どうもjackです。
8月からPythonをメインにエンジニア業務に従事することになりまして。
諸事情により12月までに7キロの減量を目標に生きてる駆け出しエンジニアです。
今回は表題の通りDjangoのCRUDで体重を記録したいと思います。
私の開発環境は以下となっています。
- Python 3.6.7
- Django 2.2.3
- macOS
では、早速。
前回、既にqiitaディレクトリを作成しているのでその中に体重計管理アプリを作成していきます。
python3 manage.py startapp weight
作成したweightアプリの存在をsettings.pyに編集。
INSTALLED_APPS = [
'django.contrib.admin',
'django.contrib.auth',
'django.contrib.contenttypes',
'django.contrib.sessions',
'django.contrib.messages',
'django.contrib.staticfiles',
'weight',
今回の入力値は「体重」と「日付」です。
従ってweightディレクトリにあるmodels.pyにこれらを入力していきます。
from django.db import models
from django.utils import timezone
# Create your models here.
class Weight(models.Model):
weight = models.FloatField(default=0)
date = models.DateField(default=timezone.now)
入力が終わったらデータベースの作成を行います。
python3 manage.py makemigrations
python3 manage.py migrate
この作成したモデルをもとに入力フォームを作成していきます。
その為にweightアプリのディレクトリにforms.pyファイルを作成します。
├── qiita
│ ├── __pycache__
│ ├── _init_.py
│ ├── setting.py
│ ├── urls.py
│ └── wsgi.py
├── weight
│ ├── migrations
│ ├── _init_.py
│ ├── admin.py
│ ├── apps.py
│ ├── forms.py ←これ
│ ├── models.py
│ ├── tests.py
│ └── views.py
└── manage.py
編集内容は以下の通りです。
from django import forms
from .models import Weight
class WeightForm(forms.ModelForm):
class Meta:
model = Weight
fields = ['weight', 'date']
次にviews.pyの編集を行います。
from django.shortcuts import render, redirect
from .models import Weight
from .forms import WeightForm
# Create your views here.
def index(request):
data = Weight.objects.all()
params = {
'title' : 'my weight memory',
'data' : data
}
return render(request, 'weight/index.html', params)
def create(request):
params = {
'title': 'score',
'form': WeightForm()
}
if (request.method=='POST'):
obj = Weight()
weight = WeightForm(request.POST, instance=obj)
weight.save()
return redirect(to='/weight')
return render(request, 'weight/create.html', params)
def edit(request, num):
obj = Weight.objects.get(id=num)
if (request.method=='POST'):
weight = WeightForm(request.POST, instance=obj)
weight.save()
return redirect(to='/weight')
params = {
'title' : 'edit',
'id':num,
'msg': '改ざんすなよ',
'form': WeightForm(instance=obj)
}
return render(request, 'weight/edit.html', params)
def delete(request, num):
weight = Weight.objects.get(id=num)
if (request.method=='POST'):
weight.delete()
return redirect(to='/weight')
params = {
'title': 'delete',
'msg': 'ほんまに消してええの?',
'id':num,
'obj':weight
}
return render(request, 'weight/delete.html', params)
そしてqiitaディレクトリにあるurls.pyの編集を行います。
from django.contrib import admin
from django.urls import path, include
urlpatterns = [
path('admin/', admin.site.urls),
path('weight/', include('weight.urls')),
]
次にweightアプリのディレクトリにurls.pyファイルを作成して、編集します。
├── qiita
│ ├── __pycache__
│ ├── _init_.py
│ ├── setting.py
│ ├── urls.py
│ └── wsgi.py
├── weight
│ ├── migrations
│ ├── _init_.py
│ ├── admin.py
│ ├── apps.py
│ ├── forms.py
│ ├── models.py
│ ├── tests.py
│ ├── urls.py ←これ
│ └── views.py
└── manage.py
編集内容は以下の通りです。
from django.urls import path
from . import views
urlpatterns = [
path('', views.index, name='index'),
path('create', views.create, name='create'),
path('edit/<int:num>', views.edit, name='edit'),
path('delete/<int:num>', views.delete, name='delete'),
]
次にテンプレートディレクトリを作成してその中にアプリ名と同じディレクトリを作成し、
htmlを格納していきます。
├── qiita
│ ├── __pycache__
│ ├── _init_.py
│ ├── setting.py
│ ├── urls.py
