概要
- Xcode 11.0 から導入された SwiftUI でシンプルな Hello World iOS アプリを書く
環境
- Xcode 11.0
- Swift 5.1
- SwiftUI
SwiftUI とは
Xcode - SwiftUI - Apple Developer
SwiftUIでは、Swiftを利用してすべてのAppleプラットフォーム向けのユーザーインターフェイスを、革新的かつ極めてシンプルに構築することができます。
Xcode - SwiftUI - Apple Developer
SwiftUIは宣言型シンタックスを使用しているため、ユーザーインターフェイスの動作をシンプルに記述することができます。たとえば、テキストフィールドからなるアイテムのリストを作成すると書いてから、各フィールドの配置、フォント、色を記述するといった具合です。
プロジェクトの作成
テンプレートから iOS の Single View App を選択する。

以下のオプションを選択する。
- Language: Swift
- User Interface: SwiftUI
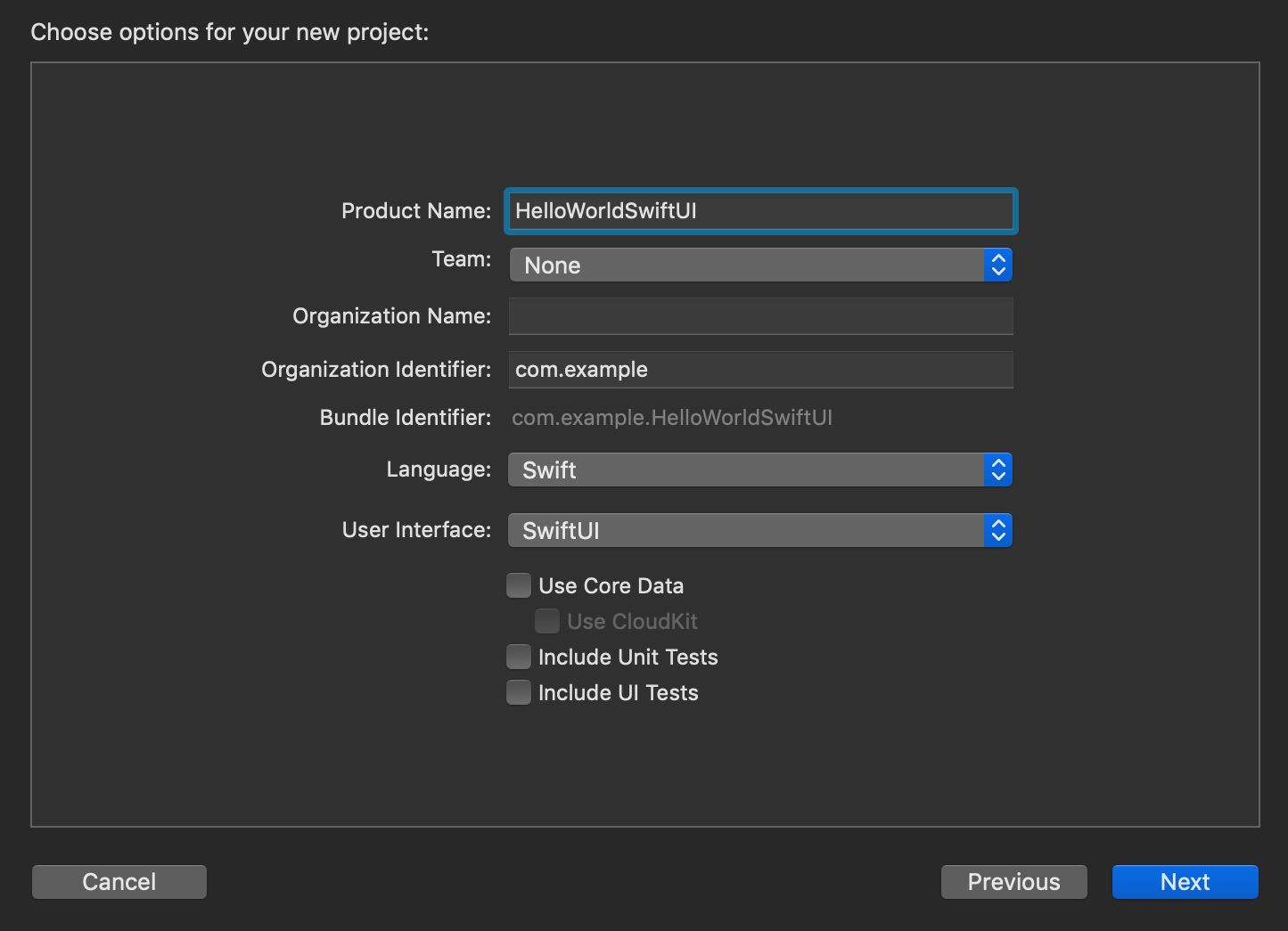
プロジェクトを作成すると、以下の3つの Swift のソースコードが用意される。
- AppDelegate.swift
- SceneDelegate.swift
- ContentView.swift
今回はこの内の ContentView.swift を修正して Hello World を実現する。
Hello World を書いてみる

import SwiftUI
// SwiftUI の View プロトコルを実装する
struct ContentView: View {
// コンテンツと動作を記述するプロパティ
var body: some View {
Text("Hello World")
}
}
View プロトコルを実装するコードでカスタムなビューを作成できる。
body プロパティに表示内容と動作を記述する。
View - SwiftUI | Apple Developer Documentation
