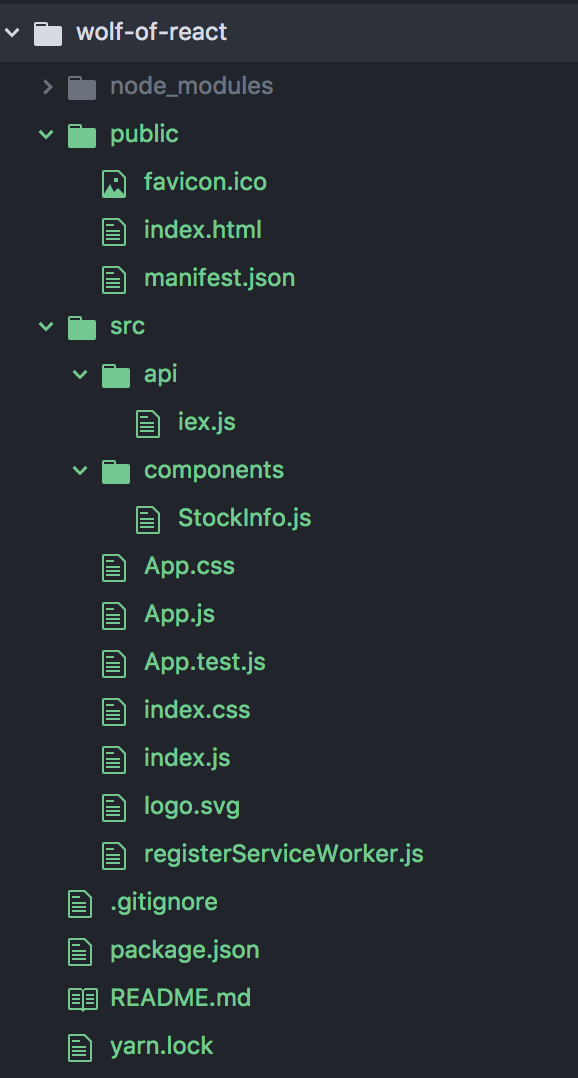

Create the new application called wolf-of-react
The axios is needed to call an API.
$ yarn create react-app wolf-of-react
$ yarn add axios
You can delete unnecessary parts like this.
public/index.html
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="utf-8">
<meta name="viewport" content="width=device-width, initial-scale=1, shrink-to-fit=no">
<meta name="theme-color" content="#000000">
<link rel="manifest" href="%PUBLIC_URL%/manifest.json">
<link rel="shortcut icon" href="%PUBLIC_URL%/favicon.ico">
<title>React App</title>
</head>
<body>
<div id="root"></div>
</body>
</html>
src/App.js
import React, { Component } from 'react';
import './App.css';
import StockInfo from './components/StockInfo';
import { loadQuoteForStock, loadCompanyLogoForStock } from './api/iex';
class App extends Component {
state = {
symbol: 'F',
quote: null
}
componentDidMount(){
this.loadQuote()
}
loadQuote() {
loadQuoteForStock(this.state.symbol)
.then((quote)=> {
console.log(quote)
this.setState({quote: quote})
})
.catch((err)=> {console.log(err)})
}
handleSymbolChange =(event)=>{
const target = event.target;
const symbol = target.value;
this.setState({symbol: symbol });
console.log(event);
}
handleButtonClick = (event) => {
console.log(event.target);
this.loadQuote();
}
render() {
return (
<div className="App">
<h1>Wolf of React</h1>
<input
value={this.state.symbol}
placeholder="Enter symbol"
onChange={this.handleSymbolChange}
/>
<button onClick={ this.handleButtonClick }>Get Quote</button>
<StockInfo {...this.state.quote}/>
</div>
)
}
}
export default App;
Create the components folder in the src folder.
And create the StockInfo.js file in the /src/components folder.
src/components/StockInfo.js
import React from 'react';
function StockInfo({
symbol,
companyName,
primaryExchange,
latestPrice,
latestSource,
week52High,
week52Low
}){
return(
<div>
<h2>{ symbol }: { companyName }</h2>
<h3>{ latestPrice } ({ latestSource} )</h3>
<dl>
<dt>Week 52 High</dt>
<dd>{ week52High }</dd>
<dt>Week 52 Low</dt>
<dd>{ week52Low }</dd>
<dt>Exchange</dt>
<dd>{ primaryExchange }</dd>
</dl>
</div>
)
}
export default StockInfo;
Create the folder called api and the iex.js file in it.
src/api/iex.js
import axios from 'axios';
const api = axios.create({
baseURL: 'https://api.iextrading.com/1.0'
});
export function loadQuoteForStock(symbol){
return api.get(`/stock/${symbol}/quote`)
.then((res)=>{
return res.data
});
}
$ yarn run start