│ └── wsgi.py
├── weight
│ ├── migrations
│ ├── templates
│ │ └── weight
│ │ ├── index.html ←こいつら
│ │ ├── create.html←こいつら
│ │ ├── delete.html←こいつら
│ │ └── edit.html ←こいつら
│ ├── _init_.py
│ ├── admin.py
│ ├── apps.py
│ ├── forms.py
│ ├── models.py
│ ├── tests.py
│ ├── urls.py
│ └── views.py
└── manage.py
作成したhtmlを編集していきます。
{% load static %}
<!DOCTYPE html>
<html lang="ja" dir="ltr">
<head>
<meta charset="utf-8">
<link rel="stylesheet" type="text/css"
href="{% static 'weight/css/style.css'%}">
<title>{{title}}</title>
</head>
<body>
<table>
<tr>
<th>ID</th>
<th>Weight</th>
<th>date</th>
<th>編集</th>
<th>削除</th>
</tr>
{% for item in data %}
<tr>
<td>{{item.id}}</td>
<td>{{item.weight}}</td>
<td>{{item.date}}</td>
<td><a href="{% url 'edit' item.id %}">edit</a></td>
<td><a href="{% url 'delete' item.id %}">delete</a></td>
</tr>
{% endfor %}
</table>
<a href="{% url 'create'%}">体重を記録する</a>
</body>
</html>
{% load static %}
<!DOCTYPE html>
<html lang="ja" dir="ltr">
<head>
<meta charset="utf-8">
<link rel="stylesheet" type="text/css"
href="{% static 'weigth/css/style.css'%}">
<title>{{title}}</title>
</head>
<body>
<h1>{{title}}</h1>
<form action="{% url 'create' %}" method="post">
{% csrf_token%}
{{ form.as_table}}
<tr>
<td></td><td><input type="submit" name="" value="send"></td>
</tr>
</form>
<a href="{% url 'index'%}">indexへ戻る</a>
</body>
</html>
{% load static%}
<!DOCTYPE html>
<html lang="ja" dir="ltr">
<head>
<meta charset="utf-8">
<link rel="stylesheet" type="text/css"
href="{% static 'weight/css/style.css'%}">
<title>{{title}}</title>
</head>
<body>
<h1>{{title}}</h1>
<h2>{{msg}}</h2>
<table>
<tr>
<th>ID</th><td>{{obj.id}}</td>
</tr>
<tr>
<th>Weight</th><td>{{obj.weight}}</td>
</tr>
<tr>
<th>Date</th><td>{{obj.date}}</td>
</tr>
<form action="{% url 'delete' id%}" method="post">
{% csrf_token %}
<tr>
<td><input type="submit" name="" value="削除"></td>
</tr>
</form>
</table>
</body>
</html>
{% load static%}
<!DOCTYPE html>
<html lang="ja" dir="ltr">
<head>
<meta charset="utf-8">
<link rel="stylesheet" type="text/css"
href="{% static 'weight/css/style.css'%}">
<title>{{title}}</title>
</head>
<body>
<h1>{{title}}</h1>
<h2>{{msg}}</h2>
<table>
<form action="{% url 'edit' id %}" method="post">
{% csrf_token%}
{{ form.as_table}}
<tr>
<td></td><td><input type="submit" name="" value="send"></td>
</tr>
</form>
</table>
</body>
</html>
最後にcssを格納する為のディレクトリを作成してstyle.cssを編集します。
├── qiita
│ ├── __pycache__
│ ├── _init_.py
│ ├── setting.py
│ ├── urls.py
│ └── wsgi.py
├── weight
│ ├── migrations
│ ├── static
│ │ └── weight
│ │ └──css
│ │ └── style.css ← こいつ
│ ├── templates
│ │ └── weight
│ │ ├── index.html
│ │ ├── create.html
│ │ ├── delete.html
│ │ └── edit.html
│ ├── _init_.py
│ ├── admin.py
│ ├── apps.py
│ ├── forms.py
│ ├── models.py
│ ├── tests.py
│ ├── urls.py
│ └── views.py
└── manage.py
table {
margin: 10px;
font-size: 14px;
text-align: center;
}
table tr th {
background-color: #009;
color: white;
padding: 2px 10px;
border-width: 2px;
text-align: center;
}
table tr rd {
background-color: #eee;
color: #666;
padding: 2px 10px;
border-width: 2px;
text-align: center;
}
ではローカルに立ち上げて見てみましょう!

編集は?

削除は?

よしよし
ただ、日付が日本語じゃない、、、
LANGUAGE_CODE = 'ja'
TIME_ZONE = 'Asia/Tokyo'
USE_I18N = True
USE_L10N = True
USE_TZ = True
これでよし。
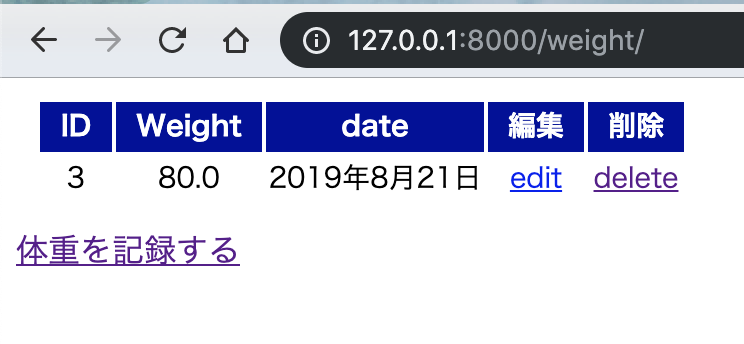
しっかり日本語表示できました!!
これで体重管理して痩せるぞーーーー!!