ここでは、PyQt5_Examplesのコードを紹介していきます。
版権はRiverbankなのでそこはご認識ください。
StyleSheetをまずは使ってみる
from PyQt5.QtWidgets import *
from PyQt5.QtGui import *
from PyQt5.Qt import *
import sys
from ui_mainwindow import Ui_MainWindow
class StyleTest(QMainWindow):
def __init__(self, parent=None):
super(StyleTest, self).__init__(parent)
self.ui = Ui_MainWindow()
self.ui.setupUi(self)
self.loadStyleSheet('coffee')
def loadStyleSheet(self, sheetName):
file = QFile('%s.qss' % sheetName.lower())
file.open(QFile.ReadOnly)
styleSheet = file.readAll()
styleSheet = str(styleSheet, encoding='utf8')
print(styleSheet)
QApplication.instance().setStyleSheet(styleSheet)
if __name__ == '__main__':
app = QApplication(sys.argv)
button = StyleTest()
button.show()
sys.exit(app.exec_())
# -*- coding: utf-8 -*-
# Form implementation generated from reading ui file 'mainwindow.ui'
#
# Created: Fri Jul 26 06:49:55 2013
# by: PyQt5 UI code generator 5.0.1-snapshot-2a99e59669ee
#
# WARNING! All changes made in this file will be lost!
from PyQt5 import QtCore, QtGui, QtWidgets
class Ui_MainWindow(object):
def setupUi(self, MainWindow):
MainWindow.setObjectName("MainWindow")
MainWindow.resize(400, 413)
self.centralwidget = QtWidgets.QWidget(MainWindow)
self.centralwidget.setObjectName("centralwidget")
self.vboxlayout = QtWidgets.QVBoxLayout(self.centralwidget)
self.vboxlayout.setContentsMargins(9, 9, 9, 9)
self.vboxlayout.setSpacing(6)
self.vboxlayout.setObjectName("vboxlayout")
self.mainFrame = QtWidgets.QFrame(self.centralwidget)
self.mainFrame.setFrameShape(QtWidgets.QFrame.StyledPanel)
self.mainFrame.setFrameShadow(QtWidgets.QFrame.Raised)
self.mainFrame.setObjectName("mainFrame")
self.gridlayout = QtWidgets.QGridLayout(self.mainFrame)
self.gridlayout.setContentsMargins(9, 9, 9, 9)
self.gridlayout.setSpacing(6)
self.gridlayout.setObjectName("gridlayout")
self.agreeCheckBox = QtWidgets.QCheckBox(self.mainFrame)
self.agreeCheckBox.setObjectName("agreeCheckBox")
self.gridlayout.addWidget(self.agreeCheckBox, 6, 0, 1, 5)
self.label = QtWidgets.QLabel(self.mainFrame)
self.label.setAlignment(QtCore.Qt.AlignRight|QtCore.Qt.AlignTop|QtCore.Qt.AlignTrailing)
self.label.setObjectName("label")
self.gridlayout.addWidget(self.label, 5, 0, 1, 1)
self.nameLabel = QtWidgets.QLabel(self.mainFrame)
self.nameLabel.setAlignment(QtCore.Qt.AlignRight|QtCore.Qt.AlignTrailing|QtCore.Qt.AlignVCenter)
self.nameLabel.setObjectName("nameLabel")
self.gridlayout.addWidget(self.nameLabel, 0, 0, 1, 1)
self.maleRadioButton = QtWidgets.QRadioButton(self.mainFrame)
self.maleRadioButton.setObjectName("maleRadioButton")
self.gridlayout.addWidget(self.maleRadioButton, 1, 1, 1, 1)
self.passwordLabel = QtWidgets.QLabel(self.mainFrame)
self.passwordLabel.setAlignment(QtCore.Qt.AlignRight|QtCore.Qt.AlignTrailing|QtCore.Qt.AlignVCenter)
self.passwordLabel.setObjectName("passwordLabel")
self.gridlayout.addWidget(self.passwordLabel, 3, 0, 1, 1)
self.countryCombo = QtWidgets.QComboBox(self.mainFrame)
self.countryCombo.setObjectName("countryCombo")
self.countryCombo.addItem("")
self.countryCombo.addItem("")
self.countryCombo.addItem("")
self.countryCombo.addItem("")
self.countryCombo.addItem("")
self.countryCombo.addItem("")
self.countryCombo.addItem("")
self.gridlayout.addWidget(self.countryCombo, 4, 1, 1, 4)
self.ageLabel = QtWidgets.QLabel(self.mainFrame)
self.ageLabel.setAlignment(QtCore.Qt.AlignRight|QtCore.Qt.AlignTrailing|QtCore.Qt.AlignVCenter)
self.ageLabel.setObjectName("ageLabel")
self.gridlayout.addWidget(self.ageLabel, 2, 0, 1, 1)
self.countryLabel = QtWidgets.QLabel(self.mainFrame)
