Androidアプリでカメラ、ローカルから画像を表示する方法
シンプルにファイル or カメラのどちらかを選択し画像を表示してみます
イメージサンプル
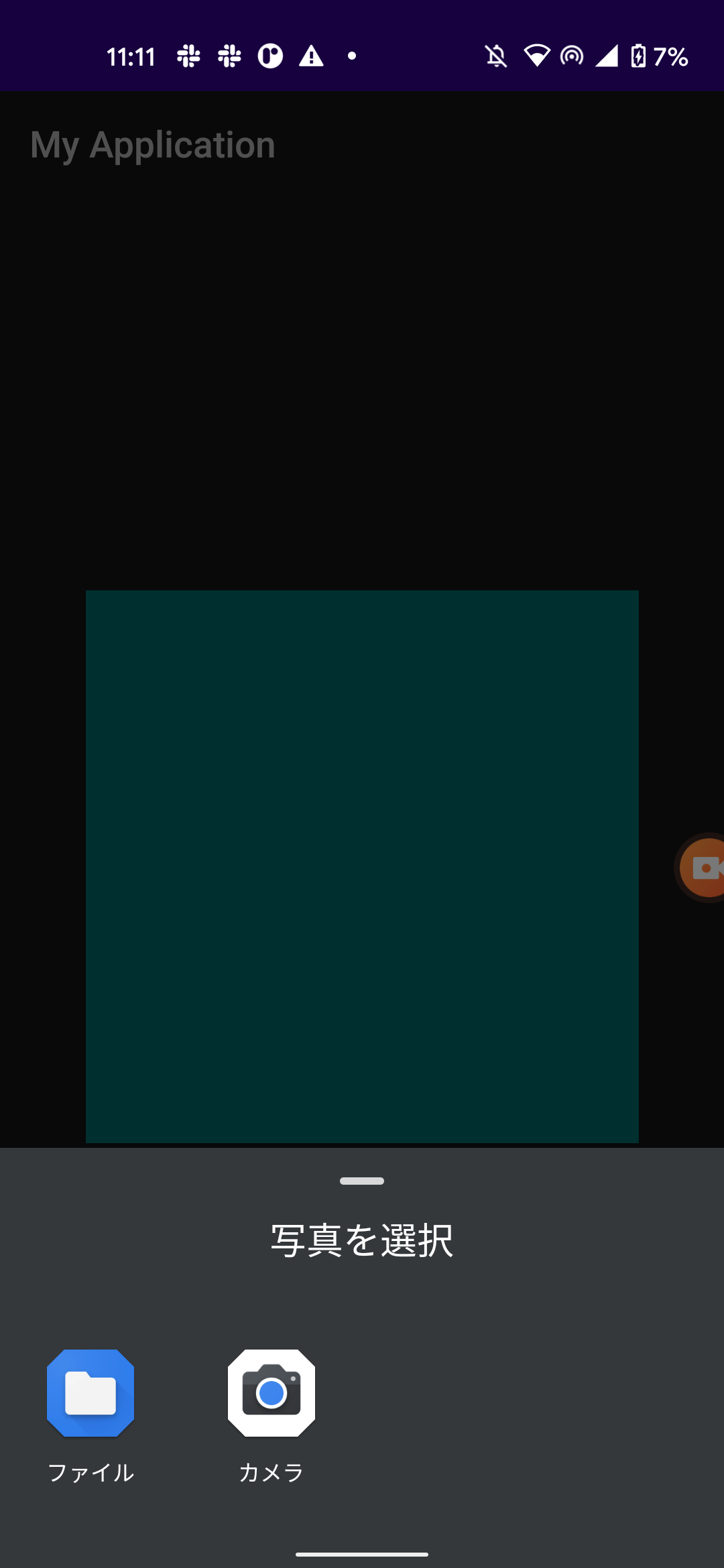
- カメラを機能を有効にする
<manifest>
<uses-feature android:name="android.hardware.camera"
android:required="true" />
</manifest>
activity_main.xml
<?xml version="1.0" encoding="utf-8"?>
<androidx.constraintlayout.widget.ConstraintLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:app="http://schemas.android.com/apk/res-auto"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
tools:context=".MainActivity">
<ImageView
android:id="@+id/upload_image"
android:layout_width="300dp"
android:layout_height="300dp"
android:background="@color/teal_700"
app:layout_constraintBottom_toBottomOf="parent"
app:layout_constraintLeft_toLeftOf="parent"
app:layout_constraintRight_toRightOf="parent"
app:layout_constraintTop_toTopOf="parent" />
</androidx.constraintlayout.widget.ConstraintLayout>
MainActivity.kt
package com.example.myapplication
import android.content.Intent
import android.graphics.Bitmap
import android.graphics.BitmapFactory
import android.os.Bundle
import android.provider.MediaStore
import android.widget.ImageView
import android.widget.Toast
import androidx.appcompat.app.AppCompatActivity
class MainActivity : AppCompatActivity() {
override fun onCreate(savedInstanceState: Bundle?) {
super.onCreate(savedInstanceState)
setContentView(R.layout.activity_main)
val uploadImage = findViewById<ImageView>(R.id.upload_image)
uploadImage.setOnClickListener {
selectPhoto()
}
}
companion object {
private const val READ_REQUEST_CODE: Int = 42
}
override fun onActivityResult(requestCode: Int, resultCode: Int, data: Intent?) {
super.onActivityResult(requestCode, resultCode, data)
val uploadImage = findViewById<ImageView>(R.id.upload_image)
if (resultCode != AppCompatActivity.RESULT_OK) {
return
}
when (requestCode) {
READ_REQUEST_CODE -> {
try {
data?.data?.also { uri ->
val inputStream = contentResolver?.openInputStream(uri)
val image = BitmapFactory.decodeStream(inputStream)
uploadImage.setImageBitmap(image)
}
} catch (e: Exception) {
Toast.makeText(this, "READ_REQUEST_CODEのエラーが発生しました", Toast.LENGTH_LONG).show()
}
}
}
}
private fun selectPhoto() {
val documentIntent = Intent(Intent.ACTION_OPEN_DOCUMENT).apply {
addCategory(Intent.CATEGORY_OPENABLE)
type = "image/*"
}
val cameraIntent = Intent(MediaStore.ACTION_IMAGE_CAPTURE).also { takePictureIntent ->
}
val target: List<Intent> = listOf(documentIntent,cameraIntent)
val intent = Intent(Intent.ACTION_VIEW)
if(intent.resolveActivity(getPackageManager()) != null) {
val chooser = Intent.createChooser(documentIntent,"写真を選択").apply {
putExtra(Intent.EXTRA_INITIAL_INTENTS , target.toTypedArray())
}
startActivityForResult(chooser, READ_REQUEST_CODE)
}
}
}