You create custom views by declaring types that conform to the View protocol. Implement the required body computed property to provide the content and behavior for your custom view.
body - View | Apple Developer Documentation
The content and behavior of the view.
地図を表示してみる
もう少しコンテンツを増やしたサンプルとして地図表示機能を作ってみる。
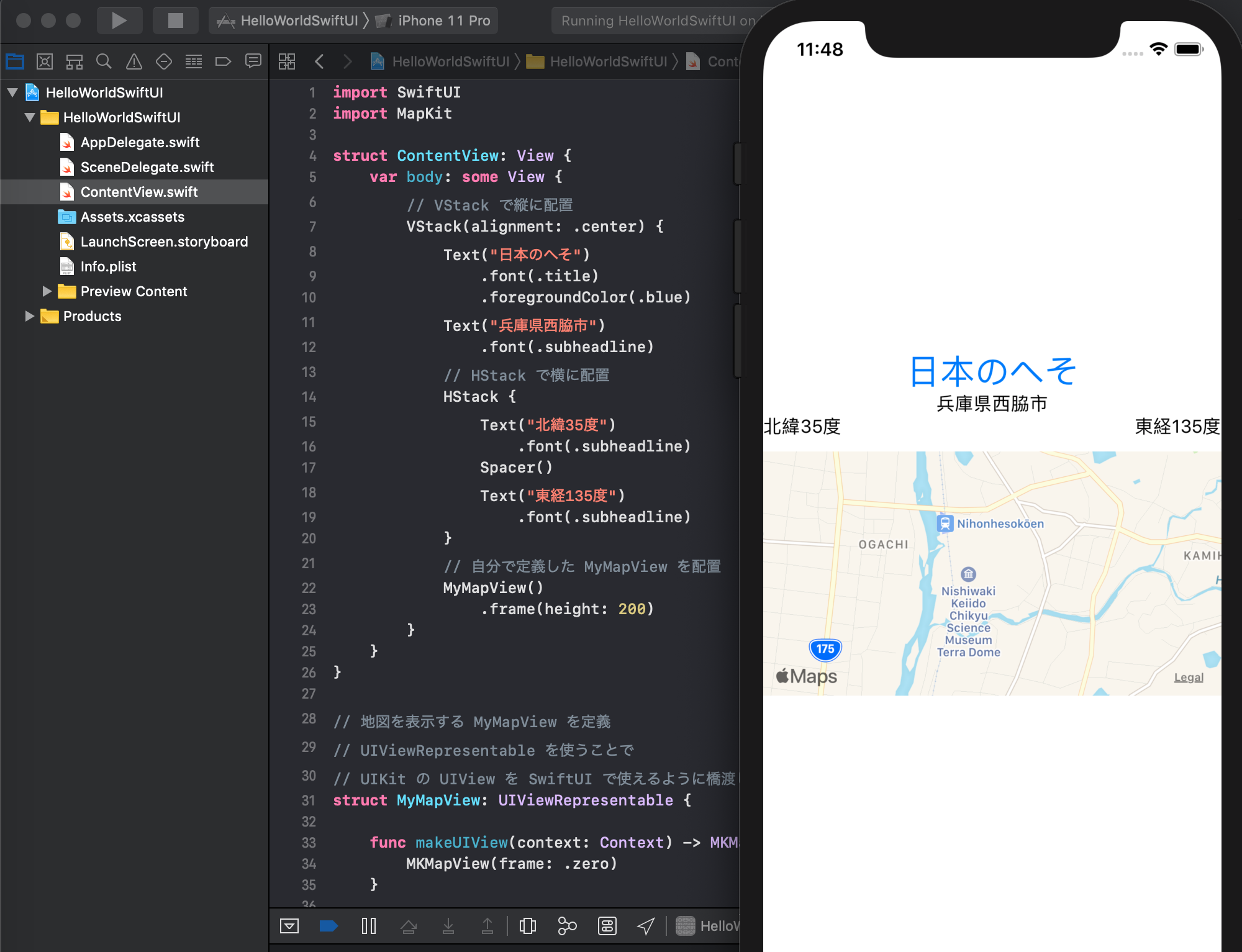
import SwiftUI
import MapKit
struct ContentView: View {
var body: some View {
// VStack で縦に配置
VStack(alignment: .center) {
Text("日本のへそ")
.font(.title)
.foregroundColor(.blue)
Text("兵庫県西脇市")
.font(.subheadline)
// HStack で横に配置
HStack {
Text("北緯35度")
.font(.subheadline)
Spacer()
Text("東経135度")
.font(.subheadline)
}
// 自分で定義した MyMapView を配置
MyMapView()
.frame(height: 200)
}
}
}
// 地図を表示する MyMapView を定義
// UIViewRepresentable を使うことで
// UIKit の UIView を SwiftUI で使えるように橋渡しできる
struct MyMapView: UIViewRepresentable {
func makeUIView(context: Context) -> MKMapView {
MKMapView(frame: .zero)
}
func updateUIView(_ view: MKMapView, context: Context) {
let coordinate = CLLocationCoordinate2D(latitude: 35, longitude: 135)
let span = MKCoordinateSpan(latitudeDelta: 0.01, longitudeDelta: 0.01)
let region = MKCoordinateRegion(center: coordinate, span: span)
view.setRegion(region, animated: true)
}
}
ビューとコントロール
SwiftUI で配置できる基本的なビューとコントロールについては Views and Controls | Apple Developer Documentation に載っている。
Text、Image、Button、NavigationLink、MenuButton、PasteButton、Toggle、Picker、DatePicker、Slider、Stepper などがある。
SwiftUI のチュートリアルが用意されている
SwiftUI Tutorials | Apple Developer Documentation からいくつかのチュートリアルを実施できる。ソースコードと見た目を見ながら段階的にUIと機能を構築してくチュートリアルになっているのでわかりやすい。
直近のチュートリアルは以下のようになっている。
- Creating and Combining Views
- Building Lists and Navigation
- Handling User Input
- Drawing Paths and Shapes
- Animating Views and Transitions
- Composing Complex Interfaces
- Working with UI Controls
- Interfacing with UIKit
- Creating a watchOS App