self.countryLabel.setAlignment(QtCore.Qt.AlignRight|QtCore.Qt.AlignTrailing|QtCore.Qt.AlignVCenter)
self.countryLabel.setObjectName("countryLabel")
self.gridlayout.addWidget(self.countryLabel, 4, 0, 1, 1)
self.genderLabel = QtWidgets.QLabel(self.mainFrame)
self.genderLabel.setAlignment(QtCore.Qt.AlignRight|QtCore.Qt.AlignTrailing|QtCore.Qt.AlignVCenter)
self.genderLabel.setObjectName("genderLabel")
self.gridlayout.addWidget(self.genderLabel, 1, 0, 1, 1)
self.passwordEdit = QtWidgets.QLineEdit(self.mainFrame)
self.passwordEdit.setEchoMode(QtWidgets.QLineEdit.Password)
self.passwordEdit.setObjectName("passwordEdit")
self.gridlayout.addWidget(self.passwordEdit, 3, 1, 1, 4)
self.femaleRadioButton = QtWidgets.QRadioButton(self.mainFrame)
self.femaleRadioButton.setObjectName("femaleRadioButton")
self.gridlayout.addWidget(self.femaleRadioButton, 1, 2, 1, 2)
self.ageSpinBox = QtWidgets.QSpinBox(self.mainFrame)
self.ageSpinBox.setMinimum(12)
self.ageSpinBox.setProperty("value", 22)
self.ageSpinBox.setObjectName("ageSpinBox")
self.gridlayout.addWidget(self.ageSpinBox, 2, 1, 1, 2)
self.nameCombo = QtWidgets.QComboBox(self.mainFrame)
self.nameCombo.setEditable(True)
self.nameCombo.setObjectName("nameCombo")
self.gridlayout.addWidget(self.nameCombo, 0, 1, 1, 4)
spacerItem = QtWidgets.QSpacerItem(40, 20, QtWidgets.QSizePolicy.Expanding, QtWidgets.QSizePolicy.Minimum)
self.gridlayout.addItem(spacerItem, 1, 4, 1, 1)
spacerItem1 = QtWidgets.QSpacerItem(61, 20, QtWidgets.QSizePolicy.Expanding, QtWidgets.QSizePolicy.Minimum)
self.gridlayout.addItem(spacerItem1, 2, 3, 1, 2)
self.buttonBox = QtWidgets.QDialogButtonBox(self.mainFrame)
self.buttonBox.setOrientation(QtCore.Qt.Horizontal)
self.buttonBox.setStandardButtons(QtWidgets.QDialogButtonBox.Cancel|QtWidgets.QDialogButtonBox.NoButton|QtWidgets.QDialogButtonBox.Ok)
self.buttonBox.setObjectName("buttonBox")
self.gridlayout.addWidget(self.buttonBox, 7, 3, 1, 2)
self.professionList = QtWidgets.QListWidget(self.mainFrame)
self.professionList.setObjectName("professionList")
item = QtWidgets.QListWidgetItem()
self.professionList.addItem(item)
item = QtWidgets.QListWidgetItem()
self.professionList.addItem(item)
item = QtWidgets.QListWidgetItem()
self.professionList.addItem(item)
self.gridlayout.addWidget(self.professionList, 5, 1, 1, 4)
self.vboxlayout.addWidget(self.mainFrame)
MainWindow.setCentralWidget(self.centralwidget)
self.menubar = QtWidgets.QMenuBar(MainWindow)
self.menubar.setGeometry(QtCore.QRect(0, 0, 400, 29))
self.menubar.setObjectName("menubar")
self.menu_File = QtWidgets.QMenu(self.menubar)
self.menu_File.setObjectName("menu_File")
self.menu_Help = QtWidgets.QMenu(self.menubar)
self.menu_Help.setObjectName("menu_Help")
MainWindow.setMenuBar(self.menubar)
self.statusbar = QtWidgets.QStatusBar(MainWindow)
self.statusbar.setObjectName("statusbar")
MainWindow.setStatusBar(self.statusbar)
self.exitAction = QtWidgets.QAction(MainWindow)
self.exitAction.setObjectName("exitAction")
self.aboutQtAction = QtWidgets.QAction(MainWindow)
self.aboutQtAction.setObjectName("aboutQtAction")
self.editStyleAction = QtWidgets.QAction(MainWindow)
self.editStyleAction.setObjectName("editStyleAction")
self.aboutAction = QtWidgets.QAction(MainWindow)
self.aboutAction.setObjectName("aboutAction")
self.menu_File.addAction(self.editStyleAction)
self.menu_File.addSeparator()
self.menu_File.addAction(self.exitAction)
self.menu_Help.addAction(self.aboutAction)
self.menu_Help.addAction(self.aboutQtAction)
self.menubar.addAction(self.menu_File.menuAction())
