はじめに
こちらの記事で紹介したツールの改良版です
前回から変更したポイント
- kintoneの全レコードが取得できるようにした
- 表を簡略化
初期設定
spreadsheetのコピー
spreadsheetをコピーして使ってください(link)
kintoneアプリ情報の設定
ユーザー情報の入力
- spreadsheetのスクリプトエディタを開いてください

- ファイルの「プロジェクトのプロパティ」を開いてください
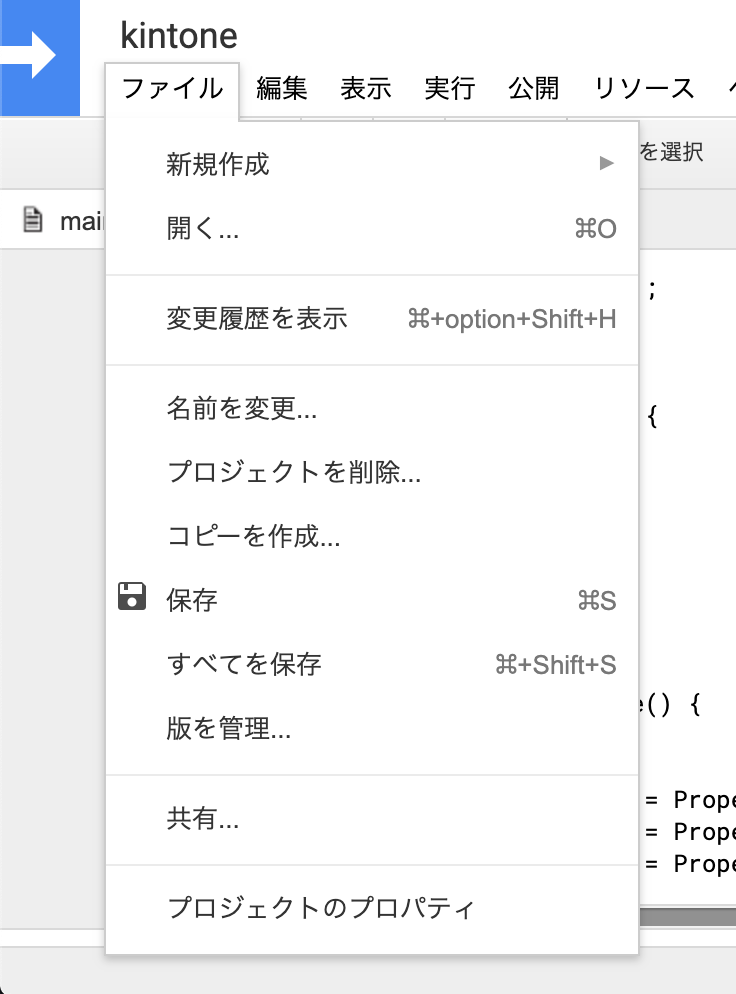
- 以下の情報を設定してください。
- subdomain - サイボウズのURLのサブドメイン(https://◯◯.cybozu.com/の◯◯の部分)
- user - ログインする時のユーザー名
- pass - ログインする時のパスワード
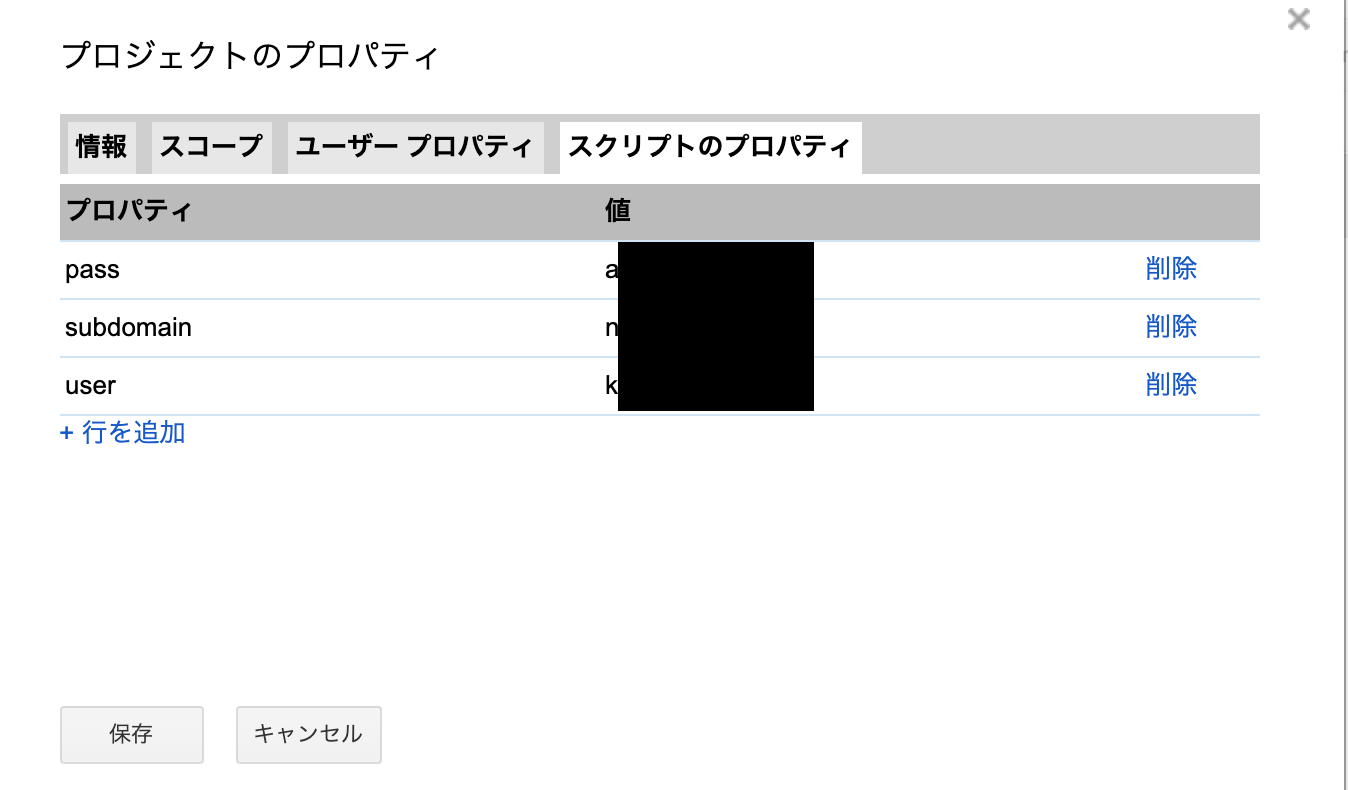
※ユーザー名、パスワードに使うアカウントは管理者権限が必要かも
アプリシートの設定
- シート名をアプリ名と同じにしてください
- 各列の見出しにはアプリのフィールドコードを設定してください(link)
実行
「概要」シートの猫の画像を押すと、処理が開始されます。
ソース
main.js
function getKintone(applicationName) {
let appList = getAppList();
appList = appList.filter(app => {
return !app.isIgnore() && !app.isDifferentName(applicationName);
});
const manager = getManeger(appList);
appList.forEach(app => {
app.setRecords(getAllRecords(manager, app.getIndex()));
app.refreshSheet();
});
}
sheet.js
var CONFIG_SHEET = {
name: 'config',
row: {
data: 2,
},
column: {
runFlag: 1,
appId: 2,
appName: 3,
}
};
function refreshSheet(sheetName, outList) {
const sheet = SpreadsheetApp.getActive().getSheetByName(sheetName);
const row = 2;
const column = 1;
sheet.getRange(row, column, sheet.getLastRow(), outList[0].length).clearContent();
sheet.getRange(row, column, outList.length, outList[0].length).setValues(outList);
}
function getSheetKeyList(sheetName){
const sheet = SpreadsheetApp.getActive().getSheetByName(sheetName);
return sheet.getRange('1:1').getValues()[0].filter(Boolean);
}
function getAppList(){
const sheet = SpreadsheetApp.getActive().getSheetByName(CONFIG_SHEET.name);
let data = sheet.getDataRange().getValues();
data.shift();
return data.map((row, index) => new App(row, index));
}
class/App.js
class App{
constructor(row, index){
this.index = index;
this.runFlag = row[CONFIG_SHEET.column.runFlag - 1];
this.appId = row[CONFIG_SHEET.column.appId - 1];
this.appName = row[CONFIG_SHEET.column.appName - 1];
