この記事では次の内容について説明します。
-
@AppStorage
を使用して、SwiftUI アプリのUserDefaults
を置き換える
2.@SceneStorage
を使用して、1つのプログラムシーン(ウィンドウ)に固有の情報を保存する -
SwiftUI
のApp Delegate
機能を実装する
@AppStorage
@AppStorage(wrappedValue: "Tokyo", "currentCity")
var currentCity
このコードは変数 currentCity
を UserDefaults
に保存された値にリンクする
UserDefaults
は currentCity
の値を求めようとし、もし求められれば、変数 currentCity
は求められた値に相当する。
User Defaults
がそれを求められなければ、デフォルトで wrappedValue
を使用する。
変数 currentCity
に変更が加えられた場合、その変更は保存される。
この SwiftUI
プログラムによりテストが可能である:
struct ContentView: View {
@AppStorage(wrappedValue: "Tokyo", "currentCity")
var currentCity
var body: some View {
Text(currentCity).padding()
TextField("", text: $currentCity)
}
}
@SceneStorage
@SceneStorage
は @AppStorage
とは違い、プログラムのシーンの状態を保存するために使います。 SceneStorage
に保存されたデータは一つのシーン内にのみ在ります。(現在iOSは一つのアプリケーションで複数のシーンを持てるようになっているためです。)
struct textTabView: View {
@SceneStorage("postContent")
var postContent = "This is a draft..."
var body: some View {
TextEditor(text: $postContent)
}
}
SwiftUI App アプリ用App Delegate
今年のWWDC 2020で、AppleはSwiftUIアプリを発表しました。SwiftUI appでは、App DelegateファイルとScene Delegateファイルがありません。このセクションでは、App DelegateのアップデートをSwiftUIアプリで受け取る方法をご説明します。
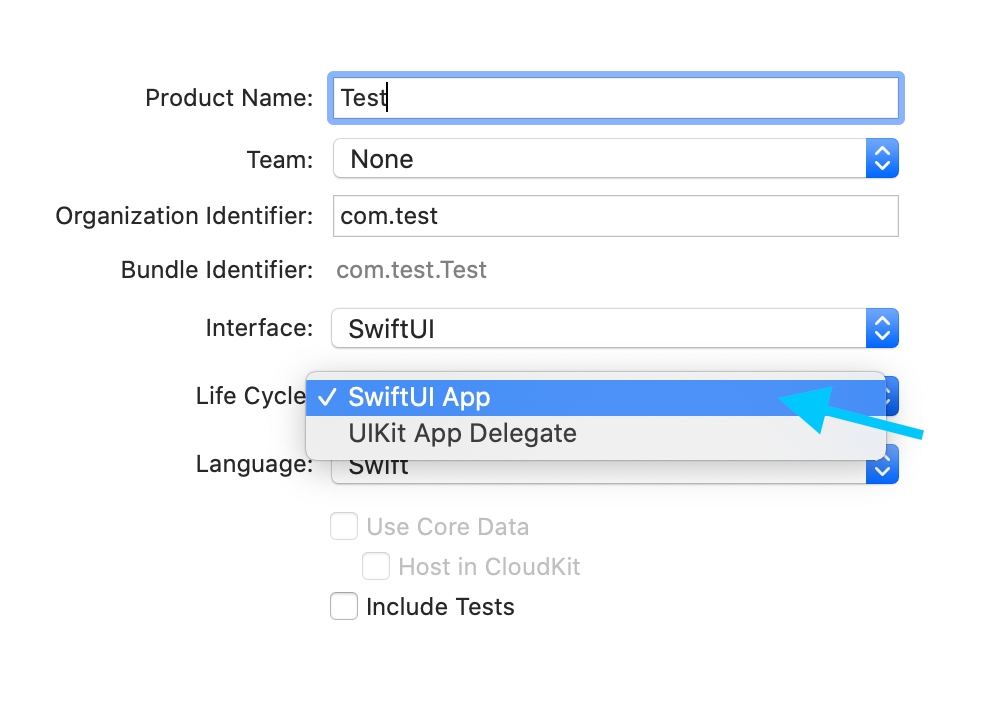
バックグラウンド (background)、アクティブ (active)、 インアクティブ (inactive) 状態
scenePhase
という環境変数を探し、その変数が変更された場合に通知されます。
struct ContentView: View {
@Environment(\.scenePhase) private var scenePhase
var body: some View {
Text("Hello, world!").padding()
.onChange(of: scenePhase) { phase in
switch phase {
case .active:
print("active")
case .inactive:
print("inactive")
case .background:
print("background")
@unknown default:
print("unknown")
}
}
}
}
ユニバーサルリンク / URLスキーム
URLスキームは、アプリを起動するために設定できるアプリ固有のURLです(app://)
ユニバーサルリンクは、ウェブサイトへアクセスした時にモバイルアプリを起動できます。
これらのイベントを処理するには、.onOpenURL
を使用します
@main
struct exploreSwiftUIApp: App {
var body: some Scene {
WindowGroup {
ContentView()
.onOpenURL { (url) in
print("Universal Link: \(url)")
}
}
}
}
App Delegateファンクションを使用する
古い AppDelegate.swift ファンクションを使用したい場合は、アダプターを追加することができます。
@main
struct exploreSwiftUIApp: App {
@UIApplicationDelegateAdaptor var delegate: AppDelegate
var body: some Scene {
WindowGroup {
ContentView()
}
}
}
class AppDelegate: NSObject, UIApplicationDelegate {
func application(_ application: UIApplication, didFinishLaunchingWithOptions launchOptions: [UIApplication.LaunchOptionsKey : Any]? = nil) -> Bool {
print("Did finish launch")
return true
}
}
他の種類の通知
以下を使って、SwiftUIで他の種類の通知を受け取ることができます
Text("Enter some text here")
.onReceive(NotificationCenter.default.publisher(for: UIApplication.keyboardDidShowNotification)) { (output) in
print("Keyboard is shown")
}