self.menubar.addAction(self.menu_Help.menuAction())
self.label.setBuddy(self.professionList)
self.nameLabel.setBuddy(self.nameCombo)
self.passwordLabel.setBuddy(self.passwordEdit)
self.ageLabel.setBuddy(self.ageSpinBox)
self.countryLabel.setBuddy(self.countryCombo)
self.retranslateUi(MainWindow)
self.countryCombo.setCurrentIndex(6)
self.professionList.setCurrentRow(0)
QtCore.QMetaObject.connectSlotsByName(MainWindow)
def retranslateUi(self, MainWindow):
_translate = QtCore.QCoreApplication.translate
MainWindow.setWindowTitle(_translate("MainWindow", "Style Sheet"))
self.agreeCheckBox.setToolTip(_translate("MainWindow", "Please read the LICENSE file before checking"))
self.agreeCheckBox.setText(_translate("MainWindow", "I accept the terms and &conditions"))
self.label.setText(_translate("MainWindow", "Profession:"))
self.nameLabel.setText(_translate("MainWindow", "&Name:"))
self.maleRadioButton.setToolTip(_translate("MainWindow", "Check this if you are male"))
self.maleRadioButton.setText(_translate("MainWindow", "&Male"))
self.passwordLabel.setText(_translate("MainWindow", "&Password:"))
self.countryCombo.setToolTip(_translate("MainWindow", "Specify country of origin"))
self.countryCombo.setStatusTip(_translate("MainWindow", "Specify country of origin"))
self.countryCombo.setItemText(0, _translate("MainWindow", "Egypt"))
self.countryCombo.setItemText(1, _translate("MainWindow", "France"))
self.countryCombo.setItemText(2, _translate("MainWindow", "Germany"))
self.countryCombo.setItemText(3, _translate("MainWindow", "India"))
self.countryCombo.setItemText(4, _translate("MainWindow", "Italy"))
self.countryCombo.setItemText(5, _translate("MainWindow", "Norway"))
self.countryCombo.setItemText(6, _translate("MainWindow", "Pakistan"))
self.ageLabel.setText(_translate("MainWindow", "&Age:"))
self.countryLabel.setText(_translate("MainWindow", "Country:"))
self.genderLabel.setText(_translate("MainWindow", "Gender:"))
self.passwordEdit.setToolTip(_translate("MainWindow", "Specify your password"))
self.passwordEdit.setStatusTip(_translate("MainWindow", "Specify your password"))
self.passwordEdit.setText(_translate("MainWindow", "Password"))
self.femaleRadioButton.setToolTip(_translate("MainWindow", "Check this if you are female"))
self.femaleRadioButton.setText(_translate("MainWindow", "&Female"))
self.ageSpinBox.setToolTip(_translate("MainWindow", "Specify your age"))
self.ageSpinBox.setStatusTip(_translate("MainWindow", "Specify your age"))
self.nameCombo.setToolTip(_translate("MainWindow", "Specify your name"))
self.professionList.setToolTip(_translate("MainWindow", "Select your profession"))
self.professionList.setStatusTip(_translate("MainWindow", "Specify your name here"))
self.professionList.setWhatsThis(_translate("MainWindow", "Specify your name here"))
__sortingEnabled = self.professionList.isSortingEnabled()
self.professionList.setSortingEnabled(False)
item = self.professionList.item(0)
item.setText(_translate("MainWindow", "Developer"))
item = self.professionList.item(1)
item.setText(_translate("MainWindow", "Student"))
item = self.professionList.item(2)
item.setText(_translate("MainWindow", "Fisherman"))
self.professionList.setSortingEnabled(__sortingEnabled)
self.menu_File.setTitle(_translate("MainWindow", "&File"))
self.menu_Help.setTitle(_translate("MainWindow", "&Help"))
self.exitAction.setText(_translate("MainWindow", "&Exit"))
self.aboutQtAction.setText(_translate("MainWindow", "About Qt"))
self.editStyleAction.setText(_translate("MainWindow", "Edit &Style..."))