this.records = undefined;
}
isIgnore(){
return !this.runFlag;
}
isDifferentName(name){
const isString = object => typeof object === 'string' || object instanceof String;
return isString(name) && this.appName !== name;
}
getKintoneAppData(){
return {
'appid' : this.appId,
'name' : this.appName,
};
}
getIndex(){
return this.index;
}
getSheetName(){
return this.appName;
}
setRecords(records){
this.records = records;
}
refreshSheet(){
const outList = this.getOutList();
refreshSheet(this.getSheetName(), outList);
}
getOutList(){
const keyList = getSheetKeyList(this.getSheetName());
if(this.records === undefined) return [['取得エラー']];
return this.records.map(record => {
return keyList.map(key => {
const value = record[key].value;
if (!Array.isArray(value)) return value;
if(value[0] instanceof Object){
return value.map(obj => JSON.stringify(obj)).join('|');
};
return value.join();
});
});
}
}
kintone.js
function getManeger(appList){
const subdomain = PropertiesService.getScriptProperties().getProperty('subdomain');
const user = PropertiesService.getScriptProperties().getProperty('user');
const pass = PropertiesService.getScriptProperties().getProperty('pass');
const apps = appList.reduce((apps, app) => {
apps[app.getIndex()] = app.getKintoneAppData();
return apps;
}, {});
return new KintoneManager.KintoneManager(subdomain, apps, user, pass);
}
function getAllRecords(manager, appName, offset = 0, limit = 500, optRecords) {
let allRecords = optRecords || [];
const query = `order by $id desc limit ${limit} offset ${offset}`;
if (offset > 10000) {
Logger.log('offsetが10000を超えたので取得を終了します。');
return allRecords;
}
let response = manager.search(appName, query);
let records = JSON.parse(response.getContentText()).records;
allRecords = allRecords.concat(records);
if (records.length === limit) {
return getAllRecords(manager, appName, offset + limit, limit, allRecords);
}
return allRecords;
}