Model
Member
名前、メールアドレス、電話番号などの投稿者メンバーの情報を保管する。
package models;
import javax.persistence.*;
import com.avaje.ebean.annotation.*;
import play.db.ebean.*;
import play.db.ebean.Model.Finder;
import java.util.*;
import play.data.validation.Constraints.*;
@Entity
public class Member extends Model {
@Id
public Long id;
@Required(message = "必須項目です。")
public String name;
@Email(message = "メールアドレスを記入ください。")
public String mail;
public String tel;
public static Finder<Long, Member> find = new Finder<Long, Member>(Long.class, Member.class);
@Override
public String toString() {
return ("[id:" + id + ",name:" + name + ",mail:" + mail + ",tel:" + tel + "]");
}
public static Member findByName(String input) {
return Member.find.where()
.eq("name", input).findList().get(0);
}
}
Message
投稿者名、投稿メッセージ、作成日時をなどの情報を保管する。
package models;
import java.util.*;
import javax.persistence.*;
import com.avaje.ebean.annotation.*;
import play.db.ebean.*;
import play.data.validation.Constraints.*;
@Entity
public class Message extends Model {
@Id
public Long id;
@Required(message = "必須項目です。")
public String name;
@Required(message = "必須項目です。")
public String message;
@CreatedTimestamp
public Date postdate;
public static Finder<Long, Message> find = new Finder<Long, Message>(Long.class, Message.class);
@Override
public String toString() {
return ("[id:" + id + ",name:" + name + ",mail:" + mail + ",message:" + message + ",date:" + postdate + "]");
}
public static Message findByName(String input) {
return Member.find.where()
.eq("name", input).findList().get(0);
}
}
補足
●なぜModelの中にメソッドを記述するのか
Aplicationに記載するという手段もあったが、Model関係の機能はModelに記述を統一するため。
●findByNameとは
引数に指定した、nameのMessageを返すメソッド。
●findByNameメソッドの「eq」とは
変数=<ExpressionList>.eq(フィールド名,値)
引数に指定した、「フィールド」の中で、「値」と等しいものをExpressionListとして返す。
●findByNameメソッドの「where」と「findList」について
where:ExpressionListを取り出す。
findList:ExpressionListに保管されているエンティティをリストとして取り出す。
※なぜ一度、「ExpressionList」で取り出すのか
→ExpressionListは、リストの要素を絞り込んだり、並びを調整したりするメソッドが用意されているため。
●Finderクラスのインスタンス作成
new Finder<プライマリキー、エンティティ>=(プライマリキー.class、エンティティ.class);
こうして作成したインスタンスをスタティックに保管し、必要に応じてそのメソッドでエンティティを取り出す。
route
GET /assets/*file controllers.Assets.at(path="/public", file)
GET / controllers.Application.index()
GET /add controllers.Application.add()