self.aboutAction.setText(_translate("MainWindow", "About"))
.QWidget {
background-color: beige;
}
/* Nice Windows-XP-style password character. */
QLineEdit[echoMode="2"] {
lineedit-password-character: 9679;
}
/* We provide a min-width and min-height for push buttons
so that they look elegant regardless of the width of the text. */
QPushButton {
background-color: palegoldenrod;
border-width: 2px;
border-color: darkkhaki;
border-style: solid;
border-radius: 5;
padding: 3px;
min-width: 9ex;
min-height: 2.5ex;
}
QPushButton:hover {
background-color: khaki;
}
/* Increase the padding, so the text is shifted when the button is
pressed. */
QPushButton:pressed {
padding-left: 5px;
padding-top: 5px;
background-color: #d0d67c;
}
QLabel, QAbstractButton {
font: bold;
}
/* Mark mandatory fields with a brownish color. */
.mandatory {
color: brown;
}
/* Bold text on status bar looks awful. */
QStatusBar QLabel {
font: normal;
}
QStatusBar::item {
border-width: 1;
border-color: darkkhaki;
border-style: solid;
border-radius: 2;
}
QComboBox, QLineEdit, QSpinBox, QTextEdit, QListView {
background-color: cornsilk;
selection-color: #0a214c;
selection-background-color: #C19A6B;
}
QListView {
show-decoration-selected: 1;
}
QListView::item:hover {
background-color: wheat;
}
/* We reserve 1 pixel space in padding. When we get the focus,
we kill the padding and enlarge the border. This makes the items
glow. */
QLineEdit, QFrame {
border-width: 2px;
padding: 1px;
border-style: solid;
border-color: darkkhaki;
border-radius: 5px;
}
/* As mentioned above, eliminate the padding and increase the border. */
QLineEdit:focus, QFrame:focus {
border-width: 3px;
padding: 0px;
}
/* A QLabel is a QFrame ... */
QLabel {
border: none;
padding: 0;
background: none;
}
/* A QToolTip is a QLabel ... */
QToolTip {
border: 2px solid darkkhaki;
padding: 5px;
border-radius: 3px;
opacity: 200;
}
/* Nice to have the background color change when hovered. */
QRadioButton:hover, QCheckBox:hover {
background-color: wheat;
}
/* Force the dialog's buttons to follow the Windows guidelines. */
QDialogButtonBox {
button-layout: 0;
}
これらを全て同じディレクトリに置いてください。
uiに対してStylesheetが適用できます。

svgviewer.pyはそのままでは動かない。
PyQt5のexamplesにpaintingsそしてsvgviewerの下にsvgviewer.pyがあります。
これはPyQt5とPython3の環境では動きません。
L280からの以下の部分
def wheelEvent(self, event):
factor = pow(1.2, event.delta() / 240.0)
self.scale(factor, factor)
event.accept()
これを、以下のようにしないと動きません。
def wheelEvent(self, event):
factor = pow(1.2, event.angleDelta().y() / 240.0)
self.scale(factor, factor)
event.accept()
