#Flutter の環境構築
今回はMAC + Visual Studio Codeで開発を行います。
こちら「公式ドキュメント」を参考に、必要なライブラリやアプリケーションをインストールしていきます。
インストールしたものはこれら。
- Flutter SDK
- Android Studio + Flutter plugin
- Visual Studio Code + Flutter, Dart 拡張機能
flutter doctor
で問題なさそうならアプリを作っていきます。
Visual Studio Codeでアプリを作る
コマンドパレット(⌘ + Shift + P)でFlutter: New Project
を実行。
プロジェクト名、保存先を決めて実行するだけでアプリが起動します。
main.dart
import 'package:flutter/material.dart';
void main() => runApp(MyApp());
class MyApp extends StatelessWidget {
// This widget is the root of your application.
@override
Widget build(BuildContext context) {
return MaterialApp(
title: 'Flutter Demo',
theme: ThemeData(
primarySwatch: Colors.blue,
),
home: MyHomePage(title: 'Flutter Demo Home Page'),
);
}
}
class MyHomePage extends StatefulWidget {
MyHomePage({Key key, this.title}) : super(key: key);
final String title;
@override
_MyHomePageState createState() => _MyHomePageState();
}
class _MyHomePageState extends State<MyHomePage> {
int _counter = 0;
void _incrementCounter() {
setState(() {
_counter++;
});
}
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(
// Here we take the value from the MyHomePage object that was created by
// the App.build method, and use it to set our appbar title.
title: Text(widget.title),
),
body: Center(
child: Column(
mainAxisAlignment: MainAxisAlignment.center,
children: <Widget>[
Text(
'You have pushed the button this many times:',
),
Text(
'$_counter',
style: Theme.of(context).textTheme.display1,
),
],
),
),
floatingActionButton: FloatingActionButton(
onPressed: _incrementCounter,
tooltip: 'Increment',
child: Icon(Icons.add),
), // This trailing comma makes auto-formatting nicer for build methods.
);
}
}

アプリっぽくするために、タブメニューを追加してみます。
_MyHomePageState
にbottomNavigationBar
の記述を追加。
デフォルトのアイコンはこちらから選べます。
_MyHomePageState
class _MyHomePageState extends State<MyHomePage> {
int _counter = 0;
void _incrementCounter() {
setState(() {
_counter++;
});
}
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(
// Here we take the value from the MyHomePage object that was created by
// the App.build method, and use it to set our appbar title.
title: Text(widget.title),
),
body: Center(
child: Column(
mainAxisAlignment: MainAxisAlignment.center,
children: <Widget>[
Text(
'You have pushed the button this many times:',
),
Text(
'$_counter',
style: Theme.of(context).textTheme.display1,
),
],
),
),
floatingActionButton: FloatingActionButton(
onPressed: _incrementCounter,
tooltip: 'Increment',
child: Icon(Icons.add),
), // This trailing comma makes auto-formatting nicer for build methods.
bottomNavigationBar: BottomNavigationBar(
items: const [
BottomNavigationBarItem(
icon: Icon(Icons.home),
title: Text('Home'),
),
BottomNavigationBarItem(
icon: Icon(Icons.textsms),
title: Text('Information'),
),
BottomNavigationBarItem(
icon: Icon(Icons.settings),
title: Text('Setting'),
),
],
),
);
}
}
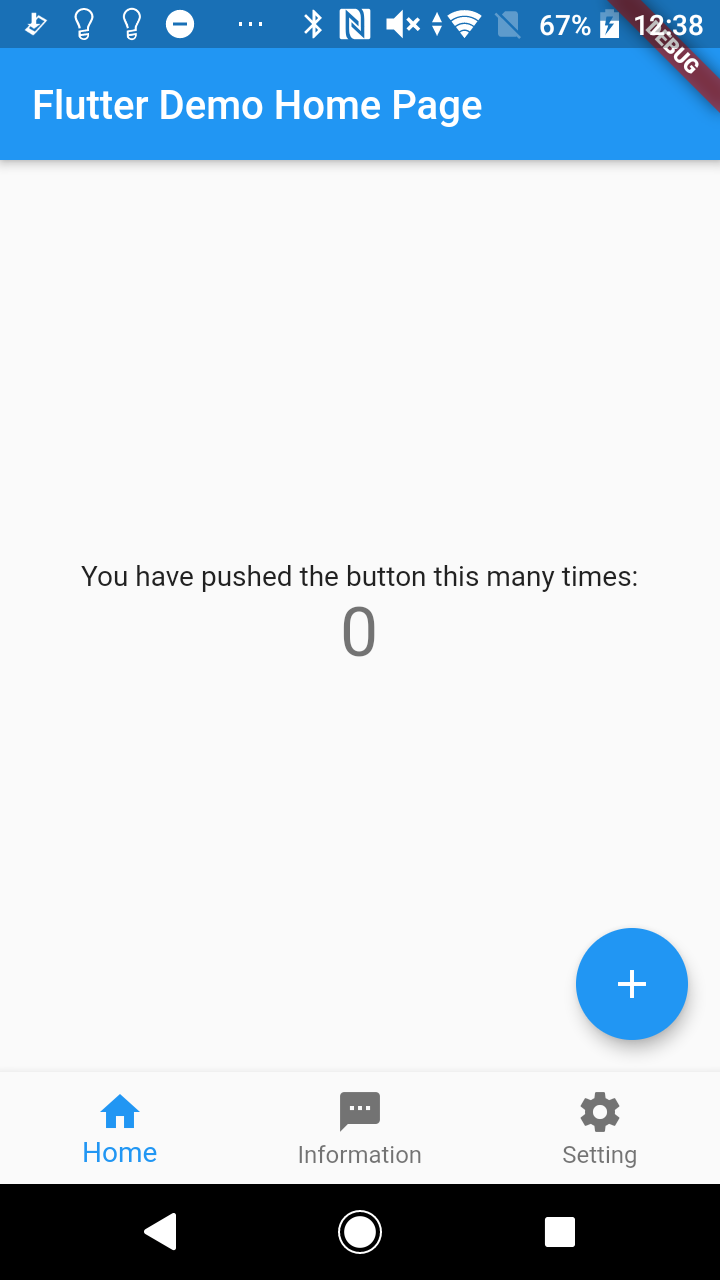
画面遷移はしませんが、それは次回。
#まとめ + 課題
作るのは簡単そう。
Hot Reload が超便利。
iOSでの動作はどうかな。
処理は簡単に書けるのかな。
OS固有の動作は?