目的
今回はSwift5でダイアログを表示させる方法を展開します。
開発環境
swift5
Xcode10.2
表示させる物
アクションスタイルがデフォルト
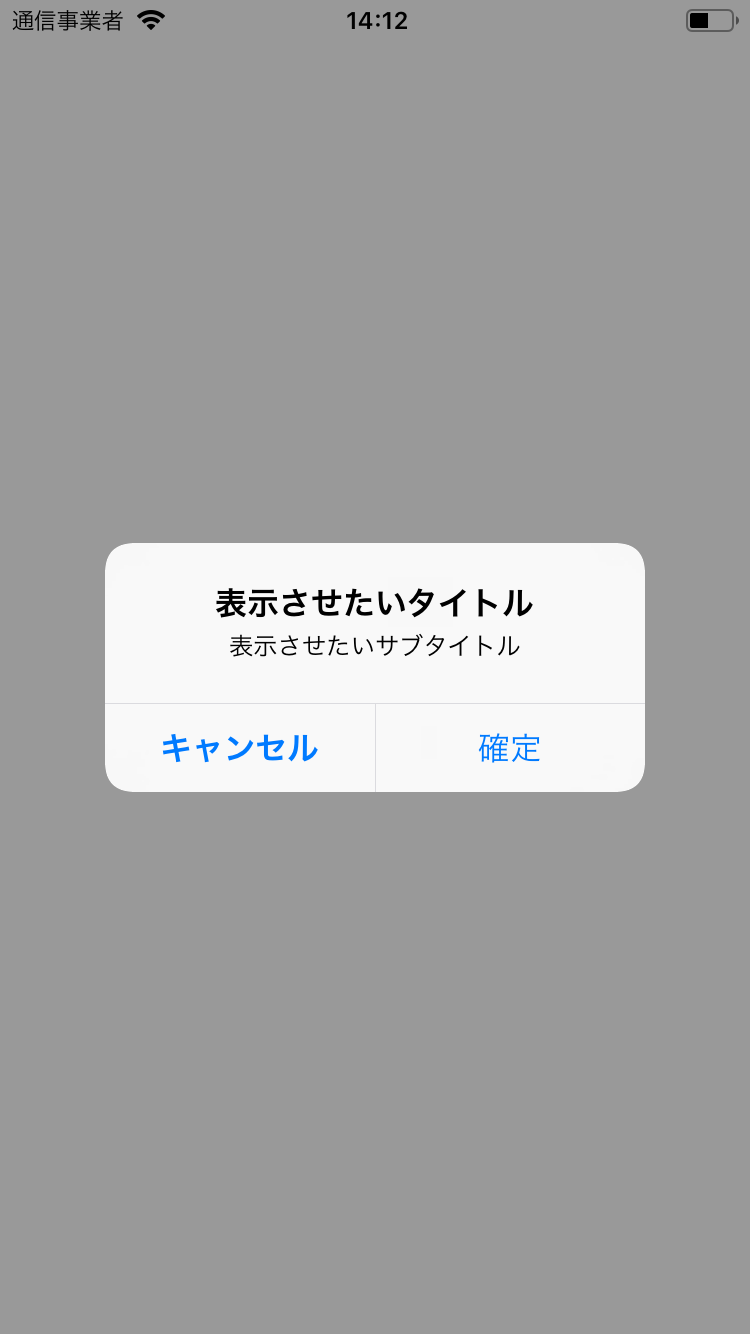
実装
アクションスタイルがデフォルト
Test.swift
//アラート生成
//UIAlertControllerのスタイルがalert
let alert: UIAlertController = UIAlertController(title: "表示させたいタイトル", message: "表示させたいサブタイトル", preferredStyle: UIAlertController.Style.alert)
// 確定ボタンの処理
let confirmAction: UIAlertAction = UIAlertAction(title: "確定", style: UIAlertAction.Style.default, handler:{
// 確定ボタンが押された時の処理をクロージャ実装する
(action: UIAlertAction!) -> Void in
//実際の処理
print("確定")
})
// キャンセルボタンの処理
let cancelAction: UIAlertAction = UIAlertAction(title: "キャンセル", style: UIAlertAction.Style.cancel, handler:{
// キャンセルボタンが押された時の処理をクロージャ実装する
(action: UIAlertAction!) -> Void in
//実際の処理
print("キャンセル")
})
//UIAlertControllerにキャンセルボタンと確定ボタンをActionを追加
alert.addAction(cancelAction)
alert.addAction(confirmAction)
//実際にAlertを表示する
present(alert, animated: true, completion: nil)
アクションスタイルがアクションシート
Test.swift
//アラート生成
//UIAlertControllerのスタイルがactionSheet
let actionSheet = UIAlertController(title: "Menu", message: "", preferredStyle: UIAlertController.Style.actionSheet)
// 表示させたいタイトル1ボタンが押された時の処理をクロージャ実装する
let action1 = UIAlertAction(title: "表示させたいタイトル1", style: UIAlertAction.Style.default, handler: {
(action: UIAlertAction!) in
//実際の処理
print("表示させたいタイトル1の処理")
})
// 表示させたいタイトル2ボタンが押された時の処理をクロージャ実装する
let action2 = UIAlertAction(title: "表示させたいタイトル2", style: UIAlertAction.Style.default, handler: {
(action: UIAlertAction!) in
//実際の処理
print("表示させたいタイトル2の処理")
})
// 閉じるボタンが押された時の処理をクロージャ実装する
//UIAlertActionのスタイルがCancelなので赤く表示される
let close = UIAlertAction(title: "閉じる", style: UIAlertAction.Style.destructive, handler: {
(action: UIAlertAction!) in
//実際の処理
print("閉じる")
})
//UIAlertControllerにタイトル1ボタンとタイトル2ボタンと閉じるボタンをActionを追加
actionSheet.addAction(action1)
actionSheet.addAction(action2)
actionSheet.addAction(close)
//実際にAlertを表示する
self.present(actionSheet, animated: true, completion: nil)
ボタンの処理なしでもかける!
上の2つはボタンを押した時の処理を書いてますがユーザーに対して、ただ周知したい時はもう少しスマートにかけます。
Test.swift
//アラートのタイトル
let dialog = UIAlertController(title: "タイトル", message: "サブタイトル", preferredStyle: .alert)
//ボタンのタイトル
dialog.addAction(UIAlertAction(title: "OK", style: .default, handler: nil))
//実際に表示させる
self.present(dialog, animated: true, completion: nil)