delta()→angleDelta()のちがいとtypeの違いで動きません。
これもQtのバージョンの違いから起こるものと思います。
ただこのコードはmouseのwheelEventで拡大縮小を実現している大事なコードなので展開します。
以下のコードでsvgviewer.pyを置き換えてください。
# !/usr/bin/env python
#############################################################################
##
## Copyright (C) 2013 Riverbank Computing Limited.
## Copyright (C) 2010 Nokia Corporation and/or its subsidiary(-ies).
## All rights reserved.
##
## This file is part of the examples of PyQt.
##
## $QT_BEGIN_LICENSE:BSD$
## You may use this file under the terms of the BSD license as follows:
##
## "Redistribution and use in source and binary forms, with or without
## modification, are permitted provided that the following conditions are
## met:
## * Redistributions of source code must retain the above copyright
## notice, this list of conditions and the following disclaimer.
## * Redistributions in binary form must reproduce the above copyright
## notice, this list of conditions and the following disclaimer in
## the documentation and/or other materials provided with the
## distribution.
## * Neither the name of Nokia Corporation and its Subsidiary(-ies) nor
## the names of its contributors may be used to endorse or promote
## products derived from this software without specific prior written
## permission.
##
## THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS
## "AS IS" AND ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT
## LIMITED TO, THE IMPLIED WARRANTIES OF MERCHANTABILITY AND FITNESS FOR
## A PARTICULAR PURPOSE ARE DISCLAIMED. IN NO EVENT SHALL THE COPYRIGHT
## OWNER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, INDIRECT, INCIDENTAL,
## SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT NOT
## LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE,
## DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY
## THEORY OF LIABILITY, WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT
## (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT OF THE USE
## OF THIS SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE."
## $QT_END_LICENSE$
##
#############################################################################
from PyQt5.QtCore import QFile, QSize, Qt
from PyQt5.QtGui import QBrush, QColor, QImage, QPainter, QPixmap, QPen
from PyQt5.QtWidgets import (QActionGroup, QApplication, QFileDialog,
QGraphicsItem, QGraphicsRectItem, QGraphicsScene, QGraphicsView,
QMainWindow, QMenu, QMessageBox, QWidget)
from PyQt5.QtOpenGL import QGL, QGLFormat, QGLWidget
from PyQt5.QtSvg import QGraphicsSvgItem
import svgviewer_rc
class MainWindow(QMainWindow):
def __init__(self):
super(MainWindow, self).__init__()
self.currentPath = ''
self.view = SvgView()
fileMenu = QMenu("&File", self)
openAction = fileMenu.addAction("&Open...")
openAction.setShortcut("Ctrl+O")
quitAction = fileMenu.addAction("E&xit")
quitAction.setShortcut("Ctrl+Q")
self.menuBar().addMenu(fileMenu)
viewMenu = QMenu("&View", self)
self.backgroundAction = viewMenu.addAction("&Background")
self.backgroundAction.setEnabled(False)
self.backgroundAction.setCheckable(True)
