Flutterアプリの新規作成時にできているでもアプリをBLoCパターンで書き直してみました。
BLoCの詳しい説明はここ
https://www.youtube.com/watch?v=PLHln7wHgPE&app=desktop
BLoCパターンとは
BLoCとはBusiness Logic Componentの略で、BLoCを使ったアプリの実装パターンをblocパターンと呼ぶ。
コンポーネントと言っても、viewを構築するコンポーネントではなく、「ひとかたまりの要素」という意味でのコンポーネントである。
以下の方針に従って開発を進めればBLoCパターンであると言える。
- BLoCの入出力インターフェースはStream,Sinkでやる
- BLoCの依存は注入可能で環境に依存しない
- BLoC内に環境ごとの条件分岐は持たない
- 以上のルールに基づくなら実装は自由
BLoCで実装
新規作成時にできている以下のアプリをBLoCパターンにしていく
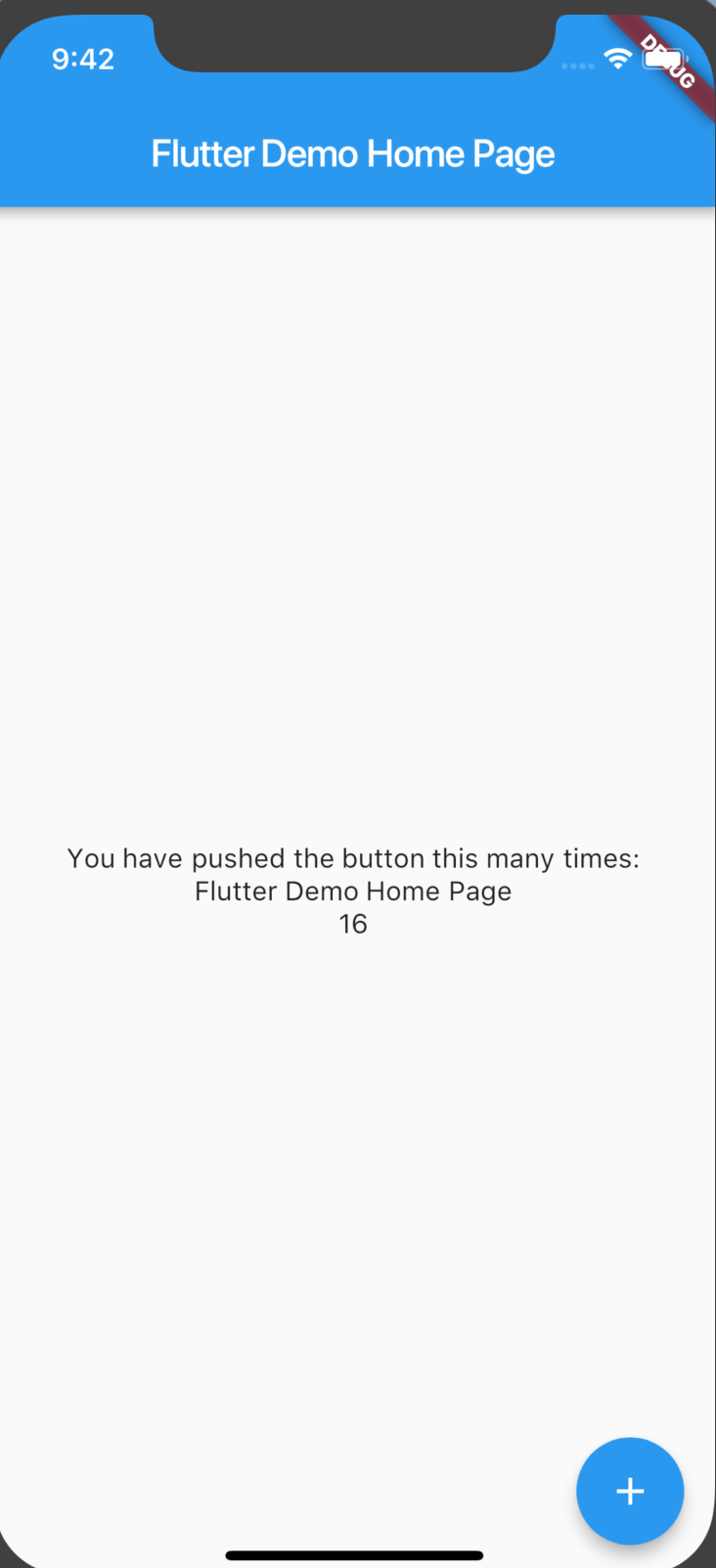
main.dart
import 'package:flutter/material.dart';
import 'dart:async';
import 'package:rxdart/rxdart.dart';
// BLoCオブジェクト作成
class CounterBloc {
StreamController counterAdditionController = StreamController();
Sink get counterAddition => counterAdditionController.sink;
BehaviorSubject<int> _count = BehaviorSubject<int>(seedValue: 0);
Stream<int> get countString => _count.stream;
CounterBloc(){
counterAdditionController.stream.listen( (addition) {
_count.add(_count.value + 1);
});
}
}
void main() => runApp(new MyApp());
class MyApp extends StatelessWidget {
@override
Widget build(BuildContext context) {
return new MaterialApp(
title: 'Flutter Demo',
theme: new ThemeData(
primarySwatch: Colors.blue,
),
home: new MyHomePage(title: 'Flutter Demo Home Page'),
);
}
}
class MyHomePage extends StatelessWidget {
String title;
MyHomePage({this.title});
CounterBloc counterBloc = CounterBloc();
@override
Widget build(BuildContext context) {
return new Scaffold(
appBar: new AppBar(
title: new Text(title),
),
body: new Center(
child: new Column(
mainAxisAlignment: MainAxisAlignment.center,
children: <Widget>[
new Text(
'You have pushed the button this many times:',
),
// データストリームから現在のカウントを取得
StreamBuilder<int>(
stream: counterBloc.countString,
builder: (context, snapshot) =>
Text(
snapshot.data.toString(),
),
),
],
),
),
floatingActionButton: new FloatingActionButton(
// ボタンが押された時Streamに通知
onPressed: () => counterBloc.counterAddition.add(null),
tooltip: 'Increment',
child: new Icon(Icons.add),
),
);
}
}