POST /create controllers.Application.create()
GET /add2 controllers.Application.add2()
POST /create2 controllers.Application.create2()
View
add
@(msg:String,form1:Form[models.Message])
@main("Sample Page"){
<h1>ADD MESSAGE</h1>
<p>@msg</p>
@helper.form(action = routes.Application.create){
@(helper.inputText(
field = form1("name")
))
@(helper.textarea(
field = form1("message")
))
<input type="submit">
}
}
add2
@(msg:String,form1:Form[models.Message])
@main("Sample Page"){
<h1>ADD MEMBER</h1>
<p>@msg</p>
@helper.form(action = routes.Application.create2){
@(helper.inputText(
field = form1("name")
))
@(helper.inputText(
field = form1("mail")
))
@(helper.inputText(
field = form1("tel")
))
<input type="submit">
}
}
補足
●helper.inputTextとは
helperパッケージのinputTextオブジェクトの呼び出し。
controllers
Aplication
package controllers;
import models.*;
import java.util.*;
import com.avaje.ebean.ExpressionList;
import play.*;
import play.data.*;
import play.data.validation.Constraints.Required;
import play.db.ebean.*;
import play.mvc.*;
import views.html.*;
public class Application extends Controller {
//ルートにアクセスした際のAction
public static Result index() {
List<Message> datas = Message.find.all();
List<Member> datas2 = Member.find.all();
return ok(index.render("データベースのサンプル", datas, datas2));
}
//Message Action =============================
//新規フォームのAction
public static Result add() {
Form<Message> f = new Form(Message.class);
return ok(add.render("投稿フォーム", f));
}
// /createにアクセスした際のアクション
public static Result create() {
Form<Message> f = new Form(Message.class).bindFromRequest();
if (!f.hasErrors()) {
Message data = f.get();
data.save();
return redirect("/");
} else {
return ok(add.render("ERROR", f));
}
}
//Member Action =============================
//メンバー作成フォームのAction
public static Result add2() {
Form<Member> f = new Form(Member.class);
return ok(add2.render("メンバー登録フォーム", f));
}
// /create2にアクセスした際のAction
public static Result create2() {
Form<Member> f = new Form(Member.class).bindFromRequest();
if (!f.hasErrors()) {
Member data = f.get();
data.save();
return redirect("/");
} else {
return ok(add2.render("ERROR", f));
}
}
}
画面
「home画面」
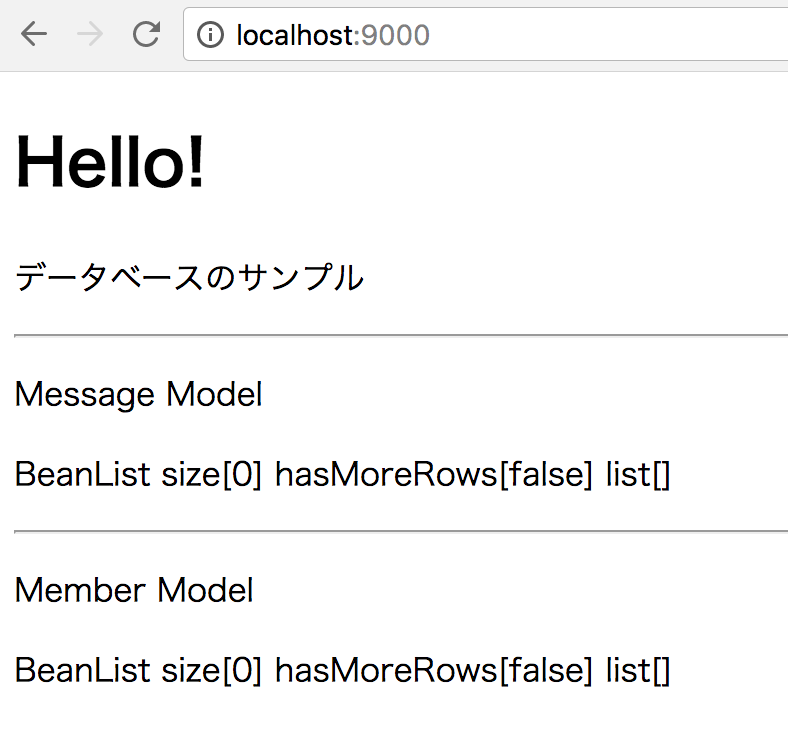
「add」
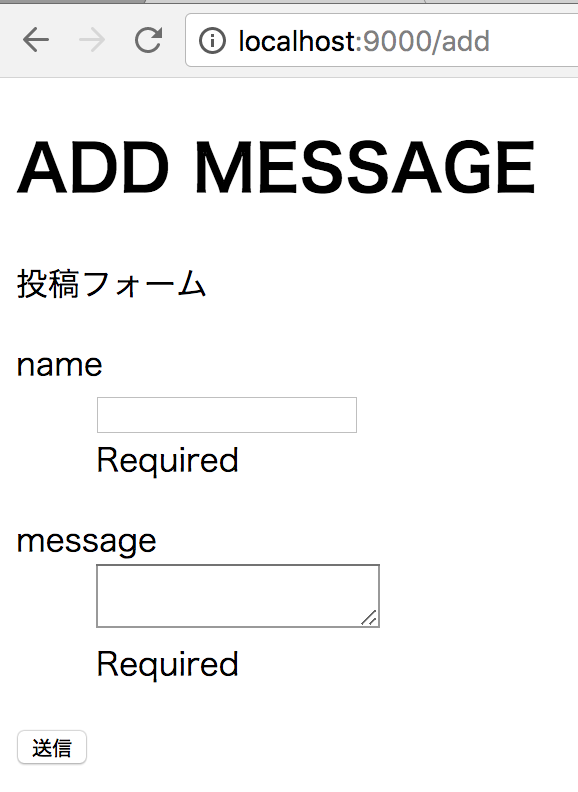
「add2」
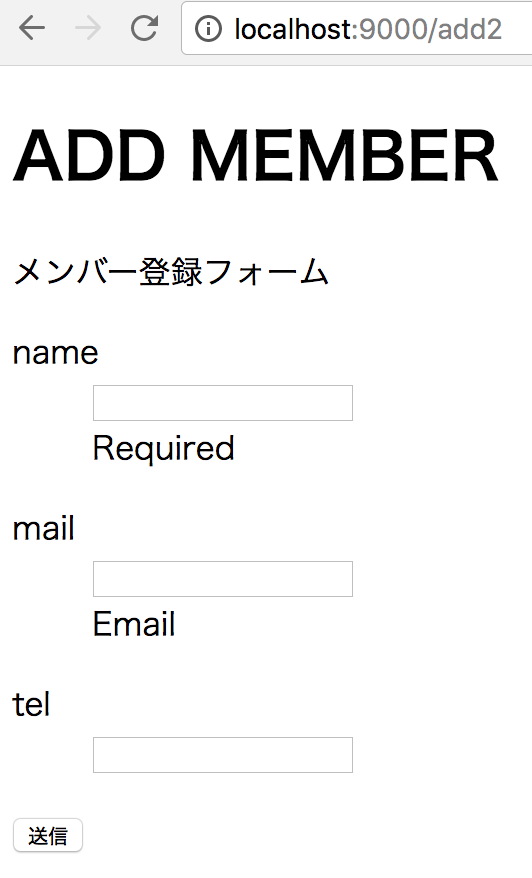