates": [
35.1706326,
136.88226594
]
},
"coordinates": {
"type": "Point",
"coordinates": [
136.88226594,
35.1706326
]
},
"place": {
"id": "d11899c51981283a",
"url": "https://api.twitter.com/1.1/geo/id/d11899c51981283a.json",
"place_type": "city",
"name": "名古屋市 中村区",
"full_name": "愛知 名古屋市 中村区",
"country_code": "JP",
"country": "日本",
"contained_within": [
],
"bounding_box": {
"type": "Polygon",
"coordinates": [
[
[
136.834336005073,
35.1427100006125
],
[
136.900606995939,
35.1427100006125
],
[
136.900606995939,
35.1884150042648
],
[
136.834336005073,
35.1884150042648
]
]
]
},
"attributes": {
}
},
"contributors": null,
"is_quote_status": false,
"retweet_count": 0,
"favorite_count": 0,
"favorited": false,
"retweeted": false,
"possibly_sensitive": false,
"possibly_sensitive_appealable": false,
"lang": "ja"
}
========== GET geo/id/:place_id ==========
{
"id": "d11899c51981283a",
"url": "https://api.twitter.com/1.1/geo/id/d11899c51981283a.json",
"place_type": "city",
"name": "名古屋市 中村区",
"full_name": "愛知 名古屋市 中村区",
"country_code": "JP",
"country": "日本",
"contained_within": [
{
"id": "d79b76231f5f6ffa",
"url": "https://api.twitter.com/1.1/geo/id/d79b76231f5f6ffa.json",
"place_type": "admin",
"name": "中部地方",
"full_name": "静岡 中部地方",
"country_code": "JP",
"country": "日本",
"centroid": [
137.49185524029832,
36.565928
],
"bounding_box": {
"type": "Polygon",
"coordinates": [
[
[
135.449192,
34.578092
],
[
135.449192,
38.553764
],
[
139.900074,
38.553764
],
[
139.900074,
34.578092
],
[
135.449192,
34.578092
]
]
]
},
"attributes": {
}
}
],
"geometry": null,
"polylines": [
],
"centroid": [
136.870387809842,
35.16556250243865
],
"bounding_box": {
"type": "Polygon",
"coordinates": [
[
[
136.834336005073,
35.1427100006125
],
[
136.834336005073,
35.1884150042648
],
[
136.900606995939,
35.1884150042648
],
[
136.900606995939,
35.1427100006125
],
[
136.834336005073,
35.1427100006125
]
]
]
},
"attributes": {
"geotagCount": "360",
"190533:id": "city_23_105"
}
}
参考資料
- Places — Twitter Developers https://dev.twitter.com/overview/api/places
- GET statuses/show/:id — Twitter Developers https://dev.twitter.com/rest/reference/get/statuses/show/id
- GET geo/id/:place_id — Twitter Developers https://dev.twitter.com/rest/reference/get/geo/id/place_id
- twitter_api | RubyGems.org | your community gem host https://rubygems.org/gems/twitter_api