self.backgroundAction.setChecked(False)
self.backgroundAction.toggled.connect(self.view.setViewBackground)
self.outlineAction = viewMenu.addAction("&Outline")
self.outlineAction.setEnabled(False)
self.outlineAction.setCheckable(True)
self.outlineAction.setChecked(True)
self.outlineAction.toggled.connect(self.view.setViewOutline)
self.menuBar().addMenu(viewMenu)
rendererMenu = QMenu("&Renderer", self)
self.nativeAction = rendererMenu.addAction("&Native")
self.nativeAction.setCheckable(True)
self.nativeAction.setChecked(True)
if QGLFormat.hasOpenGL():
self.glAction = rendererMenu.addAction("&OpenGL")
self.glAction.setCheckable(True)
self.imageAction = rendererMenu.addAction("&Image")
self.imageAction.setCheckable(True)
if QGLFormat.hasOpenGL():
rendererMenu.addSeparator()
self.highQualityAntialiasingAction = rendererMenu.addAction("&High Quality Antialiasing")
self.highQualityAntialiasingAction.setEnabled(False)
self.highQualityAntialiasingAction.setCheckable(True)
self.highQualityAntialiasingAction.setChecked(False)
self.highQualityAntialiasingAction.toggled.connect(self.view.setHighQualityAntialiasing)
rendererGroup = QActionGroup(self)
rendererGroup.addAction(self.nativeAction)
if QGLFormat.hasOpenGL():
rendererGroup.addAction(self.glAction)
rendererGroup.addAction(self.imageAction)
self.menuBar().addMenu(rendererMenu)
openAction.triggered.connect(self.openFile)
quitAction.triggered.connect(QApplication.instance().quit)
rendererGroup.triggered.connect(self.setRenderer)
self.setCentralWidget(self.view)
self.setWindowTitle("SVG Viewer")
def openFile(self, path=None):
if not path:
path, _ = QFileDialog.getOpenFileName(self, "Open SVG File",
self.currentPath, "SVG files (*.svg *.svgz *.svg.gz)")
if path:
svg_file = QFile(path)
if not svg_file.exists():
QMessageBox.critical(self, "Open SVG File",
"Could not open file '%s'." % path)
self.outlineAction.setEnabled(False)
self.backgroundAction.setEnabled(False)
return
self.view.openFile(svg_file)
if not path.startswith(':/'):
self.currentPath = path
self.setWindowTitle("%s - SVGViewer" % self.currentPath)
self.outlineAction.setEnabled(True)
self.backgroundAction.setEnabled(True)
self.resize(self.view.sizeHint() + QSize(80, 80 + self.menuBar().height()))
def setRenderer(self, action):
if QGLFormat.hasOpenGL():
self.highQualityAntialiasingAction.setEnabled(False)
if action == self.nativeAction:
self.view.setRenderer(SvgView.Native)
elif action == self.glAction:
if QGLFormat.hasOpenGL():
self.highQualityAntialiasingAction.setEnabled(True)
self.view.setRenderer(SvgView.OpenGL)
elif action == self.imageAction:
self.view.setRenderer(SvgView.Image)
class SvgView(QGraphicsView):
Native, OpenGL, Image = range(3)
def __init__(self, parent=None):
super(SvgView, self).__init__(parent)
self.renderer = SvgView.Native
self.svgItem = None
self.backgroundItem = None
self.outlineItem = None
self.image = QImage()
self.setScene(QGraphicsScene(self))
self.setTransformationAnchor(QGraphicsView.AnchorUnderMouse)
self.setDragMode(QGraphicsView.ScrollHandDrag)
self.setViewportUpdateMode(QGraphicsView.FullViewportUpdate)
# Prepare background check-board pattern.
tilePixmap = QPixmap(64, 64)
tilePixmap.fill(Qt.white)
tilePainter = QPainter(tilePixmap)
color = QColor(220, 220, 220)
tilePainter.fillRect(0, 0, 32, 32, color)
tilePainter.fillRect(32, 32, 32, 32, color)
tilePainter.end()
self.setBackgroundBrush(QBrush(tilePixmap))
def drawBackground(self, p, rect):
p.save()
p.resetTransform()
p.drawTiledPixmap(self.viewport().rect(),
self.backgroundBrush().texture())
p.restore()
def openFile(self, svg_file):
if not svg_file.exists():
return
s = self.scene()
if self.backgroundItem:
drawBackground = self.backgroundItem.isVisible()
else:
drawBackground = False
if self.outlineItem:
drawOutline = self.outlineItem.isVisible()
else:
drawOutline = True
s.clear()
self.resetTransform()
self.svgItem = QGraphicsSvgItem(svg_file.fileName())
self.svgItem.setFlags(QGraphicsItem.ItemClipsToShape)
self.svgItem.setCacheMode(QGraphicsItem.NoCache)
self.svgItem.setZValue(0)
self.backgroundItem = QGraphicsRectItem(self.svgItem.boundingRect())
self.backgroundItem.setBrush(Qt.white)
self.backgroundItem.setPen(QPen(Qt.NoPen))
self.backgroundItem.setVisible(drawBackground)
self.backgroundItem.setZValue(-1)
self.outlineItem = QGraphicsRectItem(self.svgItem.boundingRect())
outline = QPen(Qt.black, 2, Qt.DashLine)
outline.setCosmetic(True)
self.outlineItem.setPen(outline)
self.outlineItem.setBrush(QBrush(Qt.NoBrush))
self.outlineItem.setVisible(drawOutline)
self.outlineItem.setZValue(1)
s.addItem(self.backgroundItem)
s.addItem(self.svgItem)
s.addItem(self.outlineItem)
s.setSceneRect(self.outlineItem.boundingRect().adjusted(-10, -10, 10, 10))
def setRenderer(self, renderer):
self.renderer = renderer
if self.renderer == SvgView.OpenGL:
if QGLFormat.hasOpenGL():
self.setViewport(QGLWidget(QGLFormat(QGL.SampleBuffers)))
else:
self.setViewport(QWidget())
def setHighQualityAntialiasing(self, highQualityAntialiasing):
if QGLFormat.hasOpenGL():
self.setRenderHint(QPainter.HighQualityAntialiasing,
highQualityAntialiasing)
def setViewBackground(self, enable):
if self.backgroundItem:
self.backgroundItem.setVisible(enable)
def setViewOutline(self, enable):
if self.outlineItem:
self.outlineItem.setVisible(enable)
def paintEvent(self, event):
if self.renderer == SvgView.Image:
if self.image.size() != self.viewport().size():
self.image = QImage(self.viewport().size(),
QImage.Format_ARGB32_Premultiplied)
imagePainter = QPainter(self.image)
QGraphicsView.render(self, imagePainter)
imagePainter.end()
p = QPainter(self.viewport())
p.drawImage(0, 0, self.image)
else:
super(SvgView, self).paintEvent(event)
def wheelEvent(self, event):
factor = pow(1.2, event.angleDelta().y() / 240.0)
self.scale(factor, factor)
event.accept()
if __name__ == '__main__':
import sys
app = QApplication(sys.argv)
window = MainWindow()
if len(sys.argv) == 2:
window.openFile(sys.argv[1])
else:
window.openFile(':/files/bubbles.svg')
window.show()
sys.exit(app.exec_())
あとは起動して、マウスでホイールをいじってみてください。
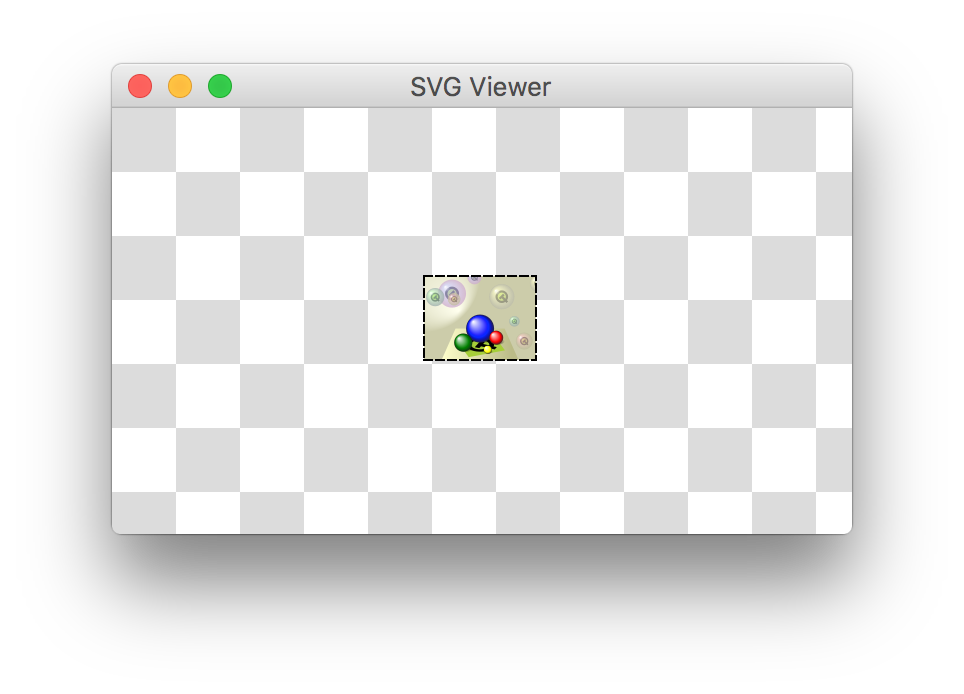

